pub struct Mesh3D {
pub vertex_positions: Option<SerializedComponentBatch>,
pub triangle_indices: Option<SerializedComponentBatch>,
pub vertex_normals: Option<SerializedComponentBatch>,
pub vertex_colors: Option<SerializedComponentBatch>,
pub vertex_texcoords: Option<SerializedComponentBatch>,
pub albedo_factor: Option<SerializedComponentBatch>,
pub albedo_texture_buffer: Option<SerializedComponentBatch>,
pub albedo_texture_format: Option<SerializedComponentBatch>,
pub class_ids: Option<SerializedComponentBatch>,
}
Expand description
Archetype: A 3D triangle mesh as specified by its per-mesh and per-vertex properties.
See also archetypes::Asset3D
.
If there are multiple archetypes::InstancePoses3D
instances logged to the same entity as a mesh,
an instance of the mesh will be drawn for each transform.
§Examples
§Simple indexed 3D mesh
fn main() -> Result<(), Box<dyn std::error::Error>> {
let rec = rerun::RecordingStreamBuilder::new("rerun_example_mesh3d_indexed").spawn()?;
rec.log(
"triangle",
&rerun::Mesh3D::new([[0.0, 1.0, 0.0], [1.0, 0.0, 0.0], [0.0, 0.0, 0.0]])
.with_vertex_normals([[0.0, 0.0, 1.0]])
.with_vertex_colors([0x0000FFFF, 0x00FF00FF, 0xFF0000FF])
.with_triangle_indices([[2, 1, 0]]),
)?;
Ok(())
}
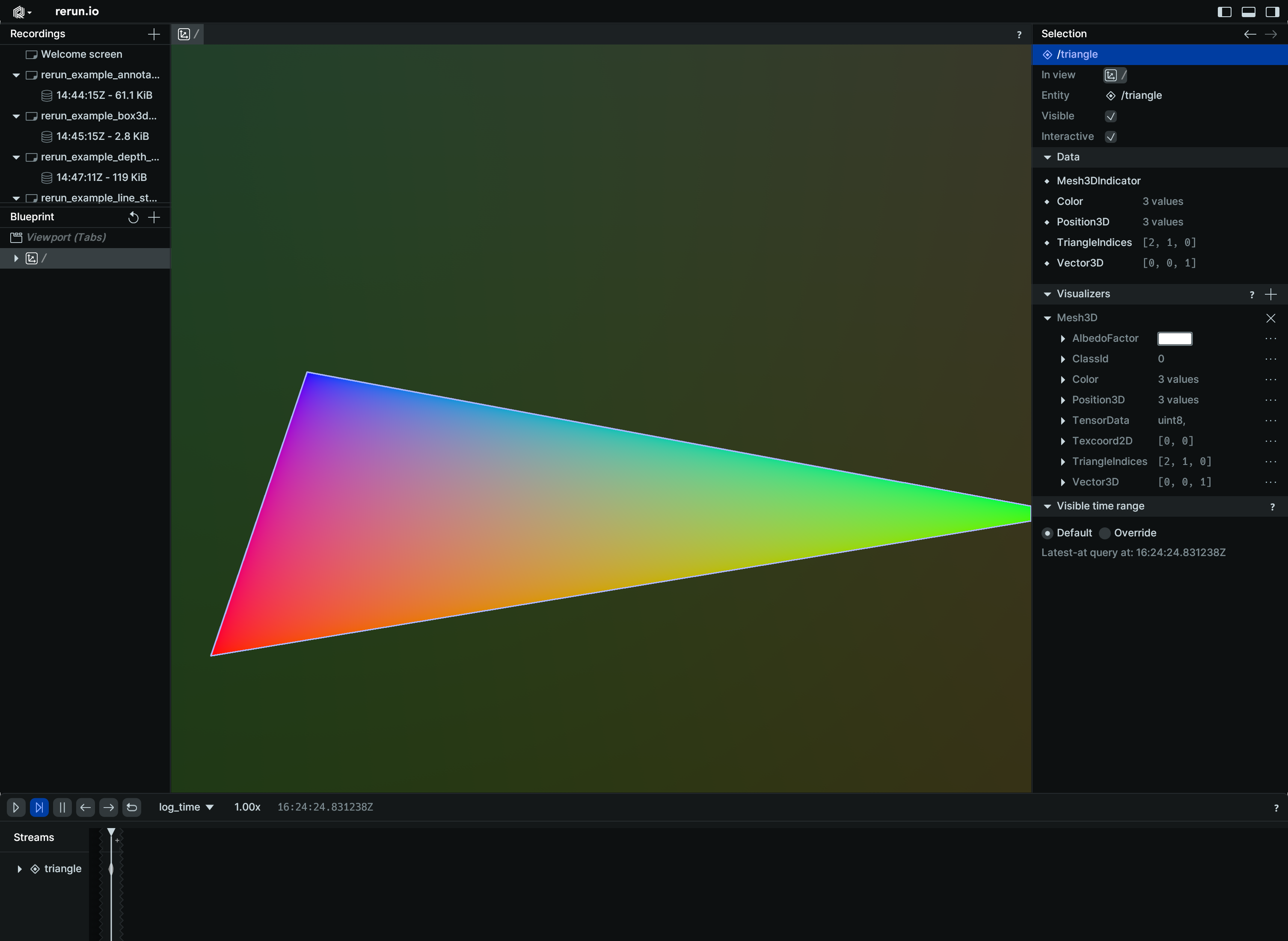
§3D mesh with instancing
fn main() -> Result<(), Box<dyn std::error::Error>> {
let rec = rerun::RecordingStreamBuilder::new("rerun_example_mesh3d_instancing").spawn()?;
rec.set_time_sequence("frame", 0);
rec.log(
"shape",
&rerun::Mesh3D::new([
[1.0, 1.0, 1.0],
[-1.0, -1.0, 1.0],
[-1.0, 1.0, -1.0],
[1.0, -1.0, -1.0],
])
.with_triangle_indices([[0, 1, 2], [0, 1, 3], [0, 2, 3], [1, 2, 3]])
.with_vertex_colors([0xFF0000FF, 0x00FF00FF, 0x00000FFFF, 0xFFFF00FF]),
)?;
// This box will not be affected by its parent's instance poses!
rec.log(
"shape/box",
&rerun::Boxes3D::from_half_sizes([[5.0, 5.0, 5.0]]),
)?;
for i in 0..100 {
rec.set_time_sequence("frame", i);
rec.log(
"shape",
&rerun::InstancePoses3D::new()
.with_translations([
[2.0, 0.0, 0.0],
[0.0, 2.0, 0.0],
[0.0, -2.0, 0.0],
[-2.0, 0.0, 0.0],
])
.with_rotation_axis_angles([rerun::RotationAxisAngle::new(
[0.0, 0.0, 1.0],
rerun::Angle::from_degrees(i as f32 * 2.0),
)]),
)?;
}
Ok(())
}
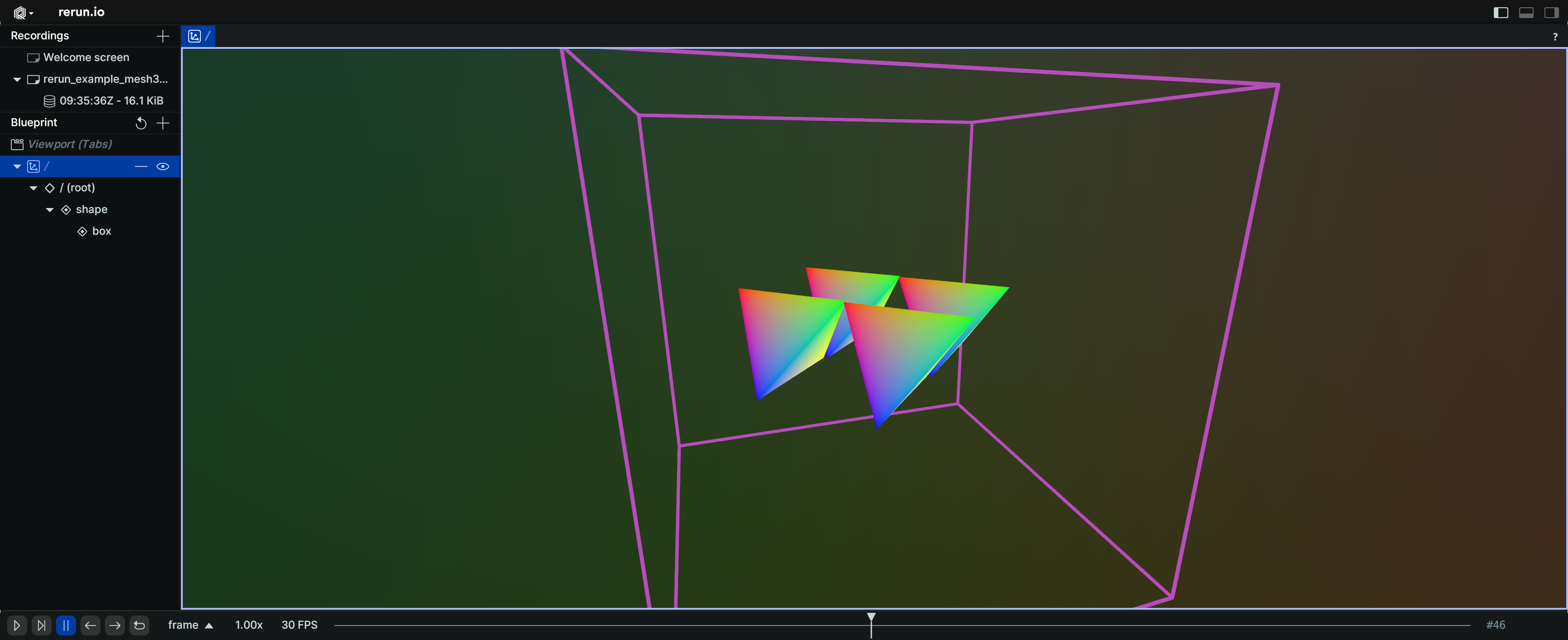
Fields§
§vertex_positions: Option<SerializedComponentBatch>
The positions of each vertex.
If no triangle_indices
are specified, then each triplet of positions is interpreted as a triangle.
triangle_indices: Option<SerializedComponentBatch>
Optional indices for the triangles that make up the mesh.
vertex_normals: Option<SerializedComponentBatch>
An optional normal for each vertex.
vertex_colors: Option<SerializedComponentBatch>
An optional color for each vertex.
vertex_texcoords: Option<SerializedComponentBatch>
An optional uv texture coordinate for each vertex.
albedo_factor: Option<SerializedComponentBatch>
A color multiplier applied to the whole mesh.
albedo_texture_buffer: Option<SerializedComponentBatch>
Optional albedo texture.
Used with the components::Texcoord2D
of the mesh.
Currently supports only sRGB(A) textures, ignoring alpha.
(meaning that the tensor must have 3 or 4 channels and use the u8
format)
albedo_texture_format: Option<SerializedComponentBatch>
The format of the albedo_texture_buffer
, if any.
class_ids: Option<SerializedComponentBatch>
Optional class Ids for the vertices.
The components::ClassId
provides colors and labels if not specified explicitly.
Implementations§
Source§impl Mesh3D
impl Mesh3D
Sourcepub fn descriptor_vertex_positions() -> ComponentDescriptor
pub fn descriptor_vertex_positions() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::vertex_positions
.
Sourcepub fn descriptor_triangle_indices() -> ComponentDescriptor
pub fn descriptor_triangle_indices() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::triangle_indices
.
Sourcepub fn descriptor_vertex_normals() -> ComponentDescriptor
pub fn descriptor_vertex_normals() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::vertex_normals
.
Sourcepub fn descriptor_vertex_colors() -> ComponentDescriptor
pub fn descriptor_vertex_colors() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::vertex_colors
.
Sourcepub fn descriptor_vertex_texcoords() -> ComponentDescriptor
pub fn descriptor_vertex_texcoords() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::vertex_texcoords
.
Sourcepub fn descriptor_albedo_factor() -> ComponentDescriptor
pub fn descriptor_albedo_factor() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::albedo_factor
.
Sourcepub fn descriptor_albedo_texture_buffer() -> ComponentDescriptor
pub fn descriptor_albedo_texture_buffer() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::albedo_texture_buffer
.
Sourcepub fn descriptor_albedo_texture_format() -> ComponentDescriptor
pub fn descriptor_albedo_texture_format() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::albedo_texture_format
.
Sourcepub fn descriptor_class_ids() -> ComponentDescriptor
pub fn descriptor_class_ids() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::class_ids
.
Sourcepub fn descriptor_indicator() -> ComponentDescriptor
pub fn descriptor_indicator() -> ComponentDescriptor
Returns the ComponentDescriptor
for the associated indicator component.
Source§impl Mesh3D
impl Mesh3D
Sourcepub const NUM_COMPONENTS: usize = 10usize
pub const NUM_COMPONENTS: usize = 10usize
The total number of components in the archetype: 1 required, 3 recommended, 6 optional
Source§impl Mesh3D
impl Mesh3D
Sourcepub fn new(
vertex_positions: impl IntoIterator<Item = impl Into<Position3D>>,
) -> Self
pub fn new( vertex_positions: impl IntoIterator<Item = impl Into<Position3D>>, ) -> Self
Create a new Mesh3D
.
Sourcepub fn update_fields() -> Self
pub fn update_fields() -> Self
Update only some specific fields of a Mesh3D
.
Sourcepub fn clear_fields() -> Self
pub fn clear_fields() -> Self
Clear all the fields of a Mesh3D
.
Sourcepub fn columns<I>(
self,
_lengths: I,
) -> SerializationResult<impl Iterator<Item = SerializedComponentColumn>>
pub fn columns<I>( self, _lengths: I, ) -> SerializationResult<impl Iterator<Item = SerializedComponentColumn>>
Partitions the component data into multiple sub-batches.
Specifically, this transforms the existing SerializedComponentBatch
es data into SerializedComponentColumn
s
instead, via SerializedComponentBatch::partitioned
.
This makes it possible to use RecordingStream::send_columns
to send columnar data directly into Rerun.
The specified lengths
must sum to the total length of the component batch.
Sourcepub fn columns_of_unit_batches(
self,
) -> SerializationResult<impl Iterator<Item = SerializedComponentColumn>>
pub fn columns_of_unit_batches( self, ) -> SerializationResult<impl Iterator<Item = SerializedComponentColumn>>
Helper to partition the component data into unit-length sub-batches.
This is semantically similar to calling Self::columns
with std::iter::take(1).repeat(n)
,
where n
is automatically guessed.
Sourcepub fn with_vertex_positions(
self,
vertex_positions: impl IntoIterator<Item = impl Into<Position3D>>,
) -> Self
pub fn with_vertex_positions( self, vertex_positions: impl IntoIterator<Item = impl Into<Position3D>>, ) -> Self
The positions of each vertex.
If no triangle_indices
are specified, then each triplet of positions is interpreted as a triangle.
Sourcepub fn with_triangle_indices(
self,
triangle_indices: impl IntoIterator<Item = impl Into<TriangleIndices>>,
) -> Self
pub fn with_triangle_indices( self, triangle_indices: impl IntoIterator<Item = impl Into<TriangleIndices>>, ) -> Self
Optional indices for the triangles that make up the mesh.
Sourcepub fn with_vertex_normals(
self,
vertex_normals: impl IntoIterator<Item = impl Into<Vector3D>>,
) -> Self
pub fn with_vertex_normals( self, vertex_normals: impl IntoIterator<Item = impl Into<Vector3D>>, ) -> Self
An optional normal for each vertex.
Sourcepub fn with_vertex_colors(
self,
vertex_colors: impl IntoIterator<Item = impl Into<Color>>,
) -> Self
pub fn with_vertex_colors( self, vertex_colors: impl IntoIterator<Item = impl Into<Color>>, ) -> Self
An optional color for each vertex.
Sourcepub fn with_vertex_texcoords(
self,
vertex_texcoords: impl IntoIterator<Item = impl Into<Texcoord2D>>,
) -> Self
pub fn with_vertex_texcoords( self, vertex_texcoords: impl IntoIterator<Item = impl Into<Texcoord2D>>, ) -> Self
An optional uv texture coordinate for each vertex.
Sourcepub fn with_albedo_factor(self, albedo_factor: impl Into<AlbedoFactor>) -> Self
pub fn with_albedo_factor(self, albedo_factor: impl Into<AlbedoFactor>) -> Self
A color multiplier applied to the whole mesh.
Sourcepub fn with_many_albedo_factor(
self,
albedo_factor: impl IntoIterator<Item = impl Into<AlbedoFactor>>,
) -> Self
pub fn with_many_albedo_factor( self, albedo_factor: impl IntoIterator<Item = impl Into<AlbedoFactor>>, ) -> Self
This method makes it possible to pack multiple crate::components::AlbedoFactor
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_albedo_factor
should
be used when logging a single row’s worth of data.
Sourcepub fn with_albedo_texture_buffer(
self,
albedo_texture_buffer: impl Into<ImageBuffer>,
) -> Self
pub fn with_albedo_texture_buffer( self, albedo_texture_buffer: impl Into<ImageBuffer>, ) -> Self
Optional albedo texture.
Used with the components::Texcoord2D
of the mesh.
Currently supports only sRGB(A) textures, ignoring alpha.
(meaning that the tensor must have 3 or 4 channels and use the u8
format)
Sourcepub fn with_many_albedo_texture_buffer(
self,
albedo_texture_buffer: impl IntoIterator<Item = impl Into<ImageBuffer>>,
) -> Self
pub fn with_many_albedo_texture_buffer( self, albedo_texture_buffer: impl IntoIterator<Item = impl Into<ImageBuffer>>, ) -> Self
This method makes it possible to pack multiple crate::components::ImageBuffer
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_albedo_texture_buffer
should
be used when logging a single row’s worth of data.
Sourcepub fn with_albedo_texture_format(
self,
albedo_texture_format: impl Into<ImageFormat>,
) -> Self
pub fn with_albedo_texture_format( self, albedo_texture_format: impl Into<ImageFormat>, ) -> Self
The format of the albedo_texture_buffer
, if any.
Sourcepub fn with_many_albedo_texture_format(
self,
albedo_texture_format: impl IntoIterator<Item = impl Into<ImageFormat>>,
) -> Self
pub fn with_many_albedo_texture_format( self, albedo_texture_format: impl IntoIterator<Item = impl Into<ImageFormat>>, ) -> Self
This method makes it possible to pack multiple crate::components::ImageFormat
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_albedo_texture_format
should
be used when logging a single row’s worth of data.
Sourcepub fn with_class_ids(
self,
class_ids: impl IntoIterator<Item = impl Into<ClassId>>,
) -> Self
pub fn with_class_ids( self, class_ids: impl IntoIterator<Item = impl Into<ClassId>>, ) -> Self
Optional class Ids for the vertices.
The components::ClassId
provides colors and labels if not specified explicitly.
Source§impl Mesh3D
impl Mesh3D
Sourcepub fn with_albedo_texture_image(self, image: impl Into<Image>) -> Self
pub fn with_albedo_texture_image(self, image: impl Into<Image>) -> Self
Use this image as the albedo texture.
Sourcepub fn with_albedo_texture(
self,
image_format: impl Into<ImageFormat>,
image_buffer: impl Into<ImageBuffer>,
) -> Self
pub fn with_albedo_texture( self, image_format: impl Into<ImageFormat>, image_buffer: impl Into<ImageBuffer>, ) -> Self
Use this image as the albedo texture.
Sourcepub fn sanity_check(&self) -> Result<(), Mesh3DError>
pub fn sanity_check(&self) -> Result<(), Mesh3DError>
Check that this is a valid mesh, e.g. that the vertex indices are within bounds and that we have the same number of positions and normals (if any).
Only use this when logging a whole new mesh. Not meaningful for field updates!
Sourcepub fn num_vertices(&self) -> usize
pub fn num_vertices(&self) -> usize
The total number of vertices.
Sourcepub fn num_triangles(&self) -> usize
pub fn num_triangles(&self) -> usize
The total number of triangles.
Trait Implementations§
Source§impl Archetype for Mesh3D
impl Archetype for Mesh3D
Source§type Indicator = GenericIndicatorComponent<Mesh3D>
type Indicator = GenericIndicatorComponent<Mesh3D>
Source§fn name() -> ArchetypeName
fn name() -> ArchetypeName
rerun.archetypes.Points2D
.Source§fn display_name() -> &'static str
fn display_name() -> &'static str
Source§fn required_components() -> Cow<'static, [ComponentDescriptor]>
fn required_components() -> Cow<'static, [ComponentDescriptor]>
Source§fn recommended_components() -> Cow<'static, [ComponentDescriptor]>
fn recommended_components() -> Cow<'static, [ComponentDescriptor]>
Source§fn optional_components() -> Cow<'static, [ComponentDescriptor]>
fn optional_components() -> Cow<'static, [ComponentDescriptor]>
Source§fn all_components() -> Cow<'static, [ComponentDescriptor]>
fn all_components() -> Cow<'static, [ComponentDescriptor]>
Source§fn from_arrow_components(
arrow_data: impl IntoIterator<Item = (ComponentDescriptor, ArrayRef)>,
) -> DeserializationResult<Self>
fn from_arrow_components( arrow_data: impl IntoIterator<Item = (ComponentDescriptor, ArrayRef)>, ) -> DeserializationResult<Self>
ComponentNames
, deserializes them
into this archetype. Read moreSource§fn from_arrow(
data: impl IntoIterator<Item = (Field, Arc<dyn Array>)>,
) -> Result<Self, DeserializationError>where
Self: Sized,
fn from_arrow(
data: impl IntoIterator<Item = (Field, Arc<dyn Array>)>,
) -> Result<Self, DeserializationError>where
Self: Sized,
Source§impl AsComponents for Mesh3D
impl AsComponents for Mesh3D
Source§fn as_serialized_batches(&self) -> Vec<SerializedComponentBatch>
fn as_serialized_batches(&self) -> Vec<SerializedComponentBatch>
SerializedComponentBatch
es. Read moreSource§impl SizeBytes for Mesh3D
impl SizeBytes for Mesh3D
Source§fn heap_size_bytes(&self) -> u64
fn heap_size_bytes(&self) -> u64
self
uses on the heap. Read moreSource§fn total_size_bytes(&self) -> u64
fn total_size_bytes(&self) -> u64
self
in bytes, accounting for both stack and heap space.Source§fn stack_size_bytes(&self) -> u64
fn stack_size_bytes(&self) -> u64
self
on the stack, in bytes. Read moreimpl ArchetypeReflectionMarker for Mesh3D
impl StructuralPartialEq for Mesh3D
Auto Trait Implementations§
impl Freeze for Mesh3D
impl !RefUnwindSafe for Mesh3D
impl Send for Mesh3D
impl Sync for Mesh3D
impl Unpin for Mesh3D
impl !UnwindSafe for Mesh3D
Blanket Implementations§
Source§impl<T> BorrowMut<T> for Twhere
T: ?Sized,
impl<T> BorrowMut<T> for Twhere
T: ?Sized,
Source§fn borrow_mut(&mut self) -> &mut T
fn borrow_mut(&mut self) -> &mut T
Source§impl<T> CheckedAs for T
impl<T> CheckedAs for T
Source§fn checked_as<Dst>(self) -> Option<Dst>where
T: CheckedCast<Dst>,
fn checked_as<Dst>(self) -> Option<Dst>where
T: CheckedCast<Dst>,
Source§impl<Src, Dst> CheckedCastFrom<Src> for Dstwhere
Src: CheckedCast<Dst>,
impl<Src, Dst> CheckedCastFrom<Src> for Dstwhere
Src: CheckedCast<Dst>,
Source§fn checked_cast_from(src: Src) -> Option<Dst>
fn checked_cast_from(src: Src) -> Option<Dst>
Source§impl<T> CloneToUninit for Twhere
T: Clone,
impl<T> CloneToUninit for Twhere
T: Clone,
§impl<T> Downcast for Twhere
T: Any,
impl<T> Downcast for Twhere
T: Any,
§fn into_any(self: Box<T>) -> Box<dyn Any>
fn into_any(self: Box<T>) -> Box<dyn Any>
Box<dyn Trait>
(where Trait: Downcast
) to Box<dyn Any>
. Box<dyn Any>
can
then be further downcast
into Box<ConcreteType>
where ConcreteType
implements Trait
.§fn into_any_rc(self: Rc<T>) -> Rc<dyn Any>
fn into_any_rc(self: Rc<T>) -> Rc<dyn Any>
Rc<Trait>
(where Trait: Downcast
) to Rc<Any>
. Rc<Any>
can then be
further downcast
into Rc<ConcreteType>
where ConcreteType
implements Trait
.§fn as_any(&self) -> &(dyn Any + 'static)
fn as_any(&self) -> &(dyn Any + 'static)
&Trait
(where Trait: Downcast
) to &Any
. This is needed since Rust cannot
generate &Any
’s vtable from &Trait
’s.§fn as_any_mut(&mut self) -> &mut (dyn Any + 'static)
fn as_any_mut(&mut self) -> &mut (dyn Any + 'static)
&mut Trait
(where Trait: Downcast
) to &Any
. This is needed since Rust cannot
generate &mut Any
’s vtable from &mut Trait
’s.§impl<T> DowncastSync for T
impl<T> DowncastSync for T
§impl<T> Instrument for T
impl<T> Instrument for T
§fn instrument(self, span: Span) -> Instrumented<Self>
fn instrument(self, span: Span) -> Instrumented<Self>
§fn in_current_span(self) -> Instrumented<Self>
fn in_current_span(self) -> Instrumented<Self>
Source§impl<T> IntoEither for T
impl<T> IntoEither for T
Source§fn into_either(self, into_left: bool) -> Either<Self, Self>
fn into_either(self, into_left: bool) -> Either<Self, Self>
self
into a Left
variant of Either<Self, Self>
if into_left
is true
.
Converts self
into a Right
variant of Either<Self, Self>
otherwise. Read moreSource§fn into_either_with<F>(self, into_left: F) -> Either<Self, Self>
fn into_either_with<F>(self, into_left: F) -> Either<Self, Self>
self
into a Left
variant of Either<Self, Self>
if into_left(&self)
returns true
.
Converts self
into a Right
variant of Either<Self, Self>
otherwise. Read more