pub struct Tensor {
pub data: Option<SerializedComponentBatch>,
pub value_range: Option<SerializedComponentBatch>,
}
Expand description
Archetype: An N-dimensional array of numbers.
§Example
§Simple tensor
use ndarray::{Array, ShapeBuilder as _};
use rand::prelude::*;
fn main() -> Result<(), Box<dyn std::error::Error>> {
let rec = rerun::RecordingStreamBuilder::new("rerun_example_tensor").spawn()?;
let mut data = Array::<u8, _>::default((8, 6, 3, 5).f());
let mut rng = rand::rngs::SmallRng::seed_from_u64(42);
data.map_inplace(|x| *x = rng.gen());
let tensor =
rerun::Tensor::try_from(data)?.with_dim_names(["width", "height", "channel", "batch"]);
rec.log("tensor", &tensor)?;
Ok(())
}
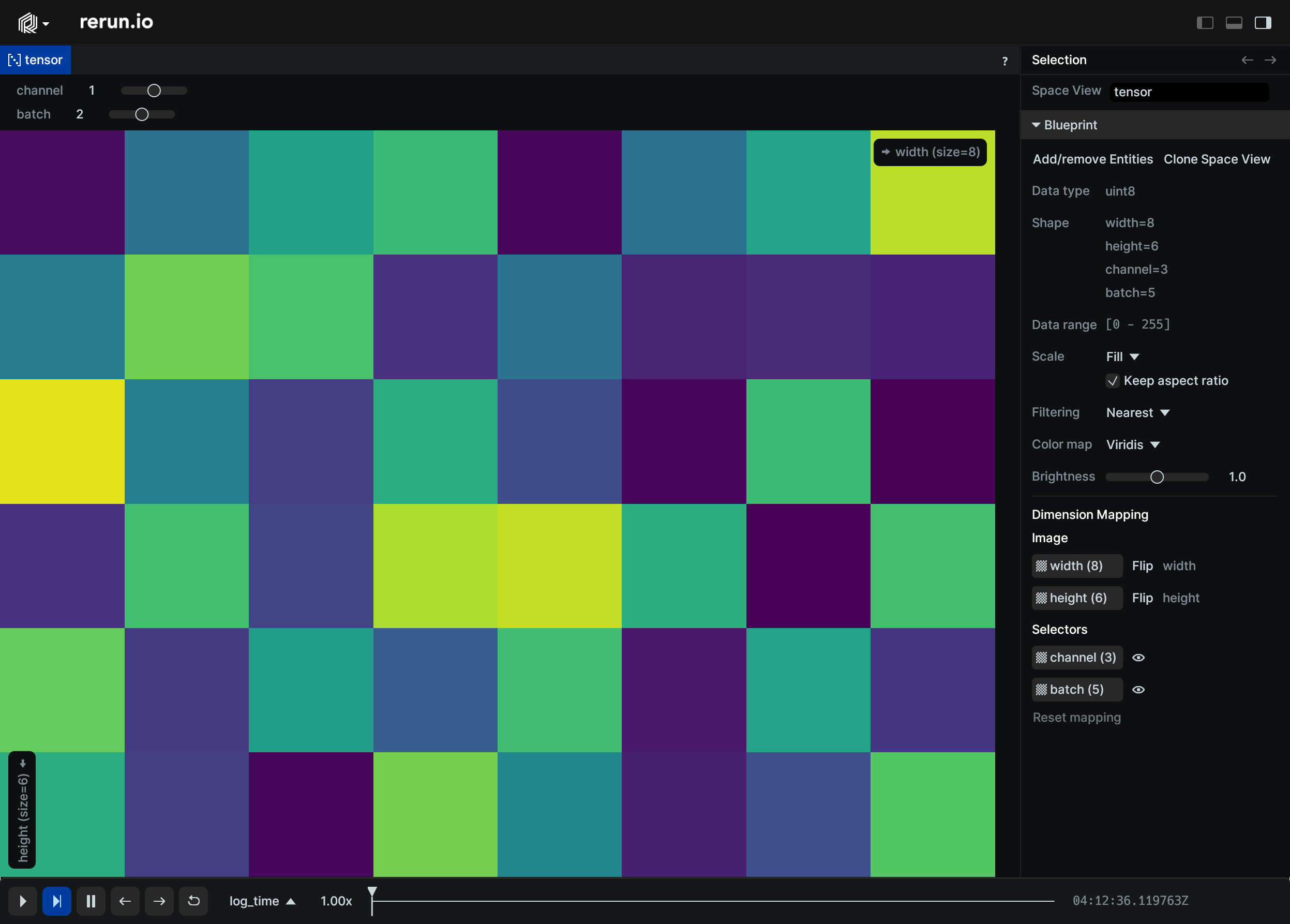
Fields§
§data: Option<SerializedComponentBatch>
The tensor data
value_range: Option<SerializedComponentBatch>
The expected range of values.
This is typically the expected range of valid values. Everything outside of the range is clamped to the range for the purpose of colormpaping. Any colormap applied for display, will map this range.
If not specified, the range will be automatically estimated from the data. Note that the Viewer may try to guess a wider range than the minimum/maximum of values in the contents of the tensor. E.g. if all values are positive, some bigger than 1.0 and all smaller than 255.0, the Viewer will guess that the data likely came from an 8bit image, thus assuming a range of 0-255.
Implementations§
Source§impl Tensor
impl Tensor
Sourcepub fn descriptor_data() -> ComponentDescriptor
pub fn descriptor_data() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::data
.
Sourcepub fn descriptor_value_range() -> ComponentDescriptor
pub fn descriptor_value_range() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::value_range
.
Sourcepub fn descriptor_indicator() -> ComponentDescriptor
pub fn descriptor_indicator() -> ComponentDescriptor
Returns the ComponentDescriptor
for the associated indicator component.
Source§impl Tensor
impl Tensor
Sourcepub const NUM_COMPONENTS: usize = 3usize
pub const NUM_COMPONENTS: usize = 3usize
The total number of components in the archetype: 1 required, 1 recommended, 1 optional
Source§impl Tensor
impl Tensor
Sourcepub fn new(data: impl Into<TensorData>) -> Tensor
pub fn new(data: impl Into<TensorData>) -> Tensor
Create a new Tensor
.
Sourcepub fn update_fields() -> Tensor
pub fn update_fields() -> Tensor
Update only some specific fields of a Tensor
.
Sourcepub fn clear_fields() -> Tensor
pub fn clear_fields() -> Tensor
Clear all the fields of a Tensor
.
Sourcepub fn columns<I>(
self,
_lengths: I,
) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
pub fn columns<I>( self, _lengths: I, ) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
Partitions the component data into multiple sub-batches.
Specifically, this transforms the existing SerializedComponentBatch
es data into SerializedComponentColumn
s
instead, via SerializedComponentBatch::partitioned
.
This makes it possible to use RecordingStream::send_columns
to send columnar data directly into Rerun.
The specified lengths
must sum to the total length of the component batch.
Sourcepub fn columns_of_unit_batches(
self,
) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
pub fn columns_of_unit_batches( self, ) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
Helper to partition the component data into unit-length sub-batches.
This is semantically similar to calling Self::columns
with std::iter::take(1).repeat(n)
,
where n
is automatically guessed.
Sourcepub fn with_data(self, data: impl Into<TensorData>) -> Tensor
pub fn with_data(self, data: impl Into<TensorData>) -> Tensor
The tensor data
Sourcepub fn with_many_data(
self,
data: impl IntoIterator<Item = impl Into<TensorData>>,
) -> Tensor
pub fn with_many_data( self, data: impl IntoIterator<Item = impl Into<TensorData>>, ) -> Tensor
This method makes it possible to pack multiple crate::components::TensorData
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_data
should
be used when logging a single row’s worth of data.
Sourcepub fn with_value_range(self, value_range: impl Into<ValueRange>) -> Tensor
pub fn with_value_range(self, value_range: impl Into<ValueRange>) -> Tensor
The expected range of values.
This is typically the expected range of valid values. Everything outside of the range is clamped to the range for the purpose of colormpaping. Any colormap applied for display, will map this range.
If not specified, the range will be automatically estimated from the data. Note that the Viewer may try to guess a wider range than the minimum/maximum of values in the contents of the tensor. E.g. if all values are positive, some bigger than 1.0 and all smaller than 255.0, the Viewer will guess that the data likely came from an 8bit image, thus assuming a range of 0-255.
Sourcepub fn with_many_value_range(
self,
value_range: impl IntoIterator<Item = impl Into<ValueRange>>,
) -> Tensor
pub fn with_many_value_range( self, value_range: impl IntoIterator<Item = impl Into<ValueRange>>, ) -> Tensor
This method makes it possible to pack multiple crate::components::ValueRange
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_value_range
should
be used when logging a single row’s worth of data.
Source§impl Tensor
impl Tensor
Sourcepub fn try_from<T>(data: T) -> Result<Tensor, <T as TryInto<TensorData>>::Error>where
T: TryInto<TensorData>,
pub fn try_from<T>(data: T) -> Result<Tensor, <T as TryInto<TensorData>>::Error>where
T: TryInto<TensorData>,
Try to construct a Tensor
from anything that can be converted into TensorData
This is useful for constructing a tensor from an ndarray.
Sourcepub fn with_dim_names(
self,
names: impl IntoIterator<Item = impl Into<ArrowString>>,
) -> Tensor
pub fn with_dim_names( self, names: impl IntoIterator<Item = impl Into<ArrowString>>, ) -> Tensor
Update the names
of the contained TensorData
dimensions.
Any existing names will be overwritten.
If the wrong number of names are given, a warning will be logged, and the names might not show up correctly.
Source§impl Tensor
impl Tensor
Sourcepub fn from_image(
image: impl Into<DynamicImage>,
) -> Result<Tensor, TensorImageLoadError>
pub fn from_image( image: impl Into<DynamicImage>, ) -> Result<Tensor, TensorImageLoadError>
Construct a tensor from something that can be turned into a image::DynamicImage
.
Requires the image
feature.
Sourcepub fn from_dynamic_image(
image: DynamicImage,
) -> Result<Tensor, TensorImageLoadError>
pub fn from_dynamic_image( image: DynamicImage, ) -> Result<Tensor, TensorImageLoadError>
Construct a tensor from image::DynamicImage
.
Requires the image
feature.
Trait Implementations§
Source§impl Archetype for Tensor
impl Archetype for Tensor
Source§type Indicator = GenericIndicatorComponent<Tensor>
type Indicator = GenericIndicatorComponent<Tensor>
Source§fn name() -> ArchetypeName
fn name() -> ArchetypeName
rerun.archetypes.Points2D
.Source§fn display_name() -> &'static str
fn display_name() -> &'static str
Source§fn required_components() -> Cow<'static, [ComponentDescriptor]>
fn required_components() -> Cow<'static, [ComponentDescriptor]>
Source§fn recommended_components() -> Cow<'static, [ComponentDescriptor]>
fn recommended_components() -> Cow<'static, [ComponentDescriptor]>
Source§fn optional_components() -> Cow<'static, [ComponentDescriptor]>
fn optional_components() -> Cow<'static, [ComponentDescriptor]>
Source§fn all_components() -> Cow<'static, [ComponentDescriptor]>
fn all_components() -> Cow<'static, [ComponentDescriptor]>
Source§fn from_arrow_components(
arrow_data: impl IntoIterator<Item = (ComponentDescriptor, Arc<dyn Array>)>,
) -> Result<Tensor, DeserializationError>
fn from_arrow_components( arrow_data: impl IntoIterator<Item = (ComponentDescriptor, Arc<dyn Array>)>, ) -> Result<Tensor, DeserializationError>
ComponentNames
, deserializes them
into this archetype. Read moreSource§fn from_arrow(
data: impl IntoIterator<Item = (Field, Arc<dyn Array>)>,
) -> Result<Self, DeserializationError>where
Self: Sized,
fn from_arrow(
data: impl IntoIterator<Item = (Field, Arc<dyn Array>)>,
) -> Result<Self, DeserializationError>where
Self: Sized,
Source§impl AsComponents for Tensor
impl AsComponents for Tensor
Source§fn as_serialized_batches(&self) -> Vec<SerializedComponentBatch>
fn as_serialized_batches(&self) -> Vec<SerializedComponentBatch>
SerializedComponentBatch
es. Read moreSource§impl SizeBytes for Tensor
impl SizeBytes for Tensor
Source§fn heap_size_bytes(&self) -> u64
fn heap_size_bytes(&self) -> u64
self
uses on the heap. Read moreSource§fn total_size_bytes(&self) -> u64
fn total_size_bytes(&self) -> u64
self
in bytes, accounting for both stack and heap space.Source§fn stack_size_bytes(&self) -> u64
fn stack_size_bytes(&self) -> u64
self
on the stack, in bytes. Read moreimpl ArchetypeReflectionMarker for Tensor
impl StructuralPartialEq for Tensor
Auto Trait Implementations§
impl Freeze for Tensor
impl !RefUnwindSafe for Tensor
impl Send for Tensor
impl Sync for Tensor
impl Unpin for Tensor
impl !UnwindSafe for Tensor
Blanket Implementations§
Source§impl<T> BorrowMut<T> for Twhere
T: ?Sized,
impl<T> BorrowMut<T> for Twhere
T: ?Sized,
Source§fn borrow_mut(&mut self) -> &mut T
fn borrow_mut(&mut self) -> &mut T
Source§impl<T> CheckedAs for T
impl<T> CheckedAs for T
Source§fn checked_as<Dst>(self) -> Option<Dst>where
T: CheckedCast<Dst>,
fn checked_as<Dst>(self) -> Option<Dst>where
T: CheckedCast<Dst>,
Source§impl<Src, Dst> CheckedCastFrom<Src> for Dstwhere
Src: CheckedCast<Dst>,
impl<Src, Dst> CheckedCastFrom<Src> for Dstwhere
Src: CheckedCast<Dst>,
Source§fn checked_cast_from(src: Src) -> Option<Dst>
fn checked_cast_from(src: Src) -> Option<Dst>
Source§impl<T> CloneToUninit for Twhere
T: Clone,
impl<T> CloneToUninit for Twhere
T: Clone,
§impl<T> Conv for T
impl<T> Conv for T
§impl<T> Downcast for Twhere
T: Any,
impl<T> Downcast for Twhere
T: Any,
§fn into_any(self: Box<T>) -> Box<dyn Any>
fn into_any(self: Box<T>) -> Box<dyn Any>
Box<dyn Trait>
(where Trait: Downcast
) to Box<dyn Any>
. Box<dyn Any>
can
then be further downcast
into Box<ConcreteType>
where ConcreteType
implements Trait
.§fn into_any_rc(self: Rc<T>) -> Rc<dyn Any>
fn into_any_rc(self: Rc<T>) -> Rc<dyn Any>
Rc<Trait>
(where Trait: Downcast
) to Rc<Any>
. Rc<Any>
can then be
further downcast
into Rc<ConcreteType>
where ConcreteType
implements Trait
.§fn as_any(&self) -> &(dyn Any + 'static)
fn as_any(&self) -> &(dyn Any + 'static)
&Trait
(where Trait: Downcast
) to &Any
. This is needed since Rust cannot
generate &Any
’s vtable from &Trait
’s.§fn as_any_mut(&mut self) -> &mut (dyn Any + 'static)
fn as_any_mut(&mut self) -> &mut (dyn Any + 'static)
&mut Trait
(where Trait: Downcast
) to &Any
. This is needed since Rust cannot
generate &mut Any
’s vtable from &mut Trait
’s.§impl<T> DowncastSync for T
impl<T> DowncastSync for T
§impl<T> Instrument for T
impl<T> Instrument for T
§fn instrument(self, span: Span) -> Instrumented<Self>
fn instrument(self, span: Span) -> Instrumented<Self>
§fn in_current_span(self) -> Instrumented<Self>
fn in_current_span(self) -> Instrumented<Self>
Source§impl<T> IntoEither for T
impl<T> IntoEither for T
Source§fn into_either(self, into_left: bool) -> Either<Self, Self>
fn into_either(self, into_left: bool) -> Either<Self, Self>
self
into a Left
variant of Either<Self, Self>
if into_left
is true
.
Converts self
into a Right
variant of Either<Self, Self>
otherwise. Read moreSource§fn into_either_with<F>(self, into_left: F) -> Either<Self, Self>
fn into_either_with<F>(self, into_left: F) -> Either<Self, Self>
self
into a Left
variant of Either<Self, Self>
if into_left(&self)
returns true
.
Converts self
into a Right
variant of Either<Self, Self>
otherwise. Read moreSource§impl<T> IntoRequest<T> for T
impl<T> IntoRequest<T> for T
Source§fn into_request(self) -> Request<T>
fn into_request(self) -> Request<T>
T
in a tonic::Request
Source§impl<Src, Dst> LosslessTryInto<Dst> for Srcwhere
Dst: LosslessTryFrom<Src>,
impl<Src, Dst> LosslessTryInto<Dst> for Srcwhere
Dst: LosslessTryFrom<Src>,
Source§fn lossless_try_into(self) -> Option<Dst>
fn lossless_try_into(self) -> Option<Dst>
Source§impl<Src, Dst> LossyInto<Dst> for Srcwhere
Dst: LossyFrom<Src>,
impl<Src, Dst> LossyInto<Dst> for Srcwhere
Dst: LossyFrom<Src>,
Source§fn lossy_into(self) -> Dst
fn lossy_into(self) -> Dst
§impl<T> NoneValue for Twhere
T: Default,
impl<T> NoneValue for Twhere
T: Default,
type NoneType = T
§fn null_value() -> T
fn null_value() -> T
Source§impl<T> OverflowingAs for T
impl<T> OverflowingAs for T
Source§fn overflowing_as<Dst>(self) -> (Dst, bool)where
T: OverflowingCast<Dst>,
fn overflowing_as<Dst>(self) -> (Dst, bool)where
T: OverflowingCast<Dst>,
Source§impl<Src, Dst> OverflowingCastFrom<Src> for Dstwhere
Src: OverflowingCast<Dst>,
impl<Src, Dst> OverflowingCastFrom<Src> for Dstwhere
Src: OverflowingCast<Dst>,
Source§fn overflowing_cast_from(src: Src) -> (Dst, bool)
fn overflowing_cast_from(src: Src) -> (Dst, bool)
§impl<T> Pipe for Twhere
T: ?Sized,
impl<T> Pipe for Twhere
T: ?Sized,
§fn pipe<R>(self, func: impl FnOnce(Self) -> R) -> Rwhere
Self: Sized,
fn pipe<R>(self, func: impl FnOnce(Self) -> R) -> Rwhere
Self: Sized,
§fn pipe_ref<'a, R>(&'a self, func: impl FnOnce(&'a Self) -> R) -> Rwhere
R: 'a,
fn pipe_ref<'a, R>(&'a self, func: impl FnOnce(&'a Self) -> R) -> Rwhere
R: 'a,
self
and passes that borrow into the pipe function. Read more§fn pipe_ref_mut<'a, R>(&'a mut self, func: impl FnOnce(&'a mut Self) -> R) -> Rwhere
R: 'a,
fn pipe_ref_mut<'a, R>(&'a mut self, func: impl FnOnce(&'a mut Self) -> R) -> Rwhere
R: 'a,
self
and passes that borrow into the pipe function. Read more§fn pipe_borrow<'a, B, R>(&'a self, func: impl FnOnce(&'a B) -> R) -> R
fn pipe_borrow<'a, B, R>(&'a self, func: impl FnOnce(&'a B) -> R) -> R
§fn pipe_borrow_mut<'a, B, R>(
&'a mut self,
func: impl FnOnce(&'a mut B) -> R,
) -> R
fn pipe_borrow_mut<'a, B, R>( &'a mut self, func: impl FnOnce(&'a mut B) -> R, ) -> R
§fn pipe_as_ref<'a, U, R>(&'a self, func: impl FnOnce(&'a U) -> R) -> R
fn pipe_as_ref<'a, U, R>(&'a self, func: impl FnOnce(&'a U) -> R) -> R
self
, then passes self.as_ref()
into the pipe function.§fn pipe_as_mut<'a, U, R>(&'a mut self, func: impl FnOnce(&'a mut U) -> R) -> R
fn pipe_as_mut<'a, U, R>(&'a mut self, func: impl FnOnce(&'a mut U) -> R) -> R
self
, then passes self.as_mut()
into the pipe
function.§fn pipe_deref<'a, T, R>(&'a self, func: impl FnOnce(&'a T) -> R) -> R
fn pipe_deref<'a, T, R>(&'a self, func: impl FnOnce(&'a T) -> R) -> R
self
, then passes self.deref()
into the pipe function.§impl<T> Pointable for T
impl<T> Pointable for T
Source§impl<T> SaturatingAs for T
impl<T> SaturatingAs for T
Source§fn saturating_as<Dst>(self) -> Dstwhere
T: SaturatingCast<Dst>,
fn saturating_as<Dst>(self) -> Dstwhere
T: SaturatingCast<Dst>,
Source§impl<Src, Dst> SaturatingCastFrom<Src> for Dstwhere
Src: SaturatingCast<Dst>,
impl<Src, Dst> SaturatingCastFrom<Src> for Dstwhere
Src: SaturatingCast<Dst>,
Source§fn saturating_cast_from(src: Src) -> Dst
fn saturating_cast_from(src: Src) -> Dst
§impl<T> Tap for T
impl<T> Tap for T
§fn tap_borrow<B>(self, func: impl FnOnce(&B)) -> Self
fn tap_borrow<B>(self, func: impl FnOnce(&B)) -> Self
Borrow<B>
of a value. Read more§fn tap_borrow_mut<B>(self, func: impl FnOnce(&mut B)) -> Self
fn tap_borrow_mut<B>(self, func: impl FnOnce(&mut B)) -> Self
BorrowMut<B>
of a value. Read more§fn tap_ref<R>(self, func: impl FnOnce(&R)) -> Self
fn tap_ref<R>(self, func: impl FnOnce(&R)) -> Self
AsRef<R>
view of a value. Read more§fn tap_ref_mut<R>(self, func: impl FnOnce(&mut R)) -> Self
fn tap_ref_mut<R>(self, func: impl FnOnce(&mut R)) -> Self
AsMut<R>
view of a value. Read more§fn tap_deref<T>(self, func: impl FnOnce(&T)) -> Self
fn tap_deref<T>(self, func: impl FnOnce(&T)) -> Self
Deref::Target
of a value. Read more§fn tap_deref_mut<T>(self, func: impl FnOnce(&mut T)) -> Self
fn tap_deref_mut<T>(self, func: impl FnOnce(&mut T)) -> Self
Deref::Target
of a value. Read more§fn tap_dbg(self, func: impl FnOnce(&Self)) -> Self
fn tap_dbg(self, func: impl FnOnce(&Self)) -> Self
.tap()
only in debug builds, and is erased in release builds.§fn tap_mut_dbg(self, func: impl FnOnce(&mut Self)) -> Self
fn tap_mut_dbg(self, func: impl FnOnce(&mut Self)) -> Self
.tap_mut()
only in debug builds, and is erased in release
builds.§fn tap_borrow_dbg<B>(self, func: impl FnOnce(&B)) -> Self
fn tap_borrow_dbg<B>(self, func: impl FnOnce(&B)) -> Self
.tap_borrow()
only in debug builds, and is erased in release
builds.§fn tap_borrow_mut_dbg<B>(self, func: impl FnOnce(&mut B)) -> Self
fn tap_borrow_mut_dbg<B>(self, func: impl FnOnce(&mut B)) -> Self
.tap_borrow_mut()
only in debug builds, and is erased in release
builds.§fn tap_ref_dbg<R>(self, func: impl FnOnce(&R)) -> Self
fn tap_ref_dbg<R>(self, func: impl FnOnce(&R)) -> Self
.tap_ref()
only in debug builds, and is erased in release
builds.§fn tap_ref_mut_dbg<R>(self, func: impl FnOnce(&mut R)) -> Self
fn tap_ref_mut_dbg<R>(self, func: impl FnOnce(&mut R)) -> Self
.tap_ref_mut()
only in debug builds, and is erased in release
builds.§fn tap_deref_dbg<T>(self, func: impl FnOnce(&T)) -> Self
fn tap_deref_dbg<T>(self, func: impl FnOnce(&T)) -> Self
.tap_deref()
only in debug builds, and is erased in release
builds.