Struct rerun::archetypes::Image
source · pub struct Image {
pub buffer: Option<SerializedComponentBatch>,
pub format: Option<SerializedComponentBatch>,
pub opacity: Option<SerializedComponentBatch>,
pub draw_order: Option<SerializedComponentBatch>,
}
Expand description
Archetype: A monochrome or color image.
See also archetypes::DepthImage
and archetypes::SegmentationImage
.
Rerun also supports compressed images (JPEG, PNG, …), using archetypes::EncodedImage
.
For images that refer to video frames see archetypes::VideoFrameReference
.
Compressing images or using video data instead can save a lot of bandwidth and memory.
The raw image data is stored as a single buffer of bytes in a components::Blob
.
The meaning of these bytes is determined by the components::ImageFormat
which specifies the resolution
and the pixel format (e.g. RGB, RGBA, …).
The order of dimensions in the underlying components::Blob
follows the typical
row-major, interleaved-pixel image format.
§Examples
§image_simple
:
use ndarray::{s, Array, ShapeBuilder};
fn main() -> Result<(), Box<dyn std::error::Error>> {
let rec = rerun::RecordingStreamBuilder::new("rerun_example_image").spawn()?;
let mut image = Array::<u8, _>::zeros((200, 300, 3).f());
image.slice_mut(s![.., .., 0]).fill(255);
image.slice_mut(s![50..150, 50..150, 0]).fill(0);
image.slice_mut(s![50..150, 50..150, 1]).fill(255);
rec.log(
"image",
&rerun::Image::from_color_model_and_tensor(rerun::ColorModel::RGB, image)?,
)?;
Ok(())
}
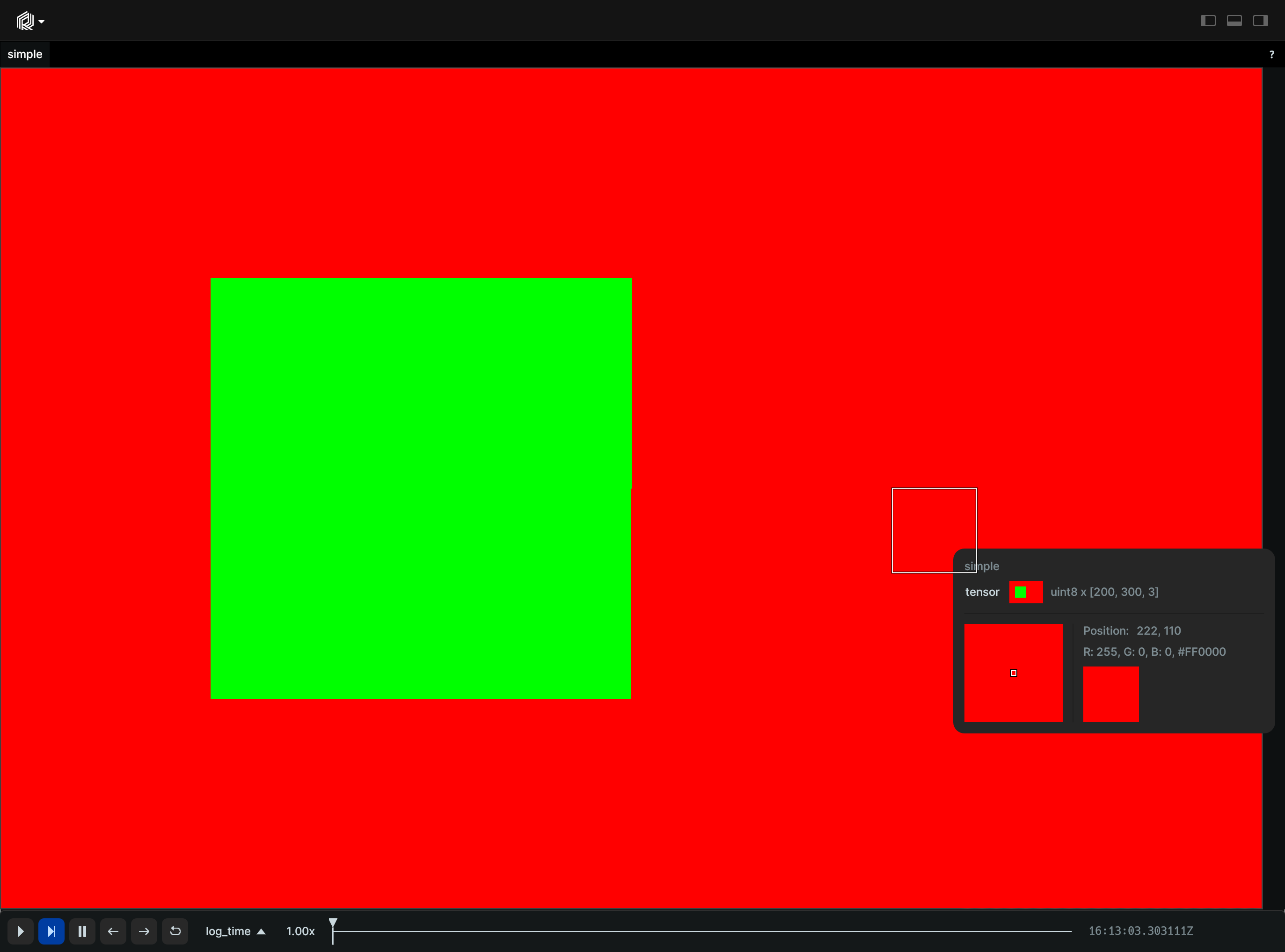
§Logging images with various formats
use rerun::external::ndarray;
fn main() -> Result<(), Box<dyn std::error::Error>> {
let rec = rerun::RecordingStreamBuilder::new("rerun_example_image_formats").spawn()?;
// Simple gradient image
let image = ndarray::Array3::from_shape_fn((256, 256, 3), |(y, x, c)| match c {
0 => x as u8,
1 => (x + y).min(255) as u8,
2 => y as u8,
_ => unreachable!(),
});
// RGB image
rec.log(
"image_rgb",
&rerun::Image::from_color_model_and_tensor(rerun::ColorModel::RGB, image.clone())?,
)?;
// Green channel only (Luminance)
rec.log(
"image_green_only",
&rerun::Image::from_color_model_and_tensor(
rerun::ColorModel::L,
image.slice(ndarray::s![.., .., 1]).to_owned(),
)?,
)?;
// BGR image
rec.log(
"image_bgr",
&rerun::Image::from_color_model_and_tensor(
rerun::ColorModel::BGR,
image.slice(ndarray::s![.., .., ..;-1]).to_owned(),
)?,
)?;
// New image with Separate Y/U/V planes with 4:2:2 chroma downsampling
let mut yuv_bytes = Vec::with_capacity(256 * 256 + 128 * 256 * 2);
yuv_bytes.extend(std::iter::repeat(128).take(256 * 256)); // Fixed value for Y.
yuv_bytes.extend((0..256).flat_map(|_y| (0..128).map(|x| x * 2))); // Gradient for U.
yuv_bytes.extend((0..256).flat_map(|y| std::iter::repeat(y as u8).take(128))); // Gradient for V.
rec.log(
"image_yuv422",
&rerun::Image::from_pixel_format(
[256, 256],
rerun::PixelFormat::Y_U_V16_FullRange,
yuv_bytes,
),
)?;
Ok(())
}
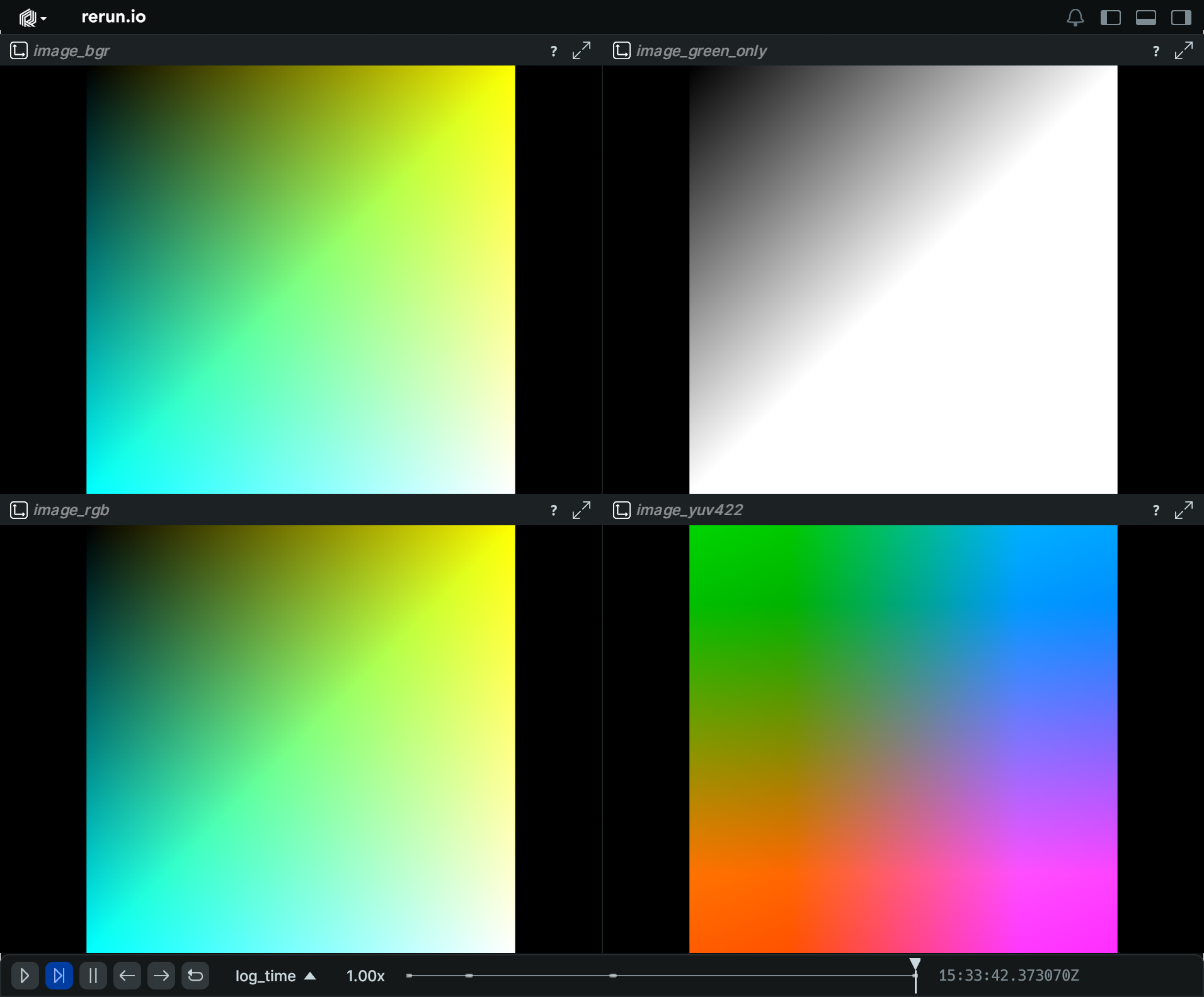
Fields§
§buffer: Option<SerializedComponentBatch>
The raw image data.
format: Option<SerializedComponentBatch>
The format of the image.
opacity: Option<SerializedComponentBatch>
Opacity of the image, useful for layering several images.
Defaults to 1.0 (fully opaque).
draw_order: Option<SerializedComponentBatch>
An optional floating point value that specifies the 2D drawing order.
Objects with higher values are drawn on top of those with lower values.
Implementations§
source§impl Image
impl Image
sourcepub fn descriptor_buffer() -> ComponentDescriptor
pub fn descriptor_buffer() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::buffer
.
sourcepub fn descriptor_format() -> ComponentDescriptor
pub fn descriptor_format() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::format
.
sourcepub fn descriptor_opacity() -> ComponentDescriptor
pub fn descriptor_opacity() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::opacity
.
sourcepub fn descriptor_draw_order() -> ComponentDescriptor
pub fn descriptor_draw_order() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::draw_order
.
sourcepub fn descriptor_indicator() -> ComponentDescriptor
pub fn descriptor_indicator() -> ComponentDescriptor
Returns the ComponentDescriptor
for the associated indicator component.
source§impl Image
impl Image
sourcepub const NUM_COMPONENTS: usize = 5usize
pub const NUM_COMPONENTS: usize = 5usize
The total number of components in the archetype: 2 required, 1 recommended, 2 optional
source§impl Image
impl Image
sourcepub fn new(
buffer: impl Into<ImageBuffer>,
format: impl Into<ImageFormat>,
) -> Image
pub fn new( buffer: impl Into<ImageBuffer>, format: impl Into<ImageFormat>, ) -> Image
Create a new Image
.
sourcepub fn update_fields() -> Image
pub fn update_fields() -> Image
Update only some specific fields of a Image
.
sourcepub fn clear_fields() -> Image
pub fn clear_fields() -> Image
Clear all the fields of a Image
.
sourcepub fn columns<I>(
self,
_lengths: I,
) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
pub fn columns<I>( self, _lengths: I, ) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
Partitions the component data into multiple sub-batches.
Specifically, this transforms the existing SerializedComponentBatch
es data into SerializedComponentColumn
s
instead, via SerializedComponentBatch::partitioned
.
This makes it possible to use RecordingStream::send_columns
to send columnar data directly into Rerun.
The specified lengths
must sum to the total length of the component batch.
sourcepub fn columns_of_unit_batches(
self,
) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
pub fn columns_of_unit_batches( self, ) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
Helper to partition the component data into unit-length sub-batches.
This is semantically similar to calling Self::columns
with std::iter::take(1).repeat(n)
,
where n
is automatically guessed.
sourcepub fn with_buffer(self, buffer: impl Into<ImageBuffer>) -> Image
pub fn with_buffer(self, buffer: impl Into<ImageBuffer>) -> Image
The raw image data.
sourcepub fn with_many_buffer(
self,
buffer: impl IntoIterator<Item = impl Into<ImageBuffer>>,
) -> Image
pub fn with_many_buffer( self, buffer: impl IntoIterator<Item = impl Into<ImageBuffer>>, ) -> Image
This method makes it possible to pack multiple crate::components::ImageBuffer
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_buffer
should
be used when logging a single row’s worth of data.
sourcepub fn with_format(self, format: impl Into<ImageFormat>) -> Image
pub fn with_format(self, format: impl Into<ImageFormat>) -> Image
The format of the image.
sourcepub fn with_many_format(
self,
format: impl IntoIterator<Item = impl Into<ImageFormat>>,
) -> Image
pub fn with_many_format( self, format: impl IntoIterator<Item = impl Into<ImageFormat>>, ) -> Image
This method makes it possible to pack multiple crate::components::ImageFormat
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_format
should
be used when logging a single row’s worth of data.
sourcepub fn with_opacity(self, opacity: impl Into<Opacity>) -> Image
pub fn with_opacity(self, opacity: impl Into<Opacity>) -> Image
Opacity of the image, useful for layering several images.
Defaults to 1.0 (fully opaque).
sourcepub fn with_many_opacity(
self,
opacity: impl IntoIterator<Item = impl Into<Opacity>>,
) -> Image
pub fn with_many_opacity( self, opacity: impl IntoIterator<Item = impl Into<Opacity>>, ) -> Image
This method makes it possible to pack multiple crate::components::Opacity
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_opacity
should
be used when logging a single row’s worth of data.
sourcepub fn with_draw_order(self, draw_order: impl Into<DrawOrder>) -> Image
pub fn with_draw_order(self, draw_order: impl Into<DrawOrder>) -> Image
An optional floating point value that specifies the 2D drawing order.
Objects with higher values are drawn on top of those with lower values.
sourcepub fn with_many_draw_order(
self,
draw_order: impl IntoIterator<Item = impl Into<DrawOrder>>,
) -> Image
pub fn with_many_draw_order( self, draw_order: impl IntoIterator<Item = impl Into<DrawOrder>>, ) -> Image
This method makes it possible to pack multiple crate::components::DrawOrder
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_draw_order
should
be used when logging a single row’s worth of data.
source§impl Image
impl Image
sourcepub fn from_color_model_and_tensor<T>(
color_model: ColorModel,
data: T,
) -> Result<Image, ImageConstructionError<T>>
pub fn from_color_model_and_tensor<T>( color_model: ColorModel, data: T, ) -> Result<Image, ImageConstructionError<T>>
Try to construct an Image
from a color model (L, RGB, RGBA, …) and anything that can be converted into TensorData
.
Will return an ImageConstructionError
if the shape of the tensor data does not match the given color model.
This is useful for constructing an Image
from an ndarray.
See also Self::from_pixel_format
.
sourcepub fn from_pixel_format(
_: [u32; 2],
pixel_format: PixelFormat,
bytes: impl Into<ImageBuffer>,
) -> Image
pub fn from_pixel_format( _: [u32; 2], pixel_format: PixelFormat, bytes: impl Into<ImageBuffer>, ) -> Image
Construct an image from a byte buffer given its resolution and pixel format.
See also Self::from_color_model_and_tensor
.
sourcepub fn from_color_model_and_bytes(
bytes: impl Into<ImageBuffer>,
_: [u32; 2],
color_model: ColorModel,
datatype: ChannelDatatype,
) -> Image
pub fn from_color_model_and_bytes( bytes: impl Into<ImageBuffer>, _: [u32; 2], color_model: ColorModel, datatype: ChannelDatatype, ) -> Image
Construct an image from a byte buffer given its resolution, color model, and data type.
See also Self::from_color_model_and_tensor
.
sourcepub fn from_elements<T>(
elements: &[T],
_: [u32; 2],
color_model: ColorModel,
) -> Imagewhere
T: ImageChannelType,
pub fn from_elements<T>(
elements: &[T],
_: [u32; 2],
color_model: ColorModel,
) -> Imagewhere
T: ImageChannelType,
Construct an image from a byte buffer given its resolution, color model, and using the data type of the given vector.
sourcepub fn from_l8(bytes: impl Into<ImageBuffer>, resolution: [u32; 2]) -> Image
pub fn from_l8(bytes: impl Into<ImageBuffer>, resolution: [u32; 2]) -> Image
From an 8-bit grayscale image.
sourcepub fn from_rgb24(bytes: impl Into<ImageBuffer>, resolution: [u32; 2]) -> Image
pub fn from_rgb24(bytes: impl Into<ImageBuffer>, resolution: [u32; 2]) -> Image
Assumes RGB, 8-bit per channel, interleaved as RGBRGBRGB
.
sourcepub fn from_rgba32(bytes: impl Into<ImageBuffer>, resolution: [u32; 2]) -> Image
pub fn from_rgba32(bytes: impl Into<ImageBuffer>, resolution: [u32; 2]) -> Image
Assumes RGBA, 8-bit per channel, with separate alpha.
sourcepub fn from_file_path(filepath: impl AsRef<Path>) -> Result<EncodedImage, Error>
👎Deprecated: Use EncodedImage::from_file instead
pub fn from_file_path(filepath: impl AsRef<Path>) -> Result<EncodedImage, Error>
Creates a new Image
from a file.
The image format will be inferred from the path (extension), or the contents if that fails.
sourcepub fn from_file_contents(
contents: Vec<u8>,
_format: Option<ImageFormat>,
) -> EncodedImage
👎Deprecated: Use EncodedImage::from_file_contents instead
pub fn from_file_contents( contents: Vec<u8>, _format: Option<ImageFormat>, ) -> EncodedImage
Creates a new Image
from the contents of a file.
If unspecified, the image format will be inferred from the contents.
source§impl Image
impl Image
sourcepub fn from_image_bytes(
format: ImageFormat,
file_contents: &[u8],
) -> Result<Image, ImageLoadError>
pub fn from_image_bytes( format: ImageFormat, file_contents: &[u8], ) -> Result<Image, ImageLoadError>
Construct an image from the contents of an image file.
This will spend CPU cycles decoding the image.
To save CPU time and storage, we recommend you instead use
EncodedImage::from_file_contents
.
Requires the image
feature.
sourcepub fn from_image(
image: impl Into<DynamicImage>,
) -> Result<Image, ImageConversionError>
pub fn from_image( image: impl Into<DynamicImage>, ) -> Result<Image, ImageConversionError>
Construct an image from something that can be turned into a image::DynamicImage
.
Requires the image
feature.
sourcepub fn from_dynamic_image(
image: DynamicImage,
) -> Result<Image, ImageConversionError>
pub fn from_dynamic_image( image: DynamicImage, ) -> Result<Image, ImageConversionError>
Construct an image from image::DynamicImage
.
Requires the image
feature.
Trait Implementations§
source§impl Archetype for Image
impl Archetype for Image
§type Indicator = GenericIndicatorComponent<Image>
type Indicator = GenericIndicatorComponent<Image>
source§fn name() -> ArchetypeName
fn name() -> ArchetypeName
rerun.archetypes.Points2D
.source§fn display_name() -> &'static str
fn display_name() -> &'static str
source§fn required_components() -> Cow<'static, [ComponentDescriptor]>
fn required_components() -> Cow<'static, [ComponentDescriptor]>
source§fn recommended_components() -> Cow<'static, [ComponentDescriptor]>
fn recommended_components() -> Cow<'static, [ComponentDescriptor]>
source§fn optional_components() -> Cow<'static, [ComponentDescriptor]>
fn optional_components() -> Cow<'static, [ComponentDescriptor]>
source§fn all_components() -> Cow<'static, [ComponentDescriptor]>
fn all_components() -> Cow<'static, [ComponentDescriptor]>
source§fn from_arrow_components(
arrow_data: impl IntoIterator<Item = (ComponentDescriptor, Arc<dyn Array>)>,
) -> Result<Image, DeserializationError>
fn from_arrow_components( arrow_data: impl IntoIterator<Item = (ComponentDescriptor, Arc<dyn Array>)>, ) -> Result<Image, DeserializationError>
ComponentNames
, deserializes them
into this archetype. Read moresource§fn from_arrow(
data: impl IntoIterator<Item = (Field, Arc<dyn Array>)>,
) -> Result<Self, DeserializationError>where
Self: Sized,
fn from_arrow(
data: impl IntoIterator<Item = (Field, Arc<dyn Array>)>,
) -> Result<Self, DeserializationError>where
Self: Sized,
source§impl AsComponents for Image
impl AsComponents for Image
source§fn as_serialized_batches(&self) -> Vec<SerializedComponentBatch>
fn as_serialized_batches(&self) -> Vec<SerializedComponentBatch>
SerializedComponentBatch
es. Read moresource§impl PartialEq for Image
impl PartialEq for Image
source§impl SizeBytes for Image
impl SizeBytes for Image
source§fn heap_size_bytes(&self) -> u64
fn heap_size_bytes(&self) -> u64
self
uses on the heap. Read moresource§fn total_size_bytes(&self) -> u64
fn total_size_bytes(&self) -> u64
self
in bytes, accounting for both stack and heap space.source§fn stack_size_bytes(&self) -> u64
fn stack_size_bytes(&self) -> u64
self
on the stack, in bytes. Read moreimpl ArchetypeReflectionMarker for Image
impl StructuralPartialEq for Image
Auto Trait Implementations§
impl Freeze for Image
impl !RefUnwindSafe for Image
impl Send for Image
impl Sync for Image
impl Unpin for Image
impl !UnwindSafe for Image
Blanket Implementations§
source§impl<T> BorrowMut<T> for Twhere
T: ?Sized,
impl<T> BorrowMut<T> for Twhere
T: ?Sized,
source§fn borrow_mut(&mut self) -> &mut T
fn borrow_mut(&mut self) -> &mut T
source§impl<T> CheckedAs for T
impl<T> CheckedAs for T
source§fn checked_as<Dst>(self) -> Option<Dst>where
T: CheckedCast<Dst>,
fn checked_as<Dst>(self) -> Option<Dst>where
T: CheckedCast<Dst>,
source§impl<Src, Dst> CheckedCastFrom<Src> for Dstwhere
Src: CheckedCast<Dst>,
impl<Src, Dst> CheckedCastFrom<Src> for Dstwhere
Src: CheckedCast<Dst>,
source§fn checked_cast_from(src: Src) -> Option<Dst>
fn checked_cast_from(src: Src) -> Option<Dst>
source§impl<T> CloneToUninit for Twhere
T: Clone,
impl<T> CloneToUninit for Twhere
T: Clone,
source§default unsafe fn clone_to_uninit(&self, dst: *mut T)
default unsafe fn clone_to_uninit(&self, dst: *mut T)
clone_to_uninit
)§impl<T> Conv for T
impl<T> Conv for T
§impl<T> Downcast for Twhere
T: Any,
impl<T> Downcast for Twhere
T: Any,
§fn into_any(self: Box<T>) -> Box<dyn Any>
fn into_any(self: Box<T>) -> Box<dyn Any>
Box<dyn Trait>
(where Trait: Downcast
) to Box<dyn Any>
. Box<dyn Any>
can
then be further downcast
into Box<ConcreteType>
where ConcreteType
implements Trait
.§fn into_any_rc(self: Rc<T>) -> Rc<dyn Any>
fn into_any_rc(self: Rc<T>) -> Rc<dyn Any>
Rc<Trait>
(where Trait: Downcast
) to Rc<Any>
. Rc<Any>
can then be
further downcast
into Rc<ConcreteType>
where ConcreteType
implements Trait
.§fn as_any(&self) -> &(dyn Any + 'static)
fn as_any(&self) -> &(dyn Any + 'static)
&Trait
(where Trait: Downcast
) to &Any
. This is needed since Rust cannot
generate &Any
’s vtable from &Trait
’s.§fn as_any_mut(&mut self) -> &mut (dyn Any + 'static)
fn as_any_mut(&mut self) -> &mut (dyn Any + 'static)
&mut Trait
(where Trait: Downcast
) to &Any
. This is needed since Rust cannot
generate &mut Any
’s vtable from &mut Trait
’s.§impl<T> DowncastSync for T
impl<T> DowncastSync for T
§impl<T> Instrument for T
impl<T> Instrument for T
§fn instrument(self, span: Span) -> Instrumented<Self>
fn instrument(self, span: Span) -> Instrumented<Self>
§fn in_current_span(self) -> Instrumented<Self>
fn in_current_span(self) -> Instrumented<Self>
source§impl<T> IntoEither for T
impl<T> IntoEither for T
source§fn into_either(self, into_left: bool) -> Either<Self, Self>
fn into_either(self, into_left: bool) -> Either<Self, Self>
self
into a Left
variant of Either<Self, Self>
if into_left
is true
.
Converts self
into a Right
variant of Either<Self, Self>
otherwise. Read moresource§fn into_either_with<F>(self, into_left: F) -> Either<Self, Self>
fn into_either_with<F>(self, into_left: F) -> Either<Self, Self>
self
into a Left
variant of Either<Self, Self>
if into_left(&self)
returns true
.
Converts self
into a Right
variant of Either<Self, Self>
otherwise. Read moresource§impl<T> IntoRequest<T> for T
impl<T> IntoRequest<T> for T
source§fn into_request(self) -> Request<T>
fn into_request(self) -> Request<T>
T
in a tonic::Request
source§impl<Src, Dst> LosslessTryInto<Dst> for Srcwhere
Dst: LosslessTryFrom<Src>,
impl<Src, Dst> LosslessTryInto<Dst> for Srcwhere
Dst: LosslessTryFrom<Src>,
source§fn lossless_try_into(self) -> Option<Dst>
fn lossless_try_into(self) -> Option<Dst>
source§impl<Src, Dst> LossyInto<Dst> for Srcwhere
Dst: LossyFrom<Src>,
impl<Src, Dst> LossyInto<Dst> for Srcwhere
Dst: LossyFrom<Src>,
source§fn lossy_into(self) -> Dst
fn lossy_into(self) -> Dst
§impl<T> NoneValue for Twhere
T: Default,
impl<T> NoneValue for Twhere
T: Default,
type NoneType = T
§fn null_value() -> T
fn null_value() -> T
source§impl<T> OverflowingAs for T
impl<T> OverflowingAs for T
source§fn overflowing_as<Dst>(self) -> (Dst, bool)where
T: OverflowingCast<Dst>,
fn overflowing_as<Dst>(self) -> (Dst, bool)where
T: OverflowingCast<Dst>,
source§impl<Src, Dst> OverflowingCastFrom<Src> for Dstwhere
Src: OverflowingCast<Dst>,
impl<Src, Dst> OverflowingCastFrom<Src> for Dstwhere
Src: OverflowingCast<Dst>,
source§fn overflowing_cast_from(src: Src) -> (Dst, bool)
fn overflowing_cast_from(src: Src) -> (Dst, bool)
§impl<T> Pipe for Twhere
T: ?Sized,
impl<T> Pipe for Twhere
T: ?Sized,
§fn pipe<R>(self, func: impl FnOnce(Self) -> R) -> Rwhere
Self: Sized,
fn pipe<R>(self, func: impl FnOnce(Self) -> R) -> Rwhere
Self: Sized,
§fn pipe_ref<'a, R>(&'a self, func: impl FnOnce(&'a Self) -> R) -> Rwhere
R: 'a,
fn pipe_ref<'a, R>(&'a self, func: impl FnOnce(&'a Self) -> R) -> Rwhere
R: 'a,
self
and passes that borrow into the pipe function. Read more§fn pipe_ref_mut<'a, R>(&'a mut self, func: impl FnOnce(&'a mut Self) -> R) -> Rwhere
R: 'a,
fn pipe_ref_mut<'a, R>(&'a mut self, func: impl FnOnce(&'a mut Self) -> R) -> Rwhere
R: 'a,
self
and passes that borrow into the pipe function. Read more§fn pipe_borrow<'a, B, R>(&'a self, func: impl FnOnce(&'a B) -> R) -> R
fn pipe_borrow<'a, B, R>(&'a self, func: impl FnOnce(&'a B) -> R) -> R
§fn pipe_borrow_mut<'a, B, R>(
&'a mut self,
func: impl FnOnce(&'a mut B) -> R,
) -> R
fn pipe_borrow_mut<'a, B, R>( &'a mut self, func: impl FnOnce(&'a mut B) -> R, ) -> R
§fn pipe_as_ref<'a, U, R>(&'a self, func: impl FnOnce(&'a U) -> R) -> R
fn pipe_as_ref<'a, U, R>(&'a self, func: impl FnOnce(&'a U) -> R) -> R
self
, then passes self.as_ref()
into the pipe function.§fn pipe_as_mut<'a, U, R>(&'a mut self, func: impl FnOnce(&'a mut U) -> R) -> R
fn pipe_as_mut<'a, U, R>(&'a mut self, func: impl FnOnce(&'a mut U) -> R) -> R
self
, then passes self.as_mut()
into the pipe
function.§fn pipe_deref<'a, T, R>(&'a self, func: impl FnOnce(&'a T) -> R) -> R
fn pipe_deref<'a, T, R>(&'a self, func: impl FnOnce(&'a T) -> R) -> R
self
, then passes self.deref()
into the pipe function.§impl<T> Pointable for T
impl<T> Pointable for T
source§impl<T> SaturatingAs for T
impl<T> SaturatingAs for T
source§fn saturating_as<Dst>(self) -> Dstwhere
T: SaturatingCast<Dst>,
fn saturating_as<Dst>(self) -> Dstwhere
T: SaturatingCast<Dst>,
source§impl<Src, Dst> SaturatingCastFrom<Src> for Dstwhere
Src: SaturatingCast<Dst>,
impl<Src, Dst> SaturatingCastFrom<Src> for Dstwhere
Src: SaturatingCast<Dst>,
source§fn saturating_cast_from(src: Src) -> Dst
fn saturating_cast_from(src: Src) -> Dst
§impl<T> Tap for T
impl<T> Tap for T
§fn tap_borrow<B>(self, func: impl FnOnce(&B)) -> Self
fn tap_borrow<B>(self, func: impl FnOnce(&B)) -> Self
Borrow<B>
of a value. Read more§fn tap_borrow_mut<B>(self, func: impl FnOnce(&mut B)) -> Self
fn tap_borrow_mut<B>(self, func: impl FnOnce(&mut B)) -> Self
BorrowMut<B>
of a value. Read more§fn tap_ref<R>(self, func: impl FnOnce(&R)) -> Self
fn tap_ref<R>(self, func: impl FnOnce(&R)) -> Self
AsRef<R>
view of a value. Read more§fn tap_ref_mut<R>(self, func: impl FnOnce(&mut R)) -> Self
fn tap_ref_mut<R>(self, func: impl FnOnce(&mut R)) -> Self
AsMut<R>
view of a value. Read more§fn tap_deref<T>(self, func: impl FnOnce(&T)) -> Self
fn tap_deref<T>(self, func: impl FnOnce(&T)) -> Self
Deref::Target
of a value. Read more§fn tap_deref_mut<T>(self, func: impl FnOnce(&mut T)) -> Self
fn tap_deref_mut<T>(self, func: impl FnOnce(&mut T)) -> Self
Deref::Target
of a value. Read more§fn tap_dbg(self, func: impl FnOnce(&Self)) -> Self
fn tap_dbg(self, func: impl FnOnce(&Self)) -> Self
.tap()
only in debug builds, and is erased in release builds.§fn tap_mut_dbg(self, func: impl FnOnce(&mut Self)) -> Self
fn tap_mut_dbg(self, func: impl FnOnce(&mut Self)) -> Self
.tap_mut()
only in debug builds, and is erased in release
builds.§fn tap_borrow_dbg<B>(self, func: impl FnOnce(&B)) -> Self
fn tap_borrow_dbg<B>(self, func: impl FnOnce(&B)) -> Self
.tap_borrow()
only in debug builds, and is erased in release
builds.§fn tap_borrow_mut_dbg<B>(self, func: impl FnOnce(&mut B)) -> Self
fn tap_borrow_mut_dbg<B>(self, func: impl FnOnce(&mut B)) -> Self
.tap_borrow_mut()
only in debug builds, and is erased in release
builds.§fn tap_ref_dbg<R>(self, func: impl FnOnce(&R)) -> Self
fn tap_ref_dbg<R>(self, func: impl FnOnce(&R)) -> Self
.tap_ref()
only in debug builds, and is erased in release
builds.§fn tap_ref_mut_dbg<R>(self, func: impl FnOnce(&mut R)) -> Self
fn tap_ref_mut_dbg<R>(self, func: impl FnOnce(&mut R)) -> Self
.tap_ref_mut()
only in debug builds, and is erased in release
builds.§fn tap_deref_dbg<T>(self, func: impl FnOnce(&T)) -> Self
fn tap_deref_dbg<T>(self, func: impl FnOnce(&T)) -> Self
.tap_deref()
only in debug builds, and is erased in release
builds.