Struct rerun::sdk::archetypes::TextDocument
source · pub struct TextDocument {
pub text: Text,
pub media_type: Option<MediaType>,
}
Expand description
Archetype: A text element intended to be displayed in its own text box.
Supports raw text and markdown.
§Example
§Markdown text document
fn main() -> Result<(), Box<dyn std::error::Error>> {
let rec = rerun::RecordingStreamBuilder::new("rerun_example_text_document").spawn()?;
rec.log(
"text_document",
&rerun::TextDocument::new("Hello, TextDocument!"),
)?;
rec.log(
"markdown",
&rerun::TextDocument::from_markdown(
r#"
[Click here to see the raw text](recording://markdown:Text).
Basic formatting:
| **Feature** | **Alternative** |
| ----------------- | --------------- |
| Plain | |
| *italics* | _italics_ |
| **bold** | __bold__ |
| ~~strikethrough~~ | |
| `inline code` | |
----------------------------------
# Support
- [x] [Commonmark](https://commonmark.org/help/) support
- [x] GitHub-style strikethrough, tables, and checkboxes
- Basic syntax highlighting for:
- [x] C and C++
- [x] Python
- [x] Rust
- [ ] Other languages
# Links
You can link to [an entity](recording://markdown),
a [specific instance of an entity](recording://markdown[#0]),
or a [specific component](recording://markdown:Text).
Of course you can also have [normal https links](https://github.com/rerun-io/rerun), e.g. <https://rerun.io>.
# Image

"#.trim(),
)
)?;
Ok(())
}
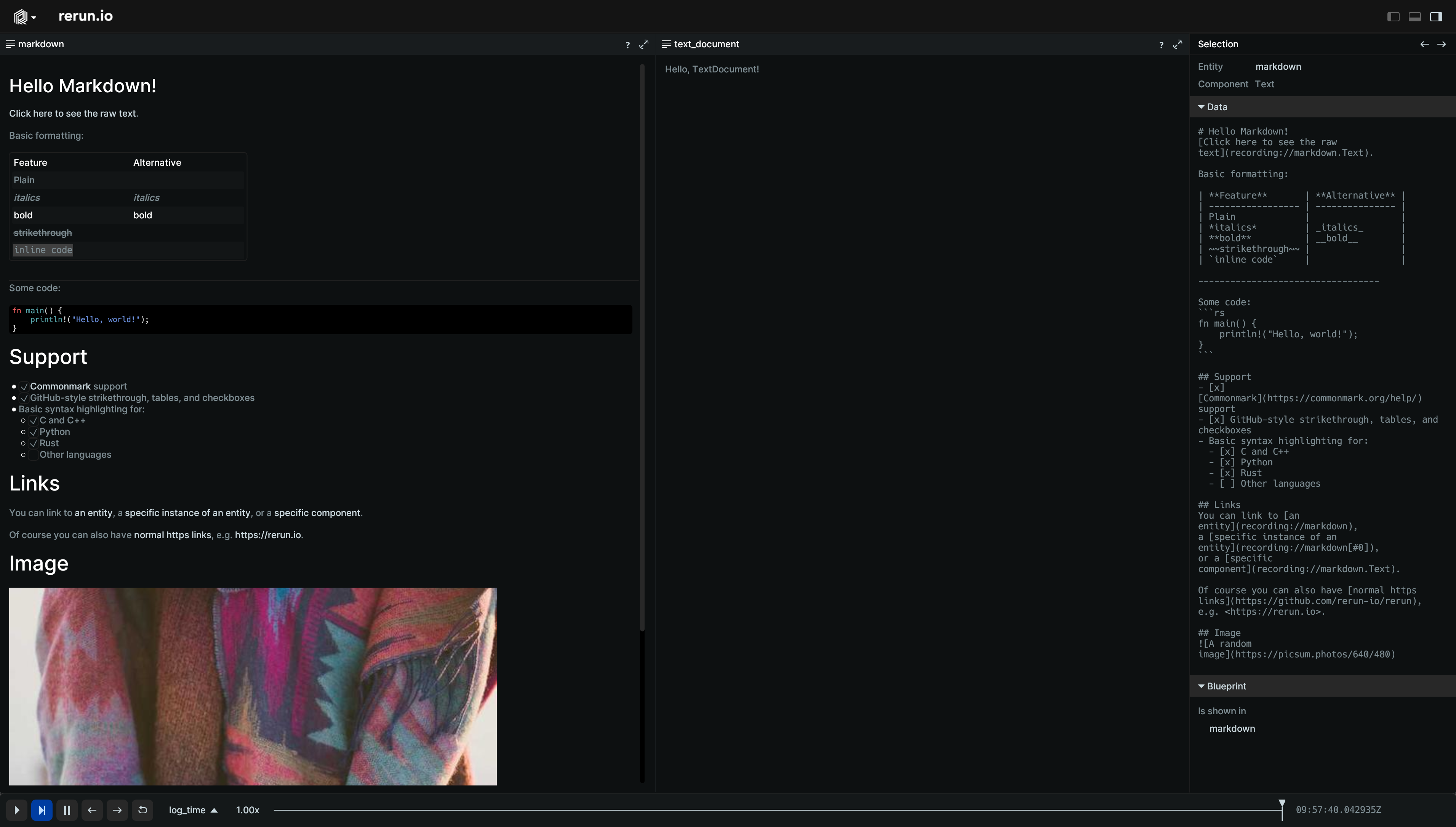
Fields§
§text: Text
Contents of the text document.
media_type: Option<MediaType>
The Media Type of the text.
For instance:
text/plain
text/markdown
If omitted, text/plain
is assumed.
Implementations§
source§impl TextDocument
impl TextDocument
sourcepub const NUM_COMPONENTS: usize = 3usize
pub const NUM_COMPONENTS: usize = 3usize
The total number of components in the archetype: 1 required, 1 recommended, 1 optional
source§impl TextDocument
impl TextDocument
sourcepub fn new(text: impl Into<Text>) -> TextDocument
pub fn new(text: impl Into<Text>) -> TextDocument
Create a new TextDocument
.
sourcepub fn with_media_type(self, media_type: impl Into<MediaType>) -> TextDocument
pub fn with_media_type(self, media_type: impl Into<MediaType>) -> TextDocument
The Media Type of the text.
For instance:
text/plain
text/markdown
If omitted, text/plain
is assumed.
source§impl TextDocument
impl TextDocument
sourcepub fn from_file_path(filepath: impl AsRef<Path>) -> Result<TextDocument, Error>
pub fn from_file_path(filepath: impl AsRef<Path>) -> Result<TextDocument, Error>
Creates a new TextDocument
from a utf8 file.
The media type will be inferred from the path (extension), or the contents if that fails.
sourcepub fn from_file_contents(
contents: Vec<u8>,
media_type: Option<impl Into<MediaType>>,
) -> Result<TextDocument, Error>
pub fn from_file_contents( contents: Vec<u8>, media_type: Option<impl Into<MediaType>>, ) -> Result<TextDocument, Error>
Creates a new TextDocument
from the contents of a utf8 file.
If unspecified, the media type will be inferred from the contents.
sourcepub fn from_markdown(markdown: impl Into<Text>) -> TextDocument
pub fn from_markdown(markdown: impl Into<Text>) -> TextDocument
Creates a new TextDocument
containing Markdown.
Equivalent to TextDocument::new(markdown).with_media_type(MediaType::markdown())
.
Trait Implementations§
source§impl Archetype for TextDocument
impl Archetype for TextDocument
§type Indicator = GenericIndicatorComponent<TextDocument>
type Indicator = GenericIndicatorComponent<TextDocument>
source§fn name() -> ArchetypeName
fn name() -> ArchetypeName
rerun.archetypes.Points2D
.source§fn display_name() -> &'static str
fn display_name() -> &'static str
source§fn indicator() -> ComponentBatchCowWithDescriptor<'static>
fn indicator() -> ComponentBatchCowWithDescriptor<'static>
source§fn required_components() -> Cow<'static, [ComponentDescriptor]>
fn required_components() -> Cow<'static, [ComponentDescriptor]>
source§fn recommended_components() -> Cow<'static, [ComponentDescriptor]>
fn recommended_components() -> Cow<'static, [ComponentDescriptor]>
source§fn optional_components() -> Cow<'static, [ComponentDescriptor]>
fn optional_components() -> Cow<'static, [ComponentDescriptor]>
source§fn all_components() -> Cow<'static, [ComponentDescriptor]>
fn all_components() -> Cow<'static, [ComponentDescriptor]>
source§fn from_arrow2_components(
arrow_data: impl IntoIterator<Item = (ComponentName, Box<dyn Array>)>,
) -> Result<TextDocument, DeserializationError>
fn from_arrow2_components( arrow_data: impl IntoIterator<Item = (ComponentName, Box<dyn Array>)>, ) -> Result<TextDocument, DeserializationError>
ComponentNames
, deserializes them
into this archetype. Read moresource§fn from_arrow(
data: impl IntoIterator<Item = (Field, Arc<dyn Array>)>,
) -> Result<Self, DeserializationError>where
Self: Sized,
fn from_arrow(
data: impl IntoIterator<Item = (Field, Arc<dyn Array>)>,
) -> Result<Self, DeserializationError>where
Self: Sized,
source§fn from_arrow2(
data: impl IntoIterator<Item = (Field, Box<dyn Array>)>,
) -> Result<Self, DeserializationError>where
Self: Sized,
fn from_arrow2(
data: impl IntoIterator<Item = (Field, Box<dyn Array>)>,
) -> Result<Self, DeserializationError>where
Self: Sized,
source§fn from_arrow_components(
data: impl IntoIterator<Item = (ComponentName, Arc<dyn Array>)>,
) -> Result<Self, DeserializationError>where
Self: Sized,
fn from_arrow_components(
data: impl IntoIterator<Item = (ComponentName, Arc<dyn Array>)>,
) -> Result<Self, DeserializationError>where
Self: Sized,
ComponentNames
, deserializes them
into this archetype. Read moresource§impl AsComponents for TextDocument
impl AsComponents for TextDocument
source§fn as_component_batches(&self) -> Vec<ComponentBatchCowWithDescriptor<'_>>
fn as_component_batches(&self) -> Vec<ComponentBatchCowWithDescriptor<'_>>
ComponentBatch
s. Read moresource§impl Clone for TextDocument
impl Clone for TextDocument
source§fn clone(&self) -> TextDocument
fn clone(&self) -> TextDocument
1.0.0 · source§fn clone_from(&mut self, source: &Self)
fn clone_from(&mut self, source: &Self)
source
. Read moresource§impl Debug for TextDocument
impl Debug for TextDocument
source§impl PartialEq for TextDocument
impl PartialEq for TextDocument
source§fn eq(&self, other: &TextDocument) -> bool
fn eq(&self, other: &TextDocument) -> bool
self
and other
values to be equal, and is used
by ==
.source§impl SizeBytes for TextDocument
impl SizeBytes for TextDocument
source§fn heap_size_bytes(&self) -> u64
fn heap_size_bytes(&self) -> u64
self
on the heap, in bytes.source§fn total_size_bytes(&self) -> u64
fn total_size_bytes(&self) -> u64
self
in bytes, accounting for both stack and heap space.source§fn stack_size_bytes(&self) -> u64
fn stack_size_bytes(&self) -> u64
self
on the stack, in bytes. Read moreimpl ArchetypeReflectionMarker for TextDocument
impl Eq for TextDocument
impl StructuralPartialEq for TextDocument
Auto Trait Implementations§
impl Freeze for TextDocument
impl RefUnwindSafe for TextDocument
impl Send for TextDocument
impl Sync for TextDocument
impl Unpin for TextDocument
impl UnwindSafe for TextDocument
Blanket Implementations§
source§impl<T> BorrowMut<T> for Twhere
T: ?Sized,
impl<T> BorrowMut<T> for Twhere
T: ?Sized,
source§fn borrow_mut(&mut self) -> &mut T
fn borrow_mut(&mut self) -> &mut T
source§impl<T> CheckedAs for T
impl<T> CheckedAs for T
source§fn checked_as<Dst>(self) -> Option<Dst>where
T: CheckedCast<Dst>,
fn checked_as<Dst>(self) -> Option<Dst>where
T: CheckedCast<Dst>,
source§impl<Src, Dst> CheckedCastFrom<Src> for Dstwhere
Src: CheckedCast<Dst>,
impl<Src, Dst> CheckedCastFrom<Src> for Dstwhere
Src: CheckedCast<Dst>,
source§fn checked_cast_from(src: Src) -> Option<Dst>
fn checked_cast_from(src: Src) -> Option<Dst>
§impl<T> Downcast for Twhere
T: Any,
impl<T> Downcast for Twhere
T: Any,
§fn into_any(self: Box<T>) -> Box<dyn Any>
fn into_any(self: Box<T>) -> Box<dyn Any>
Box<dyn Trait>
(where Trait: Downcast
) to Box<dyn Any>
. Box<dyn Any>
can
then be further downcast
into Box<ConcreteType>
where ConcreteType
implements Trait
.§fn into_any_rc(self: Rc<T>) -> Rc<dyn Any>
fn into_any_rc(self: Rc<T>) -> Rc<dyn Any>
Rc<Trait>
(where Trait: Downcast
) to Rc<Any>
. Rc<Any>
can then be
further downcast
into Rc<ConcreteType>
where ConcreteType
implements Trait
.§fn as_any(&self) -> &(dyn Any + 'static)
fn as_any(&self) -> &(dyn Any + 'static)
&Trait
(where Trait: Downcast
) to &Any
. This is needed since Rust cannot
generate &Any
’s vtable from &Trait
’s.§fn as_any_mut(&mut self) -> &mut (dyn Any + 'static)
fn as_any_mut(&mut self) -> &mut (dyn Any + 'static)
&mut Trait
(where Trait: Downcast
) to &Any
. This is needed since Rust cannot
generate &mut Any
’s vtable from &mut Trait
’s.§impl<T> DowncastSync for T
impl<T> DowncastSync for T
§impl<Q, K> Equivalent<K> for Q
impl<Q, K> Equivalent<K> for Q
§fn equivalent(&self, key: &K) -> bool
fn equivalent(&self, key: &K) -> bool
§impl<Q, K> Equivalent<K> for Q
impl<Q, K> Equivalent<K> for Q
§fn equivalent(&self, key: &K) -> bool
fn equivalent(&self, key: &K) -> bool
key
and return true
if they are equal.source§impl<Q, K> Equivalent<K> for Q
impl<Q, K> Equivalent<K> for Q
source§fn equivalent(&self, key: &K) -> bool
fn equivalent(&self, key: &K) -> bool
key
and return true
if they are equal.§impl<T> Instrument for T
impl<T> Instrument for T
§fn instrument(self, span: Span) -> Instrumented<Self>
fn instrument(self, span: Span) -> Instrumented<Self>
§fn in_current_span(self) -> Instrumented<Self>
fn in_current_span(self) -> Instrumented<Self>
source§impl<T> IntoEither for T
impl<T> IntoEither for T
source§fn into_either(self, into_left: bool) -> Either<Self, Self> ⓘ
fn into_either(self, into_left: bool) -> Either<Self, Self> ⓘ
self
into a Left
variant of Either<Self, Self>
if into_left
is true
.
Converts self
into a Right
variant of Either<Self, Self>
otherwise. Read moresource§fn into_either_with<F>(self, into_left: F) -> Either<Self, Self> ⓘ
fn into_either_with<F>(self, into_left: F) -> Either<Self, Self> ⓘ
self
into a Left
variant of Either<Self, Self>
if into_left(&self)
returns true
.
Converts self
into a Right
variant of Either<Self, Self>
otherwise. Read moresource§impl<T> IntoRequest<T> for T
impl<T> IntoRequest<T> for T
source§fn into_request(self) -> Request<T>
fn into_request(self) -> Request<T>
T
in a tonic::Request