APIs
rerun.blueprint
BlueprintPart = Union[ContainerLike, TopPanel, BlueprintPanel, SelectionPanel, TimePanel]
module-attribute
The types that make up a blueprint.
ContainerLike = Union[Container, SpaceView]
module-attribute
A type that can be converted to a container.
These types all implement a to_container()
method that wraps them in the necessary
helper classes.
class Blueprint
The top-level description of the viewer blueprint.
def __init__(*parts, auto_layout=None, auto_space_views=None, collapse_panels=False)
Construct a new blueprint from the given parts.
Each BlueprintPart can be one of the following:
It is an error to provide more than one of instance of any of the panel types.
Blueprints only have a single top-level "root" container that defines the viewport.
If you provide multiple ContainerLike
instances, they will be combined under a single
root Tab
container.
PARAMETER | DESCRIPTION |
---|---|
*parts |
The parts of the blueprint.
TYPE:
|
auto_layout |
Whether to automatically layout the viewport. If
TYPE:
|
auto_space_views |
Whether to automatically add space views to the viewport. If
TYPE:
|
collapse_panels |
Whether to collapse panels in the viewer. Defaults to This fully hides the blueprint/selection panels, and shows the simplified time panel.
TYPE:
|
def connect(application_id, *, addr=None, make_active=True, make_default=True)
Connect to a remote Rerun Viewer on the given ip:port and send this blueprint.
PARAMETER | DESCRIPTION |
---|---|
application_id |
The application ID to use for this blueprint. This must match the application ID used when initiating rerun for any data logging you wish to associate with this blueprint.
TYPE:
|
addr |
The ip:port to connect to
TYPE:
|
make_active |
Immediately make this the active blueprint for the associated
TYPE:
|
make_default |
Make this the default blueprint for the
TYPE:
|
def save(application_id, path=None)
Save this blueprint to a file. Rerun recommends the .rbl
suffix.
PARAMETER | DESCRIPTION |
---|---|
application_id |
The application ID to use for this blueprint. This must match the application ID used when initiating rerun for any data logging you wish to associate with this blueprint.
TYPE:
|
path |
The path to save the blueprint to. Defaults to
TYPE:
|
def spawn(application_id, port=9876, memory_limit='75%', hide_welcome_screen=False)
Spawn a Rerun viewer with this blueprint.
PARAMETER | DESCRIPTION |
---|---|
application_id |
The application ID to use for this blueprint. This must match the application ID used when initiating rerun for any data logging you wish to associate with this blueprint.
TYPE:
|
port |
The port to listen on.
TYPE:
|
memory_limit |
An upper limit on how much memory the Rerun Viewer should use.
When this limit is reached, Rerun will drop the oldest data.
Example:
TYPE:
|
hide_welcome_screen |
Hide the normal Rerun welcome screen.
TYPE:
|
def to_blueprint()
Conform with the BlueprintLike
interface.
class Container
Base class for all container types.
Consider using one of the subclasses instead of this class directly:
These are ergonomic helpers on top of rerun.blueprint.archetypes.ContainerBlueprint.
def __init__(*args, contents=None, kind, column_shares=None, row_shares=None, grid_columns=None, active_tab=None, name)
Construct a new container.
PARAMETER | DESCRIPTION |
---|---|
*args |
All positional arguments are forwarded to the |
contents |
The contents of the container. Each item in the iterable must be a
TYPE:
|
kind |
The kind of the container. This must correspond to a known container kind.
Prefer to use one of the subclasses of
TYPE:
|
column_shares |
The layout shares of the columns in the container. The share is used to determine what fraction of the total width each
column should take up. The column with index
TYPE:
|
row_shares |
The layout shares of the rows in the container. The share is used to determine what fraction of the total height each
row should take up. The row with index
TYPE:
|
grid_columns |
The number of columns in the grid. This is only applicable to |
active_tab |
The active tab in the container. This is only applicable to |
name |
The name of the container
TYPE:
|
def blueprint_path()
The blueprint path where this space view will be logged.
Note that although this is an EntityPath
, is scoped to the blueprint tree and
not a part of the regular data hierarchy.
def to_blueprint()
Convert this container to a full blueprint.
def to_container()
Convert this space view to a container.
class Horizontal
Bases: Container
A horizontal container.
def __init__(*args, contents=None, column_shares=None, name=None)
Construct a new horizontal container.
PARAMETER | DESCRIPTION |
---|---|
*args |
All positional arguments are forwarded to the |
contents |
The contents of the container. Each item in the iterable must be a
TYPE:
|
column_shares |
The layout shares of the columns in the container. The share is used to determine what fraction of the total width each
column should take up. The column with index
TYPE:
|
name |
The name of the container
TYPE:
|
def blueprint_path()
The blueprint path where this space view will be logged.
Note that although this is an EntityPath
, is scoped to the blueprint tree and
not a part of the regular data hierarchy.
def to_blueprint()
Convert this container to a full blueprint.
def to_container()
Convert this space view to a container.
class Vertical
Bases: Container
A vertical container.
def __init__(*args, contents=None, row_shares=None, name=None)
Construct a new vertical container.
PARAMETER | DESCRIPTION |
---|---|
*args |
All positional arguments are forwarded to the |
contents |
The contents of the container. Each item in the iterable must be a
TYPE:
|
row_shares |
The layout shares of the rows in the container. The share is used to determine what fraction of the total height each
row should take up. The row with index
TYPE:
|
name |
The name of the container
TYPE:
|
def blueprint_path()
The blueprint path where this space view will be logged.
Note that although this is an EntityPath
, is scoped to the blueprint tree and
not a part of the regular data hierarchy.
def to_blueprint()
Convert this container to a full blueprint.
def to_container()
Convert this space view to a container.
class Grid
Bases: Container
A grid container.
def __init__(*args, contents=None, column_shares=None, row_shares=None, grid_columns=None, name=None)
Construct a new grid container.
PARAMETER | DESCRIPTION |
---|---|
*args |
All positional arguments are forwarded to the |
contents |
The contents of the container. Each item in the iterable must be a
TYPE:
|
column_shares |
The layout shares of the columns in the container. The share is used to determine what fraction of the total width each
column should take up. The column with index
TYPE:
|
row_shares |
The layout shares of the rows in the container. The share is used to determine what fraction of the total height each
row should take up. The row with index
TYPE:
|
grid_columns |
The number of columns in the grid. |
name |
The name of the container
TYPE:
|
def blueprint_path()
The blueprint path where this space view will be logged.
Note that although this is an EntityPath
, is scoped to the blueprint tree and
not a part of the regular data hierarchy.
def to_blueprint()
Convert this container to a full blueprint.
def to_container()
Convert this space view to a container.
class Tabs
Bases: Container
A tab container.
def __init__(*args, contents=None, active_tab=None, name=None)
Construct a new tab container.
PARAMETER | DESCRIPTION |
---|---|
*args |
All positional arguments are forwarded to the |
contents |
The contents of the container. Each item in the iterable must be a
TYPE:
|
active_tab |
The index or name of the active tab. |
name |
The name of the container
TYPE:
|
def blueprint_path()
The blueprint path where this space view will be logged.
Note that although this is an EntityPath
, is scoped to the blueprint tree and
not a part of the regular data hierarchy.
def to_blueprint()
Convert this container to a full blueprint.
def to_container()
Convert this space view to a container.
class SpaceView
Base class for all space view types.
Consider using one of the subclasses instead of this class directly:
- rerun.blueprint.BarChartView
- rerun.blueprint.Spatial2DView
- rerun.blueprint.Spatial3DView
- rerun.blueprint.TensorView
- rerun.blueprint.TextDocumentView
- rerun.blueprint.TextLogView
- rerun.blueprint.TimeSeriesView
These are ergonomic helpers on top of rerun.blueprint.archetypes.SpaceViewBlueprint.
def __init__(*, class_identifier, origin, contents, name, visible=None, properties={}, defaults=[], overrides={})
Construct a blueprint for a new space view.
PARAMETER | DESCRIPTION |
---|---|
name |
The name of the space view.
TYPE:
|
class_identifier |
The class of the space view to add. This must correspond to a known space view class.
Prefer to use one of the subclasses of
TYPE:
|
origin |
The
TYPE:
|
contents |
The contents of the space view specified as a query expression. This is either a single expression, or a list of multiple expressions. See rerun.blueprint.archetypes.SpaceViewContents.
TYPE:
|
visible |
Whether this space view is visible. Defaults to true if not specified.
TYPE:
|
properties |
Dictionary of property archetypes to add to space view's internal hierarchy.
TYPE:
|
defaults |
List of default components or component batches to add to the space view. When an archetype in the view is missing a component included in this set, the value of default will be used instead of the normal fallback for the visualizer.
TYPE:
|
overrides |
Dictionary of overrides to apply to the space view. The key is the path to the entity where the override should be applied. The value is a list of component or component batches to apply to the entity. Important note: the path must be a fully qualified entity path starting at the root. The override paths
do not yet support
TYPE:
|
def blueprint_path()
The blueprint path where this space view will be logged.
Note that although this is an EntityPath
, is scoped to the blueprint tree and
not a part of the regular data hierarchy.
def to_blueprint()
Convert this space view to a full blueprint.
def to_container()
Convert this space view to a container.
class BarChartView
Bases: SpaceView
View: A bar chart view.
Example
Use a blueprint to create a BarChartView.:
import rerun as rr
import rerun.blueprint as rrb
rr.init("rerun_example_bar_chart", spawn=True)
# It's recommended to log bar charts with the `rr.BarChart` archetype,
# but single dimensional tensors can also be used if a `BarChartView` is created explicitly.
rr.log("tensor", rr.Tensor([8, 4, 0, 9, 1, 4, 1, 6, 9, 0]))
# Create a bar chart view to display the chart.
blueprint = rrb.Blueprint(rrb.BarChartView(origin="tensor", name="Bar Chart"), collapse_panels=True)
rr.send_blueprint(blueprint)
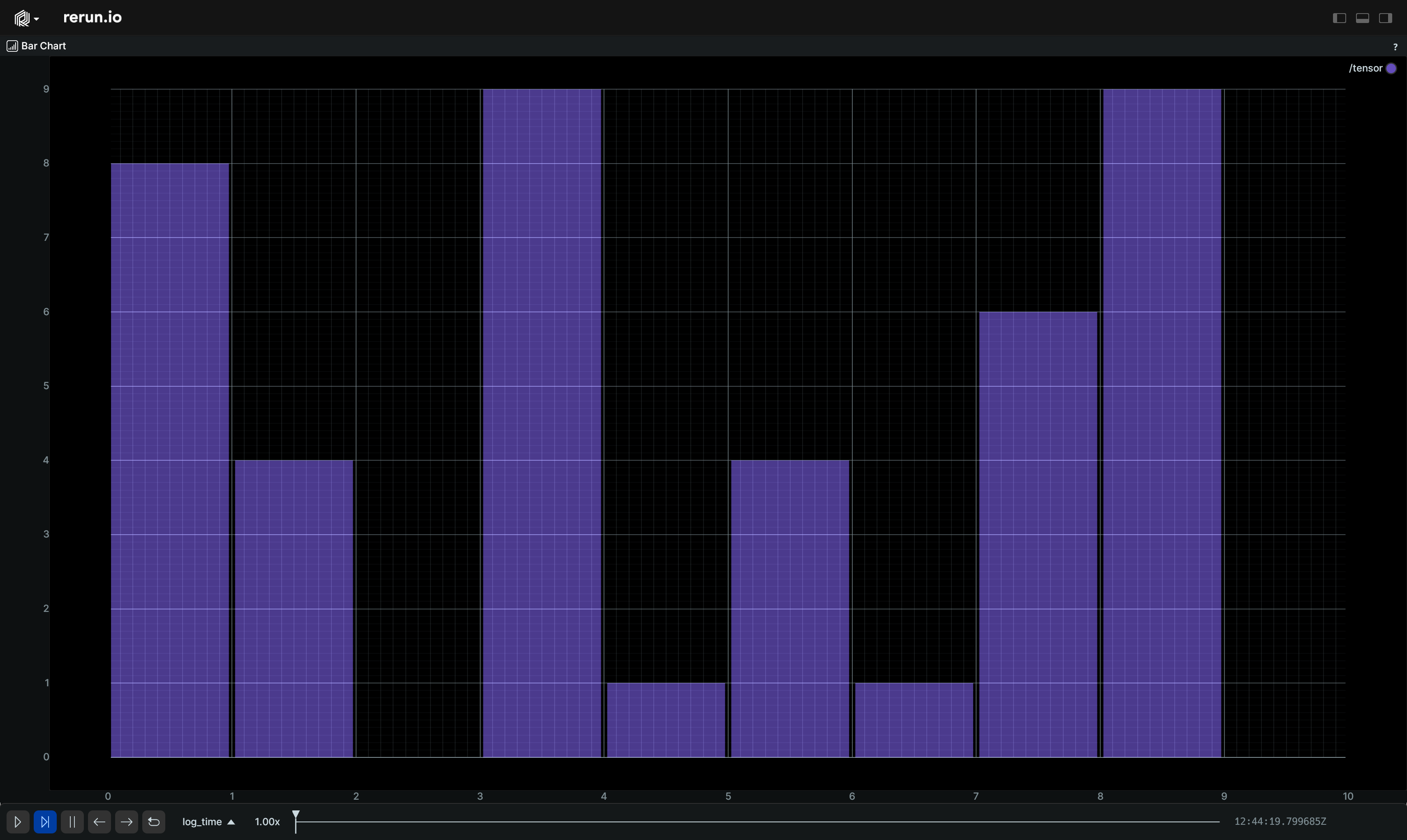
def __init__(*, origin='/', contents='$origin/**', name=None, visible=None, defaults=[], overrides={}, plot_legend=None)
Construct a blueprint for a new BarChartView view.
PARAMETER | DESCRIPTION |
---|---|
origin |
The
TYPE:
|
contents |
The contents of the view specified as a query expression. This is either a single expression, or a list of multiple expressions. See rerun.blueprint.archetypes.SpaceViewContents.
TYPE:
|
name |
The display name of the view.
TYPE:
|
visible |
Whether this view is visible. Defaults to true if not specified.
TYPE:
|
defaults |
List of default components or component batches to add to the space view. When an archetype in the view is missing a component included in this set, the value of default will be used instead of the normal fallback for the visualizer.
TYPE:
|
overrides |
Dictionary of overrides to apply to the space view. The key is the path to the entity where the override should be applied. The value is a list of component or component batches to apply to the entity. Important note: the path must be a fully qualified entity path starting at the root. The override paths
do not yet support
TYPE:
|
plot_legend |
Configures the legend of the plot.
TYPE:
|
def blueprint_path()
The blueprint path where this space view will be logged.
Note that although this is an EntityPath
, is scoped to the blueprint tree and
not a part of the regular data hierarchy.
def to_blueprint()
Convert this space view to a full blueprint.
def to_container()
Convert this space view to a container.
class Spatial2DView
Bases: SpaceView
View: For viewing spatial 2D data.
Example
Use a blueprint to customize a Spatial2DView.:
import numpy as np
import rerun as rr
import rerun.blueprint as rrb
rr.init("rerun_example_spatial_2d", spawn=True)
# Create a spiral of points:
n = 150
angle = np.linspace(0, 10 * np.pi, n)
spiral_radius = np.linspace(0.0, 3.0, n) ** 2
positions = np.column_stack((np.cos(angle) * spiral_radius, np.sin(angle) * spiral_radius))
colors = np.dstack((np.linspace(255, 255, n), np.linspace(255, 0, n), np.linspace(0, 255, n)))[0].astype(int)
radii = np.linspace(0.01, 0.7, n)
rr.log("points", rr.Points2D(positions, colors=colors, radii=radii))
# Create a Spatial2D view to display the points.
blueprint = rrb.Blueprint(
rrb.Spatial2DView(
origin="/",
name="2D Scene",
# Set the background color
background=[105, 20, 105],
# Note that this range is smaller than the range of the points,
# so some points will not be visible.
visual_bounds=rrb.VisualBounds2D(x_range=[-5, 5], y_range=[-5, 5]),
),
collapse_panels=True,
)
rr.send_blueprint(blueprint)
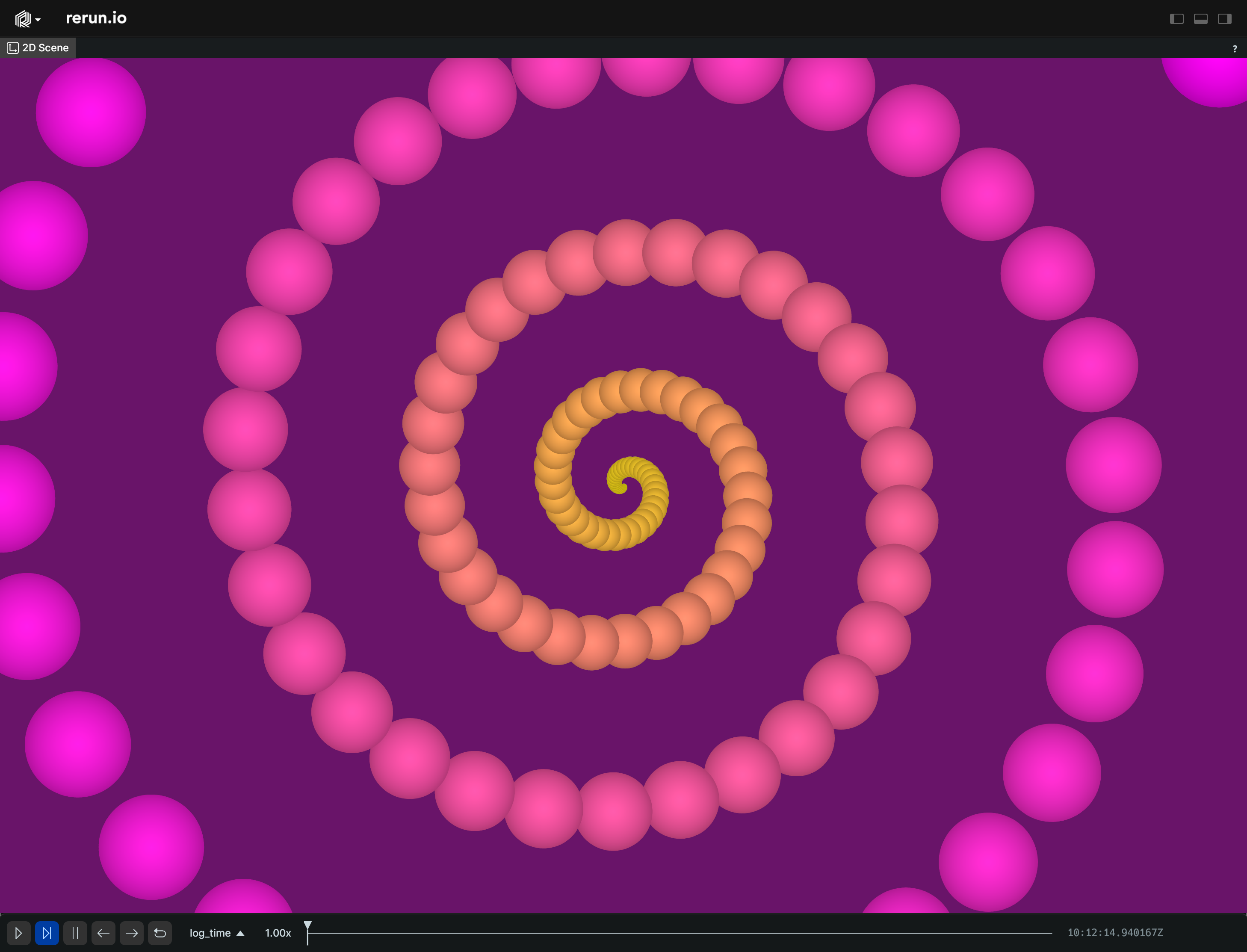
def __init__(*, origin='/', contents='$origin/**', name=None, visible=None, defaults=[], overrides={}, background=None, visual_bounds=None, time_ranges=None)
Construct a blueprint for a new Spatial2DView view.
PARAMETER | DESCRIPTION |
---|---|
origin |
The
TYPE:
|
contents |
The contents of the view specified as a query expression. This is either a single expression, or a list of multiple expressions. See rerun.blueprint.archetypes.SpaceViewContents.
TYPE:
|
name |
The display name of the view.
TYPE:
|
visible |
Whether this view is visible. Defaults to true if not specified.
TYPE:
|
defaults |
List of default components or component batches to add to the space view. When an archetype in the view is missing a component included in this set, the value of default will be used instead of the normal fallback for the visualizer.
TYPE:
|
overrides |
Dictionary of overrides to apply to the space view. The key is the path to the entity where the override should be applied. The value is a list of component or component batches to apply to the entity. Important note: the path must be a fully qualified entity path starting at the root. The override paths
do not yet support
TYPE:
|
background |
Configuration for the background of the view.
TYPE:
|
visual_bounds |
The visible parts of the scene, in the coordinate space of the scene. Everything within these bounds are guaranteed to be visible. Somethings outside of these bounds may also be visible due to letterboxing.
TYPE:
|
time_ranges |
Configures which range on each timeline is shown by this view (unless specified differently per entity). If not specified, the default is to show the latest state of each component. If a timeline is specified more than once, the first entry will be used.
TYPE:
|
def blueprint_path()
The blueprint path where this space view will be logged.
Note that although this is an EntityPath
, is scoped to the blueprint tree and
not a part of the regular data hierarchy.
def to_blueprint()
Convert this space view to a full blueprint.
def to_container()
Convert this space view to a container.
class Spatial3DView
Bases: SpaceView
View: For viewing spatial 3D data.
Example
Use a blueprint to customize a Spatial3DView.:
import rerun as rr
import rerun.blueprint as rrb
from numpy.random import default_rng
rr.init("rerun_example_spatial_3d", spawn=True)
# Create some random points.
rng = default_rng(12345)
positions = rng.uniform(-5, 5, size=[50, 3])
colors = rng.uniform(0, 255, size=[50, 3])
radii = rng.uniform(0.1, 0.5, size=[50])
rr.log("points", rr.Points3D(positions, colors=colors, radii=radii))
rr.log("box", rr.Boxes3D(half_sizes=[5, 5, 5], colors=0))
# Create a Spatial3D view to display the points.
blueprint = rrb.Blueprint(
rrb.Spatial3DView(
origin="/",
name="3D Scene",
# Set the background color to light blue.
background=[100, 149, 237],
),
collapse_panels=True,
)
rr.send_blueprint(blueprint)
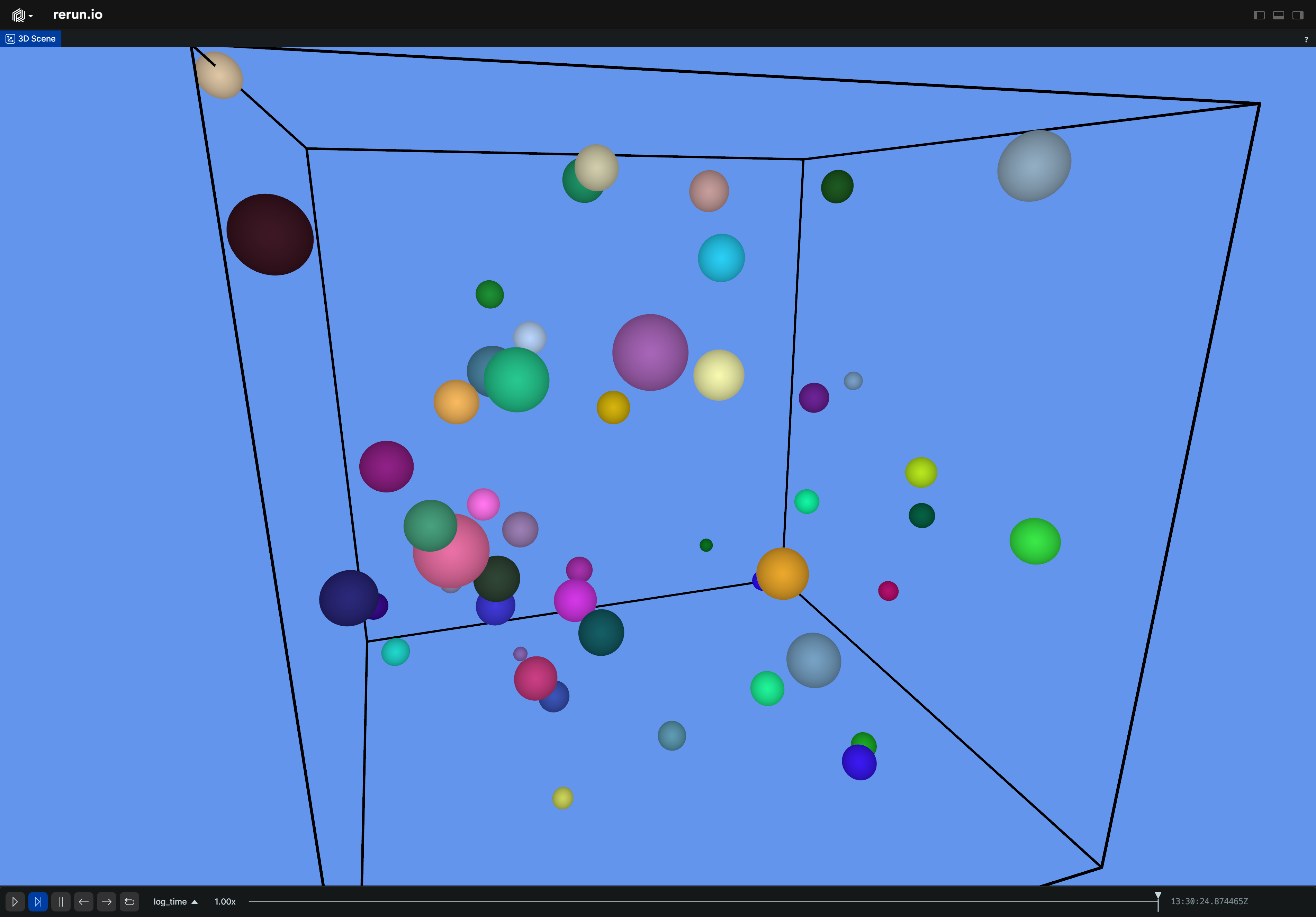
def __init__(*, origin='/', contents='$origin/**', name=None, visible=None, defaults=[], overrides={}, background=None, time_ranges=None)
Construct a blueprint for a new Spatial3DView view.
PARAMETER | DESCRIPTION |
---|---|
origin |
The
TYPE:
|
contents |
The contents of the view specified as a query expression. This is either a single expression, or a list of multiple expressions. See rerun.blueprint.archetypes.SpaceViewContents.
TYPE:
|
name |
The display name of the view.
TYPE:
|
visible |
Whether this view is visible. Defaults to true if not specified.
TYPE:
|
defaults |
List of default components or component batches to add to the space view. When an archetype in the view is missing a component included in this set, the value of default will be used instead of the normal fallback for the visualizer.
TYPE:
|
overrides |
Dictionary of overrides to apply to the space view. The key is the path to the entity where the override should be applied. The value is a list of component or component batches to apply to the entity. Important note: the path must be a fully qualified entity path starting at the root. The override paths
do not yet support
TYPE:
|
background |
Configuration for the background of the view.
TYPE:
|
time_ranges |
Configures which range on each timeline is shown by this view (unless specified differently per entity). If not specified, the default is to show the latest state of each component. If a timeline is specified more than once, the first entry will be used.
TYPE:
|
def blueprint_path()
The blueprint path where this space view will be logged.
Note that although this is an EntityPath
, is scoped to the blueprint tree and
not a part of the regular data hierarchy.
def to_blueprint()
Convert this space view to a full blueprint.
def to_container()
Convert this space view to a container.
class TensorView
Bases: SpaceView
View: A view on a tensor of any dimensionality.
Example
Use a blueprint to create a TensorView.:
import numpy as np
import rerun as rr
import rerun.blueprint as rrb
rr.init("rerun_example_tensor", spawn=True)
tensor = np.random.randint(0, 256, (32, 240, 320, 3), dtype=np.uint8)
rr.log("tensor", rr.Tensor(tensor, dim_names=("batch", "x", "y", "channel")))
blueprint = rrb.Blueprint(
rrb.TensorView(
origin="tensor",
name="Tensor",
# Explicitly pick which dimensions to show.
slice_selection=rrb.TensorSliceSelection(
# Use the first dimension as width.
width=1,
# Use the second dimension as height and invert it.
height=rr.TensorDimensionSelection(dimension=2, invert=True),
# Set which indices to show for the other dimensions.
indices=[
rr.TensorDimensionIndexSelection(dimension=2, index=4),
rr.TensorDimensionIndexSelection(dimension=3, index=5),
],
# Show a slider for dimension 2 only. If not specified, all dimensions in `indices` will have sliders.
slider=[2],
),
# Set a scalar mapping with a custom colormap, gamma and magnification filter.
scalar_mapping=rrb.TensorScalarMapping(colormap="turbo", gamma=1.5, mag_filter="linear"),
# Fill the view, ignoring aspect ratio.
view_fit="fill",
),
collapse_panels=True,
)
rr.send_blueprint(blueprint)
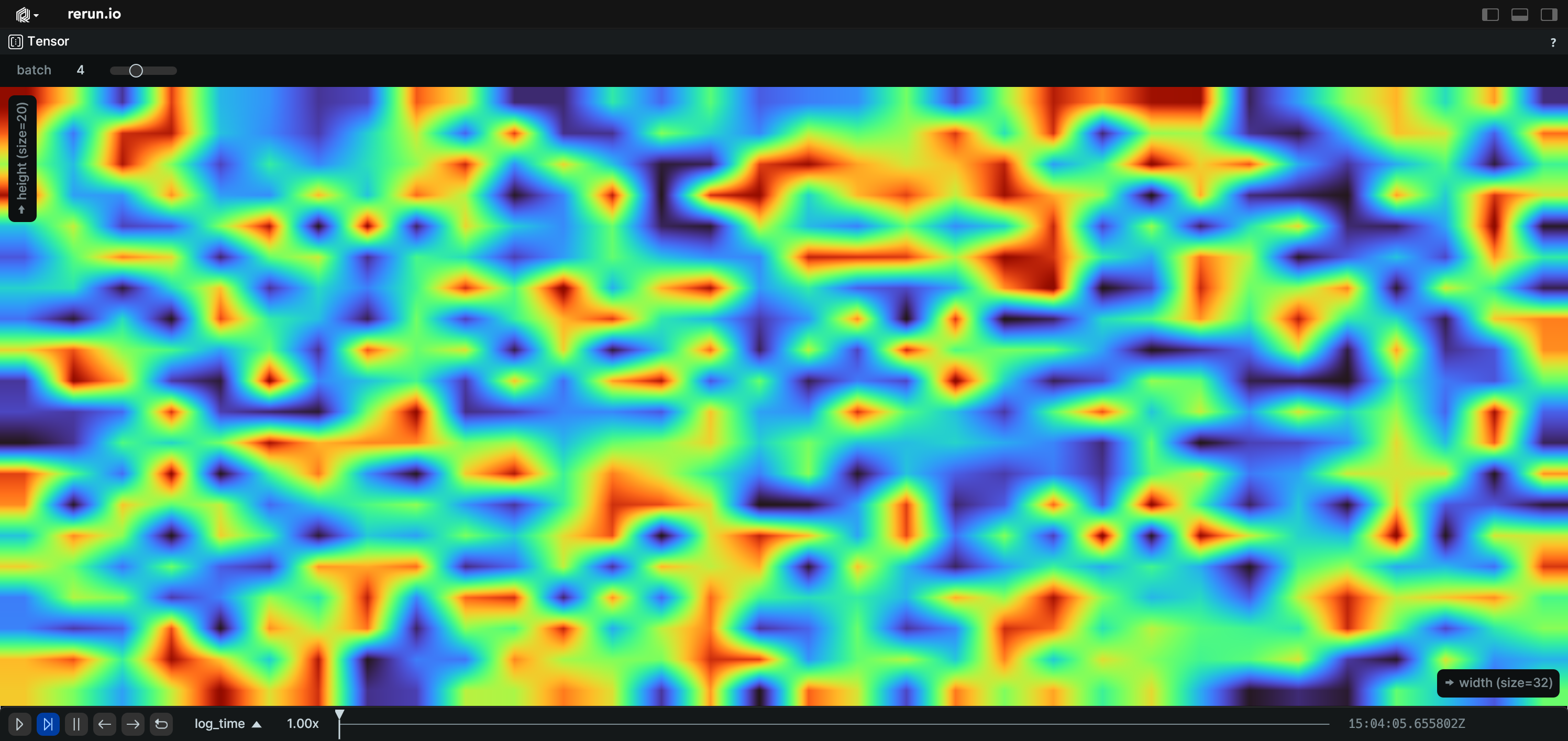
def __init__(*, origin='/', contents='$origin/**', name=None, visible=None, defaults=[], overrides={}, slice_selection=None, scalar_mapping=None, view_fit=None)
Construct a blueprint for a new TensorView view.
PARAMETER | DESCRIPTION |
---|---|
origin |
The
TYPE:
|
contents |
The contents of the view specified as a query expression. This is either a single expression, or a list of multiple expressions. See rerun.blueprint.archetypes.SpaceViewContents.
TYPE:
|
name |
The display name of the view.
TYPE:
|
visible |
Whether this view is visible. Defaults to true if not specified.
TYPE:
|
defaults |
List of default components or component batches to add to the space view. When an archetype in the view is missing a component included in this set, the value of default will be used instead of the normal fallback for the visualizer.
TYPE:
|
overrides |
Dictionary of overrides to apply to the space view. The key is the path to the entity where the override should be applied. The value is a list of component or component batches to apply to the entity. Important note: the path must be a fully qualified entity path starting at the root. The override paths
do not yet support
TYPE:
|
slice_selection |
How to select the slice of the tensor to show.
TYPE:
|
scalar_mapping |
Configures how scalars are mapped to color.
TYPE:
|
view_fit |
Configures how the selected slice should fit into the view.
TYPE:
|
def blueprint_path()
The blueprint path where this space view will be logged.
Note that although this is an EntityPath
, is scoped to the blueprint tree and
not a part of the regular data hierarchy.
def to_blueprint()
Convert this space view to a full blueprint.
def to_container()
Convert this space view to a container.
class TextDocumentView
Bases: SpaceView
View: A view of a single text document, for use with the TextDocument
archetype.
Example
Use a blueprint to show a text document.:
import rerun as rr
import rerun.blueprint as rrb
rr.init("rerun_example_text_document", spawn=True)
rr.log(
"markdown",
rr.TextDocument(
'''
# Hello Markdown!
[Click here to see the raw text](recording://markdown:Text).
Basic formatting:
| **Feature** | **Alternative** |
| ----------------- | --------------- |
| Plain | |
| *italics* | _italics_ |
| **bold** | __bold__ |
| ~~strikethrough~~ | |
| `inline code` | |
----------------------------------
## Support
- [x] [Commonmark](https://commonmark.org/help/) support
- [x] GitHub-style strikethrough, tables, and checkboxes
- Basic syntax highlighting for:
- [x] C and C++
- [x] Python
- [x] Rust
- [ ] Other languages
## Links
You can link to [an entity](recording://markdown),
a [specific instance of an entity](recording://markdown[#0]),
or a [specific component](recording://markdown:Text).
Of course you can also have [normal https links](https://github.com/rerun-io/rerun), e.g. <https://rerun.io>.
## Image

'''.strip(),
media_type=rr.MediaType.MARKDOWN,
),
)
# Create a text view that displays the markdown.
blueprint = rrb.Blueprint(rrb.TextDocumentView(origin="markdown", name="Markdown example"), collapse_panels=True)
rr.send_blueprint(blueprint)
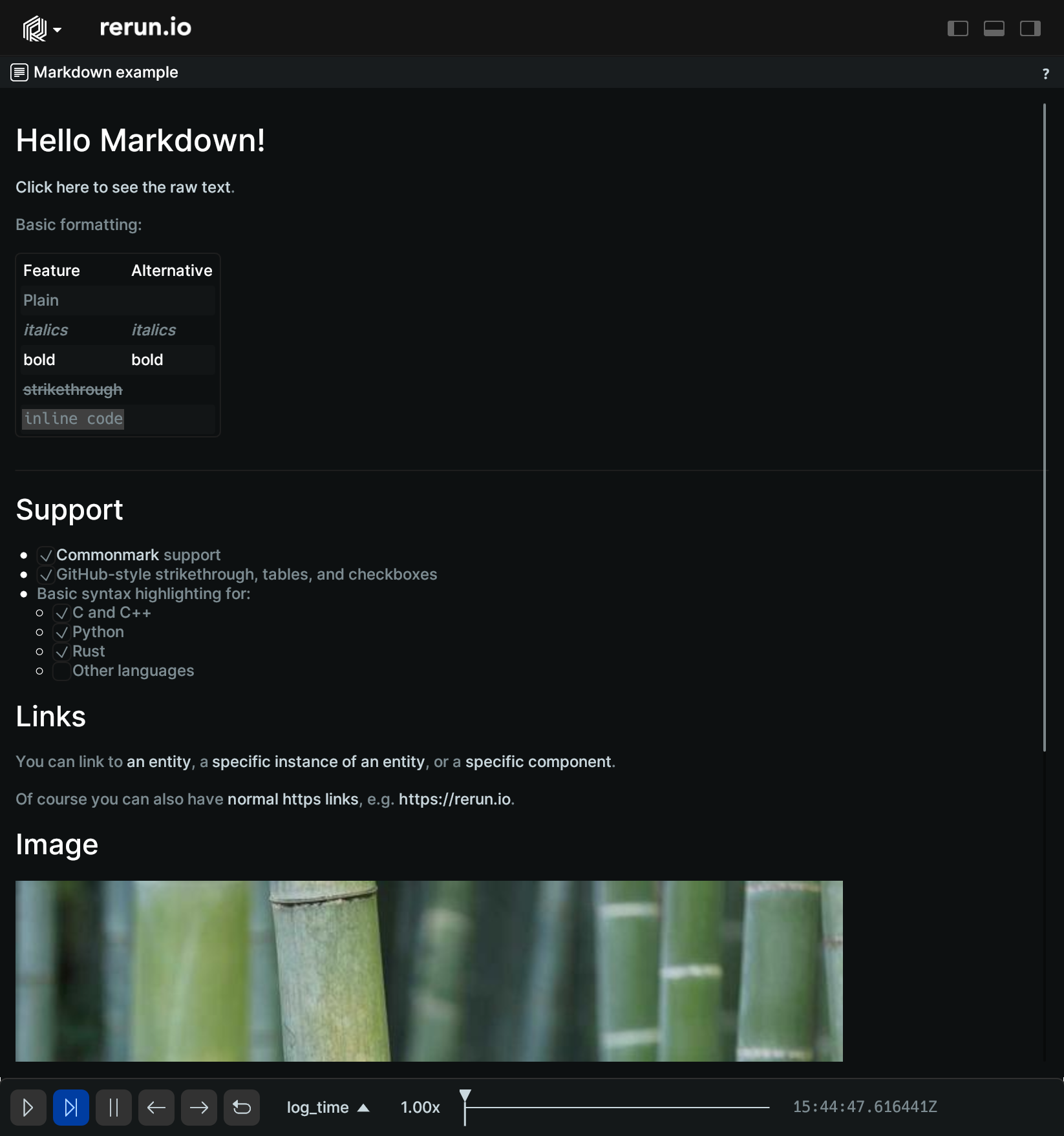
def __init__(*, origin='/', contents='$origin/**', name=None, visible=None, defaults=[], overrides={})
Construct a blueprint for a new TextDocumentView view.
PARAMETER | DESCRIPTION |
---|---|
origin |
The
TYPE:
|
contents |
The contents of the view specified as a query expression. This is either a single expression, or a list of multiple expressions. See rerun.blueprint.archetypes.SpaceViewContents.
TYPE:
|
name |
The display name of the view.
TYPE:
|
visible |
Whether this view is visible. Defaults to true if not specified.
TYPE:
|
defaults |
List of default components or component batches to add to the space view. When an archetype in the view is missing a component included in this set, the value of default will be used instead of the normal fallback for the visualizer.
TYPE:
|
overrides |
Dictionary of overrides to apply to the space view. The key is the path to the entity where the override should be applied. The value is a list of component or component batches to apply to the entity. Important note: the path must be a fully qualified entity path starting at the root. The override paths
do not yet support
TYPE:
|
def blueprint_path()
The blueprint path where this space view will be logged.
Note that although this is an EntityPath
, is scoped to the blueprint tree and
not a part of the regular data hierarchy.
def to_blueprint()
Convert this space view to a full blueprint.
def to_container()
Convert this space view to a container.
class TextLogView
Bases: SpaceView
View: A view of a text log, for use with the TextLog
archetype.
Example
Use a blueprint to show a TextLogView.:
import rerun as rr
import rerun.blueprint as rrb
rr.init("rerun_example_text_log", spawn=True)
rr.set_time_sequence("time", 0)
rr.log("log/status", rr.TextLog("Application started.", level=rr.TextLogLevel.INFO))
rr.set_time_sequence("time", 5)
rr.log("log/other", rr.TextLog("A warning.", level=rr.TextLogLevel.WARN))
for i in range(10):
rr.set_time_sequence("time", i)
rr.log("log/status", rr.TextLog(f"Processing item {i}.", level=rr.TextLogLevel.INFO))
# Create a text view that displays all logs.
blueprint = rrb.Blueprint(rrb.TextLogView(origin="/log", name="Text Logs"), collapse_panels=True)
rr.send_blueprint(blueprint)
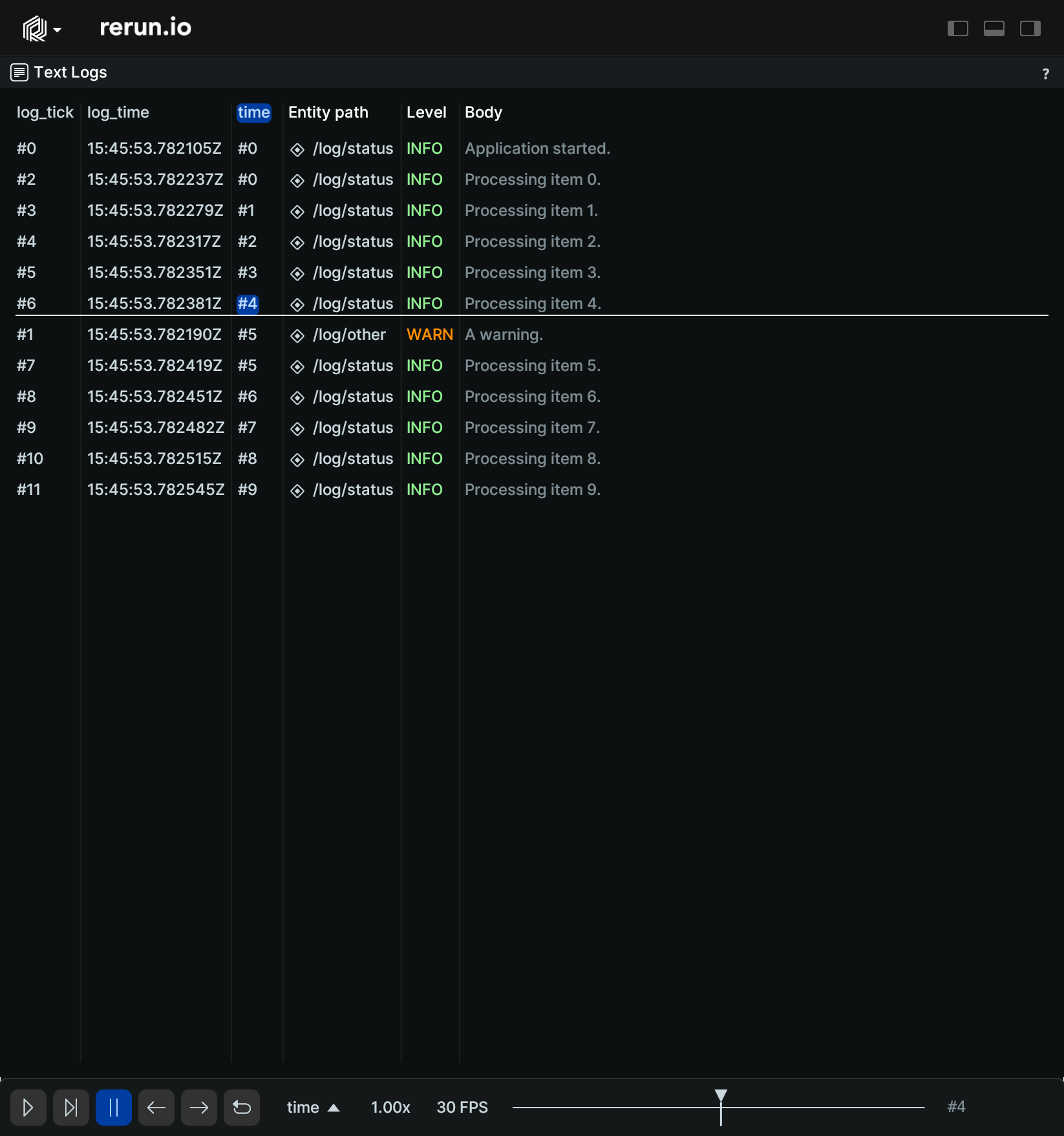
def __init__(*, origin='/', contents='$origin/**', name=None, visible=None, defaults=[], overrides={})
Construct a blueprint for a new TextLogView view.
PARAMETER | DESCRIPTION |
---|---|
origin |
The
TYPE:
|
contents |
The contents of the view specified as a query expression. This is either a single expression, or a list of multiple expressions. See rerun.blueprint.archetypes.SpaceViewContents.
TYPE:
|
name |
The display name of the view.
TYPE:
|
visible |
Whether this view is visible. Defaults to true if not specified.
TYPE:
|
defaults |
List of default components or component batches to add to the space view. When an archetype in the view is missing a component included in this set, the value of default will be used instead of the normal fallback for the visualizer.
TYPE:
|
overrides |
Dictionary of overrides to apply to the space view. The key is the path to the entity where the override should be applied. The value is a list of component or component batches to apply to the entity. Important note: the path must be a fully qualified entity path starting at the root. The override paths
do not yet support
TYPE:
|
def blueprint_path()
The blueprint path where this space view will be logged.
Note that although this is an EntityPath
, is scoped to the blueprint tree and
not a part of the regular data hierarchy.
def to_blueprint()
Convert this space view to a full blueprint.
def to_container()
Convert this space view to a container.
class TimeSeriesView
Bases: SpaceView
View: A time series view for scalars over time, for use with the Scalars
archetype.
Example
Use a blueprint to customize a TimeSeriesView.:
import math
import rerun as rr
import rerun.blueprint as rrb
rr.init("rerun_example_timeseries", spawn=True)
# Log some trigonometric functions
rr.log("trig/sin", rr.SeriesLine(color=[255, 0, 0], name="sin(0.01t)"), static=True)
rr.log("trig/cos", rr.SeriesLine(color=[0, 255, 0], name="cos(0.01t)"), static=True)
rr.log("trig/cos", rr.SeriesLine(color=[0, 0, 255], name="cos(0.01t) scaled"), static=True)
for t in range(0, int(math.pi * 4 * 100.0)):
rr.set_time_sequence("timeline0", t)
rr.set_time_seconds("timeline1", t)
rr.log("trig/sin", rr.Scalar(math.sin(float(t) / 100.0)))
rr.log("trig/cos", rr.Scalar(math.cos(float(t) / 100.0)))
rr.log("trig/cos_scaled", rr.Scalar(math.cos(float(t) / 100.0) * 2.0))
# Create a TimeSeries View
blueprint = rrb.Blueprint(
rrb.TimeSeriesView(
origin="/trig",
# Set a custom Y axis.
axis_y=rrb.ScalarAxis(range=(-1.0, 1.0), zoom_lock=True),
# Configure the legend.
plot_legend=rrb.PlotLegend(visible=False),
# Set time different time ranges for different timelines.
time_ranges=[
# Sliding window depending on the time cursor for the first timeline.
rrb.VisibleTimeRange(
"timeline0",
start=rrb.TimeRangeBoundary.cursor_relative(seq=-100),
end=rrb.TimeRangeBoundary.cursor_relative(),
),
# Time range from some point to the end of the timeline for the second timeline.
rrb.VisibleTimeRange(
"timeline1",
start=rrb.TimeRangeBoundary.absolute(seconds=300.0),
end=rrb.TimeRangeBoundary.infinite(),
),
],
),
collapse_panels=True,
)
rr.send_blueprint(blueprint)
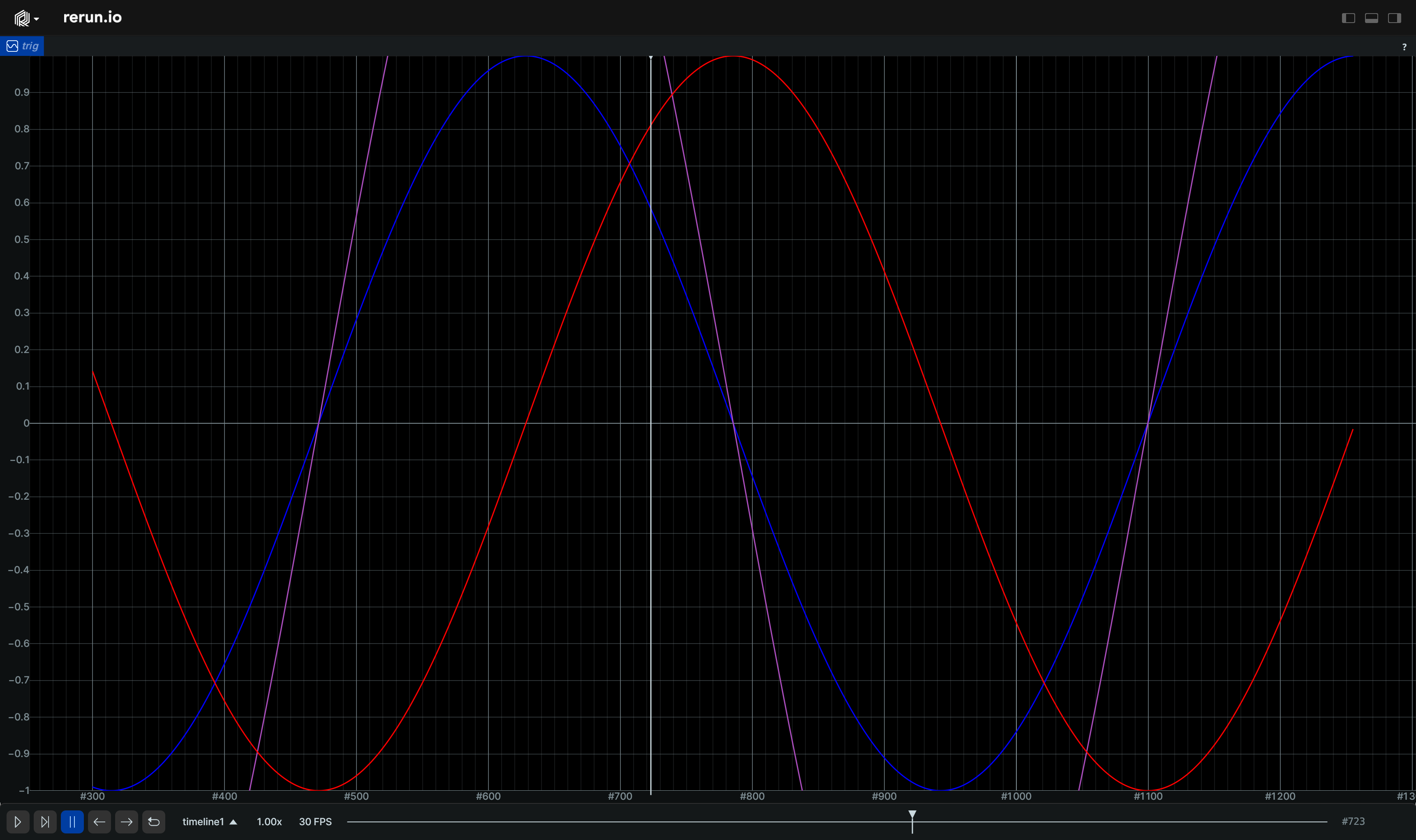
def __init__(*, origin='/', contents='$origin/**', name=None, visible=None, defaults=[], overrides={}, axis_y=None, plot_legend=None, time_ranges=None)
Construct a blueprint for a new TimeSeriesView view.
PARAMETER | DESCRIPTION |
---|---|
origin |
The
TYPE:
|
contents |
The contents of the view specified as a query expression. This is either a single expression, or a list of multiple expressions. See rerun.blueprint.archetypes.SpaceViewContents.
TYPE:
|
name |
The display name of the view.
TYPE:
|
visible |
Whether this view is visible. Defaults to true if not specified.
TYPE:
|
defaults |
List of default components or component batches to add to the space view. When an archetype in the view is missing a component included in this set, the value of default will be used instead of the normal fallback for the visualizer.
TYPE:
|
overrides |
Dictionary of overrides to apply to the space view. The key is the path to the entity where the override should be applied. The value is a list of component or component batches to apply to the entity. Important note: the path must be a fully qualified entity path starting at the root. The override paths
do not yet support
TYPE:
|
axis_y |
Configures the vertical axis of the plot.
TYPE:
|
plot_legend |
Configures the legend of the plot.
TYPE:
|
time_ranges |
Configures which range on each timeline is shown by this view (unless specified differently per entity). If not specified, the default is to show the entire timeline. If a timeline is specified more than once, the first entry will be used.
TYPE:
|
def blueprint_path()
The blueprint path where this space view will be logged.
Note that although this is an EntityPath
, is scoped to the blueprint tree and
not a part of the regular data hierarchy.
def to_blueprint()
Convert this space view to a full blueprint.
def to_container()
Convert this space view to a container.
class BlueprintPanel
Bases: Panel
The state of the blueprint panel.
def __init__(*, expanded=None, state=None)
Construct a new blueprint panel.
PARAMETER | DESCRIPTION |
---|---|
expanded |
Deprecated. Use
TYPE:
|
state |
Whether the panel is expanded, collapsed, or hidden. Collapsed and hidden both fully hide the blueprint panel.
TYPE:
|
def blueprint_path()
The blueprint path where this space view will be logged.
Note that although this is an EntityPath
, is scoped to the blueprint tree and
not a part of the regular data hierarchy.
class SelectionPanel
Bases: Panel
The state of the selection panel.
def __init__(*, expanded=None, state=None)
Construct a new selection panel.
PARAMETER | DESCRIPTION |
---|---|
expanded |
Deprecated. Use
TYPE:
|
state |
Whether the panel is expanded, collapsed, or hidden. Collapsed and hidden both fully hide the selection panel.
TYPE:
|
def blueprint_path()
The blueprint path where this space view will be logged.
Note that although this is an EntityPath
, is scoped to the blueprint tree and
not a part of the regular data hierarchy.
class TimePanel
Bases: Panel
The state of the time panel.
def __init__(*, expanded=None, state=None)
Construct a new time panel.
PARAMETER | DESCRIPTION |
---|---|
expanded |
Deprecated. Use
TYPE:
|
state |
Whether the panel is expanded, collapsed, or hidden. Expanded fully shows the panel, collapsed shows a simplified panel, hidden fully hides the panel.
TYPE:
|
def blueprint_path()
The blueprint path where this space view will be logged.
Note that although this is an EntityPath
, is scoped to the blueprint tree and
not a part of the regular data hierarchy.