Archetypes
rerun.archetypes
class AnnotationContext
Bases: Archetype
Archetype: The AnnotationContext
provides additional information on how to display entities.
Entities can use ClassId
s and KeypointId
s to provide annotations, and
the labels and colors will be looked up in the appropriate
AnnotationContext
. We use the first annotation context we find in the
path-hierarchy when searching up through the ancestors of a given entity
path.
See also ClassDescription
.
Example
Segmentation:
import numpy as np
import rerun as rr
rr.init("rerun_example_annotation_context_segmentation", spawn=True)
# Create a simple segmentation image
image = np.zeros((200, 300), dtype=np.uint8)
image[50:100, 50:120] = 1
image[100:180, 130:280] = 2
# Log an annotation context to assign a label and color to each class
rr.log("segmentation", rr.AnnotationContext([(1, "red", (255, 0, 0)), (2, "green", (0, 255, 0))]), timeless=True)
rr.log("segmentation/image", rr.SegmentationImage(image))
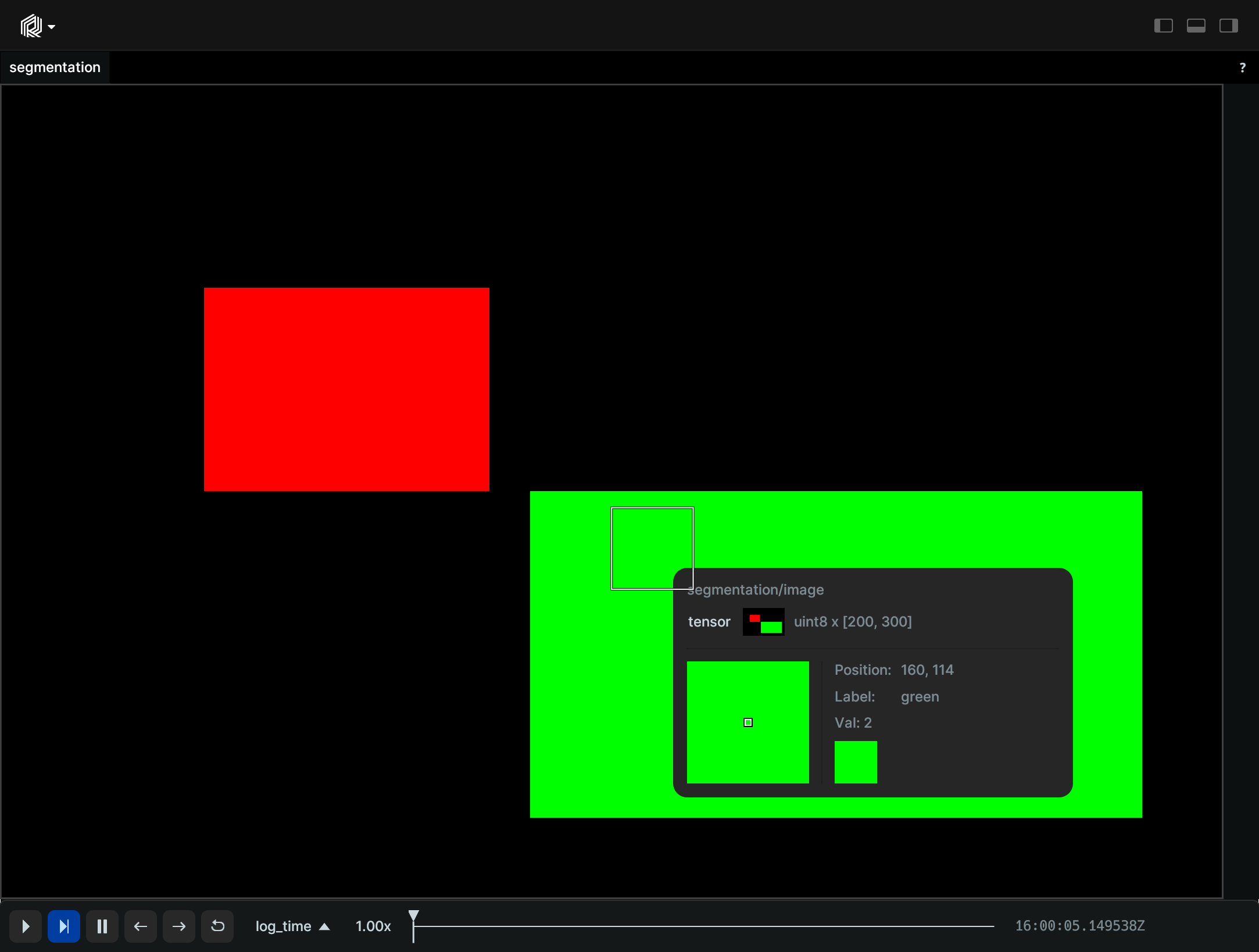
def __init__(context)
Create a new instance of the AnnotationContext archetype.
PARAMETER | DESCRIPTION |
---|---|
context |
List of class descriptions, mapping class indices to class names, colors etc.
TYPE:
|
class Arrows2D
Bases: Arrows2DExt
, Archetype
Archetype: 2D arrows with optional colors, radii, labels, etc.
Example
Simple batch of 2D arrows:
import rerun as rr
rr.init("rerun_example_arrow2d", spawn=True)
rr.log(
"arrows",
rr.Arrows2D(
origins=[[0.25, 0.0], [0.25, 0.0], [-0.1, -0.1]],
vectors=[[1.0, 0.0], [0.0, -1.0], [-0.7, 0.7]],
colors=[[255, 0, 0], [0, 255, 0], [127, 0, 255]],
labels=["right", "up", "left-down"],
radii=0.025,
),
)
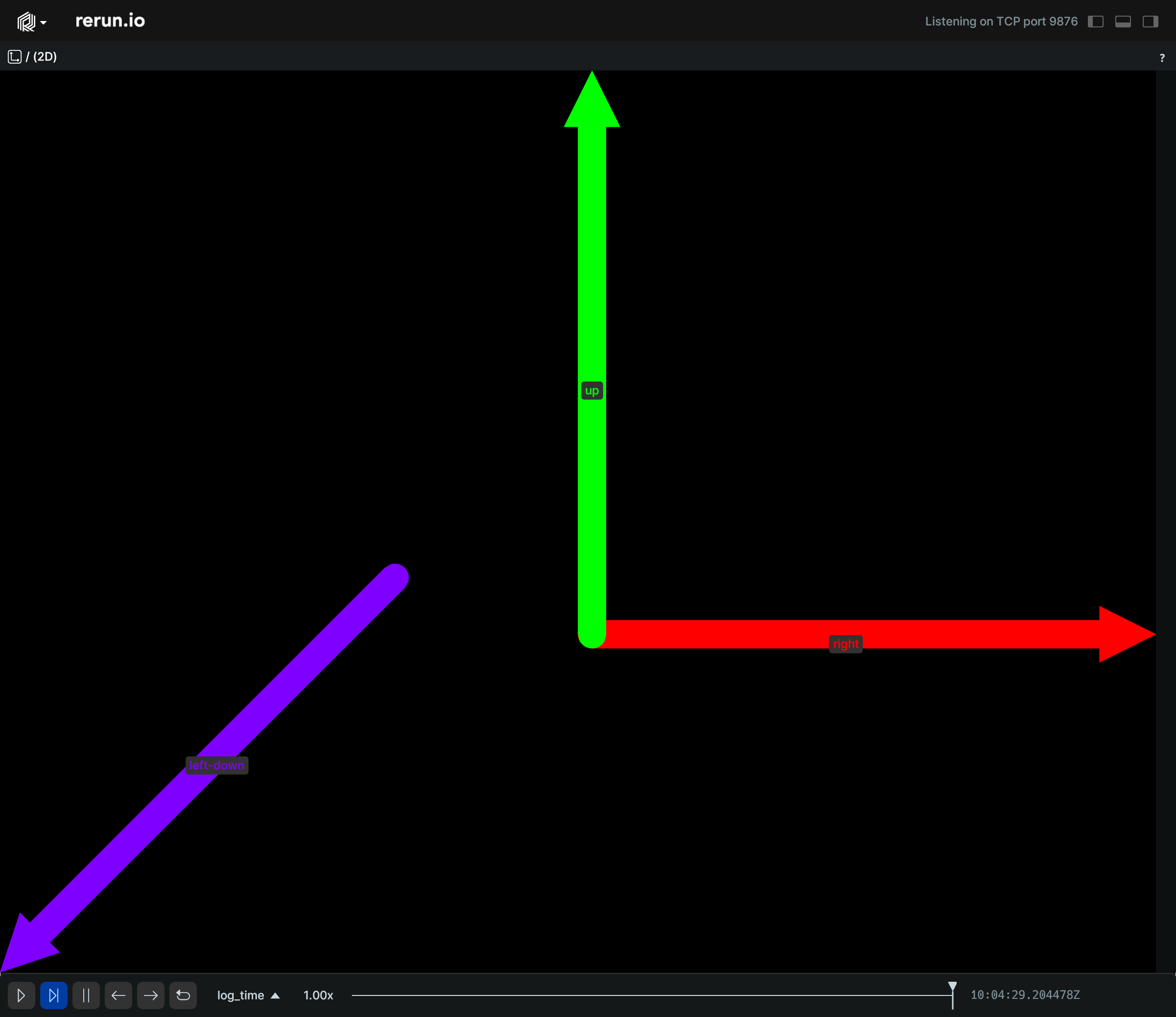
def __init__(*, vectors, origins=None, radii=None, colors=None, labels=None, class_ids=None)
Create a new instance of the Arrows2D archetype.
PARAMETER | DESCRIPTION |
---|---|
vectors |
All the vectors for each arrow in the batch.
TYPE:
|
origins |
All the origin points for each arrow in the batch. If no origins are set, (0, 0, 0) is used as the origin for each arrow.
TYPE:
|
radii |
Optional radii for the arrows. The shaft is rendered as a line with
TYPE:
|
colors |
Optional colors for the points.
TYPE:
|
labels |
Optional text labels for the arrows.
TYPE:
|
class_ids |
Optional class Ids for the points. The class ID provides colors and labels if not specified explicitly.
TYPE:
|
class Arrows3D
Bases: Arrows3DExt
, Archetype
Archetype: 3D arrows with optional colors, radii, labels, etc.
Example
Simple batch of 3D arrows:
from math import tau
import numpy as np
import rerun as rr
rr.init("rerun_example_arrow3d", spawn=True)
lengths = np.log2(np.arange(0, 100) + 1)
angles = np.arange(start=0, stop=tau, step=tau * 0.01)
origins = np.zeros((100, 3))
vectors = np.column_stack([np.sin(angles) * lengths, np.zeros(100), np.cos(angles) * lengths])
colors = [[1.0 - c, c, 0.5, 0.5] for c in angles / tau]
rr.log("arrows", rr.Arrows3D(origins=origins, vectors=vectors, colors=colors))
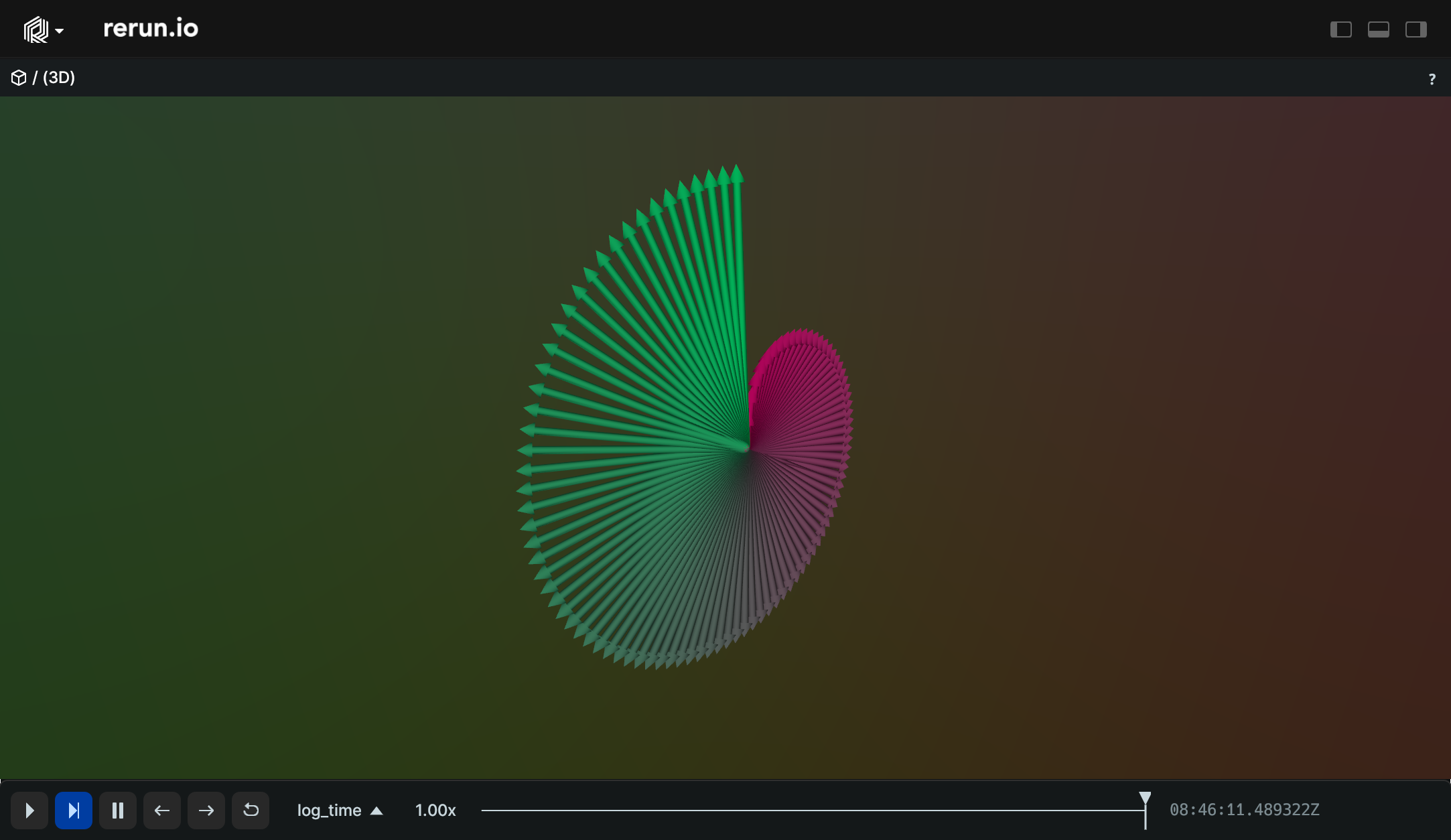
def __init__(*, vectors, origins=None, radii=None, colors=None, labels=None, class_ids=None)
Create a new instance of the Arrows3D archetype.
PARAMETER | DESCRIPTION |
---|---|
vectors |
All the vectors for each arrow in the batch.
TYPE:
|
origins |
All the origin points for each arrow in the batch. If no origins are set, (0, 0, 0) is used as the origin for each arrow.
TYPE:
|
radii |
Optional radii for the arrows. The shaft is rendered as a line with
TYPE:
|
colors |
Optional colors for the points.
TYPE:
|
labels |
Optional text labels for the arrows.
TYPE:
|
class_ids |
Optional class Ids for the points. The class ID provides colors and labels if not specified explicitly.
TYPE:
|
class Asset3D
Bases: Asset3DExt
, Archetype
Archetype: A prepacked 3D asset (.gltf
, .glb
, .obj
, .stl
, etc.).
See also Mesh3D
.
Example
Simple 3D asset:
import sys
import rerun as rr
if len(sys.argv) < 2:
print(f"Usage: {sys.argv[0]} <path_to_asset.[gltf|glb|obj|stl]>")
sys.exit(1)
rr.init("rerun_example_asset3d", spawn=True)
rr.log("world", rr.ViewCoordinates.RIGHT_HAND_Z_UP, timeless=True) # Set an up-axis
rr.log("world/asset", rr.Asset3D(path=sys.argv[1]))
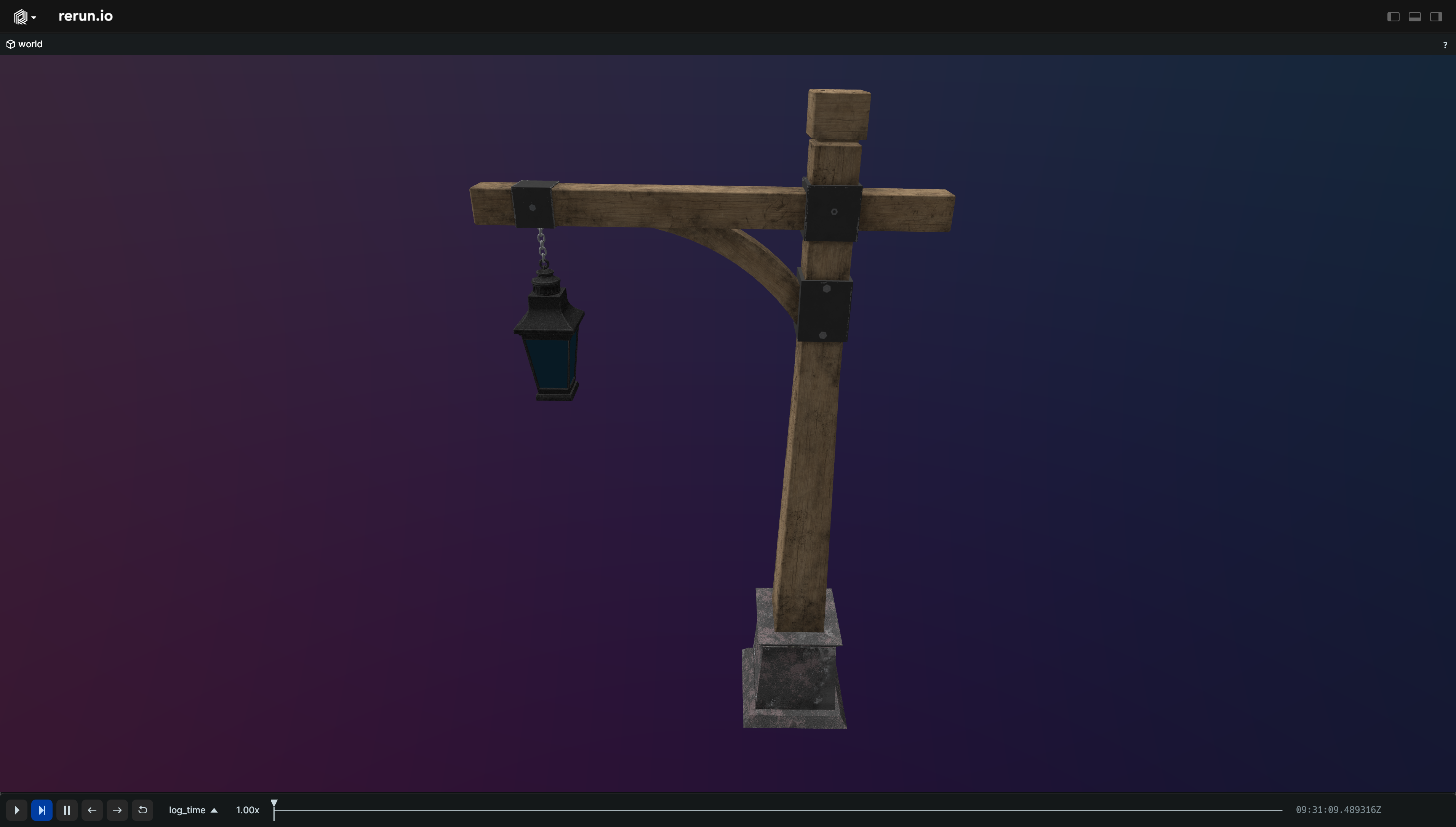
def __init__(*, path=None, contents=None, media_type=None, transform=None)
Create a new instance of the Asset3D archetype.
PARAMETER | DESCRIPTION |
---|---|
path |
A path to an file stored on the local filesystem. Mutually
exclusive with |
contents |
The contents of the file. Can be a BufferedReader, BytesIO, or
bytes. Mutually exclusive with
TYPE:
|
media_type |
The Media Type of the asset. For instance:
* If omitted, it will be guessed from the
TYPE:
|
transform |
An out-of-tree transform. Applies a transformation to the asset itself without impacting its children.
TYPE:
|
class BarChart
Bases: BarChartExt
, Archetype
Archetype: A bar chart.
The x values will be the indices of the array, and the bar heights will be the provided values.
Example
Simple bar chart:
import rerun as rr
rr.init("rerun_example_bar_chart", spawn=True)
rr.log("bar_chart", rr.BarChart([8, 4, 0, 9, 1, 4, 1, 6, 9, 0]))
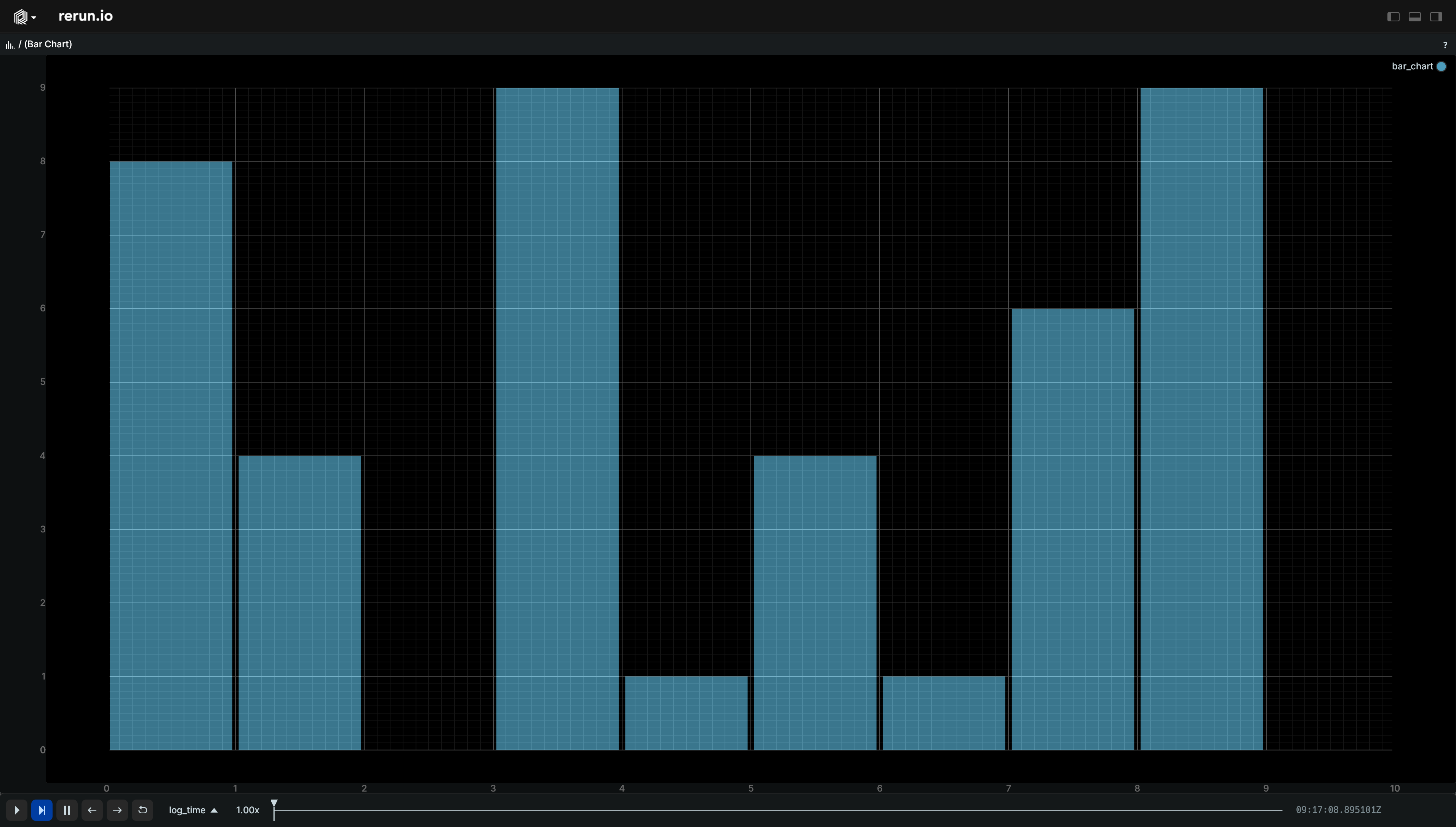
def __init__(values, *, color=None)
Create a new instance of the BarChart archetype.
PARAMETER | DESCRIPTION |
---|---|
values |
The values. Should always be a rank-1 tensor.
TYPE:
|
color |
The color of the bar chart
TYPE:
|
class Boxes2D
Bases: Boxes2DExt
, Archetype
Archetype: 2D boxes with half-extents and optional center, rotations, rotations, colors etc.
Example
Simple 2D boxes:
import rerun as rr
rr.init("rerun_example_box2d", spawn=True)
rr.log("simple", rr.Boxes2D(mins=[-1, -1], sizes=[2, 2]))
# Log an extra rect to set the view bounds
rr.log("bounds", rr.Boxes2D(sizes=[4.0, 3.0]))
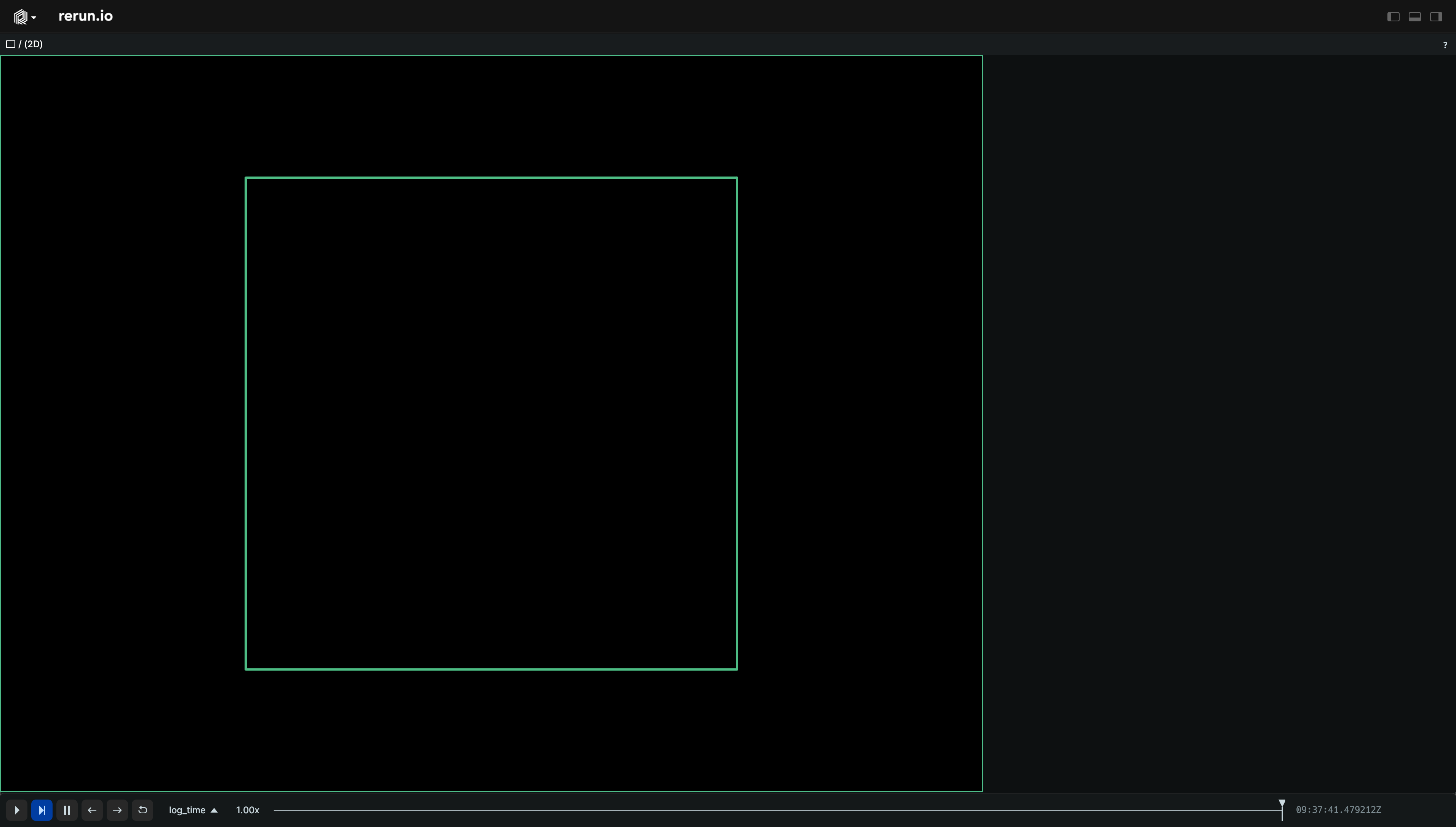
def __init__(*, sizes=None, mins=None, half_sizes=None, centers=None, array=None, array_format=None, radii=None, colors=None, labels=None, draw_order=None, class_ids=None)
Create a new instance of the Boxes2D archetype.
PARAMETER | DESCRIPTION |
---|---|
sizes |
Full extents in x/y.
Incompatible with
TYPE:
|
half_sizes |
All half-extents that make up the batch of boxes. Specify this instead of
TYPE:
|
mins |
Minimum coordinates of the boxes. Specify this instead of
TYPE:
|
array |
An array of boxes in the format specified by
TYPE:
|
array_format |
How to interpret the data in
TYPE:
|
centers |
Optional center positions of the boxes.
TYPE:
|
colors |
Optional colors for the boxes.
TYPE:
|
radii |
Optional radii for the lines that make up the boxes.
TYPE:
|
labels |
Optional text labels for the boxes.
TYPE:
|
draw_order |
An optional floating point value that specifies the 2D drawing order. Objects with higher values are drawn on top of those with lower values. The default for 2D boxes is 10.0.
TYPE:
|
class_ids |
Optional The class ID provides colors and labels if not specified explicitly.
TYPE:
|
class Boxes3D
Bases: Boxes3DExt
, Archetype
Archetype: 3D boxes with half-extents and optional center, rotations, rotations, colors etc.
Example
Batch of 3D boxes:
import rerun as rr
from rerun.datatypes import Angle, Quaternion, Rotation3D, RotationAxisAngle
rr.init("rerun_example_box3d_batch", spawn=True)
rr.log(
"batch",
rr.Boxes3D(
centers=[[2, 0, 0], [-2, 0, 0], [0, 0, 2]],
half_sizes=[[2.0, 2.0, 1.0], [1.0, 1.0, 0.5], [2.0, 0.5, 1.0]],
rotations=[
Rotation3D.identity(),
Quaternion(xyzw=[0.0, 0.0, 0.382683, 0.923880]), # 45 degrees around Z
RotationAxisAngle(axis=[0, 1, 0], angle=Angle(deg=30)),
],
radii=0.025,
colors=[(255, 0, 0), (0, 255, 0), (0, 0, 255)],
labels=["red", "green", "blue"],
),
)
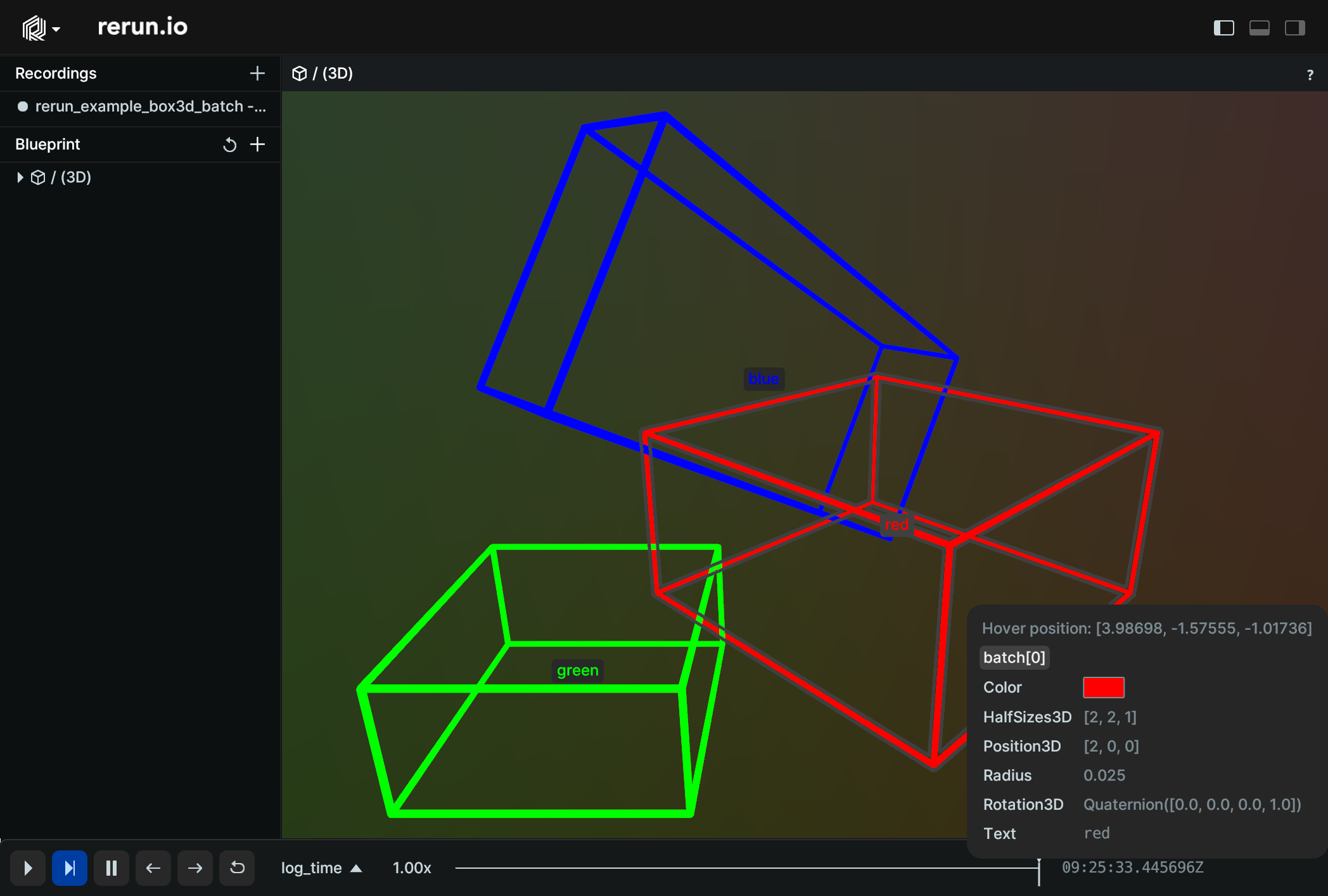
def __init__(*, sizes=None, mins=None, half_sizes=None, centers=None, rotations=None, colors=None, radii=None, labels=None, class_ids=None)
Create a new instance of the Boxes3D archetype.
PARAMETER | DESCRIPTION |
---|---|
sizes |
Full extents in x/y/z. Specify this instead of
TYPE:
|
half_sizes |
All half-extents that make up the batch of boxes. Specify this instead of
TYPE:
|
mins |
Minimum coordinates of the boxes. Specify this instead of Only valid when used together with either
TYPE:
|
centers |
Optional center positions of the boxes.
TYPE:
|
rotations |
Optional rotations of the boxes.
TYPE:
|
colors |
Optional colors for the boxes.
TYPE:
|
radii |
Optional radii for the lines that make up the boxes.
TYPE:
|
labels |
Optional text labels for the boxes.
TYPE:
|
class_ids |
Optional The class ID provides colors and labels if not specified explicitly.
TYPE:
|
class Clear
Bases: ClearExt
, Archetype
Archetype: Empties all the components of an entity.
The presence of a clear means that a latest-at query of components at a given path(s) will not return any components that were logged at those paths before the clear. Any logged components after the clear are unaffected by the clear.
This implies that a range query that includes time points that are before the clear, still returns all components at the given path(s). Meaning that in practice clears are ineffective when making use of visible time ranges. Scalar plots are an exception: they track clears and use them to represent holes in the data (i.e. discontinuous lines).
Example
Flat:
import rerun as rr
rr.init("rerun_example_clear", spawn=True)
vectors = [(1.0, 0.0, 0.0), (0.0, -1.0, 0.0), (-1.0, 0.0, 0.0), (0.0, 1.0, 0.0)]
origins = [(-0.5, 0.5, 0.0), (0.5, 0.5, 0.0), (0.5, -0.5, 0.0), (-0.5, -0.5, 0.0)]
colors = [(200, 0, 0), (0, 200, 0), (0, 0, 200), (200, 0, 200)]
# Log a handful of arrows.
for i, (vector, origin, color) in enumerate(zip(vectors, origins, colors)):
rr.log(f"arrows/{i}", rr.Arrows3D(vectors=vector, origins=origin, colors=color))
# Now clear them, one by one on each tick.
for i in range(len(vectors)):
rr.log(f"arrows/{i}", rr.Clear(recursive=False)) # or `rr.Clear.flat()`
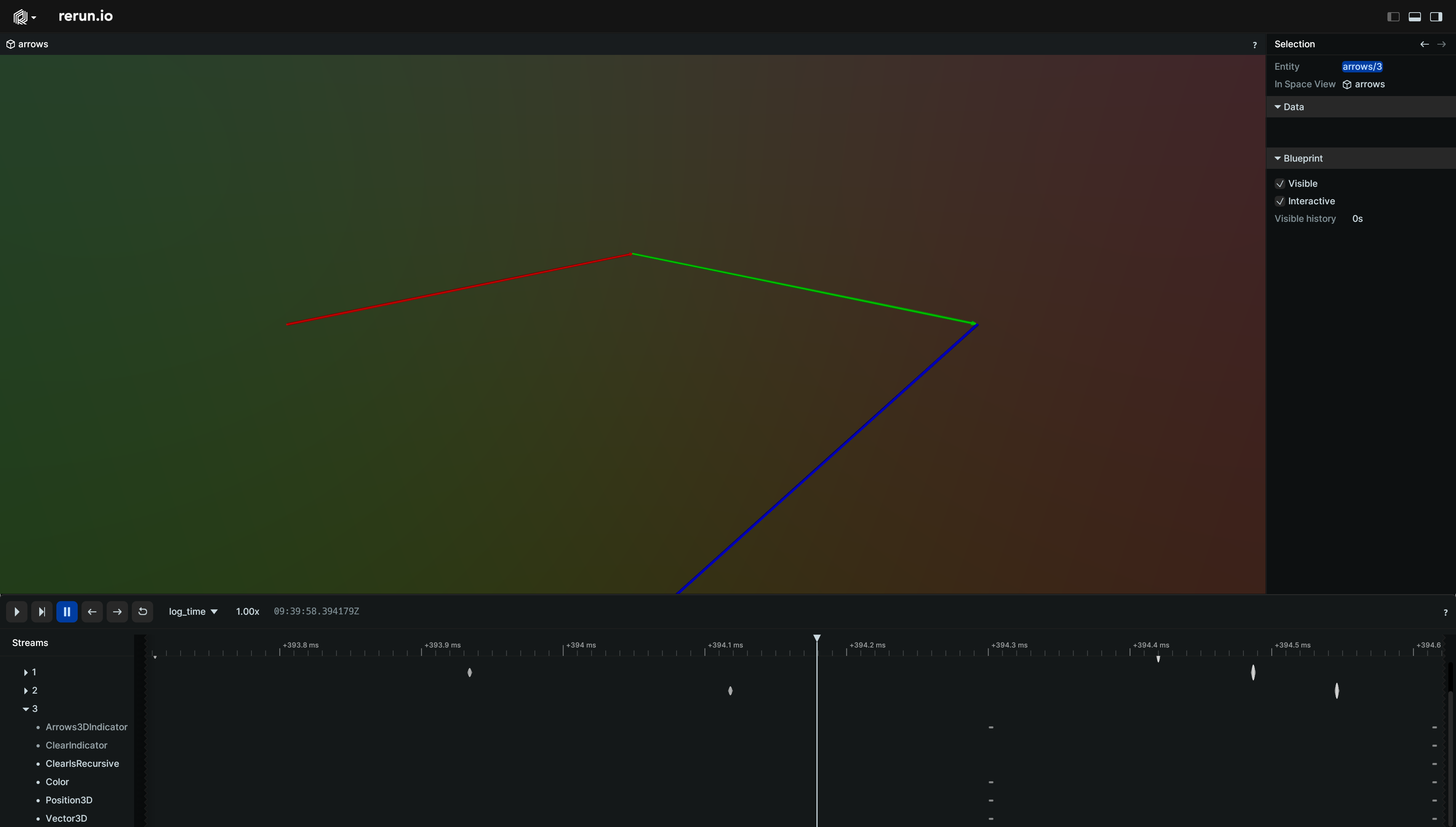
def __init__(*, recursive)
Create a new instance of the Clear archetype.
PARAMETER | DESCRIPTION |
---|---|
recursive |
Whether to recursively clear all children.
TYPE:
|
def flat()
staticmethod
Returns a non-recursive clear archetype.
This will empty all components of the associated entity at the logged timepoint. Children will be left untouched.
def recursive()
staticmethod
Returns a recursive clear archetype.
This will empty all components of the associated entity at the logged timepoint, as well as all components of all its recursive children.
class DepthImage
Bases: DepthImageExt
, Archetype
Archetype: A depth image.
The shape of the TensorData
must be mappable to an HxW
tensor.
Each pixel corresponds to a depth value in units specified by meter
.
Example
Depth to 3D example:
import numpy as np
import rerun as rr
depth_image = 65535 * np.ones((200, 300), dtype=np.uint16)
depth_image[50:150, 50:150] = 20000
depth_image[130:180, 100:280] = 45000
rr.init("rerun_example_depth_image_3d", spawn=True)
# If we log a pinhole camera model, the depth gets automatically back-projected to 3D
rr.log(
"world/camera",
rr.Pinhole(
width=depth_image.shape[1],
height=depth_image.shape[0],
focal_length=200,
),
)
# Log the tensor.
rr.log("world/camera/depth", rr.DepthImage(depth_image, meter=10_000.0))
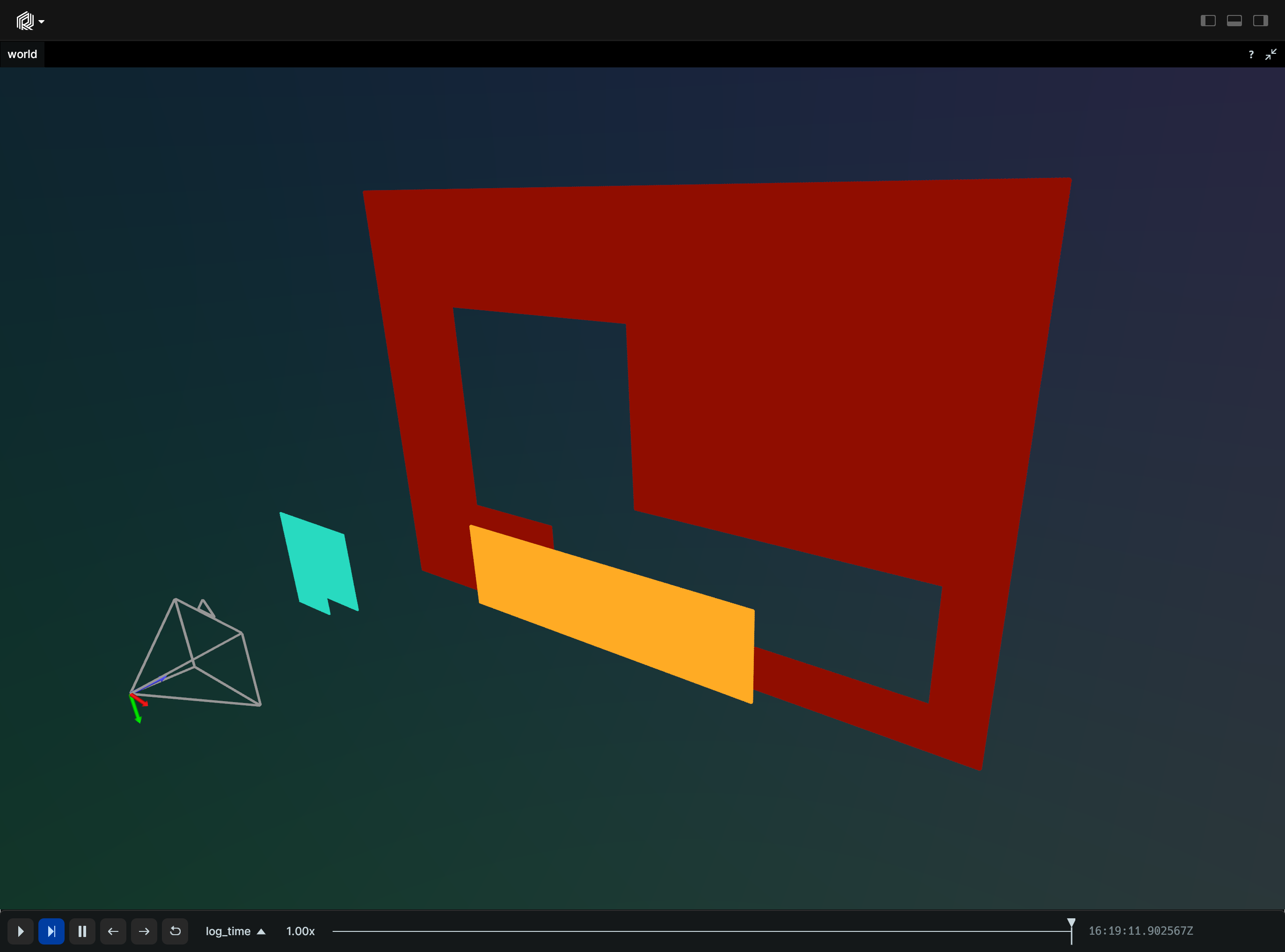
def __init__(data, *, meter=None, draw_order=None)
Create a new instance of the DepthImage archetype.
PARAMETER | DESCRIPTION |
---|---|
data |
The depth-image data. Should always be a rank-2 tensor.
TYPE:
|
meter |
An optional floating point value that specifies how long a meter is in the native depth units. For instance: with uint16, perhaps meter=1000 which would mean you have millimeter precision and a range of up to ~65 meters (2^16 / 1000).
TYPE:
|
draw_order |
An optional floating point value that specifies the 2D drawing order. Objects with higher values are drawn on top of those with lower values.
TYPE:
|
class DisconnectedSpace
Bases: DisconnectedSpaceExt
, Archetype
Archetype: Spatially disconnect this entity from its parent.
Specifies that the entity path at which this is logged is spatially disconnected from its parent, making it impossible to transform the entity path into its parent's space and vice versa. It only applies to space views that work with spatial transformations, i.e. 2D & 3D space views. This is useful for specifying that a subgraph is independent of the rest of the scene.
Example
Disconnected space:
import rerun as rr
rr.init("rerun_example_disconnected_space", spawn=True)
# These two points can be projected into the same space..
rr.log("world/room1/point", rr.Points3D([[0, 0, 0]]))
rr.log("world/room2/point", rr.Points3D([[1, 1, 1]]))
# ..but this one lives in a completely separate space!
rr.log("world/wormhole", rr.DisconnectedSpace())
rr.log("world/wormhole/point", rr.Points3D([[2, 2, 2]]))
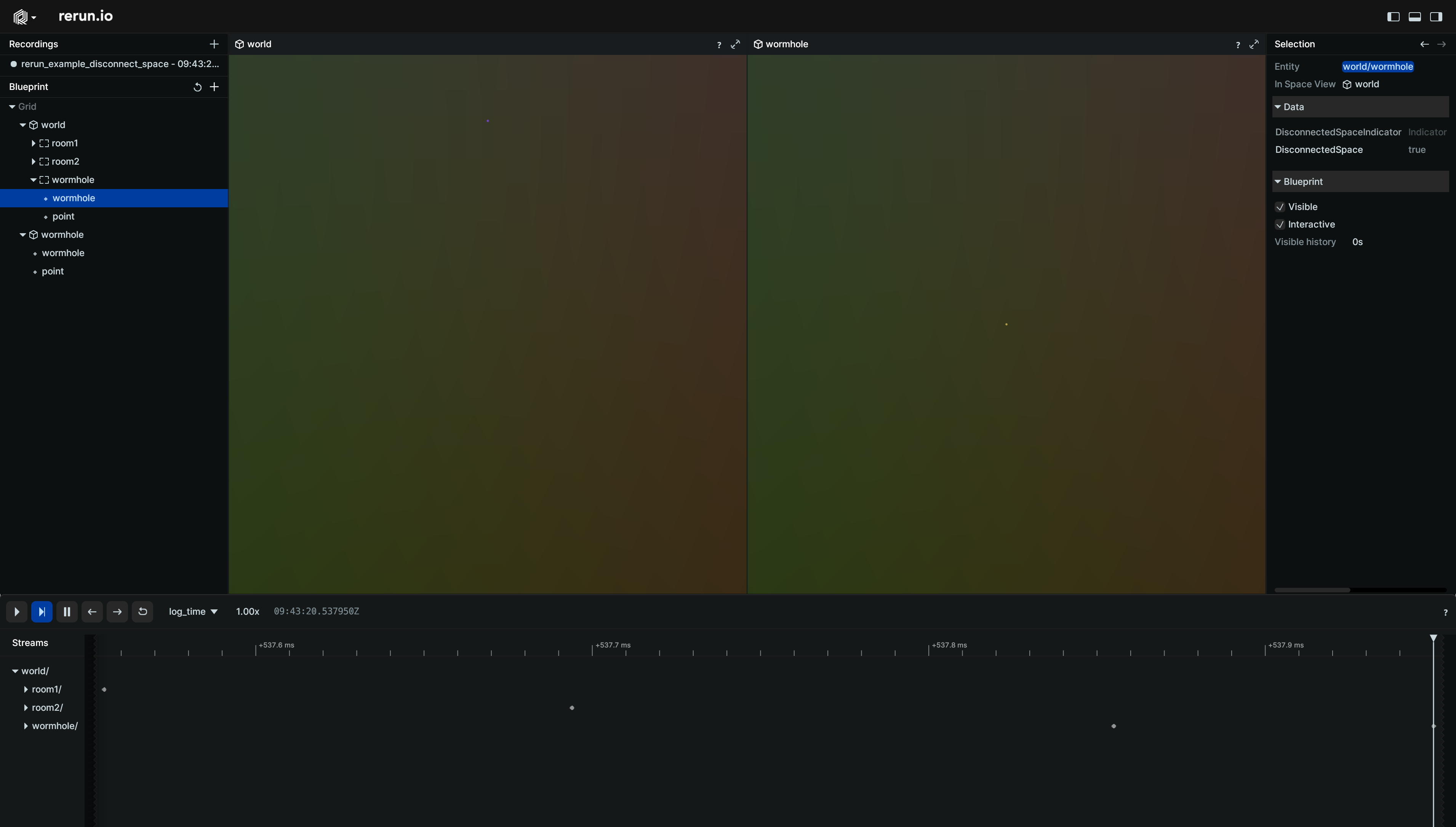
def __init__(is_disconnected=True)
Disconnect an entity from its parent.
PARAMETER | DESCRIPTION |
---|---|
is_disconnected |
Whether or not the entity should be disconnected from the rest of the scene.
Set to
TYPE:
|
class Image
Bases: ImageExt
, Archetype
Archetype: A monochrome or color image.
The shape of the TensorData
must be mappable to:
- A HxW
tensor, treated as a grayscale image.
- A HxWx3
tensor, treated as an RGB image.
- A HxWx4
tensor, treated as an RGBA image.
Leading and trailing unit-dimensions are ignored, so that
1x640x480x3x1
is treated as a 640x480x3
RGB image.
Rerun also supports compressed image encoded as JPEG, N12, and YUY2.
Using these formats can save a lot of bandwidth and memory.
To compress an image, use rerun.Image.compress
.
To pass in an already encoded image, use rerun.ImageEncoded
.
Example
image_simple
:
import numpy as np
import rerun as rr
# Create an image with numpy
image = np.zeros((200, 300, 3), dtype=np.uint8)
image[:, :, 0] = 255
image[50:150, 50:150] = (0, 255, 0)
rr.init("rerun_example_image", spawn=True)
rr.log("image", rr.Image(image))
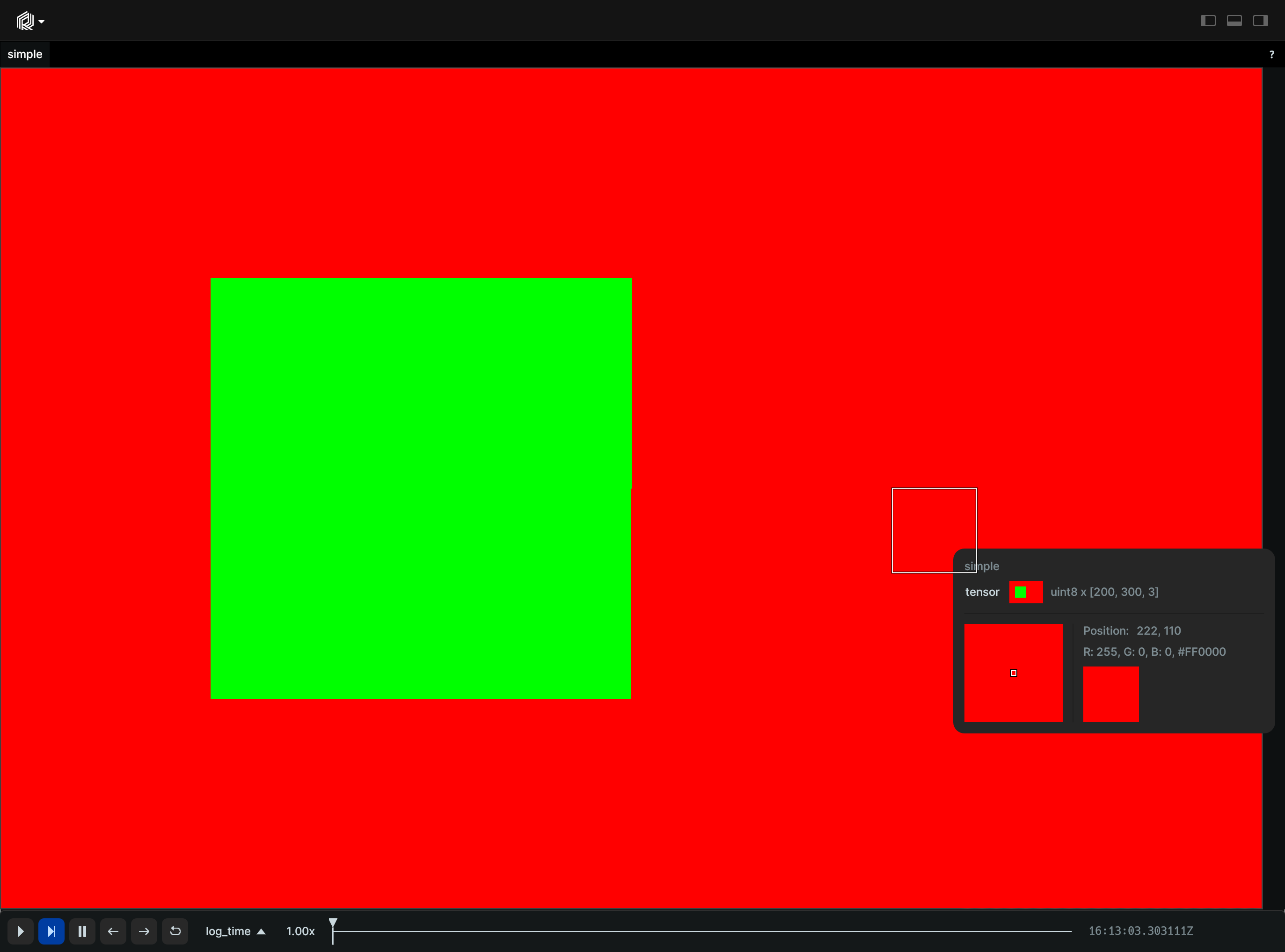
def __init__(data, *, draw_order=None)
Create a new instance of the Image archetype.
PARAMETER | DESCRIPTION |
---|---|
data |
The image data. Should always be a rank-2 or rank-3 tensor.
TYPE:
|
draw_order |
An optional floating point value that specifies the 2D drawing order. Objects with higher values are drawn on top of those with lower values.
TYPE:
|
def compress(*, jpeg_quality=95)
Converts an Image
to an rerun.ImageEncoded
using JPEG compression.
JPEG compression works best for photographs. Only RGB or Mono images are supported, not RGBA. Note that compressing to JPEG costs a bit of CPU time, both when logging and later when viewing them.
PARAMETER | DESCRIPTION |
---|---|
jpeg_quality |
Higher quality = larger file size. A quality of 95 still saves a lot of space, but is visually very similar.
TYPE:
|
class LineStrips2D
Bases: Archetype
Archetype: 2D line strips with positions and optional colors, radii, labels, etc.
Example
line_strip2d_batch
:
import rerun as rr
rr.init("rerun_example_line_strip2d_batch", spawn=True)
rr.log(
"strips",
rr.LineStrips2D(
[
[[0, 0], [2, 1], [4, -1], [6, 0]],
[[0, 3], [1, 4], [2, 2], [3, 4], [4, 2], [5, 4], [6, 3]],
],
colors=[[255, 0, 0], [0, 255, 0]],
radii=[0.025, 0.005],
labels=["one strip here", "and one strip there"],
),
)
# Log an extra rect to set the view bounds
rr.log("bounds", rr.Boxes2D(centers=[3, 1.5], half_sizes=[4.0, 4.5]))
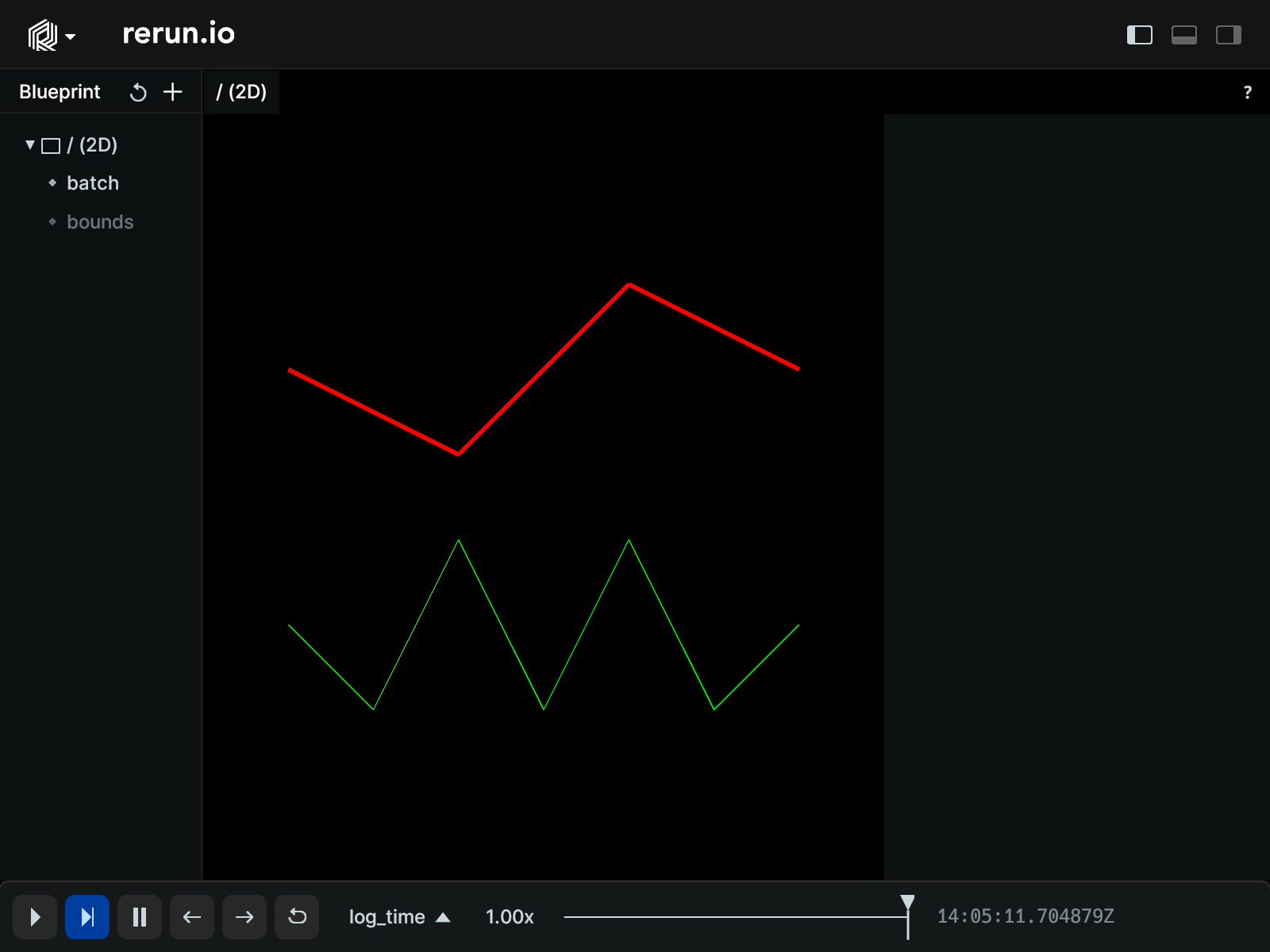
def __init__(strips, *, radii=None, colors=None, labels=None, draw_order=None, class_ids=None)
Create a new instance of the LineStrips2D archetype.
PARAMETER | DESCRIPTION |
---|---|
strips |
All the actual 2D line strips that make up the batch.
TYPE:
|
radii |
Optional radii for the line strips.
TYPE:
|
colors |
Optional colors for the line strips.
TYPE:
|
labels |
Optional text labels for the line strips.
TYPE:
|
draw_order |
An optional floating point value that specifies the 2D drawing order of each line strip. Objects with higher values are drawn on top of those with lower values.
TYPE:
|
class_ids |
Optional The class ID provides colors and labels if not specified explicitly.
TYPE:
|
class LineStrips3D
Bases: Archetype
Archetype: 3D line strips with positions and optional colors, radii, labels, etc.
Example
Many strips:
import rerun as rr
rr.init("rerun_example_line_strip3d_batch", spawn=True)
rr.log(
"strips",
rr.LineStrips3D(
[
[
[0, 0, 2],
[1, 0, 2],
[1, 1, 2],
[0, 1, 2],
],
[
[0, 0, 0],
[0, 0, 1],
[1, 0, 0],
[1, 0, 1],
[1, 1, 0],
[1, 1, 1],
[0, 1, 0],
[0, 1, 1],
],
],
colors=[[255, 0, 0], [0, 255, 0]],
radii=[0.025, 0.005],
labels=["one strip here", "and one strip there"],
),
)
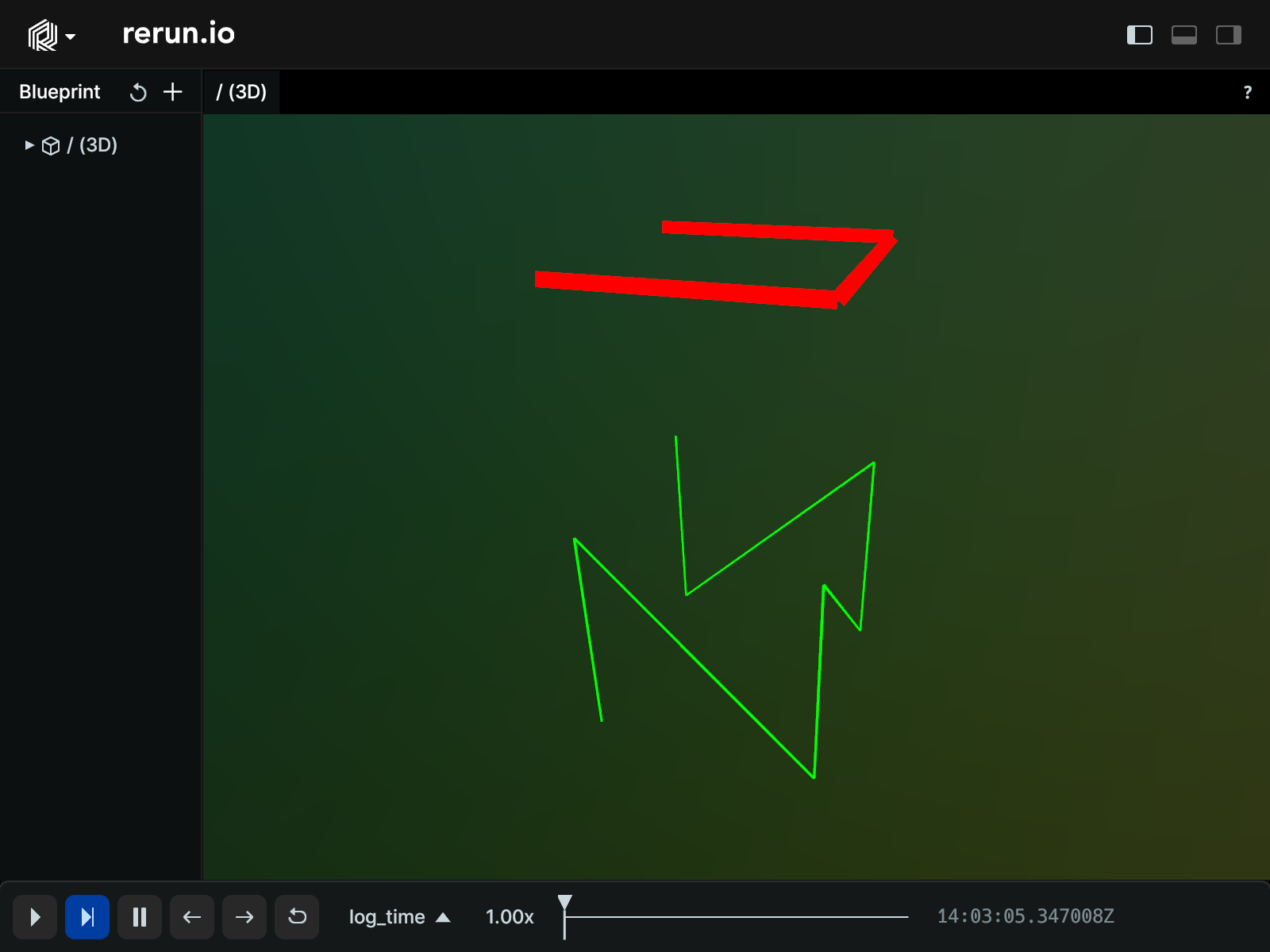
def __init__(strips, *, radii=None, colors=None, labels=None, class_ids=None)
Create a new instance of the LineStrips3D archetype.
PARAMETER | DESCRIPTION |
---|---|
strips |
All the actual 3D line strips that make up the batch.
TYPE:
|
radii |
Optional radii for the line strips.
TYPE:
|
colors |
Optional colors for the line strips.
TYPE:
|
labels |
Optional text labels for the line strips.
TYPE:
|
class_ids |
Optional The class ID provides colors and labels if not specified explicitly.
TYPE:
|
class Mesh3D
Bases: Mesh3DExt
, Archetype
Archetype: A 3D triangle mesh as specified by its per-mesh and per-vertex properties.
See also Asset3D
.
Example
Simple indexed 3D mesh:
import rerun as rr
rr.init("rerun_example_mesh3d_indexed", spawn=True)
rr.log(
"triangle",
rr.Mesh3D(
vertex_positions=[[0.0, 1.0, 0.0], [1.0, 0.0, 0.0], [0.0, 0.0, 0.0]],
vertex_normals=[0.0, 0.0, 1.0],
vertex_colors=[[0, 0, 255], [0, 255, 0], [255, 0, 0]],
indices=[2, 1, 0],
),
)
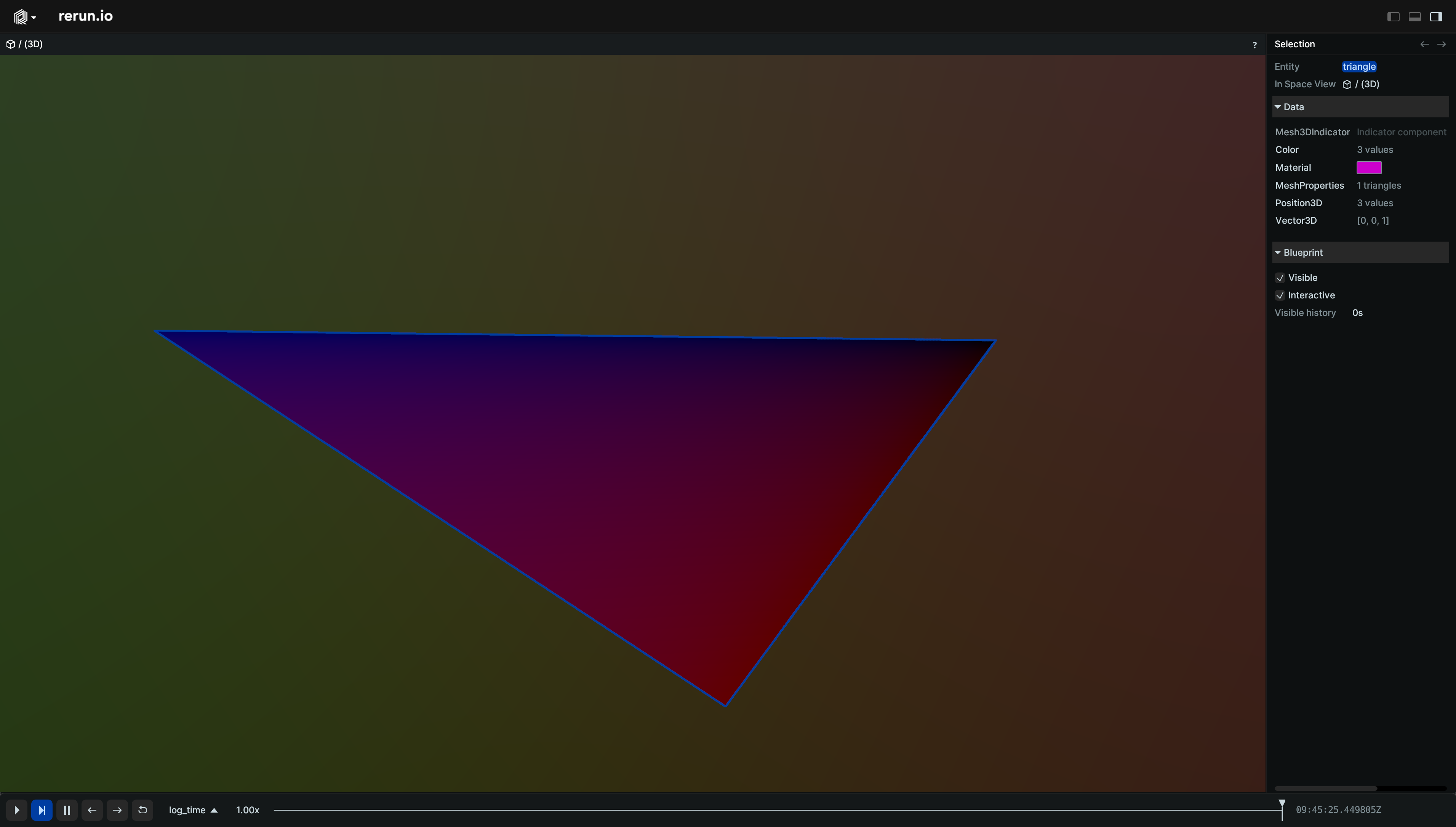
def __init__(*, vertex_positions, indices=None, mesh_properties=None, vertex_normals=None, vertex_colors=None, vertex_texcoords=None, albedo_texture=None, mesh_material=None, class_ids=None)
Create a new instance of the Mesh3D archetype.
PARAMETER | DESCRIPTION |
---|---|
vertex_positions |
The positions of each vertex.
If no
TYPE:
|
indices |
If specified, a flattened array of indices that describe the mesh's triangles,
i.e. its length must be divisible by 3.
Mutually exclusive with
TYPE:
|
mesh_properties |
Optional properties for the mesh as a whole (including indexed drawing).
Mutually exclusive with
TYPE:
|
vertex_normals |
An optional normal for each vertex.
If specified, this must have as many elements as
TYPE:
|
vertex_texcoords |
An optional texture coordinate for each vertex.
If specified, this must have as many elements as
TYPE:
|
vertex_colors |
An optional color for each vertex.
TYPE:
|
mesh_material |
Optional material properties for the mesh as a whole.
TYPE:
|
albedo_texture |
Optional albedo texture. Used with
TYPE:
|
class_ids |
Optional class Ids for the vertices. The class ID provides colors and labels if not specified explicitly.
TYPE:
|
class Pinhole
Bases: PinholeExt
, Archetype
Archetype: Camera perspective projection (a.k.a. intrinsics).
Examples:
Simple pinhole camera:
import numpy as np
import rerun as rr
rr.init("rerun_example_pinhole", spawn=True)
rng = np.random.default_rng(12345)
image = rng.uniform(0, 255, size=[3, 3, 3])
rr.log("world/image", rr.Pinhole(focal_length=3, width=3, height=3))
rr.log("world/image", rr.Image(image))
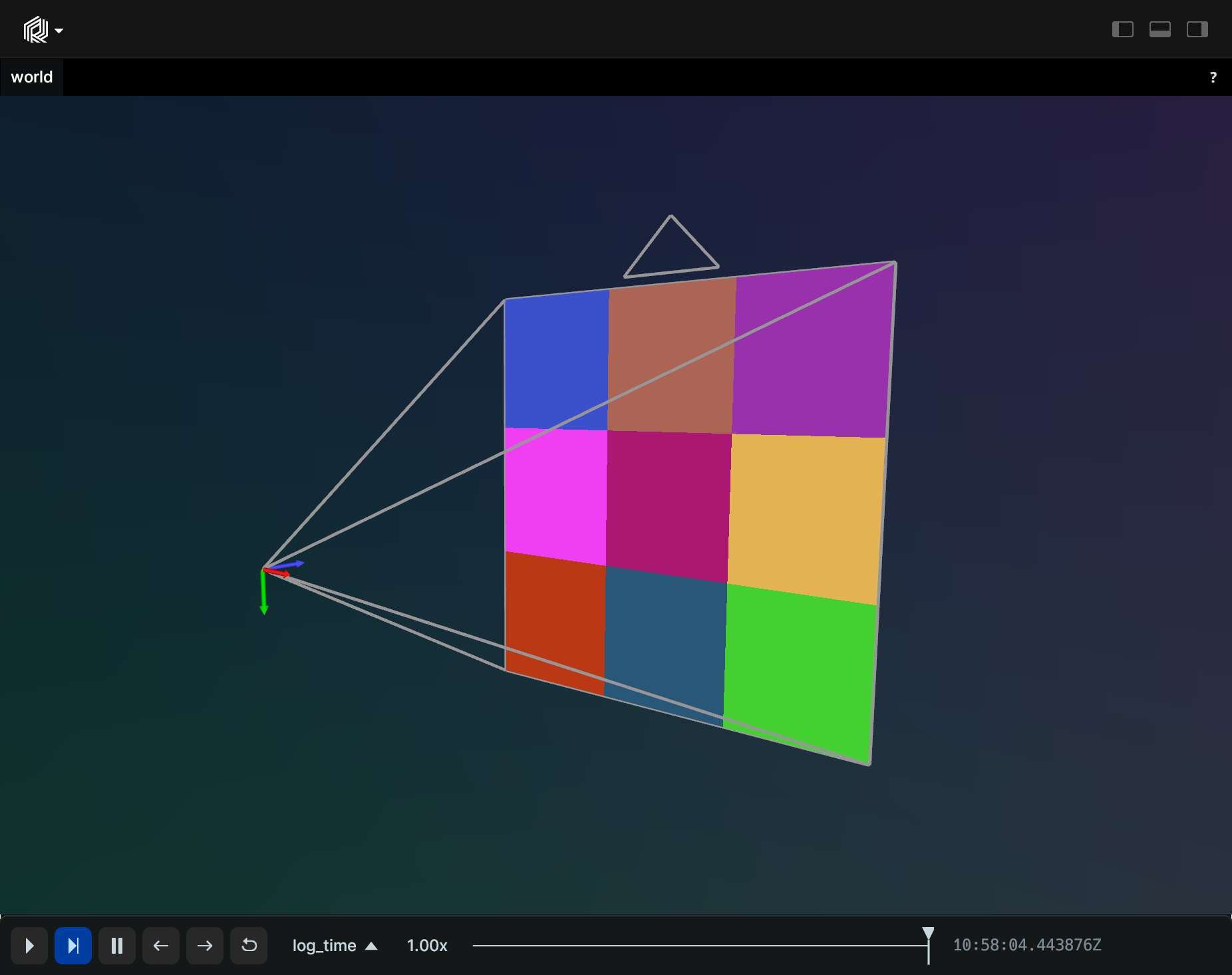
Perspective pinhole camera:
import rerun as rr
rr.init("rerun_example_pinhole_perspective", spawn=True)
rr.log("world/cam", rr.Pinhole(fov_y=0.7853982, aspect_ratio=1.7777778, camera_xyz=rr.ViewCoordinates.RUB))
rr.log("world/points", rr.Points3D([(0.0, 0.0, -0.5), (0.1, 0.1, -0.5), (-0.1, -0.1, -0.5)]))
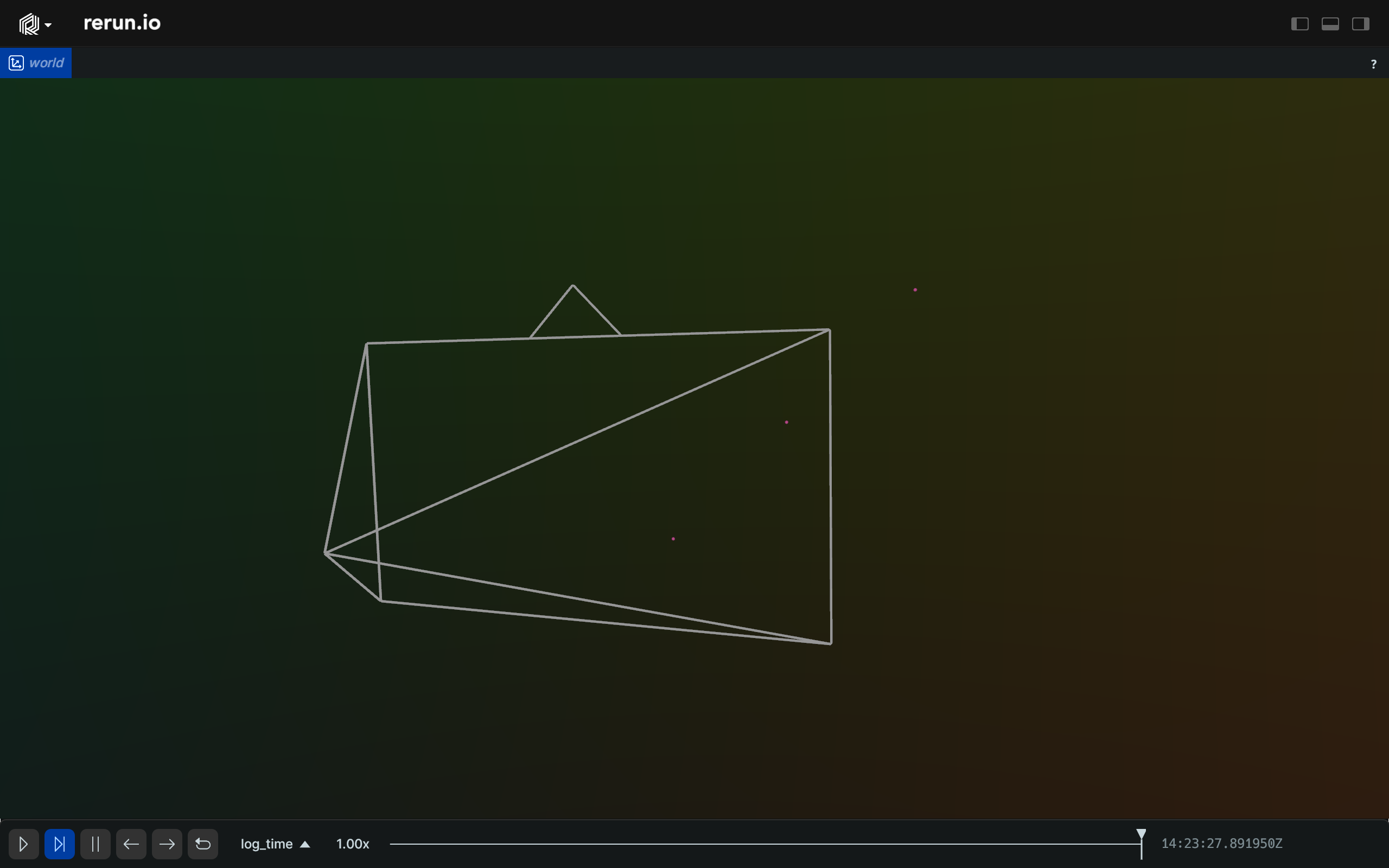
def __init__(*, image_from_camera=None, resolution=None, camera_xyz=None, width=None, height=None, focal_length=None, principal_point=None, fov_y=None, aspect_ratio=None)
Create a new instance of the Pinhole archetype.
PARAMETER | DESCRIPTION |
---|---|
image_from_camera |
Row-major intrinsics matrix for projecting from camera space to image space.
The first two axes are X=Right and Y=Down, respectively.
Projection is done along the positive third (Z=Forward) axis.
This can be specified instead of
TYPE:
|
resolution |
Pixel resolution (usually integers) of child image space. Width and height.
TYPE:
|
camera_xyz |
Sets the view coordinates for the camera. All common values are available as constants on the The default is The camera frustum will point whichever axis is set to The frustum's "up" direction will be whichever axis is set to The frustum's "right" direction will be whichever axis is set to Other common formats are NOTE: setting this to something else than The pinhole matrix (the
TYPE:
|
focal_length |
The focal length of the camera in pixels. This is the diagonal of the projection matrix. Set one value for symmetric cameras, or two values (X=Right, Y=Down) for anamorphic cameras.
TYPE:
|
principal_point |
The center of the camera in pixels. The default is half the width and height. This is the last column of the projection matrix. Expects two values along the dimensions Right and Down
TYPE:
|
width |
Width of the image in pixels. |
height |
Height of the image in pixels. |
fov_y |
Vertical field of view in radians.
TYPE:
|
aspect_ratio |
Aspect ratio (width/height).
TYPE:
|
class Points2D
Bases: Points2DExt
, Archetype
Archetype: A 2D point cloud with positions and optional colors, radii, labels, etc.
Example
Randomly distributed 2D points with varying color and radius:
import rerun as rr
from numpy.random import default_rng
rr.init("rerun_example_points2d_random", spawn=True)
rng = default_rng(12345)
positions = rng.uniform(-3, 3, size=[10, 2])
colors = rng.uniform(0, 255, size=[10, 4])
radii = rng.uniform(0, 1, size=[10])
rr.log("random", rr.Points2D(positions, colors=colors, radii=radii))
# Log an extra rect to set the view bounds
rr.log("bounds", rr.Boxes2D(half_sizes=[4, 3]))
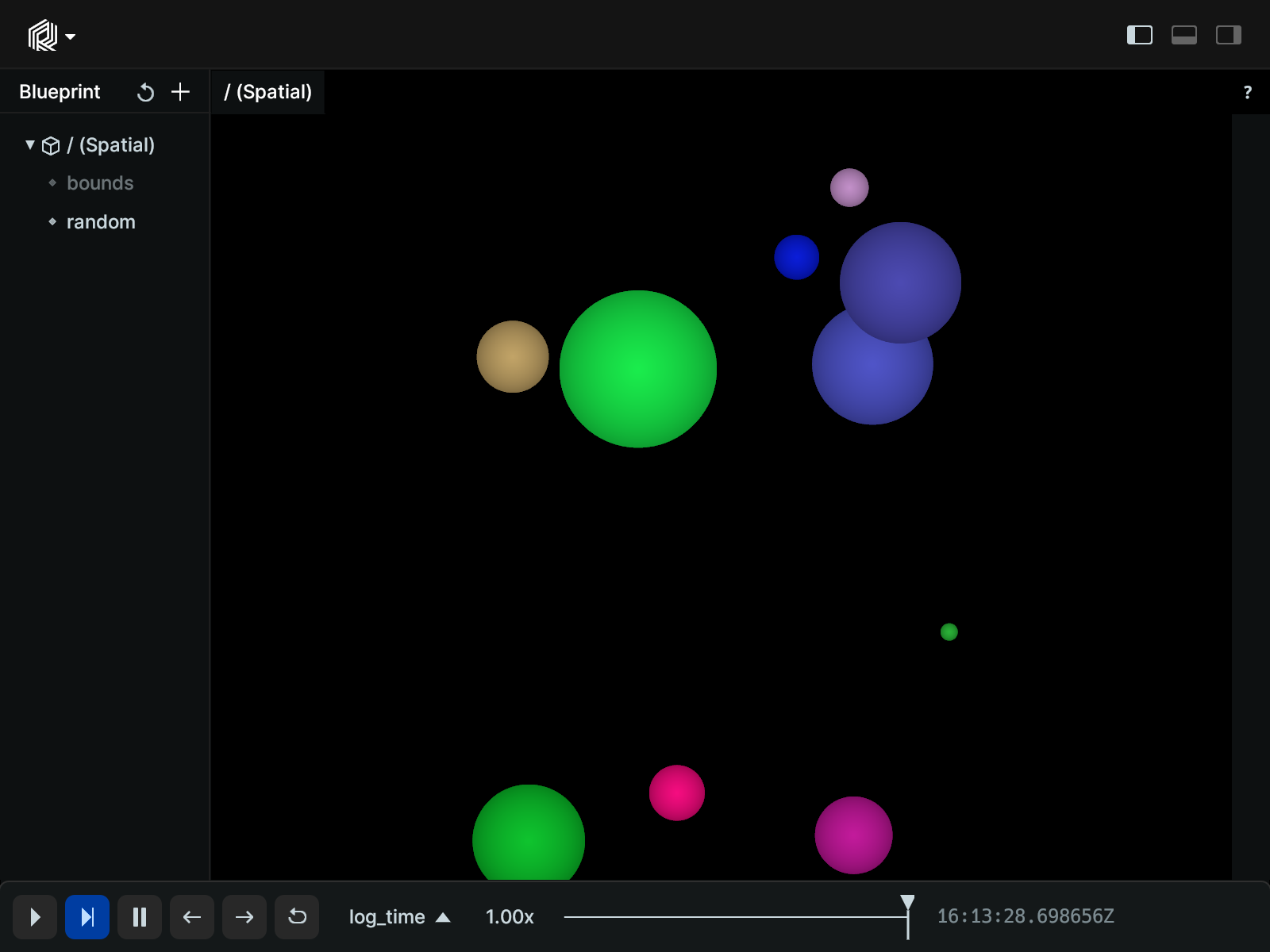
def __init__(positions, *, radii=None, colors=None, labels=None, draw_order=None, class_ids=None, keypoint_ids=None)
Create a new instance of the Points2D archetype.
PARAMETER | DESCRIPTION |
---|---|
positions |
All the 2D positions at which the point cloud shows points.
TYPE:
|
radii |
Optional radii for the points, effectively turning them into circles.
TYPE:
|
colors |
Optional colors for the points. The colors are interpreted as RGB or RGBA in sRGB gamma-space, As either 0-1 floats or 0-255 integers, with separate alpha.
TYPE:
|
labels |
Optional text labels for the points.
TYPE:
|
draw_order |
An optional floating point value that specifies the 2D drawing order. Objects with higher values are drawn on top of those with lower values.
TYPE:
|
class_ids |
Optional class Ids for the points. The class ID provides colors and labels if not specified explicitly.
TYPE:
|
keypoint_ids |
Optional keypoint IDs for the points, identifying them within a class. If keypoint IDs are passed in but no class IDs were specified, the class ID will
default to 0.
This is useful to identify points within a single classification (which is identified
with
TYPE:
|
class Points3D
Bases: Points3DExt
, Archetype
Archetype: A 3D point cloud with positions and optional colors, radii, labels, etc.
Example
Randomly distributed 3D points with varying color and radius:
import rerun as rr
from numpy.random import default_rng
rr.init("rerun_example_points3d_random", spawn=True)
rng = default_rng(12345)
positions = rng.uniform(-5, 5, size=[10, 3])
colors = rng.uniform(0, 255, size=[10, 3])
radii = rng.uniform(0, 1, size=[10])
rr.log("random", rr.Points3D(positions, colors=colors, radii=radii))
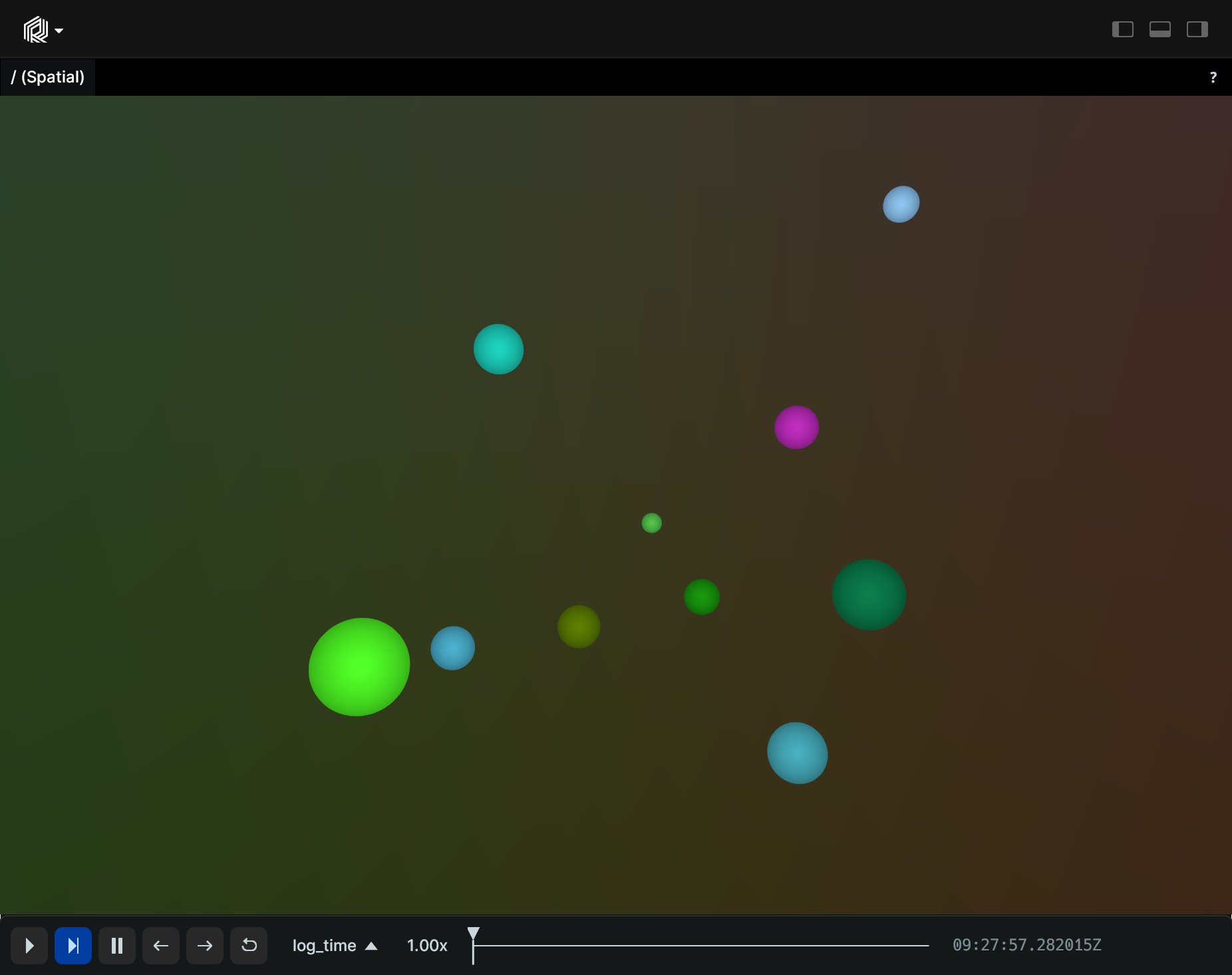
def __init__(positions, *, radii=None, colors=None, labels=None, class_ids=None, keypoint_ids=None)
Create a new instance of the Points3D archetype.
PARAMETER | DESCRIPTION |
---|---|
positions |
All the 3D positions at which the point cloud shows points.
TYPE:
|
radii |
Optional radii for the points, effectively turning them into circles.
TYPE:
|
colors |
Optional colors for the points. The colors are interpreted as RGB or RGBA in sRGB gamma-space, As either 0-1 floats or 0-255 integers, with separate alpha.
TYPE:
|
labels |
Optional text labels for the points.
TYPE:
|
class_ids |
Optional class Ids for the points. The class ID provides colors and labels if not specified explicitly.
TYPE:
|
keypoint_ids |
Optional keypoint IDs for the points, identifying them within a class. If keypoint IDs are passed in but no class IDs were specified, the class ID will
default to 0.
This is useful to identify points within a single classification (which is identified
with
TYPE:
|
class Scalar
Bases: Archetype
Archetype: Log a double-precision scalar.
The current timeline value will be used for the time/X-axis, hence scalars cannot be timeless.
When used to produce a plot, this archetype is used to provide the data that
is referenced by the SeriesLine
or SeriesPoint
archetypes. You can do
this by logging both archetypes to the same path, or alternatively configuring
the plot-specific archetypes through the blueprint.
See also SeriesPoint
, SeriesLine
.
Example
Simple line plot:
import math
import rerun as rr
rr.init("rerun_example_scalar", spawn=True)
# Log the data on a timeline called "step".
for step in range(0, 64):
rr.set_time_sequence("step", step)
rr.log("scalar", rr.Scalar(math.sin(step / 10.0)))
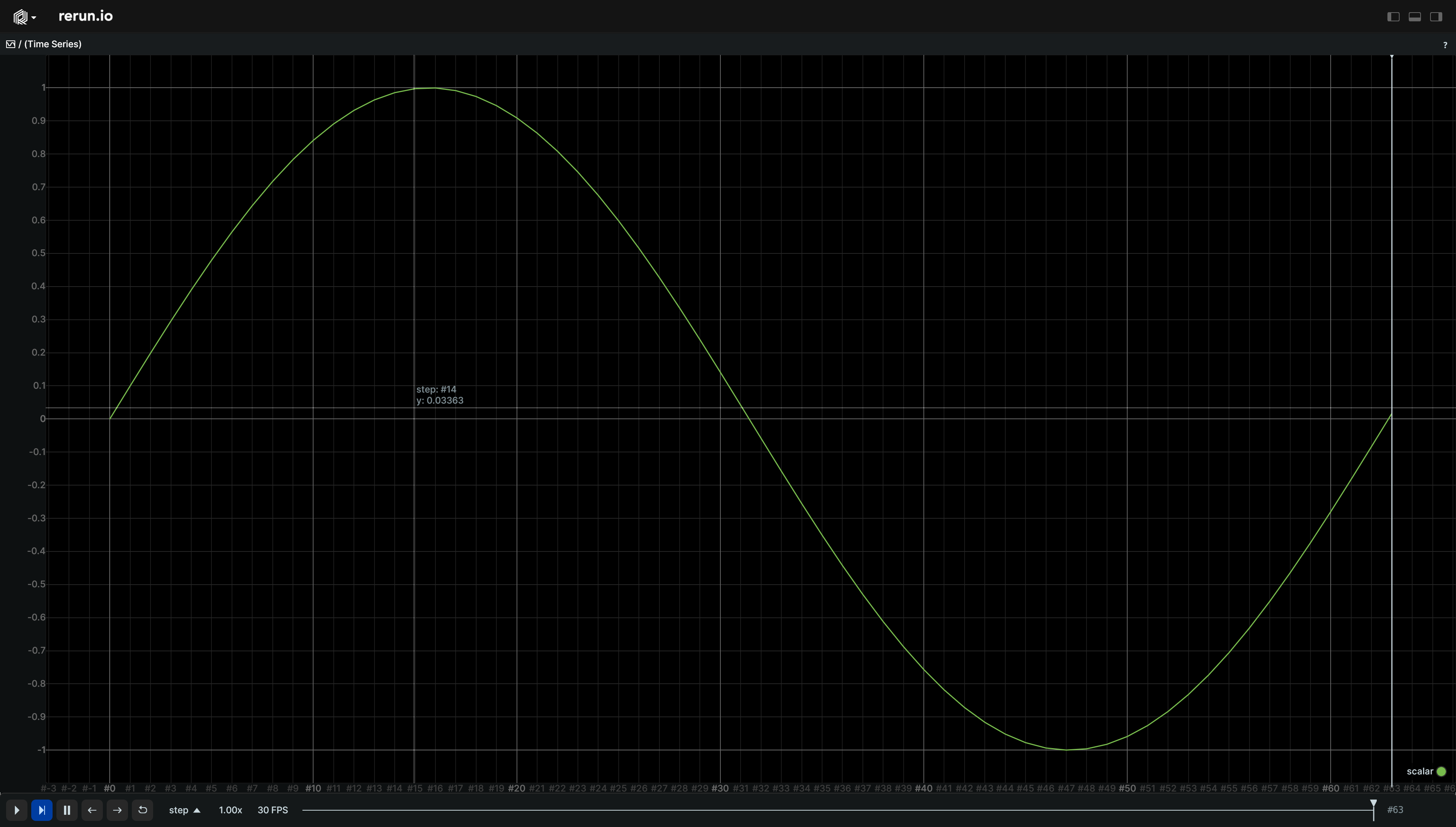
def __init__(scalar)
Create a new instance of the Scalar archetype.
PARAMETER | DESCRIPTION |
---|---|
scalar |
The scalar value to log.
TYPE:
|
class SegmentationImage
Bases: SegmentationImageExt
, Archetype
Archetype: An image made up of integer class-ids.
The shape of the TensorData
must be mappable to an HxW
tensor.
Each pixel corresponds to a class-id that will be mapped to a color based on annotation context.
In the case of floating point images, the label will be looked up based on rounding to the nearest integer value.
Leading and trailing unit-dimensions are ignored, so that
1x640x480x1
is treated as a 640x480
image.
See also AnnotationContext
to associate each class with a color and a label.
Example
Simple segmentation image:
import numpy as np
import rerun as rr
# Create a segmentation image
image = np.zeros((8, 12), dtype=np.uint8)
image[0:4, 0:6] = 1
image[4:8, 6:12] = 2
rr.init("rerun_example_segmentation_image", spawn=True)
# Assign a label and color to each class
rr.log("/", rr.AnnotationContext([(1, "red", (255, 0, 0)), (2, "green", (0, 255, 0))]), timeless=True)
rr.log("image", rr.SegmentationImage(image))
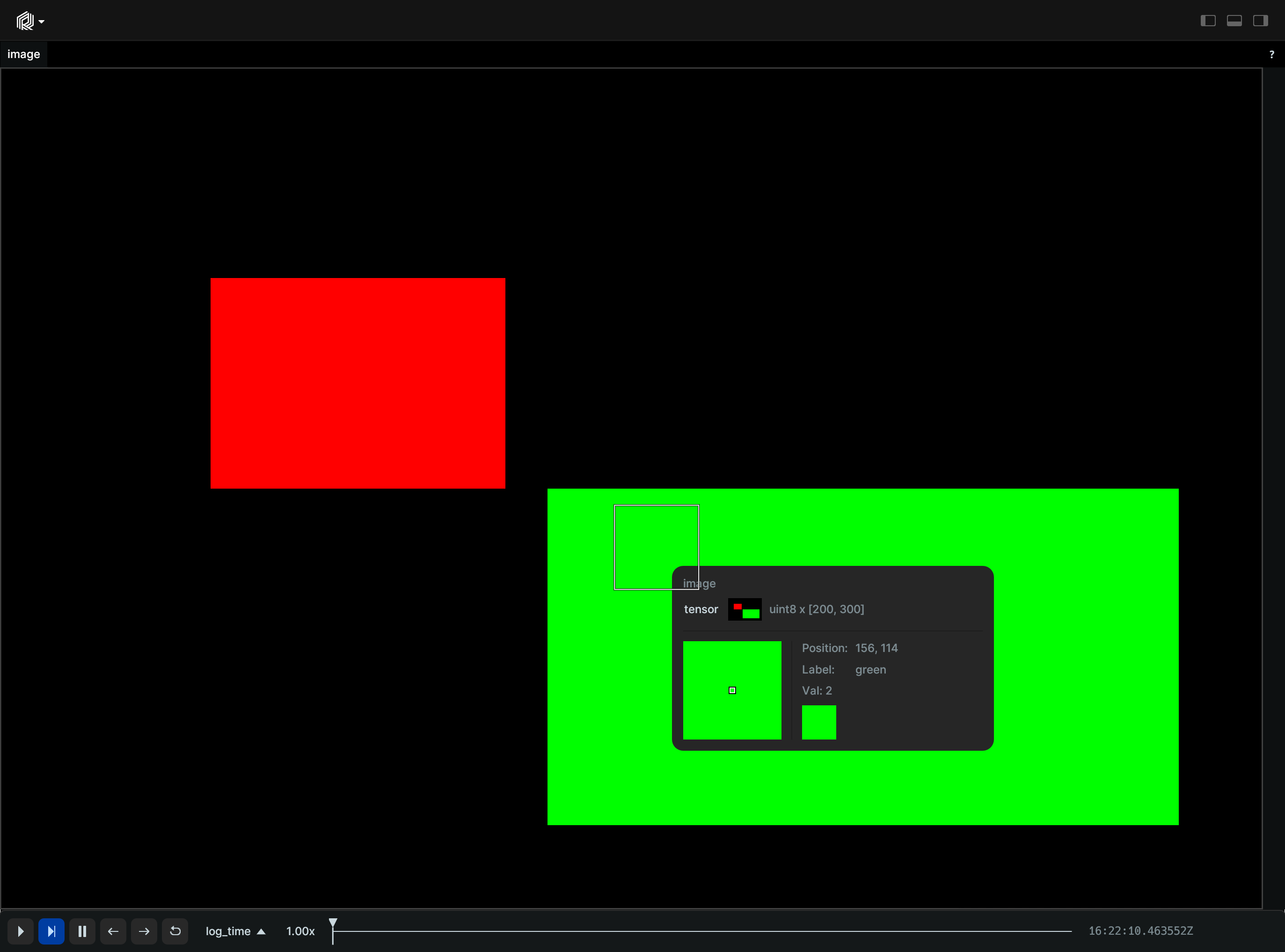
def __init__(data, *, draw_order=None)
Create a new instance of the SegmentationImage archetype.
PARAMETER | DESCRIPTION |
---|---|
data |
The image data. Should always be a rank-2 tensor.
TYPE:
|
draw_order |
An optional floating point value that specifies the 2D drawing order. Objects with higher values are drawn on top of those with lower values.
TYPE:
|
class SeriesLine
Bases: Archetype
Archetype: Define the style properties for a line series in a chart.
This archetype only provides styling information and should be logged as timeless
when possible. The underlying data needs to be logged to the same entity-path using
the Scalar
archetype.
See Scalar
Example
Line series:
from math import cos, sin, tau
import rerun as rr
rr.init("rerun_example_series_line_style", spawn=True)
# Set up plot styling:
# They are logged timeless as they don't change over time and apply to all timelines.
# Log two lines series under a shared root so that they show in the same plot by default.
rr.log("trig/sin", rr.SeriesLine(color=[255, 0, 0], name="sin(0.01t)", width=2), timeless=True)
rr.log("trig/cos", rr.SeriesLine(color=[0, 255, 0], name="cos(0.01t)", width=4), timeless=True)
# Log the data on a timeline called "step".
for t in range(0, int(tau * 2 * 100.0)):
rr.set_time_sequence("step", t)
rr.log("trig/sin", rr.Scalar(sin(float(t) / 100.0)))
rr.log("trig/cos", rr.Scalar(cos(float(t) / 100.0)))
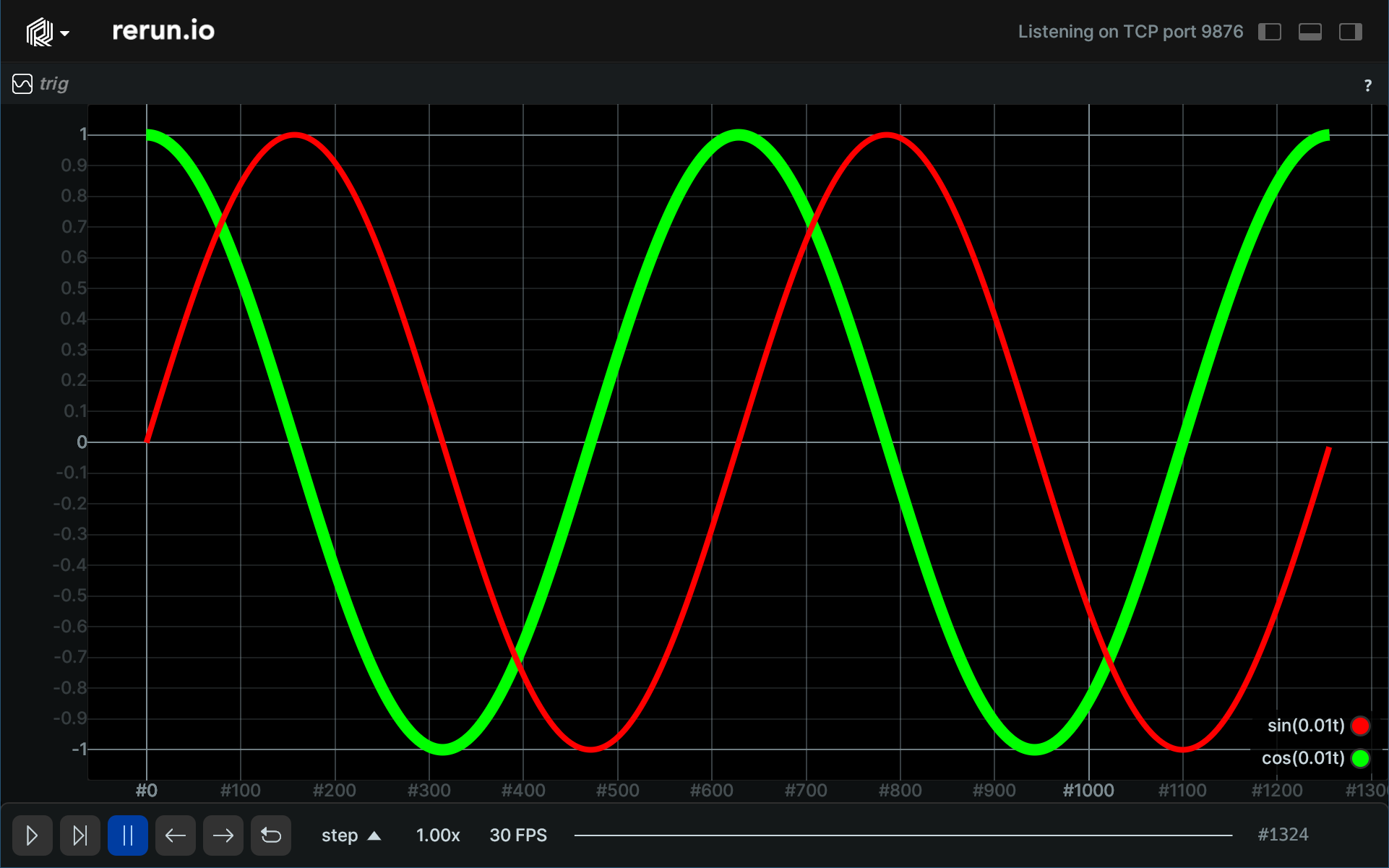
def __init__(*, color=None, width=None, name=None)
Create a new instance of the SeriesLine archetype.
PARAMETER | DESCRIPTION |
---|---|
color |
Color for the corresponding series.
TYPE:
|
width |
Stroke width for the corresponding series.
TYPE:
|
name |
Display name of the series. Used in the legend.
TYPE:
|
class SeriesPoint
Bases: Archetype
Archetype: Define the style properties for a point series in a chart.
This archetype only provides styling information and should be logged as timeless
when possible. The underlying data needs to be logged to the same entity-path using
the Scalar
archetype.
See Scalar
Example
Point series:
from math import cos, sin, tau
import rerun as rr
rr.init("rerun_example_series_point_style", spawn=True)
# Set up plot styling:
# They are logged timeless as they don't change over time and apply to all timelines.
# Log two point series under a shared root so that they show in the same plot by default.
rr.log(
"trig/sin",
rr.SeriesPoint(
color=[255, 0, 0],
name="sin(0.01t)",
marker="circle",
marker_size=4,
),
timeless=True,
)
rr.log(
"trig/cos",
rr.SeriesPoint(
color=[0, 255, 0],
name="cos(0.01t)",
marker="cross",
marker_size=2,
),
timeless=True,
)
# Log the data on a timeline called "step".
for t in range(0, int(tau * 2 * 10.0)):
rr.set_time_sequence("step", t)
rr.log("trig/sin", rr.Scalar(sin(float(t) / 10.0)))
rr.log("trig/cos", rr.Scalar(cos(float(t) / 10.0)))
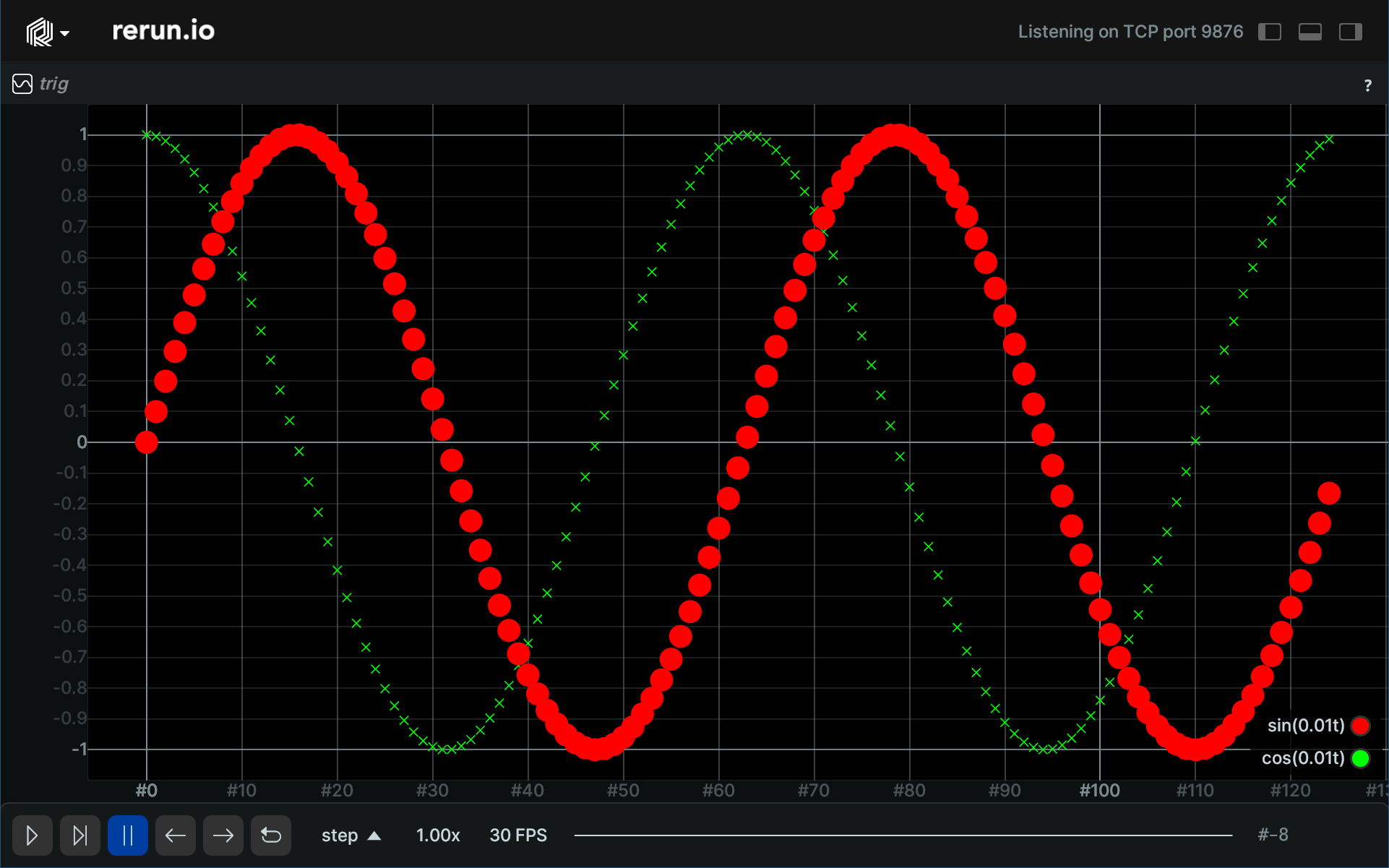
def __init__(*, color=None, marker=None, name=None, marker_size=None)
Create a new instance of the SeriesPoint archetype.
PARAMETER | DESCRIPTION |
---|---|
color |
Color for the corresponding series.
TYPE:
|
marker |
What shape to use to represent the point
TYPE:
|
name |
Display name of the series. Used in the legend.
TYPE:
|
marker_size |
Size of the marker.
TYPE:
|
class Tensor
Bases: TensorExt
, Archetype
Archetype: A generic n-dimensional Tensor.
Example
Simple tensor:
import numpy as np
import rerun as rr
tensor = np.random.randint(0, 256, (8, 6, 3, 5), dtype=np.uint8) # 4-dimensional tensor
rr.init("rerun_example_tensor", spawn=True)
# Log the tensor, assigning names to each dimension
rr.log("tensor", rr.Tensor(tensor, dim_names=("width", "height", "channel", "batch")))
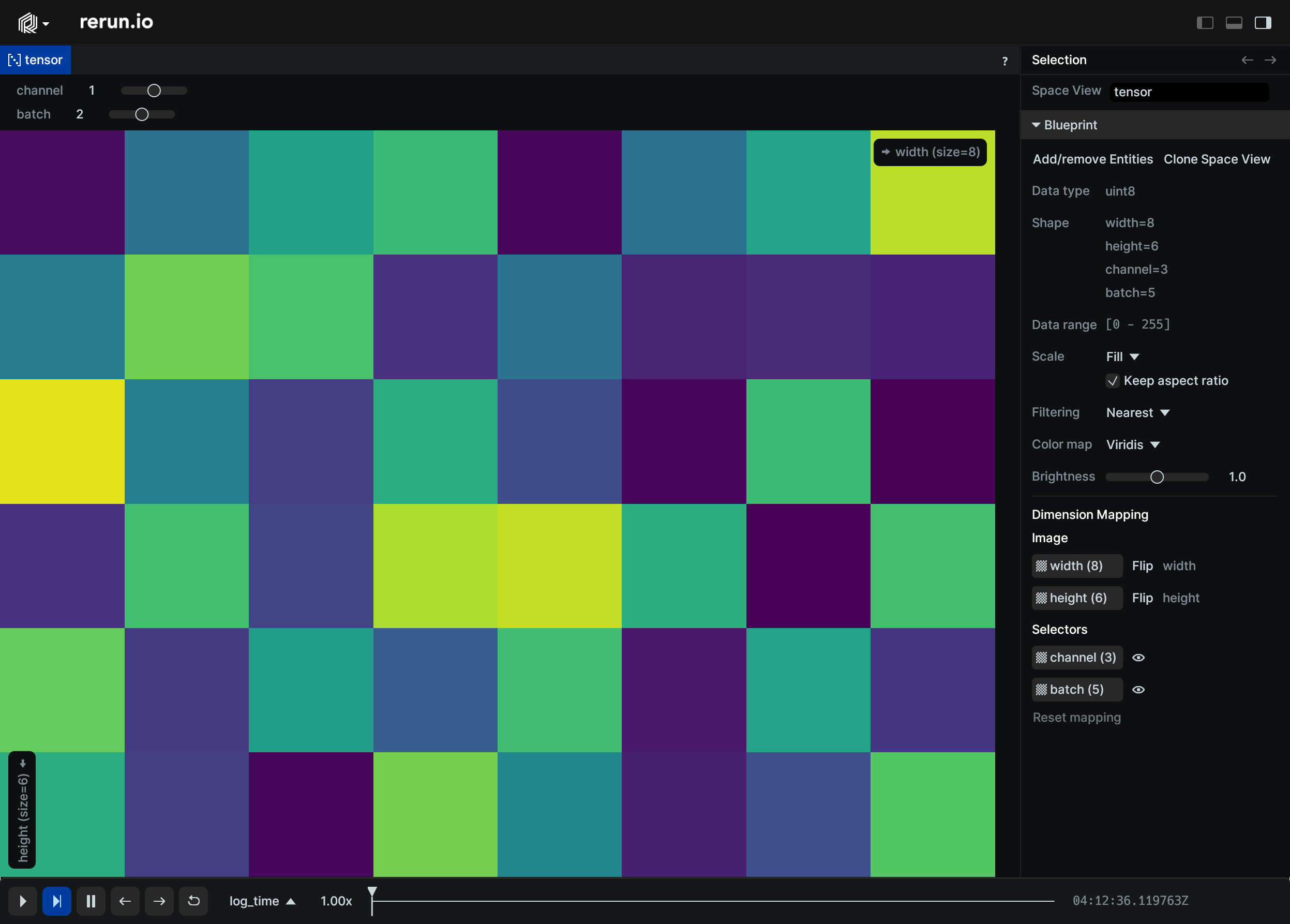
def __init__(data=None, *, dim_names=None)
Construct a Tensor
archetype.
The Tensor
archetype internally contains a single component: TensorData
.
See the TensorData
constructor for more advanced options to interpret buffers
as TensorData
of varying shapes.
For simple cases, you can pass array objects and optionally specify the names of
the dimensions. The shape of the TensorData
will be inferred from the array.
PARAMETER | DESCRIPTION |
---|---|
self |
The TensorData object to construct.
TYPE:
|
data |
A TensorData object, or type that can be converted to a numpy array.
TYPE:
|
dim_names |
The names of the tensor dimensions when generating the shape from an array. |
class TextDocument
Bases: Archetype
Archetype: A text element intended to be displayed in its own text-box.
Supports raw text and markdown.
Example
Markdown text document:
import rerun as rr
rr.init("rerun_example_text_document", spawn=True)
rr.log("text_document", rr.TextDocument("Hello, TextDocument!"))
rr.log(
"markdown",
rr.TextDocument(
'''
# Hello Markdown!
[Click here to see the raw text](recording://markdown:Text).
Basic formatting:
| **Feature** | **Alternative** |
| ----------------- | --------------- |
| Plain | |
| *italics* | _italics_ |
| **bold** | __bold__ |
| ~~strikethrough~~ | |
| `inline code` | |
----------------------------------
## Support
- [x] [Commonmark](https://commonmark.org/help/) support
- [x] GitHub-style strikethrough, tables, and checkboxes
- Basic syntax highlighting for:
- [x] C and C++
- [x] Python
- [x] Rust
- [ ] Other languages
## Links
You can link to [an entity](recording://markdown),
a [specific instance of an entity](recording://markdown[#0]),
or a [specific component](recording://markdown:Text).
Of course you can also have [normal https links](https://github.com/rerun-io/rerun), e.g. <https://rerun.io>.
## Image

'''.strip(),
media_type=rr.MediaType.MARKDOWN,
),
)
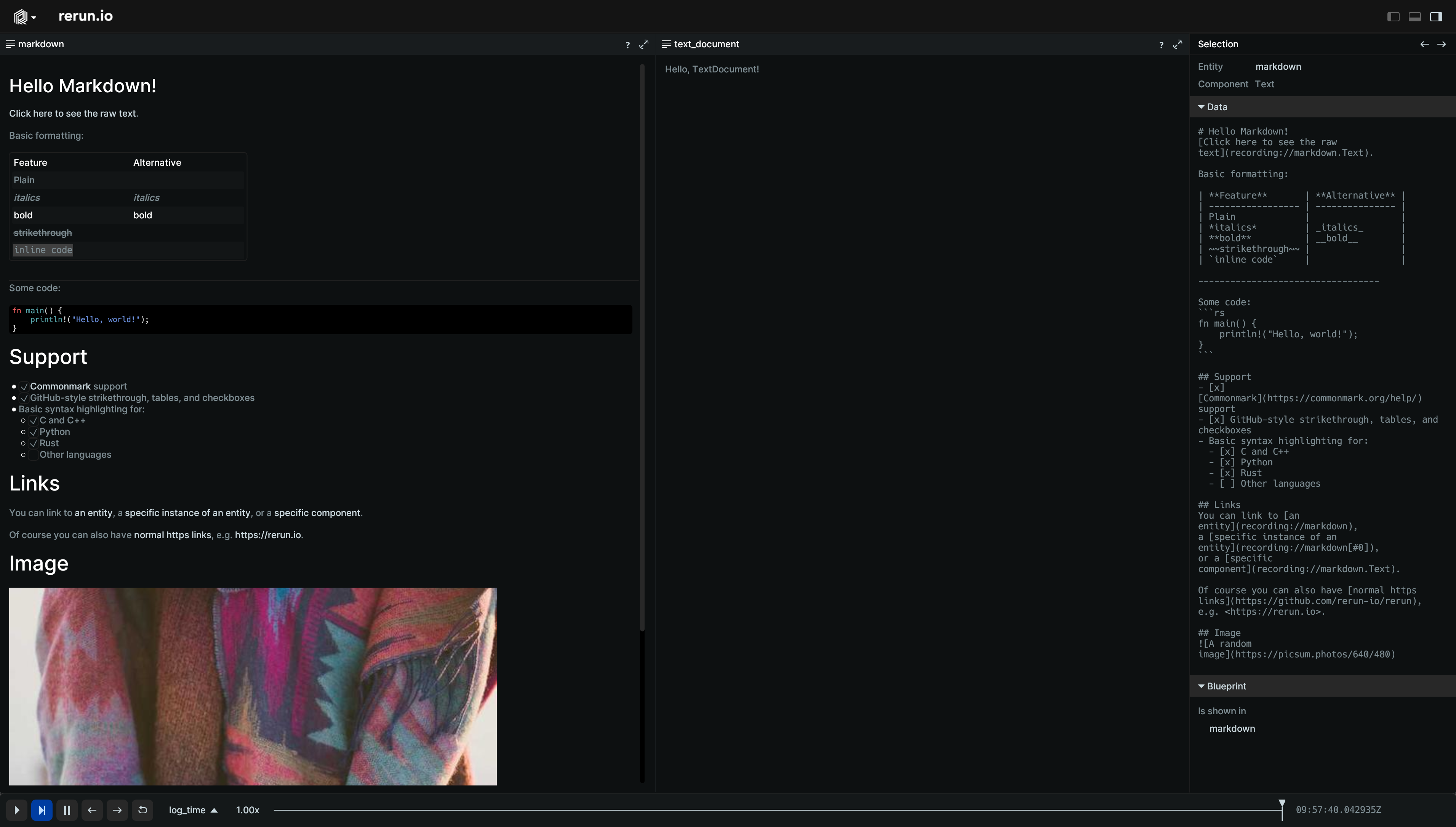
def __init__(text, *, media_type=None)
Create a new instance of the TextDocument archetype.
PARAMETER | DESCRIPTION |
---|---|
text |
Contents of the text document.
TYPE:
|
media_type |
The Media Type of the text. For instance:
* If omitted,
TYPE:
|
class TextLog
Bases: Archetype
Archetype: A log entry in a text log, comprised of a text body and its log level.
Example
text_log_integration
:
import logging
import rerun as rr
rr.init("rerun_example_text_log_integration", spawn=True)
# Log a text entry directly
rr.log("logs", rr.TextLog("this entry has loglevel TRACE", level=rr.TextLogLevel.TRACE))
# Or log via a logging handler
logging.getLogger().addHandler(rr.LoggingHandler("logs/handler"))
logging.getLogger().setLevel(-1)
logging.info("This INFO log got added through the standard logging interface")
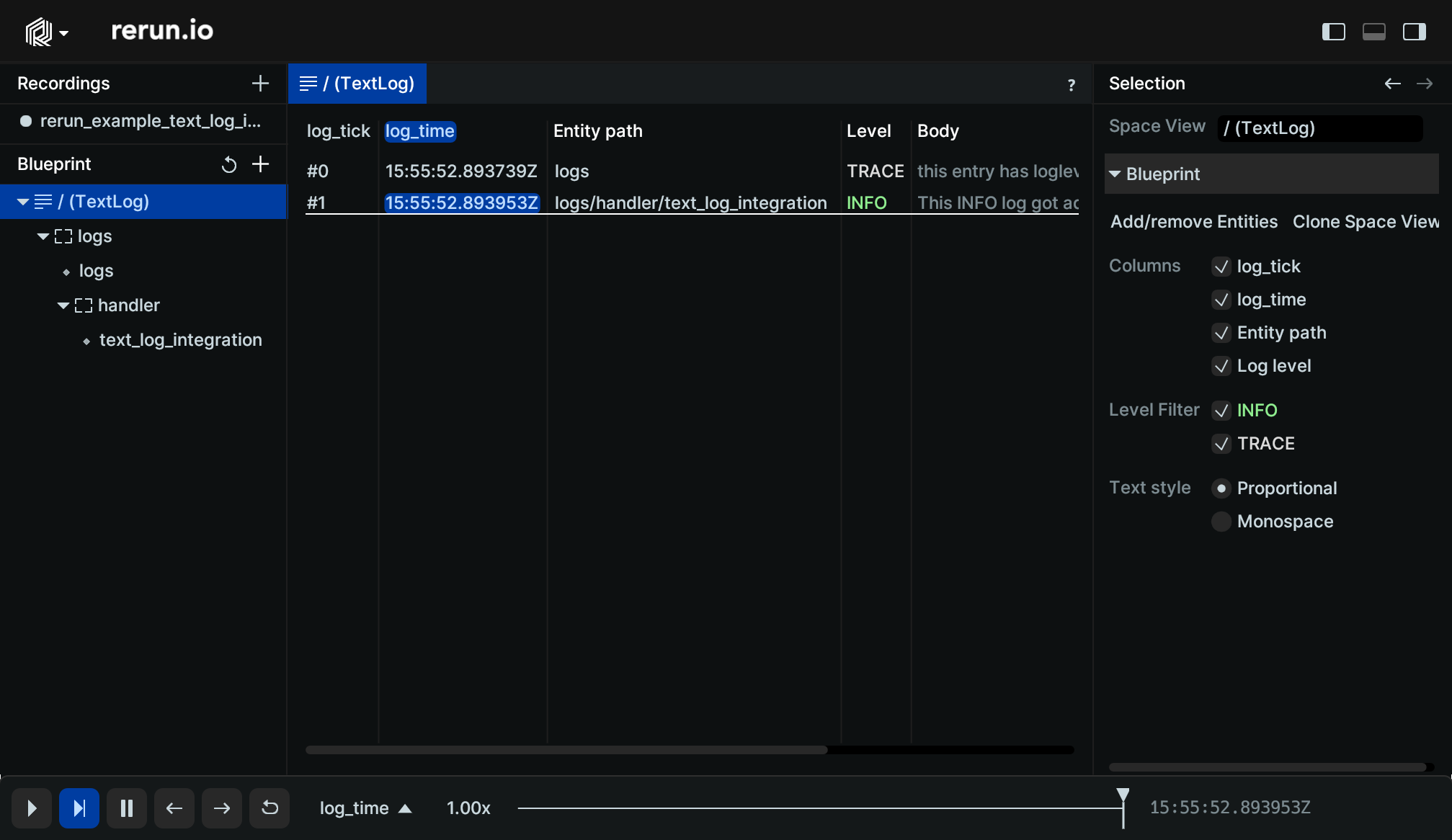
def __init__(text, *, level=None, color=None)
Create a new instance of the TextLog archetype.
PARAMETER | DESCRIPTION |
---|---|
text |
The body of the message.
TYPE:
|
level |
The verbosity level of the message. This can be used to filter the log messages in the Rerun Viewer.
TYPE:
|
color |
Optional color to use for the log line in the Rerun Viewer.
TYPE:
|
class Transform3D
Bases: Transform3DExt
, Archetype
Archetype: A 3D transform.
Example
Variety of 3D transforms:
from math import pi
import rerun as rr
from rerun.datatypes import Angle, RotationAxisAngle
rr.init("rerun_example_transform3d", spawn=True)
arrow = rr.Arrows3D(origins=[0, 0, 0], vectors=[0, 1, 0])
rr.log("base", arrow)
rr.log("base/translated", rr.Transform3D(translation=[1, 0, 0]))
rr.log("base/translated", arrow)
rr.log(
"base/rotated_scaled",
rr.Transform3D(
rotation=RotationAxisAngle(axis=[0, 0, 1], angle=Angle(rad=pi / 4)),
scale=2,
),
)
rr.log("base/rotated_scaled", arrow)
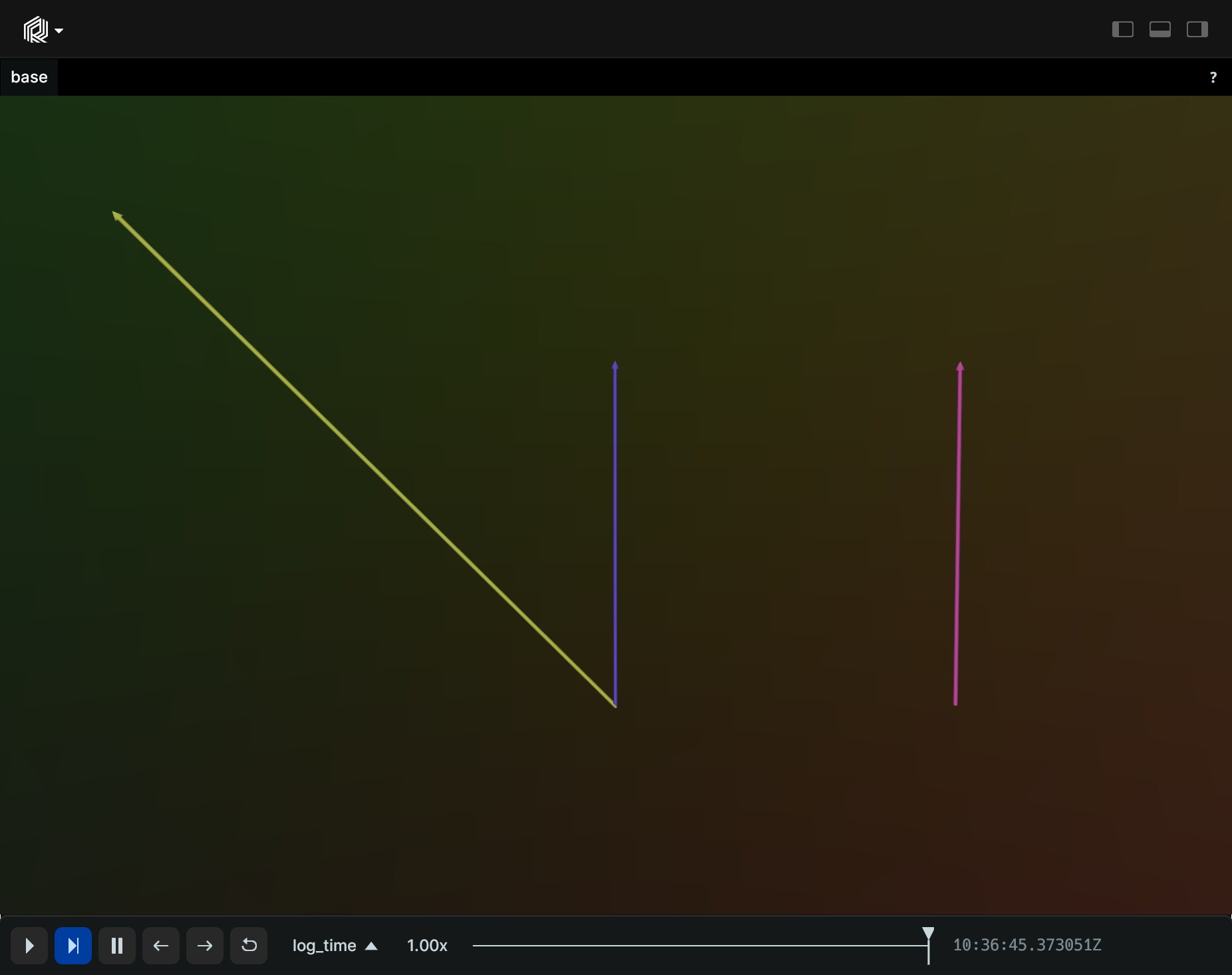
def __init__(transform=None, *, translation=None, rotation=None, scale=None, mat3x3=None, from_parent=None)
Create a new instance of the Transform3D archetype.
PARAMETER | DESCRIPTION |
---|---|
transform |
Transform using an existing Transform3D datatype object. If not provided, none of the other named parameters must be set.
TYPE:
|
translation |
3D translation vector, applied last.
Not compatible with
TYPE:
|
rotation |
3D rotation, applied second.
Not compatible with
TYPE:
|
scale |
3D scale, applied last.
Not compatible with
TYPE:
|
mat3x3 |
3x3 matrix representing scale and rotation, applied after translation.
Not compatible with
TYPE:
|
from_parent |
If true, the transform maps from the parent space to the space where the transform was logged. Otherwise, the transform maps from the space to its parent.
TYPE:
|
class ViewCoordinates
Bases: ViewCoordinatesExt
, Archetype
Archetype: How we interpret the coordinate system of an entity/space.
For instance: What is "up"? What does the Z axis mean? Is this right-handed or left-handed?
The three coordinates are always ordered as [x, y, z].
For example [Right, Down, Forward] means that the X axis points to the right, the Y axis points down, and the Z axis points forward.
Example
View coordinates for adjusting the eye camera:
import rerun as rr
rr.init("rerun_example_view_coordinates", spawn=True)
rr.log("world", rr.ViewCoordinates.RIGHT_HAND_Z_UP, timeless=True) # Set an up-axis
rr.log(
"world/xyz",
rr.Arrows3D(
vectors=[[1, 0, 0], [0, 1, 0], [0, 0, 1]],
colors=[[255, 0, 0], [0, 255, 0], [0, 0, 255]],
),
)
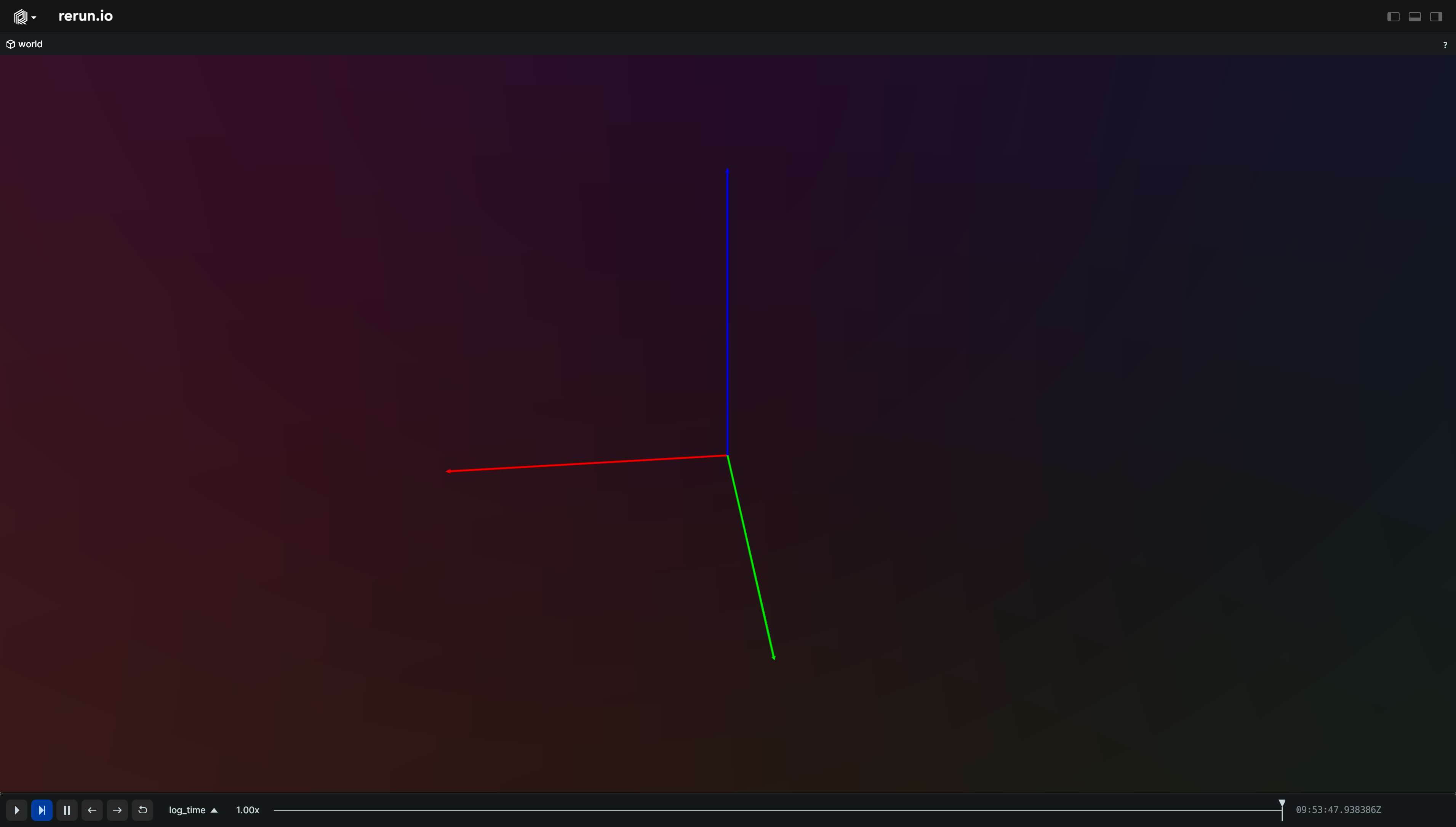
def __init__(xyz)
Create a new instance of the ViewCoordinates archetype.