Archetypes
rerun.archetypes
class AnnotationContext
Bases: Archetype
Archetype: The AnnotationContext
provides additional information on how to display entities.
Entities can use ClassId
s and KeypointId
s to provide annotations, and
the labels and colors will be looked up in the appropriate
AnnotationContext
. We use the first annotation context we find in the
path-hierarchy when searching up through the ancestors of a given entity
path.
See also ClassDescription
.
Example
Segmentation:
import numpy as np
import rerun as rr
rr.init("rerun_example_annotation_context_segmentation", spawn=True)
# Create a simple segmentation image
image = np.zeros((200, 300), dtype=np.uint8)
image[50:100, 50:120] = 1
image[100:180, 130:280] = 2
# Log an annotation context to assign a label and color to each class
rr.log("segmentation", rr.AnnotationContext([(1, "red", (255, 0, 0)), (2, "green", (0, 255, 0))]), timeless=True)
rr.log("segmentation/image", rr.SegmentationImage(image))
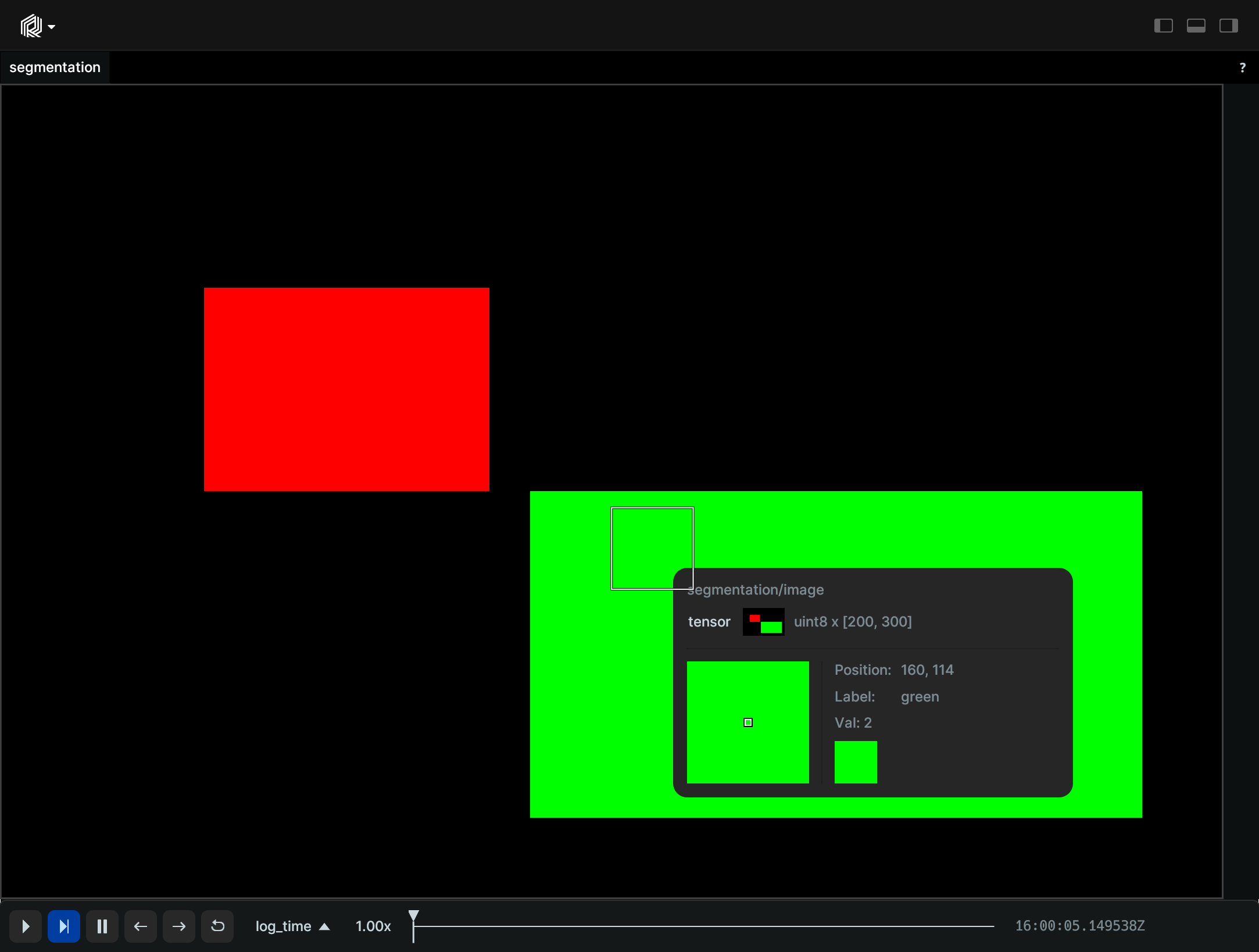
def __init__ (context)
Create a new instance of the AnnotationContext archetype.
PARAMETER | DESCRIPTION |
---|---|
context |
List of class descriptions, mapping class indices to class names, colors etc.
TYPE:
|
class Arrows3D
Bases: Arrows3DExt
, Archetype
Archetype: 3D arrows with optional colors, radii, labels, etc.
Example
Simple batch of 3D Arrows:
from math import tau
import numpy as np
import rerun as rr
rr.init("rerun_example_arrow3d", spawn=True)
lengths = np.log2(np.arange(0, 100) + 1)
angles = np.arange(start=0, stop=tau, step=tau * 0.01)
origins = np.zeros((100, 3))
vectors = np.column_stack([np.sin(angles) * lengths, np.zeros(100), np.cos(angles) * lengths])
colors = [[1.0 - c, c, 0.5, 0.5] for c in angles / tau]
rr.log("arrows", rr.Arrows3D(origins=origins, vectors=vectors, colors=colors))
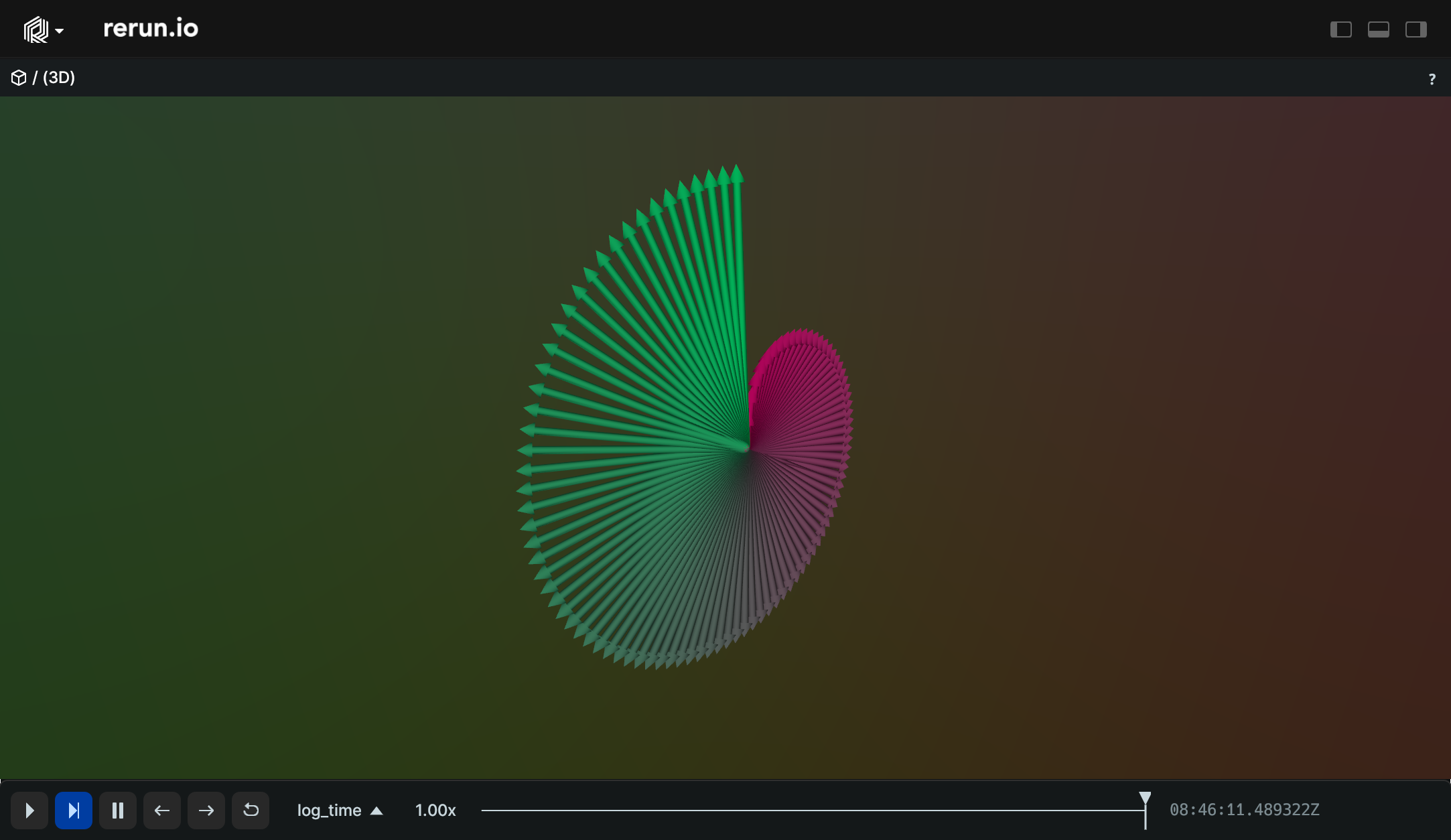
def __init__ (*, vectors, origins=None, radii=None, colors=None, labels=None, class_ids=None, instance_keys=None)
Create a new instance of the Arrows3D archetype.
PARAMETER | DESCRIPTION |
---|---|
vectors |
All the vectors for each arrow in the batch.
TYPE:
|
origins |
All the origin points for each arrow in the batch. If no origins are set, (0, 0, 0) is used as the origin for each arrow.
TYPE:
|
radii |
Optional radii for the arrows. The shaft is rendered as a line with
TYPE:
|
colors |
Optional colors for the points.
TYPE:
|
labels |
Optional text labels for the arrows.
TYPE:
|
class_ids |
Optional class Ids for the points. The class ID provides colors and labels if not specified explicitly.
TYPE:
|
instance_keys |
Unique identifiers for each individual point in the batch.
TYPE:
|