Struct rerun::sdk::archetypes::Pinhole
source · pub struct Pinhole {
pub image_from_camera: Option<SerializedComponentBatch>,
pub resolution: Option<SerializedComponentBatch>,
pub camera_xyz: Option<SerializedComponentBatch>,
pub image_plane_distance: Option<SerializedComponentBatch>,
}
Expand description
Archetype: Camera perspective projection (a.k.a. intrinsics).
§Examples
§Simple pinhole camera
use ndarray::{Array, ShapeBuilder};
fn main() -> Result<(), Box<dyn std::error::Error>> {
let rec = rerun::RecordingStreamBuilder::new("rerun_example_pinhole").spawn()?;
let mut image = Array::<u8, _>::default((3, 3, 3).f());
image.map_inplace(|x| *x = rand::random());
rec.log(
"world/image",
&rerun::Pinhole::from_focal_length_and_resolution([3., 3.], [3., 3.]),
)?;
rec.log(
"world/image",
&rerun::Image::from_color_model_and_tensor(rerun::ColorModel::RGB, image)?,
)?;
Ok(())
}
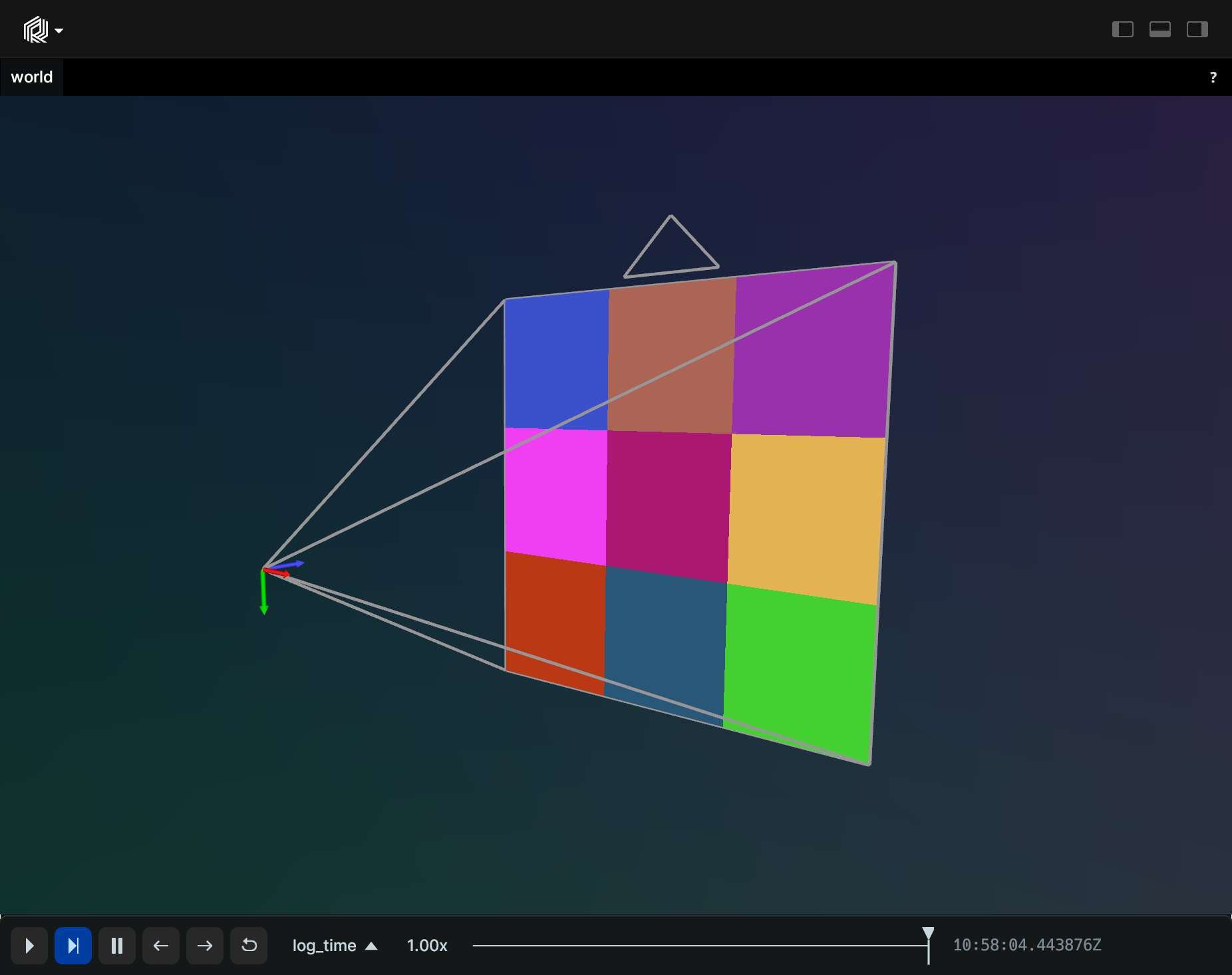
§Perspective pinhole camera
fn main() -> Result<(), Box<dyn std::error::Error>> {
let rec = rerun::RecordingStreamBuilder::new("rerun_example_pinhole_perspective").spawn()?;
let fov_y = std::f32::consts::FRAC_PI_4;
let aspect_ratio = 1.7777778;
rec.log(
"world/cam",
&rerun::Pinhole::from_fov_and_aspect_ratio(fov_y, aspect_ratio)
.with_camera_xyz(rerun::components::ViewCoordinates::RUB)
.with_image_plane_distance(0.1),
)?;
rec.log(
"world/points",
&rerun::Points3D::new([(0.0, 0.0, -0.5), (0.1, 0.1, -0.5), (-0.1, -0.1, -0.5)])
.with_radii([0.025]),
)?;
Ok(())
}
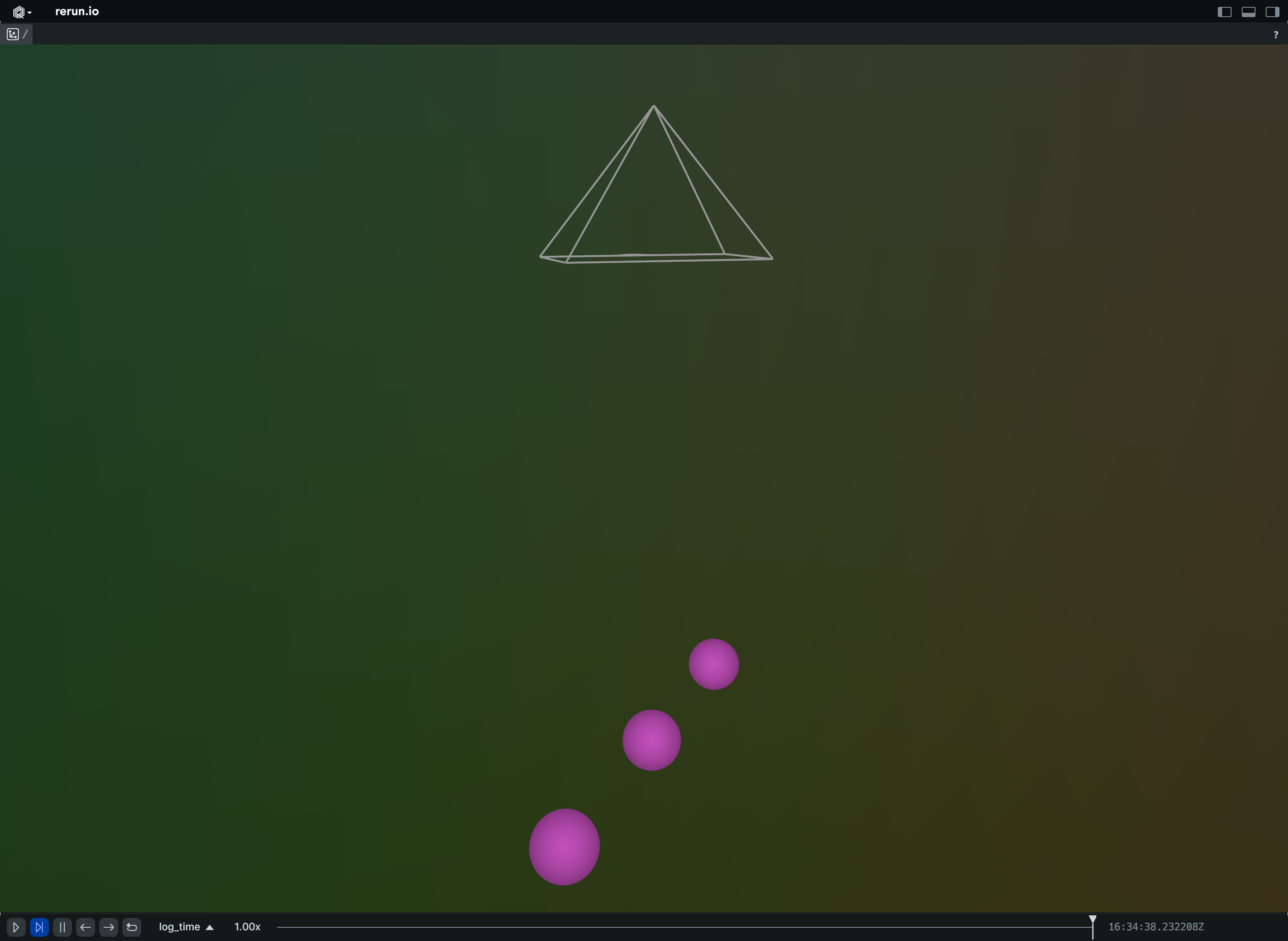
Fields§
§image_from_camera: Option<SerializedComponentBatch>
Camera projection, from image coordinates to view coordinates.
resolution: Option<SerializedComponentBatch>
Pixel resolution (usually integers) of child image space. Width and height.
Example:
[1920.0, 1440.0]
image_from_camera
project onto the space spanned by (0,0)
and resolution - 1
.
camera_xyz: Option<SerializedComponentBatch>
Sets the view coordinates for the camera.
All common values are available as constants on the components::ViewCoordinates
class.
The default is ViewCoordinates::RDF
, i.e. X=Right, Y=Down, Z=Forward, and this is also the recommended setting.
This means that the camera frustum will point along the positive Z axis of the parent space,
and the cameras “up” direction will be along the negative Y axis of the parent space.
The camera frustum will point whichever axis is set to F
(or the opposite of B
).
When logging a depth image under this entity, this is the direction the point cloud will be projected.
With RDF
, the default forward is +Z.
The frustum’s “up” direction will be whichever axis is set to U
(or the opposite of D
).
This will match the negative Y direction of pixel space (all images are assumed to have xyz=RDF).
With RDF
, the default is up is -Y.
The frustum’s “right” direction will be whichever axis is set to R
(or the opposite of L
).
This will match the positive X direction of pixel space (all images are assumed to have xyz=RDF).
With RDF
, the default right is +x.
Other common formats are RUB
(X=Right, Y=Up, Z=Back) and FLU
(X=Forward, Y=Left, Z=Up).
NOTE: setting this to something else than RDF
(the default) will change the orientation of the camera frustum,
and make the pinhole matrix not match up with the coordinate system of the pinhole entity.
The pinhole matrix (the image_from_camera
argument) always project along the third (Z) axis,
but will be re-oriented to project along the forward axis of the camera_xyz
argument.
image_plane_distance: Option<SerializedComponentBatch>
The distance from the camera origin to the image plane when the projection is shown in a 3D viewer.
This is only used for visualization purposes, and does not affect the projection itself.
Implementations§
source§impl Pinhole
impl Pinhole
sourcepub fn descriptor_image_from_camera() -> ComponentDescriptor
pub fn descriptor_image_from_camera() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::image_from_camera
.
sourcepub fn descriptor_resolution() -> ComponentDescriptor
pub fn descriptor_resolution() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::resolution
.
sourcepub fn descriptor_camera_xyz() -> ComponentDescriptor
pub fn descriptor_camera_xyz() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::camera_xyz
.
sourcepub fn descriptor_image_plane_distance() -> ComponentDescriptor
pub fn descriptor_image_plane_distance() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::image_plane_distance
.
sourcepub fn descriptor_indicator() -> ComponentDescriptor
pub fn descriptor_indicator() -> ComponentDescriptor
Returns the ComponentDescriptor
for the associated indicator component.
source§impl Pinhole
impl Pinhole
sourcepub const NUM_COMPONENTS: usize = 5usize
pub const NUM_COMPONENTS: usize = 5usize
The total number of components in the archetype: 1 required, 2 recommended, 2 optional
source§impl Pinhole
impl Pinhole
sourcepub fn new(image_from_camera: impl Into<PinholeProjection>) -> Pinhole
pub fn new(image_from_camera: impl Into<PinholeProjection>) -> Pinhole
Create a new Pinhole
.
sourcepub fn update_fields() -> Pinhole
pub fn update_fields() -> Pinhole
Update only some specific fields of a Pinhole
.
sourcepub fn clear_fields() -> Pinhole
pub fn clear_fields() -> Pinhole
Clear all the fields of a Pinhole
.
sourcepub fn columns<I>(
self,
_lengths: I,
) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
pub fn columns<I>( self, _lengths: I, ) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
Partitions the component data into multiple sub-batches.
Specifically, this transforms the existing SerializedComponentBatch
es data into SerializedComponentColumn
s
instead, via SerializedComponentBatch::partitioned
.
This makes it possible to use RecordingStream::send_columns
to send columnar data directly into Rerun.
The specified lengths
must sum to the total length of the component batch.
sourcepub fn columns_of_unit_batches(
self,
) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
pub fn columns_of_unit_batches( self, ) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
Helper to partition the component data into unit-length sub-batches.
This is semantically similar to calling Self::columns
with std::iter::take(1).repeat(n)
,
where n
is automatically guessed.
sourcepub fn with_image_from_camera(
self,
image_from_camera: impl Into<PinholeProjection>,
) -> Pinhole
pub fn with_image_from_camera( self, image_from_camera: impl Into<PinholeProjection>, ) -> Pinhole
Camera projection, from image coordinates to view coordinates.
sourcepub fn with_many_image_from_camera(
self,
image_from_camera: impl IntoIterator<Item = impl Into<PinholeProjection>>,
) -> Pinhole
pub fn with_many_image_from_camera( self, image_from_camera: impl IntoIterator<Item = impl Into<PinholeProjection>>, ) -> Pinhole
This method makes it possible to pack multiple crate::components::PinholeProjection
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_image_from_camera
should
be used when logging a single row’s worth of data.
sourcepub fn with_resolution(self, resolution: impl Into<Resolution>) -> Pinhole
pub fn with_resolution(self, resolution: impl Into<Resolution>) -> Pinhole
Pixel resolution (usually integers) of child image space. Width and height.
Example:
[1920.0, 1440.0]
image_from_camera
project onto the space spanned by (0,0)
and resolution - 1
.
sourcepub fn with_many_resolution(
self,
resolution: impl IntoIterator<Item = impl Into<Resolution>>,
) -> Pinhole
pub fn with_many_resolution( self, resolution: impl IntoIterator<Item = impl Into<Resolution>>, ) -> Pinhole
This method makes it possible to pack multiple crate::components::Resolution
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_resolution
should
be used when logging a single row’s worth of data.
sourcepub fn with_camera_xyz(self, camera_xyz: impl Into<ViewCoordinates>) -> Pinhole
pub fn with_camera_xyz(self, camera_xyz: impl Into<ViewCoordinates>) -> Pinhole
Sets the view coordinates for the camera.
All common values are available as constants on the components::ViewCoordinates
class.
The default is ViewCoordinates::RDF
, i.e. X=Right, Y=Down, Z=Forward, and this is also the recommended setting.
This means that the camera frustum will point along the positive Z axis of the parent space,
and the cameras “up” direction will be along the negative Y axis of the parent space.
The camera frustum will point whichever axis is set to F
(or the opposite of B
).
When logging a depth image under this entity, this is the direction the point cloud will be projected.
With RDF
, the default forward is +Z.
The frustum’s “up” direction will be whichever axis is set to U
(or the opposite of D
).
This will match the negative Y direction of pixel space (all images are assumed to have xyz=RDF).
With RDF
, the default is up is -Y.
The frustum’s “right” direction will be whichever axis is set to R
(or the opposite of L
).
This will match the positive X direction of pixel space (all images are assumed to have xyz=RDF).
With RDF
, the default right is +x.
Other common formats are RUB
(X=Right, Y=Up, Z=Back) and FLU
(X=Forward, Y=Left, Z=Up).
NOTE: setting this to something else than RDF
(the default) will change the orientation of the camera frustum,
and make the pinhole matrix not match up with the coordinate system of the pinhole entity.
The pinhole matrix (the image_from_camera
argument) always project along the third (Z) axis,
but will be re-oriented to project along the forward axis of the camera_xyz
argument.
sourcepub fn with_many_camera_xyz(
self,
camera_xyz: impl IntoIterator<Item = impl Into<ViewCoordinates>>,
) -> Pinhole
pub fn with_many_camera_xyz( self, camera_xyz: impl IntoIterator<Item = impl Into<ViewCoordinates>>, ) -> Pinhole
This method makes it possible to pack multiple crate::components::ViewCoordinates
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_camera_xyz
should
be used when logging a single row’s worth of data.
sourcepub fn with_image_plane_distance(
self,
image_plane_distance: impl Into<ImagePlaneDistance>,
) -> Pinhole
pub fn with_image_plane_distance( self, image_plane_distance: impl Into<ImagePlaneDistance>, ) -> Pinhole
The distance from the camera origin to the image plane when the projection is shown in a 3D viewer.
This is only used for visualization purposes, and does not affect the projection itself.
sourcepub fn with_many_image_plane_distance(
self,
image_plane_distance: impl IntoIterator<Item = impl Into<ImagePlaneDistance>>,
) -> Pinhole
pub fn with_many_image_plane_distance( self, image_plane_distance: impl IntoIterator<Item = impl Into<ImagePlaneDistance>>, ) -> Pinhole
This method makes it possible to pack multiple crate::components::ImagePlaneDistance
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_image_plane_distance
should
be used when logging a single row’s worth of data.
source§impl Pinhole
impl Pinhole
sourcepub const DEFAULT_CAMERA_XYZ: ViewCoordinates = ViewCoordinates::RDF
pub const DEFAULT_CAMERA_XYZ: ViewCoordinates = ViewCoordinates::RDF
Camera orientation used when there’s no camera orientation explicitly logged.
- x pointing right
- y pointing down
- z pointing into the image plane (this is convenient for reading out a depth image which has typically positive z values)
sourcepub fn from_focal_length_and_resolution(
focal_length: impl Into<Vec2D>,
resolution: impl Into<Vec2D>,
) -> Pinhole
pub fn from_focal_length_and_resolution( focal_length: impl Into<Vec2D>, resolution: impl Into<Vec2D>, ) -> Pinhole
Creates a pinhole from the camera focal length and resolution, both specified in pixels.
The focal length is the diagonal of the projection matrix. Set the same value for x & y value for symmetric cameras, or two values for anamorphic cameras.
Assumes the principal point to be in the middle of the sensor.
sourcepub fn from_fov_and_aspect_ratio(fov_y: f32, aspect_ratio: f32) -> Pinhole
pub fn from_fov_and_aspect_ratio(fov_y: f32, aspect_ratio: f32) -> Pinhole
Creates a pinhole from the camera vertical field of view (in radians) and aspect ratio (width/height).
Assumes the principal point to be in the middle of the sensor.
sourcepub fn with_principal_point(self, principal_point: impl Into<Vec2D>) -> Pinhole
pub fn with_principal_point(self, principal_point: impl Into<Vec2D>) -> Pinhole
Principal point of the pinhole camera, i.e. the intersection of the optical axis and the image plane.
see definition of intrinsic matrix
sourcepub fn fov_y(&self) -> Option<f32>
👎Deprecated since 0.22.0: Use Pinhole::image_from_camera_from_arrow
& Pinhole::resolution_from_arrow
to deserialize the components back,
or better use the components prior to passing it to the archetype, and then call PinholeProjection::fov_y
pub fn fov_y(&self) -> Option<f32>
Pinhole::image_from_camera_from_arrow
& Pinhole::resolution_from_arrow
to deserialize the components back,
or better use the components prior to passing it to the archetype, and then call PinholeProjection::fov_y
Field of View on the Y axis, i.e. the angle between top and bottom (in radians).
Only returns a result if both projection & resolution are set.
sourcepub fn resolution(&self) -> Option<Vec2>
👎Deprecated since 0.22.0: Use Pinhole::resolution_from_arrow
to deserialize back to a Resolution
component,
or better use the Resolution
prior to passing it to the archetype, and then use Resolution::into
for the conversion
pub fn resolution(&self) -> Option<Vec2>
Pinhole::resolution_from_arrow
to deserialize back to a Resolution
component,
or better use the Resolution
prior to passing it to the archetype, and then use Resolution::into
for the conversionThe resolution of the camera sensor in pixels.
sourcepub fn aspect_ratio(&self) -> Option<f32>
👎Deprecated since 0.22.0: Use Pinhole::resolution_from_arrow
to deserialize back to a Resolution
component,
or better use the Resolution
prior to passing it to the archetype, and then use Resolution::aspect_ratio
pub fn aspect_ratio(&self) -> Option<f32>
Pinhole::resolution_from_arrow
to deserialize back to a Resolution
component,
or better use the Resolution
prior to passing it to the archetype, and then use Resolution::aspect_ratio
Width/height ratio of the camera sensor.
sourcepub fn image_from_camera_from_arrow(
&self,
) -> Result<PinholeProjection, DeserializationError>
pub fn image_from_camera_from_arrow( &self, ) -> Result<PinholeProjection, DeserializationError>
Deserializes the pinhole projection from the image_from_camera
field.
Returns re_types_core::DeserializationError::MissingData
if the component is not present.
sourcepub fn resolution_from_arrow(&self) -> Result<Resolution, DeserializationError>
pub fn resolution_from_arrow(&self) -> Result<Resolution, DeserializationError>
Deserializes the resolution from the resolution
field.
Returns re_types_core::DeserializationError::MissingData
if the component is not present.
sourcepub fn focal_length_in_pixels(&self) -> Vec2D
👎Deprecated since 0.22.0: Use Pinhole::image_from_camera_from_arrow
instead to deserialize back to a PinholeProjection
component,
or better use the PinholeProjection
component prior to passing it to the archetype, and then call PinholeProjection::focal_length_in_pixels
pub fn focal_length_in_pixels(&self) -> Vec2D
Pinhole::image_from_camera_from_arrow
instead to deserialize back to a PinholeProjection
component,
or better use the PinholeProjection
component prior to passing it to the archetype, and then call PinholeProjection::focal_length_in_pixelsX & Y focal length in pixels.
sourcepub fn principal_point(&self) -> Vec2
👎Deprecated since 0.22.0: Use Pinhole::image_from_camera_from_arrow
instead to deserialize back to a PinholeProjection
component,
or better use the PinholeProjection
component prior to passing it to the archetype, and then call PinholeProjection::principal_point
pub fn principal_point(&self) -> Vec2
Pinhole::image_from_camera_from_arrow
instead to deserialize back to a PinholeProjection
component,
or better use the PinholeProjection
component prior to passing it to the archetype, and then call PinholeProjection::principal_pointPrincipal point of the pinhole camera, i.e. the intersection of the optical axis and the image plane.
sourcepub fn project(&self, pixel: Vec3) -> Vec3
👎Deprecated since 0.22.0: Use Pinhole::image_from_camera_from_arrow
instead to deserialize back to a PinholeProjection
component,
or better use the PinholeProjection
component prior to passing it to the archetype, and then call PinholeProjection::project
pub fn project(&self, pixel: Vec3) -> Vec3
Pinhole::image_from_camera_from_arrow
instead to deserialize back to a PinholeProjection
component,
or better use the PinholeProjection
component prior to passing it to the archetype, and then call PinholeProjection::projectProject camera-space coordinates into pixel coordinates, returning the same z/depth.
sourcepub fn unproject(&self, pixel: Vec3) -> Vec3
👎Deprecated since 0.22.0: Use Pinhole::image_from_camera_from_arrow
instead to deserialize back to a PinholeProjection
component,
or better use the PinholeProjection
component prior to passing it to the archetype, and then call PinholeProjection::unproject
pub fn unproject(&self, pixel: Vec3) -> Vec3
Pinhole::image_from_camera_from_arrow
instead to deserialize back to a PinholeProjection
component,
or better use the PinholeProjection
component prior to passing it to the archetype, and then call PinholeProjection::unprojectGiven pixel coordinates and a world-space depth, return a position in the camera space.
The returned z is the same as the input z (depth).
Trait Implementations§
source§impl Archetype for Pinhole
impl Archetype for Pinhole
§type Indicator = GenericIndicatorComponent<Pinhole>
type Indicator = GenericIndicatorComponent<Pinhole>
source§fn name() -> ArchetypeName
fn name() -> ArchetypeName
rerun.archetypes.Points2D
.source§fn display_name() -> &'static str
fn display_name() -> &'static str
source§fn required_components() -> Cow<'static, [ComponentDescriptor]>
fn required_components() -> Cow<'static, [ComponentDescriptor]>
source§fn recommended_components() -> Cow<'static, [ComponentDescriptor]>
fn recommended_components() -> Cow<'static, [ComponentDescriptor]>
source§fn optional_components() -> Cow<'static, [ComponentDescriptor]>
fn optional_components() -> Cow<'static, [ComponentDescriptor]>
source§fn all_components() -> Cow<'static, [ComponentDescriptor]>
fn all_components() -> Cow<'static, [ComponentDescriptor]>
source§fn from_arrow_components(
arrow_data: impl IntoIterator<Item = (ComponentDescriptor, Arc<dyn Array>)>,
) -> Result<Pinhole, DeserializationError>
fn from_arrow_components( arrow_data: impl IntoIterator<Item = (ComponentDescriptor, Arc<dyn Array>)>, ) -> Result<Pinhole, DeserializationError>
ComponentNames
, deserializes them
into this archetype. Read moresource§fn from_arrow(
data: impl IntoIterator<Item = (Field, Arc<dyn Array>)>,
) -> Result<Self, DeserializationError>where
Self: Sized,
fn from_arrow(
data: impl IntoIterator<Item = (Field, Arc<dyn Array>)>,
) -> Result<Self, DeserializationError>where
Self: Sized,
source§impl AsComponents for Pinhole
impl AsComponents for Pinhole
source§fn as_serialized_batches(&self) -> Vec<SerializedComponentBatch>
fn as_serialized_batches(&self) -> Vec<SerializedComponentBatch>
SerializedComponentBatch
es. Read moresource§impl PartialEq for Pinhole
impl PartialEq for Pinhole
source§impl SizeBytes for Pinhole
impl SizeBytes for Pinhole
source§fn heap_size_bytes(&self) -> u64
fn heap_size_bytes(&self) -> u64
self
uses on the heap. Read moresource§fn total_size_bytes(&self) -> u64
fn total_size_bytes(&self) -> u64
self
in bytes, accounting for both stack and heap space.source§fn stack_size_bytes(&self) -> u64
fn stack_size_bytes(&self) -> u64
self
on the stack, in bytes. Read moreimpl ArchetypeReflectionMarker for Pinhole
impl StructuralPartialEq for Pinhole
Auto Trait Implementations§
impl Freeze for Pinhole
impl !RefUnwindSafe for Pinhole
impl Send for Pinhole
impl Sync for Pinhole
impl Unpin for Pinhole
impl !UnwindSafe for Pinhole
Blanket Implementations§
source§impl<T> BorrowMut<T> for Twhere
T: ?Sized,
impl<T> BorrowMut<T> for Twhere
T: ?Sized,
source§fn borrow_mut(&mut self) -> &mut T
fn borrow_mut(&mut self) -> &mut T
source§impl<T> CheckedAs for T
impl<T> CheckedAs for T
source§fn checked_as<Dst>(self) -> Option<Dst>where
T: CheckedCast<Dst>,
fn checked_as<Dst>(self) -> Option<Dst>where
T: CheckedCast<Dst>,
source§impl<Src, Dst> CheckedCastFrom<Src> for Dstwhere
Src: CheckedCast<Dst>,
impl<Src, Dst> CheckedCastFrom<Src> for Dstwhere
Src: CheckedCast<Dst>,
source§fn checked_cast_from(src: Src) -> Option<Dst>
fn checked_cast_from(src: Src) -> Option<Dst>
source§impl<T> CloneToUninit for Twhere
T: Clone,
impl<T> CloneToUninit for Twhere
T: Clone,
source§default unsafe fn clone_to_uninit(&self, dst: *mut T)
default unsafe fn clone_to_uninit(&self, dst: *mut T)
clone_to_uninit
)§impl<T> Conv for T
impl<T> Conv for T
§impl<T> Downcast for Twhere
T: Any,
impl<T> Downcast for Twhere
T: Any,
§fn into_any(self: Box<T>) -> Box<dyn Any>
fn into_any(self: Box<T>) -> Box<dyn Any>
Box<dyn Trait>
(where Trait: Downcast
) to Box<dyn Any>
. Box<dyn Any>
can
then be further downcast
into Box<ConcreteType>
where ConcreteType
implements Trait
.§fn into_any_rc(self: Rc<T>) -> Rc<dyn Any>
fn into_any_rc(self: Rc<T>) -> Rc<dyn Any>
Rc<Trait>
(where Trait: Downcast
) to Rc<Any>
. Rc<Any>
can then be
further downcast
into Rc<ConcreteType>
where ConcreteType
implements Trait
.§fn as_any(&self) -> &(dyn Any + 'static)
fn as_any(&self) -> &(dyn Any + 'static)
&Trait
(where Trait: Downcast
) to &Any
. This is needed since Rust cannot
generate &Any
’s vtable from &Trait
’s.§fn as_any_mut(&mut self) -> &mut (dyn Any + 'static)
fn as_any_mut(&mut self) -> &mut (dyn Any + 'static)
&mut Trait
(where Trait: Downcast
) to &Any
. This is needed since Rust cannot
generate &mut Any
’s vtable from &mut Trait
’s.§impl<T> DowncastSync for T
impl<T> DowncastSync for T
§impl<T> Instrument for T
impl<T> Instrument for T
§fn instrument(self, span: Span) -> Instrumented<Self>
fn instrument(self, span: Span) -> Instrumented<Self>
§fn in_current_span(self) -> Instrumented<Self>
fn in_current_span(self) -> Instrumented<Self>
source§impl<T> IntoEither for T
impl<T> IntoEither for T
source§fn into_either(self, into_left: bool) -> Either<Self, Self>
fn into_either(self, into_left: bool) -> Either<Self, Self>
self
into a Left
variant of Either<Self, Self>
if into_left
is true
.
Converts self
into a Right
variant of Either<Self, Self>
otherwise. Read moresource§fn into_either_with<F>(self, into_left: F) -> Either<Self, Self>
fn into_either_with<F>(self, into_left: F) -> Either<Self, Self>
self
into a Left
variant of Either<Self, Self>
if into_left(&self)
returns true
.
Converts self
into a Right
variant of Either<Self, Self>
otherwise. Read moresource§impl<T> IntoRequest<T> for T
impl<T> IntoRequest<T> for T
source§fn into_request(self) -> Request<T>
fn into_request(self) -> Request<T>
T
in a tonic::Request
source§impl<Src, Dst> LosslessTryInto<Dst> for Srcwhere
Dst: LosslessTryFrom<Src>,
impl<Src, Dst> LosslessTryInto<Dst> for Srcwhere
Dst: LosslessTryFrom<Src>,
source§fn lossless_try_into(self) -> Option<Dst>
fn lossless_try_into(self) -> Option<Dst>
source§impl<Src, Dst> LossyInto<Dst> for Srcwhere
Dst: LossyFrom<Src>,
impl<Src, Dst> LossyInto<Dst> for Srcwhere
Dst: LossyFrom<Src>,
source§fn lossy_into(self) -> Dst
fn lossy_into(self) -> Dst
§impl<T> NoneValue for Twhere
T: Default,
impl<T> NoneValue for Twhere
T: Default,
type NoneType = T
§fn null_value() -> T
fn null_value() -> T
source§impl<T> OverflowingAs for T
impl<T> OverflowingAs for T
source§fn overflowing_as<Dst>(self) -> (Dst, bool)where
T: OverflowingCast<Dst>,
fn overflowing_as<Dst>(self) -> (Dst, bool)where
T: OverflowingCast<Dst>,
source§impl<Src, Dst> OverflowingCastFrom<Src> for Dstwhere
Src: OverflowingCast<Dst>,
impl<Src, Dst> OverflowingCastFrom<Src> for Dstwhere
Src: OverflowingCast<Dst>,
source§fn overflowing_cast_from(src: Src) -> (Dst, bool)
fn overflowing_cast_from(src: Src) -> (Dst, bool)
§impl<T> Pipe for Twhere
T: ?Sized,
impl<T> Pipe for Twhere
T: ?Sized,
§fn pipe<R>(self, func: impl FnOnce(Self) -> R) -> Rwhere
Self: Sized,
fn pipe<R>(self, func: impl FnOnce(Self) -> R) -> Rwhere
Self: Sized,
§fn pipe_ref<'a, R>(&'a self, func: impl FnOnce(&'a Self) -> R) -> Rwhere
R: 'a,
fn pipe_ref<'a, R>(&'a self, func: impl FnOnce(&'a Self) -> R) -> Rwhere
R: 'a,
self
and passes that borrow into the pipe function. Read more§fn pipe_ref_mut<'a, R>(&'a mut self, func: impl FnOnce(&'a mut Self) -> R) -> Rwhere
R: 'a,
fn pipe_ref_mut<'a, R>(&'a mut self, func: impl FnOnce(&'a mut Self) -> R) -> Rwhere
R: 'a,
self
and passes that borrow into the pipe function. Read more§fn pipe_borrow<'a, B, R>(&'a self, func: impl FnOnce(&'a B) -> R) -> R
fn pipe_borrow<'a, B, R>(&'a self, func: impl FnOnce(&'a B) -> R) -> R
§fn pipe_borrow_mut<'a, B, R>(
&'a mut self,
func: impl FnOnce(&'a mut B) -> R,
) -> R
fn pipe_borrow_mut<'a, B, R>( &'a mut self, func: impl FnOnce(&'a mut B) -> R, ) -> R
§fn pipe_as_ref<'a, U, R>(&'a self, func: impl FnOnce(&'a U) -> R) -> R
fn pipe_as_ref<'a, U, R>(&'a self, func: impl FnOnce(&'a U) -> R) -> R
self
, then passes self.as_ref()
into the pipe function.§fn pipe_as_mut<'a, U, R>(&'a mut self, func: impl FnOnce(&'a mut U) -> R) -> R
fn pipe_as_mut<'a, U, R>(&'a mut self, func: impl FnOnce(&'a mut U) -> R) -> R
self
, then passes self.as_mut()
into the pipe
function.§fn pipe_deref<'a, T, R>(&'a self, func: impl FnOnce(&'a T) -> R) -> R
fn pipe_deref<'a, T, R>(&'a self, func: impl FnOnce(&'a T) -> R) -> R
self
, then passes self.deref()
into the pipe function.§impl<T> Pointable for T
impl<T> Pointable for T
source§impl<T> SaturatingAs for T
impl<T> SaturatingAs for T
source§fn saturating_as<Dst>(self) -> Dstwhere
T: SaturatingCast<Dst>,
fn saturating_as<Dst>(self) -> Dstwhere
T: SaturatingCast<Dst>,
source§impl<Src, Dst> SaturatingCastFrom<Src> for Dstwhere
Src: SaturatingCast<Dst>,
impl<Src, Dst> SaturatingCastFrom<Src> for Dstwhere
Src: SaturatingCast<Dst>,
source§fn saturating_cast_from(src: Src) -> Dst
fn saturating_cast_from(src: Src) -> Dst
§impl<T> Tap for T
impl<T> Tap for T
§fn tap_borrow<B>(self, func: impl FnOnce(&B)) -> Self
fn tap_borrow<B>(self, func: impl FnOnce(&B)) -> Self
Borrow<B>
of a value. Read more§fn tap_borrow_mut<B>(self, func: impl FnOnce(&mut B)) -> Self
fn tap_borrow_mut<B>(self, func: impl FnOnce(&mut B)) -> Self
BorrowMut<B>
of a value. Read more§fn tap_ref<R>(self, func: impl FnOnce(&R)) -> Self
fn tap_ref<R>(self, func: impl FnOnce(&R)) -> Self
AsRef<R>
view of a value. Read more§fn tap_ref_mut<R>(self, func: impl FnOnce(&mut R)) -> Self
fn tap_ref_mut<R>(self, func: impl FnOnce(&mut R)) -> Self
AsMut<R>
view of a value. Read more§fn tap_deref<T>(self, func: impl FnOnce(&T)) -> Self
fn tap_deref<T>(self, func: impl FnOnce(&T)) -> Self
Deref::Target
of a value. Read more§fn tap_deref_mut<T>(self, func: impl FnOnce(&mut T)) -> Self
fn tap_deref_mut<T>(self, func: impl FnOnce(&mut T)) -> Self
Deref::Target
of a value. Read more§fn tap_dbg(self, func: impl FnOnce(&Self)) -> Self
fn tap_dbg(self, func: impl FnOnce(&Self)) -> Self
.tap()
only in debug builds, and is erased in release builds.§fn tap_mut_dbg(self, func: impl FnOnce(&mut Self)) -> Self
fn tap_mut_dbg(self, func: impl FnOnce(&mut Self)) -> Self
.tap_mut()
only in debug builds, and is erased in release
builds.§fn tap_borrow_dbg<B>(self, func: impl FnOnce(&B)) -> Self
fn tap_borrow_dbg<B>(self, func: impl FnOnce(&B)) -> Self
.tap_borrow()
only in debug builds, and is erased in release
builds.§fn tap_borrow_mut_dbg<B>(self, func: impl FnOnce(&mut B)) -> Self
fn tap_borrow_mut_dbg<B>(self, func: impl FnOnce(&mut B)) -> Self
.tap_borrow_mut()
only in debug builds, and is erased in release
builds.§fn tap_ref_dbg<R>(self, func: impl FnOnce(&R)) -> Self
fn tap_ref_dbg<R>(self, func: impl FnOnce(&R)) -> Self
.tap_ref()
only in debug builds, and is erased in release
builds.§fn tap_ref_mut_dbg<R>(self, func: impl FnOnce(&mut R)) -> Self
fn tap_ref_mut_dbg<R>(self, func: impl FnOnce(&mut R)) -> Self
.tap_ref_mut()
only in debug builds, and is erased in release
builds.§fn tap_deref_dbg<T>(self, func: impl FnOnce(&T)) -> Self
fn tap_deref_dbg<T>(self, func: impl FnOnce(&T)) -> Self
.tap_deref()
only in debug builds, and is erased in release
builds.