pub struct GeoPoints {
pub positions: Option<SerializedComponentBatch>,
pub radii: Option<SerializedComponentBatch>,
pub colors: Option<SerializedComponentBatch>,
pub class_ids: Option<SerializedComponentBatch>,
}
Expand description
Archetype: Geospatial points with positions expressed in EPSG:4326 latitude and longitude (North/East-positive degrees), and optional colors and radii.
§Example
§Log a geospatial point
fn main() -> Result<(), Box<dyn std::error::Error>> {
let rec = rerun::RecordingStreamBuilder::new("rerun_example_geo_points").spawn()?;
rec.log(
"rerun_hq",
&rerun::GeoPoints::from_lat_lon([(59.319221, 18.075631)])
.with_radii([rerun::Radius::new_ui_points(10.0)])
.with_colors([rerun::Color::from_rgb(255, 0, 0)]),
)?;
Ok(())
}
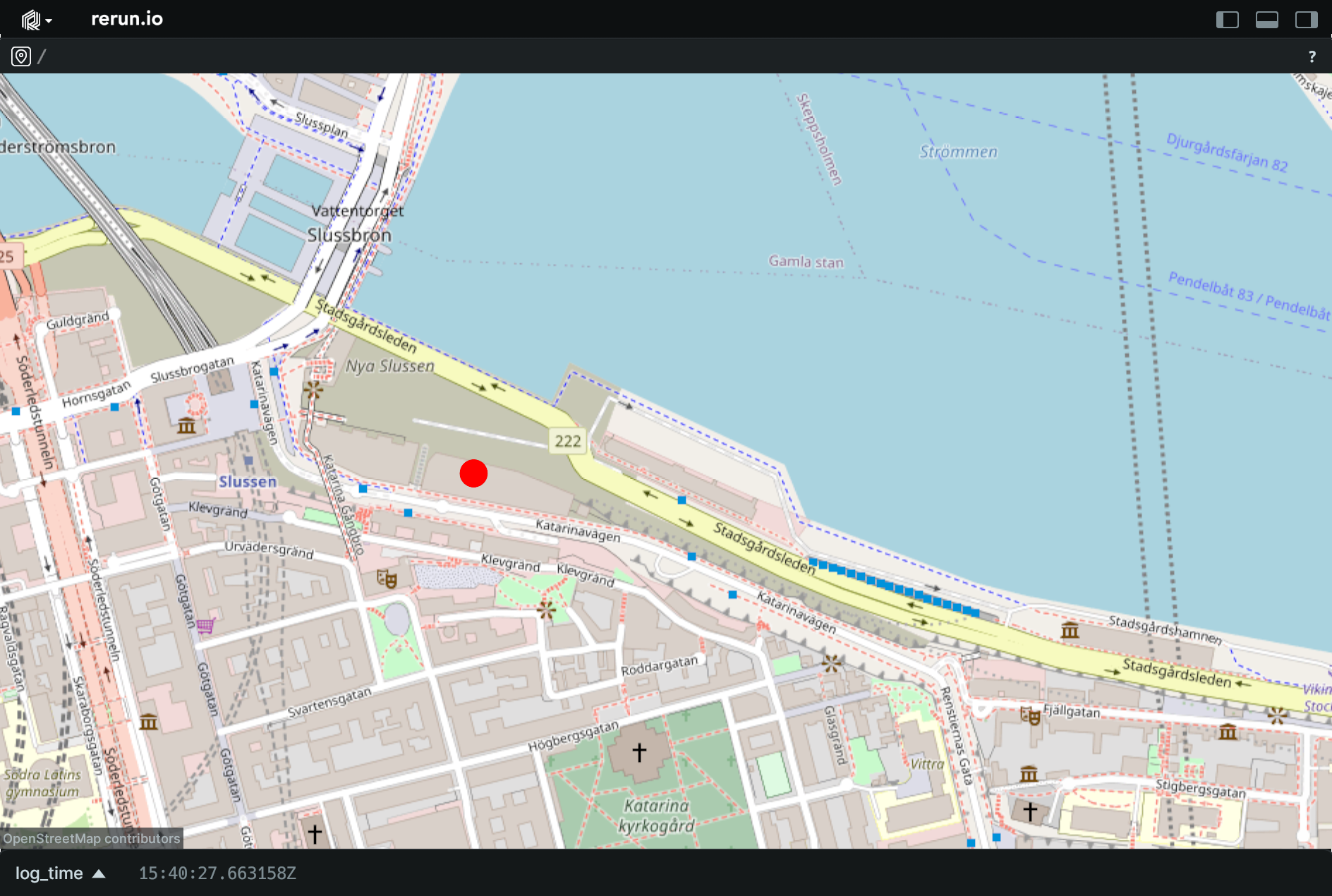
Fields§
§positions: Option<SerializedComponentBatch>
The EPSG:4326 coordinates for the points (North/East-positive degrees).
radii: Option<SerializedComponentBatch>
Optional radii for the points, effectively turning them into circles.
Note: scene units radiii are interpreted as meters.
colors: Option<SerializedComponentBatch>
Optional colors for the points.
class_ids: Option<SerializedComponentBatch>
Optional class Ids for the points.
The components::ClassId
provides colors if not specified explicitly.
Implementations§
Source§impl GeoPoints
impl GeoPoints
Sourcepub fn descriptor_positions() -> ComponentDescriptor
pub fn descriptor_positions() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::positions
.
Sourcepub fn descriptor_radii() -> ComponentDescriptor
pub fn descriptor_radii() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::radii
.
Sourcepub fn descriptor_colors() -> ComponentDescriptor
pub fn descriptor_colors() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::colors
.
Sourcepub fn descriptor_class_ids() -> ComponentDescriptor
pub fn descriptor_class_ids() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::class_ids
.
Sourcepub fn descriptor_indicator() -> ComponentDescriptor
pub fn descriptor_indicator() -> ComponentDescriptor
Returns the ComponentDescriptor
for the associated indicator component.
Source§impl GeoPoints
impl GeoPoints
Sourcepub const NUM_COMPONENTS: usize = 5usize
pub const NUM_COMPONENTS: usize = 5usize
The total number of components in the archetype: 1 required, 3 recommended, 1 optional
Source§impl GeoPoints
impl GeoPoints
Sourcepub fn update_fields() -> GeoPoints
pub fn update_fields() -> GeoPoints
Update only some specific fields of a GeoPoints
.
Sourcepub fn clear_fields() -> GeoPoints
pub fn clear_fields() -> GeoPoints
Clear all the fields of a GeoPoints
.
Sourcepub fn columns<I>(
self,
_lengths: I,
) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
pub fn columns<I>( self, _lengths: I, ) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
Partitions the component data into multiple sub-batches.
Specifically, this transforms the existing SerializedComponentBatch
es data into SerializedComponentColumn
s
instead, via SerializedComponentBatch::partitioned
.
This makes it possible to use RecordingStream::send_columns
to send columnar data directly into Rerun.
The specified lengths
must sum to the total length of the component batch.
Sourcepub fn columns_of_unit_batches(
self,
) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
pub fn columns_of_unit_batches( self, ) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
Helper to partition the component data into unit-length sub-batches.
This is semantically similar to calling Self::columns
with std::iter::take(1).repeat(n)
,
where n
is automatically guessed.
Sourcepub fn with_positions(
self,
positions: impl IntoIterator<Item = impl Into<LatLon>>,
) -> GeoPoints
pub fn with_positions( self, positions: impl IntoIterator<Item = impl Into<LatLon>>, ) -> GeoPoints
The EPSG:4326 coordinates for the points (North/East-positive degrees).
Sourcepub fn with_radii(
self,
radii: impl IntoIterator<Item = impl Into<Radius>>,
) -> GeoPoints
pub fn with_radii( self, radii: impl IntoIterator<Item = impl Into<Radius>>, ) -> GeoPoints
Optional radii for the points, effectively turning them into circles.
Note: scene units radiii are interpreted as meters.
Sourcepub fn with_colors(
self,
colors: impl IntoIterator<Item = impl Into<Color>>,
) -> GeoPoints
pub fn with_colors( self, colors: impl IntoIterator<Item = impl Into<Color>>, ) -> GeoPoints
Optional colors for the points.
Sourcepub fn with_class_ids(
self,
class_ids: impl IntoIterator<Item = impl Into<ClassId>>,
) -> GeoPoints
pub fn with_class_ids( self, class_ids: impl IntoIterator<Item = impl Into<ClassId>>, ) -> GeoPoints
Optional class Ids for the points.
The components::ClassId
provides colors if not specified explicitly.
Source§impl GeoPoints
impl GeoPoints
Sourcepub fn from_lat_lon(
positions: impl IntoIterator<Item = impl Into<LatLon>>,
) -> GeoPoints
pub fn from_lat_lon( positions: impl IntoIterator<Item = impl Into<LatLon>>, ) -> GeoPoints
Create a new GeoPoints
from EPSG:4326 latitude and longitude (North/East-positive degrees).
Trait Implementations§
Source§impl Archetype for GeoPoints
impl Archetype for GeoPoints
Source§type Indicator = GenericIndicatorComponent<GeoPoints>
type Indicator = GenericIndicatorComponent<GeoPoints>
Source§fn name() -> ArchetypeName
fn name() -> ArchetypeName
rerun.archetypes.Points2D
.Source§fn display_name() -> &'static str
fn display_name() -> &'static str
Source§fn required_components() -> Cow<'static, [ComponentDescriptor]>
fn required_components() -> Cow<'static, [ComponentDescriptor]>
Source§fn recommended_components() -> Cow<'static, [ComponentDescriptor]>
fn recommended_components() -> Cow<'static, [ComponentDescriptor]>
Source§fn optional_components() -> Cow<'static, [ComponentDescriptor]>
fn optional_components() -> Cow<'static, [ComponentDescriptor]>
Source§fn all_components() -> Cow<'static, [ComponentDescriptor]>
fn all_components() -> Cow<'static, [ComponentDescriptor]>
Source§fn from_arrow_components(
arrow_data: impl IntoIterator<Item = (ComponentDescriptor, Arc<dyn Array>)>,
) -> Result<GeoPoints, DeserializationError>
fn from_arrow_components( arrow_data: impl IntoIterator<Item = (ComponentDescriptor, Arc<dyn Array>)>, ) -> Result<GeoPoints, DeserializationError>
ComponentNames
, deserializes them
into this archetype. Read moreSource§fn from_arrow(
data: impl IntoIterator<Item = (Field, Arc<dyn Array>)>,
) -> Result<Self, DeserializationError>where
Self: Sized,
fn from_arrow(
data: impl IntoIterator<Item = (Field, Arc<dyn Array>)>,
) -> Result<Self, DeserializationError>where
Self: Sized,
Source§impl AsComponents for GeoPoints
impl AsComponents for GeoPoints
Source§fn as_serialized_batches(&self) -> Vec<SerializedComponentBatch>
fn as_serialized_batches(&self) -> Vec<SerializedComponentBatch>
SerializedComponentBatch
es. Read moreSource§impl SizeBytes for GeoPoints
impl SizeBytes for GeoPoints
Source§fn heap_size_bytes(&self) -> u64
fn heap_size_bytes(&self) -> u64
self
uses on the heap. Read moreSource§fn total_size_bytes(&self) -> u64
fn total_size_bytes(&self) -> u64
self
in bytes, accounting for both stack and heap space.Source§fn stack_size_bytes(&self) -> u64
fn stack_size_bytes(&self) -> u64
self
on the stack, in bytes. Read moreimpl ArchetypeReflectionMarker for GeoPoints
impl StructuralPartialEq for GeoPoints
Auto Trait Implementations§
impl Freeze for GeoPoints
impl !RefUnwindSafe for GeoPoints
impl Send for GeoPoints
impl Sync for GeoPoints
impl Unpin for GeoPoints
impl !UnwindSafe for GeoPoints
Blanket Implementations§
Source§impl<T> BorrowMut<T> for Twhere
T: ?Sized,
impl<T> BorrowMut<T> for Twhere
T: ?Sized,
Source§fn borrow_mut(&mut self) -> &mut T
fn borrow_mut(&mut self) -> &mut T
Source§impl<T> CheckedAs for T
impl<T> CheckedAs for T
Source§fn checked_as<Dst>(self) -> Option<Dst>where
T: CheckedCast<Dst>,
fn checked_as<Dst>(self) -> Option<Dst>where
T: CheckedCast<Dst>,
Source§impl<Src, Dst> CheckedCastFrom<Src> for Dstwhere
Src: CheckedCast<Dst>,
impl<Src, Dst> CheckedCastFrom<Src> for Dstwhere
Src: CheckedCast<Dst>,
Source§fn checked_cast_from(src: Src) -> Option<Dst>
fn checked_cast_from(src: Src) -> Option<Dst>
Source§impl<T> CloneToUninit for Twhere
T: Clone,
impl<T> CloneToUninit for Twhere
T: Clone,
§impl<T> Conv for T
impl<T> Conv for T
§impl<T> Downcast for Twhere
T: Any,
impl<T> Downcast for Twhere
T: Any,
§fn into_any(self: Box<T>) -> Box<dyn Any>
fn into_any(self: Box<T>) -> Box<dyn Any>
Box<dyn Trait>
(where Trait: Downcast
) to Box<dyn Any>
. Box<dyn Any>
can
then be further downcast
into Box<ConcreteType>
where ConcreteType
implements Trait
.§fn into_any_rc(self: Rc<T>) -> Rc<dyn Any>
fn into_any_rc(self: Rc<T>) -> Rc<dyn Any>
Rc<Trait>
(where Trait: Downcast
) to Rc<Any>
. Rc<Any>
can then be
further downcast
into Rc<ConcreteType>
where ConcreteType
implements Trait
.§fn as_any(&self) -> &(dyn Any + 'static)
fn as_any(&self) -> &(dyn Any + 'static)
&Trait
(where Trait: Downcast
) to &Any
. This is needed since Rust cannot
generate &Any
’s vtable from &Trait
’s.§fn as_any_mut(&mut self) -> &mut (dyn Any + 'static)
fn as_any_mut(&mut self) -> &mut (dyn Any + 'static)
&mut Trait
(where Trait: Downcast
) to &Any
. This is needed since Rust cannot
generate &mut Any
’s vtable from &mut Trait
’s.§impl<T> DowncastSync for T
impl<T> DowncastSync for T
§impl<T> Instrument for T
impl<T> Instrument for T
§fn instrument(self, span: Span) -> Instrumented<Self>
fn instrument(self, span: Span) -> Instrumented<Self>
§fn in_current_span(self) -> Instrumented<Self>
fn in_current_span(self) -> Instrumented<Self>
Source§impl<T> IntoEither for T
impl<T> IntoEither for T
Source§fn into_either(self, into_left: bool) -> Either<Self, Self>
fn into_either(self, into_left: bool) -> Either<Self, Self>
self
into a Left
variant of Either<Self, Self>
if into_left
is true
.
Converts self
into a Right
variant of Either<Self, Self>
otherwise. Read moreSource§fn into_either_with<F>(self, into_left: F) -> Either<Self, Self>
fn into_either_with<F>(self, into_left: F) -> Either<Self, Self>
self
into a Left
variant of Either<Self, Self>
if into_left(&self)
returns true
.
Converts self
into a Right
variant of Either<Self, Self>
otherwise. Read moreSource§impl<T> IntoRequest<T> for T
impl<T> IntoRequest<T> for T
Source§fn into_request(self) -> Request<T>
fn into_request(self) -> Request<T>
T
in a tonic::Request
Source§impl<Src, Dst> LosslessTryInto<Dst> for Srcwhere
Dst: LosslessTryFrom<Src>,
impl<Src, Dst> LosslessTryInto<Dst> for Srcwhere
Dst: LosslessTryFrom<Src>,
Source§fn lossless_try_into(self) -> Option<Dst>
fn lossless_try_into(self) -> Option<Dst>
Source§impl<Src, Dst> LossyInto<Dst> for Srcwhere
Dst: LossyFrom<Src>,
impl<Src, Dst> LossyInto<Dst> for Srcwhere
Dst: LossyFrom<Src>,
Source§fn lossy_into(self) -> Dst
fn lossy_into(self) -> Dst
§impl<T> NoneValue for Twhere
T: Default,
impl<T> NoneValue for Twhere
T: Default,
type NoneType = T
§fn null_value() -> T
fn null_value() -> T
Source§impl<T> OverflowingAs for T
impl<T> OverflowingAs for T
Source§fn overflowing_as<Dst>(self) -> (Dst, bool)where
T: OverflowingCast<Dst>,
fn overflowing_as<Dst>(self) -> (Dst, bool)where
T: OverflowingCast<Dst>,
Source§impl<Src, Dst> OverflowingCastFrom<Src> for Dstwhere
Src: OverflowingCast<Dst>,
impl<Src, Dst> OverflowingCastFrom<Src> for Dstwhere
Src: OverflowingCast<Dst>,
Source§fn overflowing_cast_from(src: Src) -> (Dst, bool)
fn overflowing_cast_from(src: Src) -> (Dst, bool)
§impl<T> Pipe for Twhere
T: ?Sized,
impl<T> Pipe for Twhere
T: ?Sized,
§fn pipe<R>(self, func: impl FnOnce(Self) -> R) -> Rwhere
Self: Sized,
fn pipe<R>(self, func: impl FnOnce(Self) -> R) -> Rwhere
Self: Sized,
§fn pipe_ref<'a, R>(&'a self, func: impl FnOnce(&'a Self) -> R) -> Rwhere
R: 'a,
fn pipe_ref<'a, R>(&'a self, func: impl FnOnce(&'a Self) -> R) -> Rwhere
R: 'a,
self
and passes that borrow into the pipe function. Read more§fn pipe_ref_mut<'a, R>(&'a mut self, func: impl FnOnce(&'a mut Self) -> R) -> Rwhere
R: 'a,
fn pipe_ref_mut<'a, R>(&'a mut self, func: impl FnOnce(&'a mut Self) -> R) -> Rwhere
R: 'a,
self
and passes that borrow into the pipe function. Read more§fn pipe_borrow<'a, B, R>(&'a self, func: impl FnOnce(&'a B) -> R) -> R
fn pipe_borrow<'a, B, R>(&'a self, func: impl FnOnce(&'a B) -> R) -> R
§fn pipe_borrow_mut<'a, B, R>(
&'a mut self,
func: impl FnOnce(&'a mut B) -> R,
) -> R
fn pipe_borrow_mut<'a, B, R>( &'a mut self, func: impl FnOnce(&'a mut B) -> R, ) -> R
§fn pipe_as_ref<'a, U, R>(&'a self, func: impl FnOnce(&'a U) -> R) -> R
fn pipe_as_ref<'a, U, R>(&'a self, func: impl FnOnce(&'a U) -> R) -> R
self
, then passes self.as_ref()
into the pipe function.§fn pipe_as_mut<'a, U, R>(&'a mut self, func: impl FnOnce(&'a mut U) -> R) -> R
fn pipe_as_mut<'a, U, R>(&'a mut self, func: impl FnOnce(&'a mut U) -> R) -> R
self
, then passes self.as_mut()
into the pipe
function.§fn pipe_deref<'a, T, R>(&'a self, func: impl FnOnce(&'a T) -> R) -> R
fn pipe_deref<'a, T, R>(&'a self, func: impl FnOnce(&'a T) -> R) -> R
self
, then passes self.deref()
into the pipe function.§impl<T> Pointable for T
impl<T> Pointable for T
Source§impl<T> SaturatingAs for T
impl<T> SaturatingAs for T
Source§fn saturating_as<Dst>(self) -> Dstwhere
T: SaturatingCast<Dst>,
fn saturating_as<Dst>(self) -> Dstwhere
T: SaturatingCast<Dst>,
Source§impl<Src, Dst> SaturatingCastFrom<Src> for Dstwhere
Src: SaturatingCast<Dst>,
impl<Src, Dst> SaturatingCastFrom<Src> for Dstwhere
Src: SaturatingCast<Dst>,
Source§fn saturating_cast_from(src: Src) -> Dst
fn saturating_cast_from(src: Src) -> Dst
§impl<T> Tap for T
impl<T> Tap for T
§fn tap_borrow<B>(self, func: impl FnOnce(&B)) -> Self
fn tap_borrow<B>(self, func: impl FnOnce(&B)) -> Self
Borrow<B>
of a value. Read more§fn tap_borrow_mut<B>(self, func: impl FnOnce(&mut B)) -> Self
fn tap_borrow_mut<B>(self, func: impl FnOnce(&mut B)) -> Self
BorrowMut<B>
of a value. Read more§fn tap_ref<R>(self, func: impl FnOnce(&R)) -> Self
fn tap_ref<R>(self, func: impl FnOnce(&R)) -> Self
AsRef<R>
view of a value. Read more§fn tap_ref_mut<R>(self, func: impl FnOnce(&mut R)) -> Self
fn tap_ref_mut<R>(self, func: impl FnOnce(&mut R)) -> Self
AsMut<R>
view of a value. Read more§fn tap_deref<T>(self, func: impl FnOnce(&T)) -> Self
fn tap_deref<T>(self, func: impl FnOnce(&T)) -> Self
Deref::Target
of a value. Read more§fn tap_deref_mut<T>(self, func: impl FnOnce(&mut T)) -> Self
fn tap_deref_mut<T>(self, func: impl FnOnce(&mut T)) -> Self
Deref::Target
of a value. Read more§fn tap_dbg(self, func: impl FnOnce(&Self)) -> Self
fn tap_dbg(self, func: impl FnOnce(&Self)) -> Self
.tap()
only in debug builds, and is erased in release builds.§fn tap_mut_dbg(self, func: impl FnOnce(&mut Self)) -> Self
fn tap_mut_dbg(self, func: impl FnOnce(&mut Self)) -> Self
.tap_mut()
only in debug builds, and is erased in release
builds.§fn tap_borrow_dbg<B>(self, func: impl FnOnce(&B)) -> Self
fn tap_borrow_dbg<B>(self, func: impl FnOnce(&B)) -> Self
.tap_borrow()
only in debug builds, and is erased in release
builds.§fn tap_borrow_mut_dbg<B>(self, func: impl FnOnce(&mut B)) -> Self
fn tap_borrow_mut_dbg<B>(self, func: impl FnOnce(&mut B)) -> Self
.tap_borrow_mut()
only in debug builds, and is erased in release
builds.§fn tap_ref_dbg<R>(self, func: impl FnOnce(&R)) -> Self
fn tap_ref_dbg<R>(self, func: impl FnOnce(&R)) -> Self
.tap_ref()
only in debug builds, and is erased in release
builds.§fn tap_ref_mut_dbg<R>(self, func: impl FnOnce(&mut R)) -> Self
fn tap_ref_mut_dbg<R>(self, func: impl FnOnce(&mut R)) -> Self
.tap_ref_mut()
only in debug builds, and is erased in release
builds.§fn tap_deref_dbg<T>(self, func: impl FnOnce(&T)) -> Self
fn tap_deref_dbg<T>(self, func: impl FnOnce(&T)) -> Self
.tap_deref()
only in debug builds, and is erased in release
builds.