pub struct Clear {
pub is_recursive: Option<SerializedComponentBatch>,
}
Expand description
Archetype: Empties all the components of an entity.
The presence of a clear means that a latest-at query of components at a given path(s) will not return any components that were logged at those paths before the clear. Any logged components after the clear are unaffected by the clear.
This implies that a range query that includes time points that are before the clear, still returns all components at the given path(s). Meaning that in practice clears are ineffective when making use of visible time ranges. Scalar plots are an exception: they track clears and use them to represent holes in the data (i.e. discontinuous lines).
§Example
§Flat
use rerun::external::glam;
fn main() -> Result<(), Box<dyn std::error::Error>> {
let rec = rerun::RecordingStreamBuilder::new("rerun_example_clear").spawn()?;
#[rustfmt::skip]
let (vectors, origins, colors) = (
[glam::Vec3::X, glam::Vec3::NEG_Y, glam::Vec3::NEG_X, glam::Vec3::Y],
[(-0.5, 0.5, 0.0), (0.5, 0.5, 0.0), (0.5, -0.5, 0.0), (-0.5, -0.5, 0.0)],
[(200, 0, 0), (0, 200, 0), (0, 0, 200), (200, 0, 200)],
);
// Log a handful of arrows.
for (i, ((vector, origin), color)) in vectors.into_iter().zip(origins).zip(colors).enumerate() {
rec.log(
format!("arrows/{i}"),
&rerun::Arrows3D::from_vectors([vector])
.with_origins([origin])
.with_colors([rerun::Color::from_rgb(color.0, color.1, color.2)]),
)?;
}
// Now clear them, one by one on each tick.
for i in 0..vectors.len() {
rec.log(format!("arrows/{i}"), &rerun::Clear::flat())?;
}
Ok(())
}
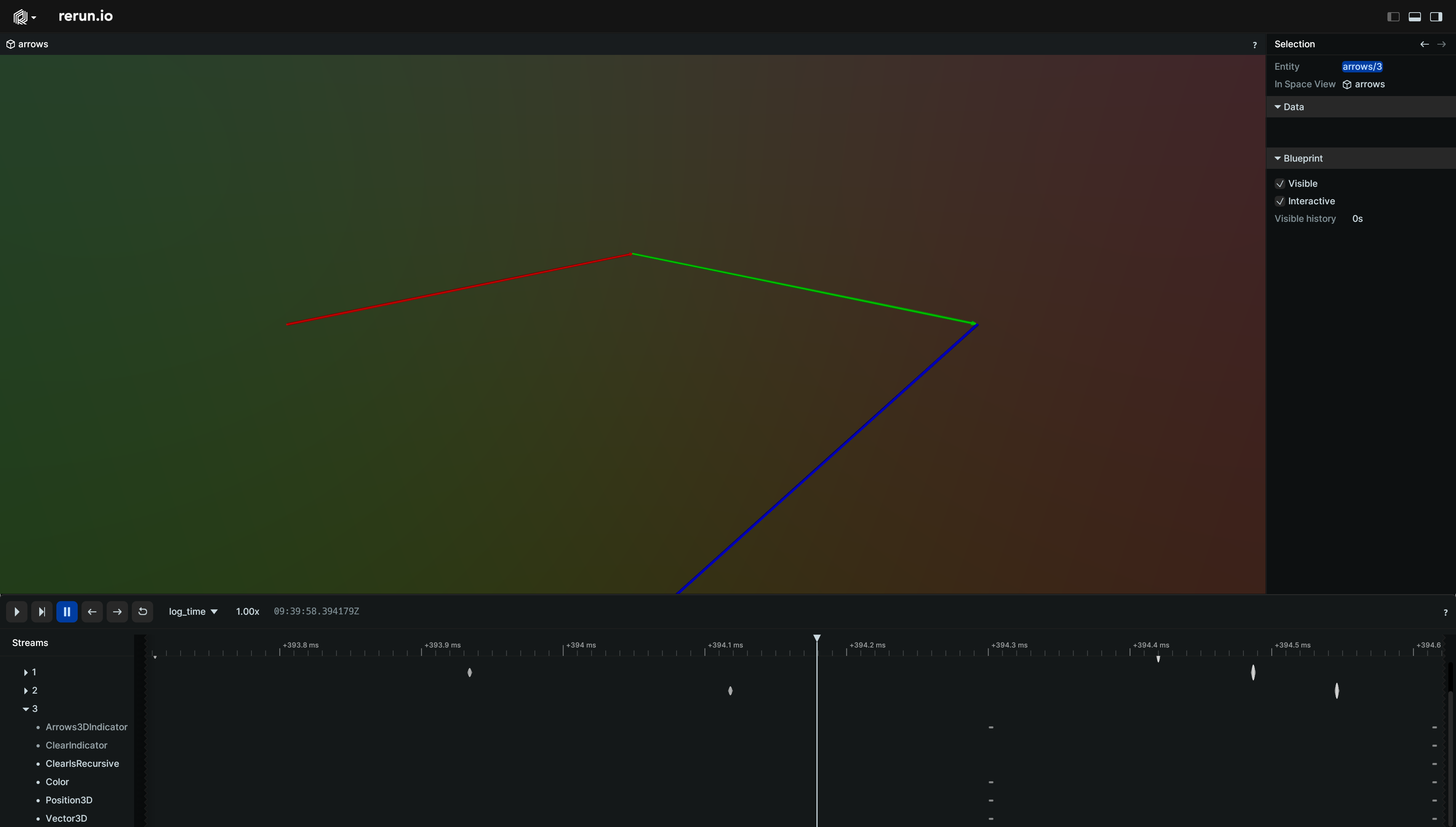
Fields§
§is_recursive: Option<SerializedComponentBatch>
Implementations§
Source§impl Clear
impl Clear
Sourcepub fn descriptor_is_recursive() -> ComponentDescriptor
pub fn descriptor_is_recursive() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::is_recursive
.
Sourcepub fn descriptor_indicator() -> ComponentDescriptor
pub fn descriptor_indicator() -> ComponentDescriptor
Returns the ComponentDescriptor
for the associated indicator component.
Source§impl Clear
impl Clear
Sourcepub const NUM_COMPONENTS: usize = 2usize
pub const NUM_COMPONENTS: usize = 2usize
The total number of components in the archetype: 1 required, 1 recommended, 0 optional
Source§impl Clear
impl Clear
Sourcepub fn new(is_recursive: impl Into<ClearIsRecursive>) -> Clear
pub fn new(is_recursive: impl Into<ClearIsRecursive>) -> Clear
Create a new Clear
.
Sourcepub fn update_fields() -> Clear
pub fn update_fields() -> Clear
Update only some specific fields of a Clear
.
Sourcepub fn clear_fields() -> Clear
pub fn clear_fields() -> Clear
Clear all the fields of a Clear
.
Sourcepub fn columns<I>(
self,
_lengths: I,
) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
pub fn columns<I>( self, _lengths: I, ) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
Partitions the component data into multiple sub-batches.
Specifically, this transforms the existing SerializedComponentBatch
es data into SerializedComponentColumn
s
instead, via SerializedComponentBatch::partitioned
.
This makes it possible to use RecordingStream::send_columns
to send columnar data directly into Rerun.
The specified lengths
must sum to the total length of the component batch.
Sourcepub fn columns_of_unit_batches(
self,
) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
pub fn columns_of_unit_batches( self, ) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
Helper to partition the component data into unit-length sub-batches.
This is semantically similar to calling Self::columns
with std::iter::take(1).repeat(n)
,
where n
is automatically guessed.
pub fn with_is_recursive( self, is_recursive: impl Into<ClearIsRecursive>, ) -> Clear
Sourcepub fn with_many_is_recursive(
self,
is_recursive: impl IntoIterator<Item = impl Into<ClearIsRecursive>>,
) -> Clear
pub fn with_many_is_recursive( self, is_recursive: impl IntoIterator<Item = impl Into<ClearIsRecursive>>, ) -> Clear
This method makes it possible to pack multiple crate::components::ClearIsRecursive
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_is_recursive
should
be used when logging a single row’s worth of data.
Trait Implementations§
Source§impl Archetype for Clear
impl Archetype for Clear
Source§type Indicator = GenericIndicatorComponent<Clear>
type Indicator = GenericIndicatorComponent<Clear>
Source§fn name() -> ArchetypeName
fn name() -> ArchetypeName
rerun.archetypes.Points2D
.Source§fn display_name() -> &'static str
fn display_name() -> &'static str
Source§fn required_components() -> Cow<'static, [ComponentDescriptor]>
fn required_components() -> Cow<'static, [ComponentDescriptor]>
Source§fn recommended_components() -> Cow<'static, [ComponentDescriptor]>
fn recommended_components() -> Cow<'static, [ComponentDescriptor]>
Source§fn optional_components() -> Cow<'static, [ComponentDescriptor]>
fn optional_components() -> Cow<'static, [ComponentDescriptor]>
Source§fn all_components() -> Cow<'static, [ComponentDescriptor]>
fn all_components() -> Cow<'static, [ComponentDescriptor]>
Source§fn from_arrow_components(
arrow_data: impl IntoIterator<Item = (ComponentDescriptor, Arc<dyn Array>)>,
) -> Result<Clear, DeserializationError>
fn from_arrow_components( arrow_data: impl IntoIterator<Item = (ComponentDescriptor, Arc<dyn Array>)>, ) -> Result<Clear, DeserializationError>
ComponentNames
, deserializes them
into this archetype. Read moreSource§fn from_arrow(
data: impl IntoIterator<Item = (Field, Arc<dyn Array>)>,
) -> Result<Self, DeserializationError>where
Self: Sized,
fn from_arrow(
data: impl IntoIterator<Item = (Field, Arc<dyn Array>)>,
) -> Result<Self, DeserializationError>where
Self: Sized,
Source§impl AsComponents for Clear
impl AsComponents for Clear
Source§fn as_serialized_batches(&self) -> Vec<SerializedComponentBatch>
fn as_serialized_batches(&self) -> Vec<SerializedComponentBatch>
SerializedComponentBatch
es. Read moreSource§impl SizeBytes for Clear
impl SizeBytes for Clear
Source§fn heap_size_bytes(&self) -> u64
fn heap_size_bytes(&self) -> u64
self
uses on the heap. Read moreSource§fn total_size_bytes(&self) -> u64
fn total_size_bytes(&self) -> u64
self
in bytes, accounting for both stack and heap space.Source§fn stack_size_bytes(&self) -> u64
fn stack_size_bytes(&self) -> u64
self
on the stack, in bytes. Read moreimpl ArchetypeReflectionMarker for Clear
impl StructuralPartialEq for Clear
Auto Trait Implementations§
impl Freeze for Clear
impl !RefUnwindSafe for Clear
impl Send for Clear
impl Sync for Clear
impl Unpin for Clear
impl !UnwindSafe for Clear
Blanket Implementations§
Source§impl<T> BorrowMut<T> for Twhere
T: ?Sized,
impl<T> BorrowMut<T> for Twhere
T: ?Sized,
Source§fn borrow_mut(&mut self) -> &mut T
fn borrow_mut(&mut self) -> &mut T
Source§impl<T> CheckedAs for T
impl<T> CheckedAs for T
Source§fn checked_as<Dst>(self) -> Option<Dst>where
T: CheckedCast<Dst>,
fn checked_as<Dst>(self) -> Option<Dst>where
T: CheckedCast<Dst>,
Source§impl<Src, Dst> CheckedCastFrom<Src> for Dstwhere
Src: CheckedCast<Dst>,
impl<Src, Dst> CheckedCastFrom<Src> for Dstwhere
Src: CheckedCast<Dst>,
Source§fn checked_cast_from(src: Src) -> Option<Dst>
fn checked_cast_from(src: Src) -> Option<Dst>
Source§impl<T> CloneToUninit for Twhere
T: Clone,
impl<T> CloneToUninit for Twhere
T: Clone,
§impl<T> Downcast for Twhere
T: Any,
impl<T> Downcast for Twhere
T: Any,
§fn into_any(self: Box<T>) -> Box<dyn Any>
fn into_any(self: Box<T>) -> Box<dyn Any>
Box<dyn Trait>
(where Trait: Downcast
) to Box<dyn Any>
. Box<dyn Any>
can
then be further downcast
into Box<ConcreteType>
where ConcreteType
implements Trait
.§fn into_any_rc(self: Rc<T>) -> Rc<dyn Any>
fn into_any_rc(self: Rc<T>) -> Rc<dyn Any>
Rc<Trait>
(where Trait: Downcast
) to Rc<Any>
. Rc<Any>
can then be
further downcast
into Rc<ConcreteType>
where ConcreteType
implements Trait
.§fn as_any(&self) -> &(dyn Any + 'static)
fn as_any(&self) -> &(dyn Any + 'static)
&Trait
(where Trait: Downcast
) to &Any
. This is needed since Rust cannot
generate &Any
’s vtable from &Trait
’s.§fn as_any_mut(&mut self) -> &mut (dyn Any + 'static)
fn as_any_mut(&mut self) -> &mut (dyn Any + 'static)
&mut Trait
(where Trait: Downcast
) to &Any
. This is needed since Rust cannot
generate &mut Any
’s vtable from &mut Trait
’s.§impl<T> DowncastSync for T
impl<T> DowncastSync for T
§impl<T> Instrument for T
impl<T> Instrument for T
§fn instrument(self, span: Span) -> Instrumented<Self>
fn instrument(self, span: Span) -> Instrumented<Self>
§fn in_current_span(self) -> Instrumented<Self>
fn in_current_span(self) -> Instrumented<Self>
Source§impl<T> IntoEither for T
impl<T> IntoEither for T
Source§fn into_either(self, into_left: bool) -> Either<Self, Self>
fn into_either(self, into_left: bool) -> Either<Self, Self>
self
into a Left
variant of Either<Self, Self>
if into_left
is true
.
Converts self
into a Right
variant of Either<Self, Self>
otherwise. Read moreSource§fn into_either_with<F>(self, into_left: F) -> Either<Self, Self>
fn into_either_with<F>(self, into_left: F) -> Either<Self, Self>
self
into a Left
variant of Either<Self, Self>
if into_left(&self)
returns true
.
Converts self
into a Right
variant of Either<Self, Self>
otherwise. Read more