Struct re_types::archetypes::AssetVideo
source · pub struct AssetVideo {
pub blob: Option<SerializedComponentBatch>,
pub media_type: Option<SerializedComponentBatch>,
}
Expand description
Archetype: A video binary.
Only MP4 containers with AV1 are generally supported, though the web viewer supports more video codecs, depending on browser.
See https://rerun.io/docs/reference/video for details of what is and isn’t supported.
In order to display a video, you also need to log a archetypes::VideoFrameReference
for each frame.
§Examples
§Video with automatically determined frames
use rerun::external::anyhow;
fn main() -> anyhow::Result<()> {
let args = _args;
let Some(path) = args.get(1) else {
// TODO(#7354): Only mp4 is supported for now.
anyhow::bail!("Usage: {} <path_to_video.[mp4]>", args[0]);
};
let rec =
rerun::RecordingStreamBuilder::new("rerun_example_asset_video_auto_frames").spawn()?;
// Log video asset which is referred to by frame references.
let video_asset = rerun::AssetVideo::from_file_path(path)?;
rec.log_static("video", &video_asset)?;
// Send automatically determined video frame timestamps.
let frame_timestamps_ns = video_asset.read_frame_timestamps_ns()?;
let video_timestamps_ns = frame_timestamps_ns
.iter()
.copied()
.map(rerun::components::VideoTimestamp::from_nanoseconds)
.collect::<Vec<_>>();
let time_column = rerun::TimeColumn::new_nanos(
"video_time",
// Note timeline values don't have to be the same as the video timestamps.
frame_timestamps_ns,
);
rec.send_columns(
"video",
[time_column],
rerun::VideoFrameReference::update_fields()
.with_many_timestamp(video_timestamps_ns)
.columns_of_unit_batches()?,
)?;
Ok(())
}
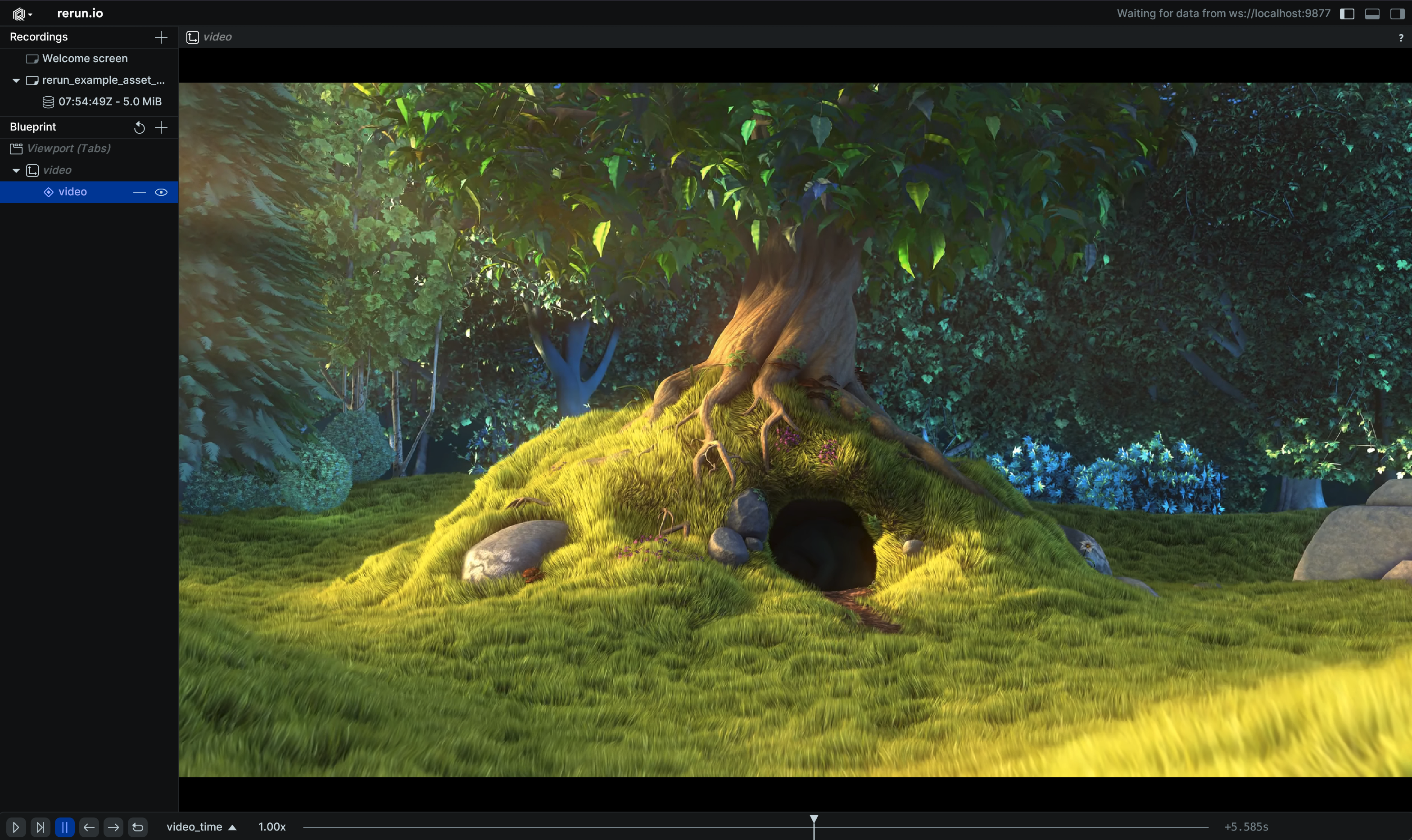
§Demonstrates manual use of video frame references
use rerun::external::anyhow;
fn main() -> anyhow::Result<()> {
let args = _args;
let Some(path) = args.get(1) else {
// TODO(#7354): Only mp4 is supported for now.
anyhow::bail!("Usage: {} <path_to_video.[mp4]>", args[0]);
};
let rec =
rerun::RecordingStreamBuilder::new("rerun_example_asset_video_manual_frames").spawn()?;
// Log video asset which is referred to by frame references.
rec.log_static("video_asset", &rerun::AssetVideo::from_file_path(path)?)?;
// Create two entities, showing the same video frozen at different times.
rec.log(
"frame_1s",
&rerun::VideoFrameReference::new(rerun::components::VideoTimestamp::from_seconds(1.0))
.with_video_reference("video_asset"),
)?;
rec.log(
"frame_2s",
&rerun::VideoFrameReference::new(rerun::components::VideoTimestamp::from_seconds(2.0))
.with_video_reference("video_asset"),
)?;
// TODO(#5520): log blueprint once supported
Ok(())
}
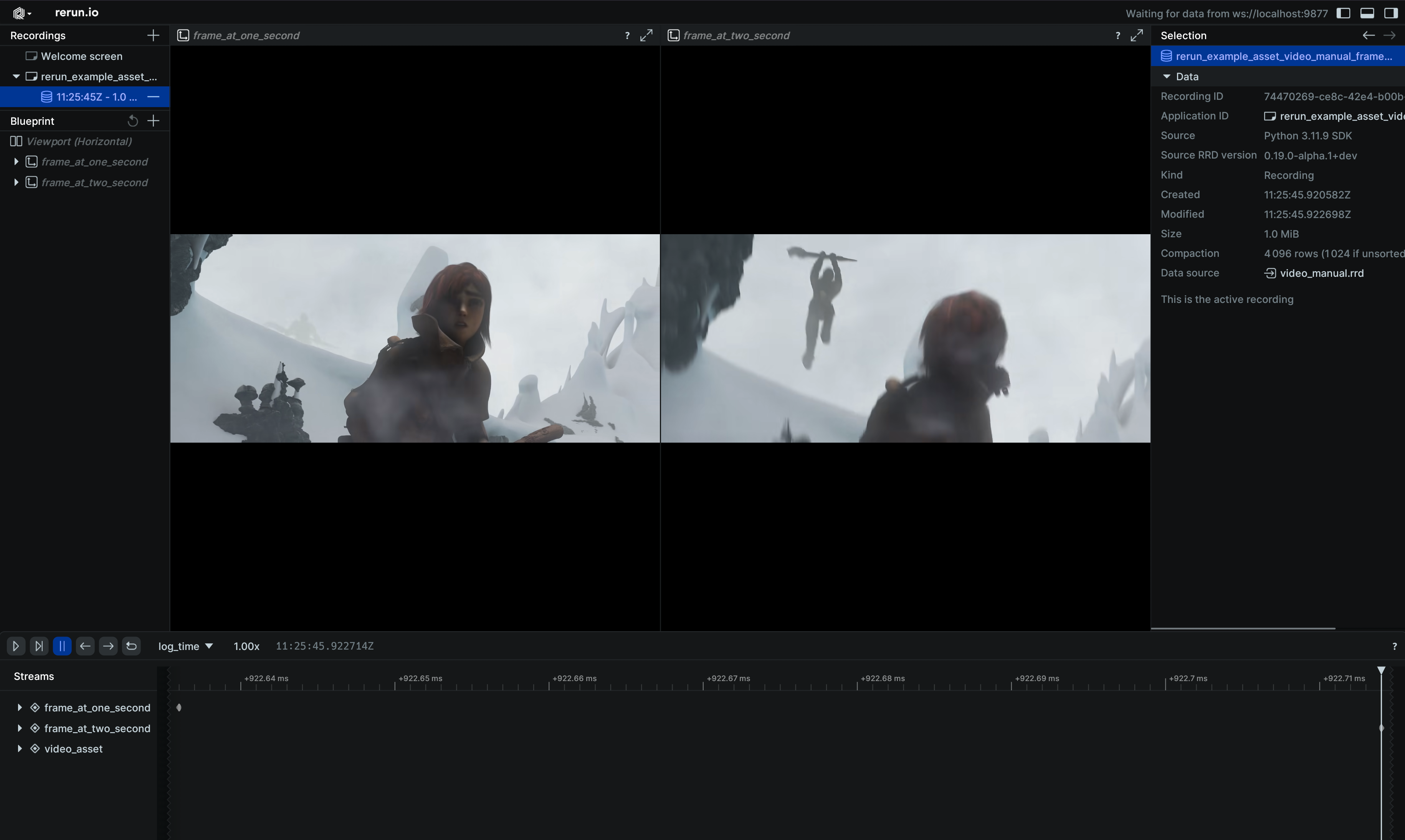
Fields§
§blob: Option<SerializedComponentBatch>
The asset’s bytes.
media_type: Option<SerializedComponentBatch>
The Media Type of the asset.
Supported values:
video/mp4
If omitted, the viewer will try to guess from the data blob. If it cannot guess, it won’t be able to render the asset.
Implementations§
source§impl AssetVideo
impl AssetVideo
sourcepub fn descriptor_blob() -> ComponentDescriptor
pub fn descriptor_blob() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::blob
.
sourcepub fn descriptor_media_type() -> ComponentDescriptor
pub fn descriptor_media_type() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::media_type
.
sourcepub fn descriptor_indicator() -> ComponentDescriptor
pub fn descriptor_indicator() -> ComponentDescriptor
Returns the ComponentDescriptor
for the associated indicator component.
source§impl AssetVideo
impl AssetVideo
sourcepub const NUM_COMPONENTS: usize = 3usize
pub const NUM_COMPONENTS: usize = 3usize
The total number of components in the archetype: 1 required, 2 recommended, 0 optional
source§impl AssetVideo
impl AssetVideo
sourcepub fn update_fields() -> Self
pub fn update_fields() -> Self
Update only some specific fields of a AssetVideo
.
sourcepub fn clear_fields() -> Self
pub fn clear_fields() -> Self
Clear all the fields of a AssetVideo
.
sourcepub fn columns<I>(
self,
_lengths: I,
) -> SerializationResult<impl Iterator<Item = SerializedComponentColumn>>
pub fn columns<I>( self, _lengths: I, ) -> SerializationResult<impl Iterator<Item = SerializedComponentColumn>>
Partitions the component data into multiple sub-batches.
Specifically, this transforms the existing SerializedComponentBatch
es data into SerializedComponentColumn
s
instead, via SerializedComponentBatch::partitioned
.
This makes it possible to use RecordingStream::send_columns
to send columnar data directly into Rerun.
The specified lengths
must sum to the total length of the component batch.
sourcepub fn columns_of_unit_batches(
self,
) -> SerializationResult<impl Iterator<Item = SerializedComponentColumn>>
pub fn columns_of_unit_batches( self, ) -> SerializationResult<impl Iterator<Item = SerializedComponentColumn>>
Helper to partition the component data into unit-length sub-batches.
This is semantically similar to calling Self::columns
with std::iter::take(1).repeat(n)
,
where n
is automatically guessed.
sourcepub fn with_many_blob(
self,
blob: impl IntoIterator<Item = impl Into<Blob>>,
) -> Self
pub fn with_many_blob( self, blob: impl IntoIterator<Item = impl Into<Blob>>, ) -> Self
This method makes it possible to pack multiple crate::components::Blob
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_blob
should
be used when logging a single row’s worth of data.
sourcepub fn with_media_type(self, media_type: impl Into<MediaType>) -> Self
pub fn with_media_type(self, media_type: impl Into<MediaType>) -> Self
The Media Type of the asset.
Supported values:
video/mp4
If omitted, the viewer will try to guess from the data blob. If it cannot guess, it won’t be able to render the asset.
sourcepub fn with_many_media_type(
self,
media_type: impl IntoIterator<Item = impl Into<MediaType>>,
) -> Self
pub fn with_many_media_type( self, media_type: impl IntoIterator<Item = impl Into<MediaType>>, ) -> Self
This method makes it possible to pack multiple crate::components::MediaType
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_media_type
should
be used when logging a single row’s worth of data.
source§impl AssetVideo
impl AssetVideo
sourcepub fn from_file_path(filepath: impl AsRef<Path>) -> Result<Self>
pub fn from_file_path(filepath: impl AsRef<Path>) -> Result<Self>
Creates a new AssetVideo
from the file contents at path
.
The MediaType
will first be guessed from the file extension, then from the file
contents if needed.
If no MediaType
can be guessed at the moment, the Rerun Viewer will try to guess one
from the data at render-time. If it can’t, rendering will fail with an error.
Returns an error if the file cannot be read.
sourcepub fn from_file_contents(
contents: Vec<u8>,
media_type: Option<impl Into<MediaType>>,
) -> Self
pub fn from_file_contents( contents: Vec<u8>, media_type: Option<impl Into<MediaType>>, ) -> Self
Creates a new AssetVideo
from the given contents
.
The MediaType
will be guessed from magic bytes in the data.
If no MediaType
can be guessed at the moment, the Rerun Viewer will try to guess one
from the data at render-time. If it can’t, rendering will fail with an error.
sourcepub fn read_frame_timestamps_ns(&self) -> Result<Vec<i64>, VideoLoadError>
pub fn read_frame_timestamps_ns(&self) -> Result<Vec<i64>, VideoLoadError>
Determines the presentation timestamps of all frames inside the video.
Returned timestamps are in nanoseconds since start and are guaranteed to be monotonically increasing.
Panics if the serialized blob data doesn’t have the right datatype.
Trait Implementations§
source§impl Archetype for AssetVideo
impl Archetype for AssetVideo
§type Indicator = GenericIndicatorComponent<AssetVideo>
type Indicator = GenericIndicatorComponent<AssetVideo>
source§fn name() -> ArchetypeName
fn name() -> ArchetypeName
rerun.archetypes.Points2D
.source§fn display_name() -> &'static str
fn display_name() -> &'static str
source§fn required_components() -> Cow<'static, [ComponentDescriptor]>
fn required_components() -> Cow<'static, [ComponentDescriptor]>
source§fn recommended_components() -> Cow<'static, [ComponentDescriptor]>
fn recommended_components() -> Cow<'static, [ComponentDescriptor]>
source§fn optional_components() -> Cow<'static, [ComponentDescriptor]>
fn optional_components() -> Cow<'static, [ComponentDescriptor]>
source§fn all_components() -> Cow<'static, [ComponentDescriptor]>
fn all_components() -> Cow<'static, [ComponentDescriptor]>
source§fn from_arrow_components(
arrow_data: impl IntoIterator<Item = (ComponentDescriptor, ArrayRef)>,
) -> DeserializationResult<Self>
fn from_arrow_components( arrow_data: impl IntoIterator<Item = (ComponentDescriptor, ArrayRef)>, ) -> DeserializationResult<Self>
ComponentNames
, deserializes them
into this archetype. Read moresource§fn from_arrow(
data: impl IntoIterator<Item = (Field, Arc<dyn Array>)>,
) -> Result<Self, DeserializationError>where
Self: Sized,
fn from_arrow(
data: impl IntoIterator<Item = (Field, Arc<dyn Array>)>,
) -> Result<Self, DeserializationError>where
Self: Sized,
source§impl AsComponents for AssetVideo
impl AsComponents for AssetVideo
source§fn as_serialized_batches(&self) -> Vec<SerializedComponentBatch>
fn as_serialized_batches(&self) -> Vec<SerializedComponentBatch>
SerializedComponentBatch
es. Read moresource§impl Clone for AssetVideo
impl Clone for AssetVideo
source§fn clone(&self) -> AssetVideo
fn clone(&self) -> AssetVideo
1.0.0 · source§fn clone_from(&mut self, source: &Self)
fn clone_from(&mut self, source: &Self)
source
. Read moresource§impl Debug for AssetVideo
impl Debug for AssetVideo
source§impl Default for AssetVideo
impl Default for AssetVideo
source§fn default() -> AssetVideo
fn default() -> AssetVideo
source§impl SizeBytes for AssetVideo
impl SizeBytes for AssetVideo
source§fn heap_size_bytes(&self) -> u64
fn heap_size_bytes(&self) -> u64
self
uses on the heap. Read moresource§fn total_size_bytes(&self) -> u64
fn total_size_bytes(&self) -> u64
self
in bytes, accounting for both stack and heap space.source§fn stack_size_bytes(&self) -> u64
fn stack_size_bytes(&self) -> u64
self
on the stack, in bytes. Read moreimpl ArchetypeReflectionMarker for AssetVideo
Auto Trait Implementations§
impl Freeze for AssetVideo
impl !RefUnwindSafe for AssetVideo
impl Send for AssetVideo
impl Sync for AssetVideo
impl Unpin for AssetVideo
impl !UnwindSafe for AssetVideo
Blanket Implementations§
source§impl<T> BorrowMut<T> for Twhere
T: ?Sized,
impl<T> BorrowMut<T> for Twhere
T: ?Sized,
source§fn borrow_mut(&mut self) -> &mut T
fn borrow_mut(&mut self) -> &mut T
source§impl<T> CheckedAs for T
impl<T> CheckedAs for T
source§fn checked_as<Dst>(self) -> Option<Dst>where
T: CheckedCast<Dst>,
fn checked_as<Dst>(self) -> Option<Dst>where
T: CheckedCast<Dst>,
source§impl<Src, Dst> CheckedCastFrom<Src> for Dstwhere
Src: CheckedCast<Dst>,
impl<Src, Dst> CheckedCastFrom<Src> for Dstwhere
Src: CheckedCast<Dst>,
source§fn checked_cast_from(src: Src) -> Option<Dst>
fn checked_cast_from(src: Src) -> Option<Dst>
source§impl<T> CloneToUninit for Twhere
T: Clone,
impl<T> CloneToUninit for Twhere
T: Clone,
source§default unsafe fn clone_to_uninit(&self, dst: *mut T)
default unsafe fn clone_to_uninit(&self, dst: *mut T)
clone_to_uninit
)§impl<T> Downcast for Twhere
T: Any,
impl<T> Downcast for Twhere
T: Any,
§fn into_any(self: Box<T>) -> Box<dyn Any>
fn into_any(self: Box<T>) -> Box<dyn Any>
Box<dyn Trait>
(where Trait: Downcast
) to Box<dyn Any>
. Box<dyn Any>
can
then be further downcast
into Box<ConcreteType>
where ConcreteType
implements Trait
.§fn into_any_rc(self: Rc<T>) -> Rc<dyn Any>
fn into_any_rc(self: Rc<T>) -> Rc<dyn Any>
Rc<Trait>
(where Trait: Downcast
) to Rc<Any>
. Rc<Any>
can then be
further downcast
into Rc<ConcreteType>
where ConcreteType
implements Trait
.§fn as_any(&self) -> &(dyn Any + 'static)
fn as_any(&self) -> &(dyn Any + 'static)
&Trait
(where Trait: Downcast
) to &Any
. This is needed since Rust cannot
generate &Any
’s vtable from &Trait
’s.§fn as_any_mut(&mut self) -> &mut (dyn Any + 'static)
fn as_any_mut(&mut self) -> &mut (dyn Any + 'static)
&mut Trait
(where Trait: Downcast
) to &Any
. This is needed since Rust cannot
generate &mut Any
’s vtable from &mut Trait
’s.§impl<T> DowncastSync for T
impl<T> DowncastSync for T
§impl<T> Instrument for T
impl<T> Instrument for T
§fn instrument(self, span: Span) -> Instrumented<Self>
fn instrument(self, span: Span) -> Instrumented<Self>
§fn in_current_span(self) -> Instrumented<Self>
fn in_current_span(self) -> Instrumented<Self>
source§impl<T> IntoEither for T
impl<T> IntoEither for T
source§fn into_either(self, into_left: bool) -> Either<Self, Self>
fn into_either(self, into_left: bool) -> Either<Self, Self>
self
into a Left
variant of Either<Self, Self>
if into_left
is true
.
Converts self
into a Right
variant of Either<Self, Self>
otherwise. Read moresource§fn into_either_with<F>(self, into_left: F) -> Either<Self, Self>
fn into_either_with<F>(self, into_left: F) -> Either<Self, Self>
self
into a Left
variant of Either<Self, Self>
if into_left(&self)
returns true
.
Converts self
into a Right
variant of Either<Self, Self>
otherwise. Read more