Struct rerun::sdk::archetypes::Transform3D
source · pub struct Transform3D {
pub translation: Option<SerializedComponentBatch>,
pub rotation_axis_angle: Option<SerializedComponentBatch>,
pub quaternion: Option<SerializedComponentBatch>,
pub scale: Option<SerializedComponentBatch>,
pub mat3x3: Option<SerializedComponentBatch>,
pub relation: Option<SerializedComponentBatch>,
pub axis_length: Option<SerializedComponentBatch>,
}
Expand description
Archetype: A transform between two 3D spaces, i.e. a pose.
From the point of view of the entity’s coordinate system, all components are applied in the inverse order they are listed here. E.g. if both a translation and a max3x3 transform are present, the 3x3 matrix is applied first, followed by the translation.
Whenever you log this archetype, it will write all components, even if you do not explicitly set them. This means that if you first log a transform with only a translation, and then log one with only a rotation, it will be resolved to a transform with only a rotation.
For transforms that affect only a single entity and do not propagate along the entity tree refer to archetypes::InstancePoses3D
.
§Examples
§Variety of 3D transforms
use std::f32::consts::TAU;
fn main() -> Result<(), Box<dyn std::error::Error>> {
let rec = rerun::RecordingStreamBuilder::new("rerun_example_transform3d").spawn()?;
let arrow = rerun::Arrows3D::from_vectors([(0.0, 1.0, 0.0)]).with_origins([(0.0, 0.0, 0.0)]);
rec.log("base", &arrow)?;
rec.log(
"base/translated",
&rerun::Transform3D::from_translation([1.0, 0.0, 0.0]),
)?;
rec.log("base/translated", &arrow)?;
rec.log(
"base/rotated_scaled",
&rerun::Transform3D::from_rotation_scale(
rerun::RotationAxisAngle::new([0.0, 0.0, 1.0], rerun::Angle::from_radians(TAU / 8.0)),
rerun::Scale3D::from(2.0),
),
)?;
rec.log("base/rotated_scaled", &arrow)?;
Ok(())
}
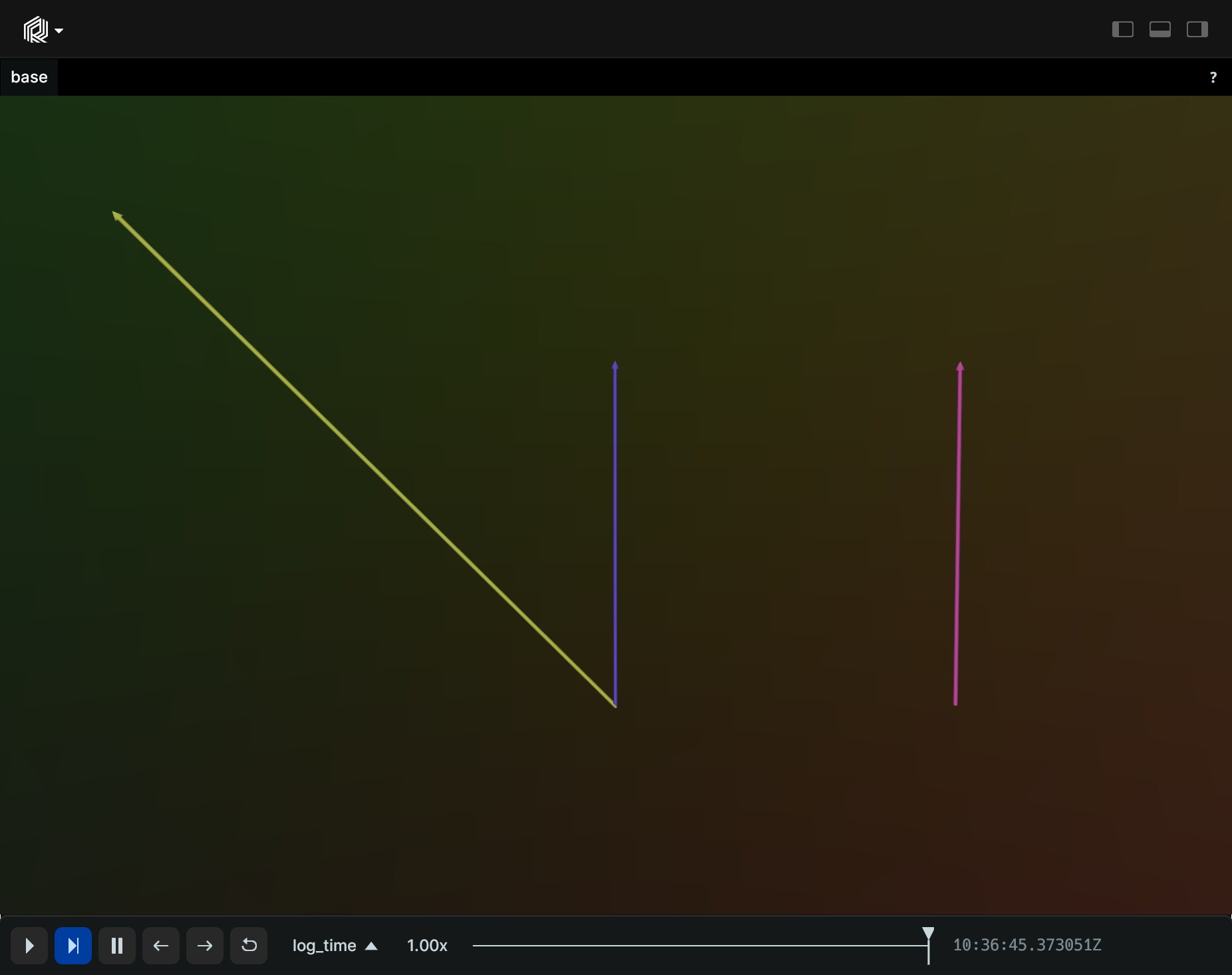
§Transform hierarchy
fn main() -> Result<(), Box<dyn std::error::Error>> {
let rec = rerun::RecordingStreamBuilder::new("rerun_example_transform3d_hierarchy").spawn()?;
// TODO(#5521): log two views as in the python example
rec.set_time_seconds("sim_time", 0.0);
// Planetary motion is typically in the XY plane.
rec.log_static("/", &rerun::ViewCoordinates::RIGHT_HAND_Z_UP())?;
// Setup points, all are in the center of their own space:
rec.log(
"sun",
&rerun::Points3D::new([[0.0, 0.0, 0.0]])
.with_radii([1.0])
.with_colors([rerun::Color::from_rgb(255, 200, 10)]),
)?;
rec.log(
"sun/planet",
&rerun::Points3D::new([[0.0, 0.0, 0.0]])
.with_radii([0.4])
.with_colors([rerun::Color::from_rgb(40, 80, 200)]),
)?;
rec.log(
"sun/planet/moon",
&rerun::Points3D::new([[0.0, 0.0, 0.0]])
.with_radii([0.15])
.with_colors([rerun::Color::from_rgb(180, 180, 180)]),
)?;
// Draw fixed paths where the planet & moon move.
let d_planet = 6.0;
let d_moon = 3.0;
let angles = (0..=100).map(|i| i as f32 * 0.01 * std::f32::consts::TAU);
let circle: Vec<_> = angles.map(|angle| [angle.sin(), angle.cos()]).collect();
rec.log(
"sun/planet_path",
&rerun::LineStrips3D::new([rerun::LineStrip3D::from_iter(
circle
.iter()
.map(|p| [p[0] * d_planet, p[1] * d_planet, 0.0]),
)]),
)?;
rec.log(
"sun/planet/moon_path",
&rerun::LineStrips3D::new([rerun::LineStrip3D::from_iter(
circle.iter().map(|p| [p[0] * d_moon, p[1] * d_moon, 0.0]),
)]),
)?;
// Movement via transforms.
for i in 0..(6 * 120) {
let time = i as f32 / 120.0;
rec.set_time_seconds("sim_time", time);
let r_moon = time * 5.0;
let r_planet = time * 2.0;
rec.log(
"sun/planet",
&rerun::Transform3D::from_translation_rotation(
[r_planet.sin() * d_planet, r_planet.cos() * d_planet, 0.0],
rerun::RotationAxisAngle {
axis: [1.0, 0.0, 0.0].into(),
angle: rerun::Angle::from_degrees(20.0),
},
),
)?;
rec.log(
"sun/planet/moon",
&rerun::Transform3D::from_translation([
r_moon.cos() * d_moon,
r_moon.sin() * d_moon,
0.0,
])
.with_relation(rerun::TransformRelation::ChildFromParent),
)?;
}
Ok(())
}
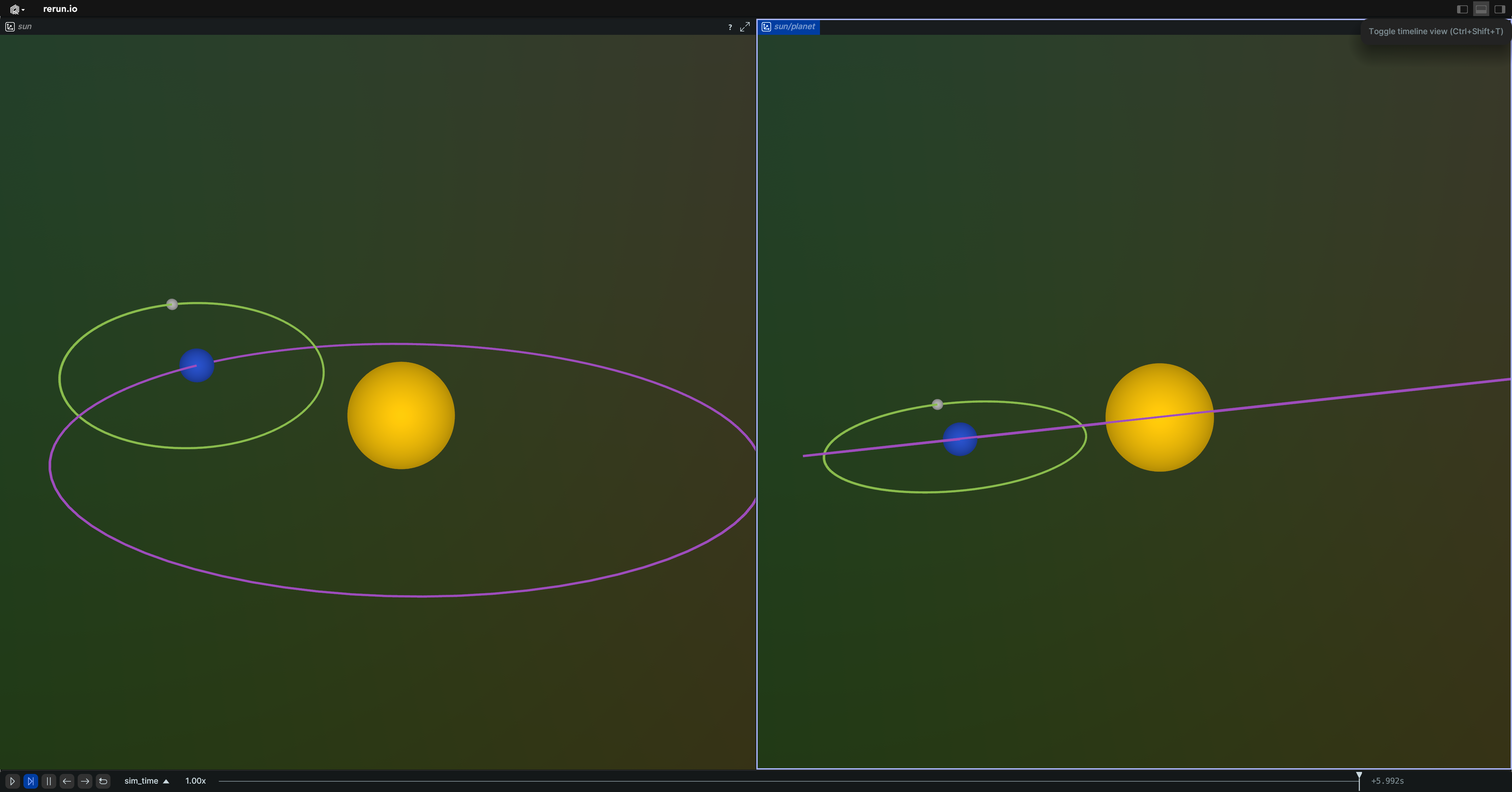
§Update a transform over time
fn main() -> Result<(), Box<dyn std::error::Error>> {
let rec =
rerun::RecordingStreamBuilder::new("rerun_example_transform3d_row_updates").spawn()?;
rec.set_time_sequence("tick", 0);
rec.log(
"box",
&[
&rerun::Boxes3D::from_half_sizes([(4.0, 2.0, 1.0)])
.with_fill_mode(rerun::FillMode::Solid) as &dyn rerun::AsComponents,
&rerun::Transform3D::default().with_axis_length(10.0),
],
)?;
for t in 0..100 {
rec.set_time_sequence("tick", t + 1);
rec.log(
"box",
&rerun::Transform3D::default()
.with_translation([0.0, 0.0, t as f32 / 10.0])
.with_rotation(rerun::RotationAxisAngle::new(
[0.0, 1.0, 0.0],
rerun::Angle::from_radians(truncated_radians((t * 4) as f32)),
)),
)?;
}
Ok(())
}
fn truncated_radians(deg: f32) -> f32 {
((deg.to_radians() * 1000.0) as i32) as f32 / 1000.0
}
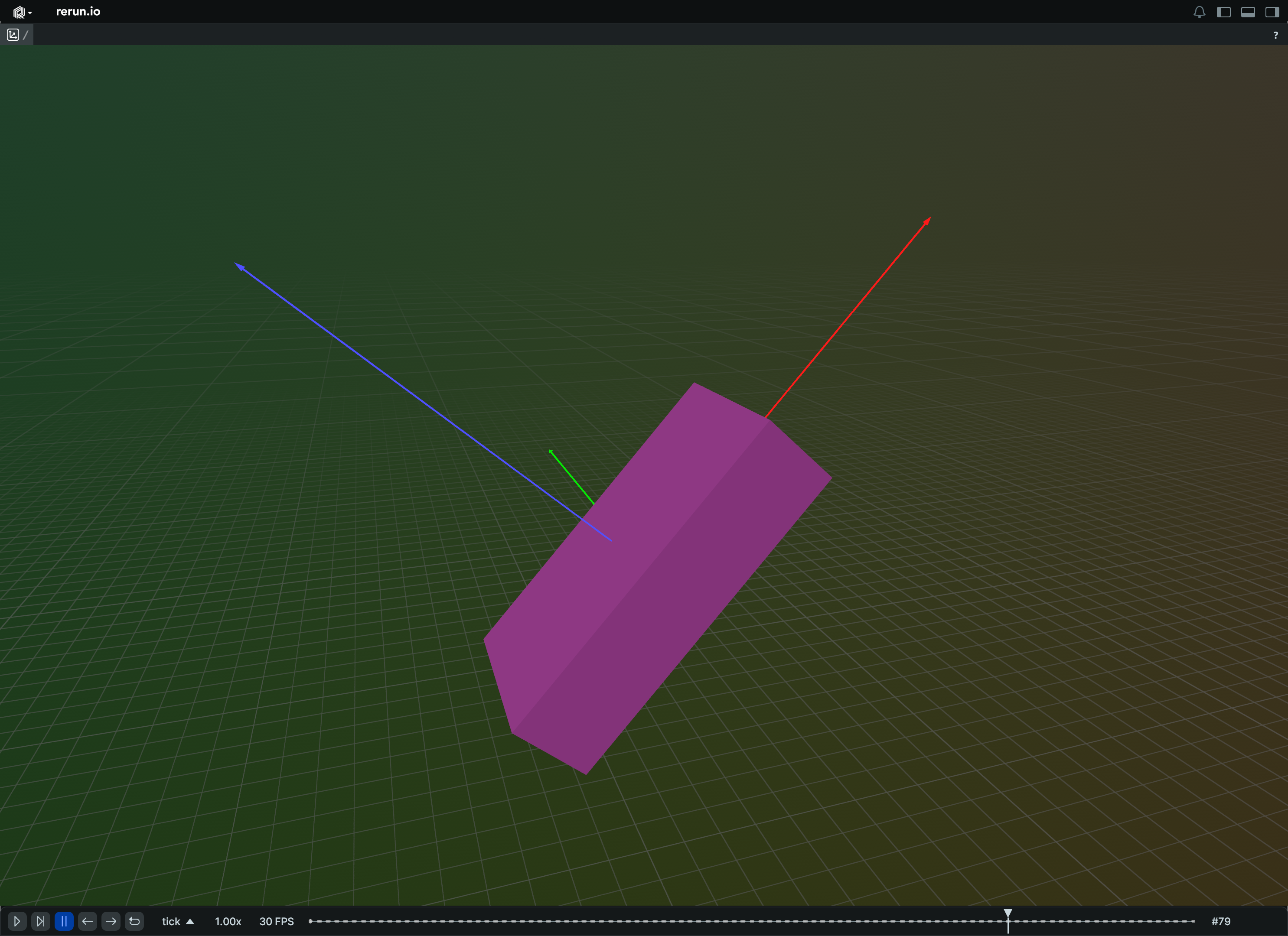
§Update a transform over time, in a single operation
fn main() -> Result<(), Box<dyn std::error::Error>> {
let rec =
rerun::RecordingStreamBuilder::new("rerun_example_transform3d_column_updates").spawn()?;
rec.set_time_sequence("tick", 0);
rec.log(
"box",
&[
&rerun::Boxes3D::from_half_sizes([(4.0, 2.0, 1.0)])
.with_fill_mode(rerun::FillMode::Solid) as &dyn rerun::AsComponents,
&rerun::Transform3D::default().with_axis_length(10.0),
],
)?;
let translations = (0..100).map(|t| [0.0, 0.0, t as f32 / 10.0]);
let rotations = (0..100)
.map(|t| truncated_radians((t * 4) as f32))
.map(|rad| rerun::RotationAxisAngle::new([0.0, 1.0, 0.0], rerun::Angle::from_radians(rad)));
let ticks = rerun::TimeColumn::new_sequence("tick", 1..101);
rec.send_columns(
"box",
[ticks],
rerun::Transform3D::default()
.with_many_translation(translations)
.with_many_rotation_axis_angle(rotations)
.columns_of_unit_batches()?,
)?;
Ok(())
}
fn truncated_radians(deg: f32) -> f32 {
((deg.to_radians() * 1000.0) as i32) as f32 / 1000.0
}
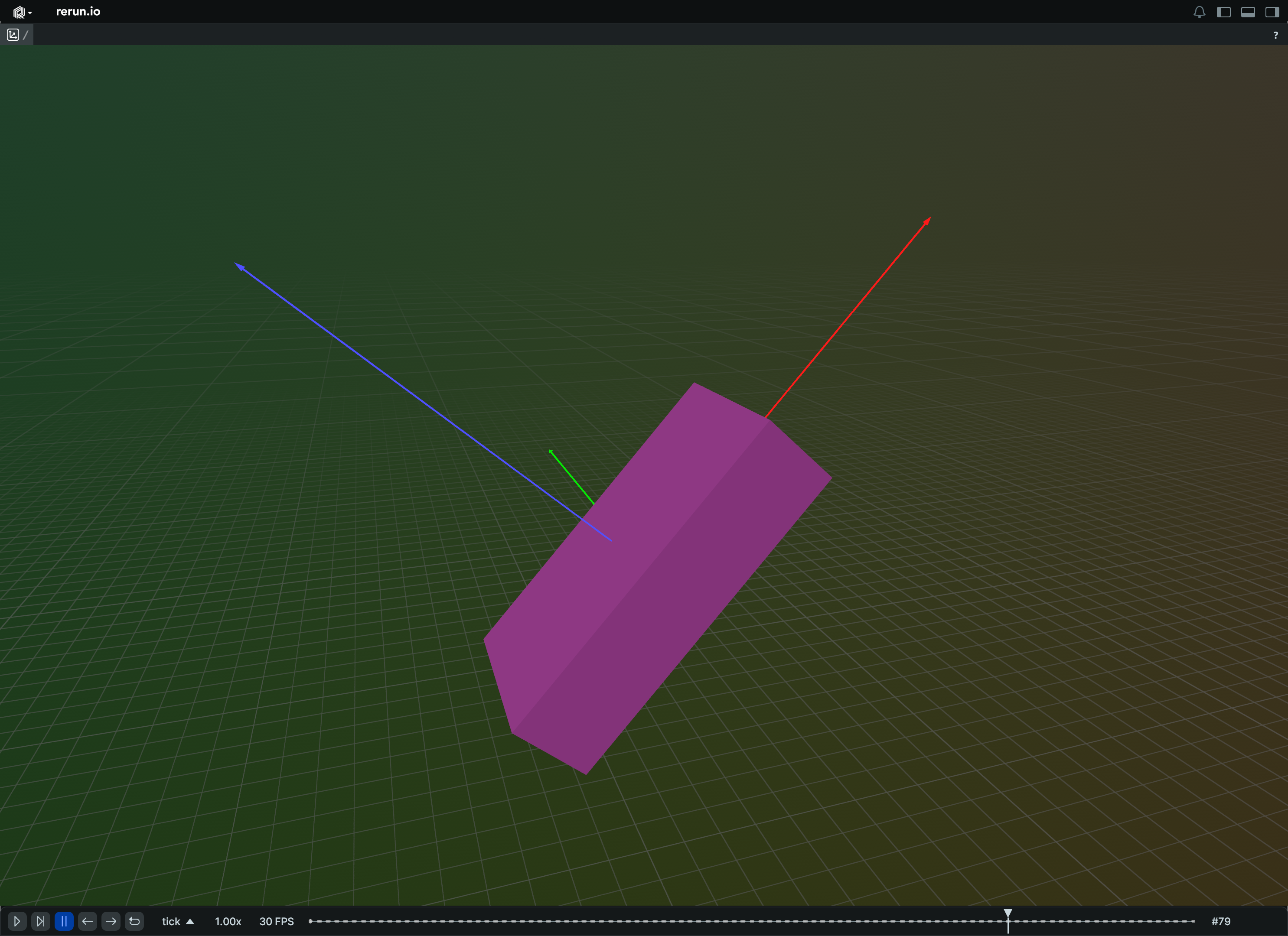
§Update specific properties of a transform over time
fn main() -> Result<(), Box<dyn std::error::Error>> {
let rec =
rerun::RecordingStreamBuilder::new("rerun_example_transform3d_partial_updates").spawn()?;
// Set up a 3D box.
rec.log(
"box",
&[
&rerun::Boxes3D::from_half_sizes([(4.0, 2.0, 1.0)])
.with_fill_mode(rerun::FillMode::Solid) as &dyn rerun::AsComponents,
&rerun::Transform3D::default().with_axis_length(10.0),
],
)?;
// Update only the rotation of the box.
for deg in 0..=45 {
let rad = truncated_radians((deg * 4) as f32);
rec.log(
"box",
&rerun::Transform3D::update_fields().with_rotation(rerun::RotationAxisAngle::new(
[0.0, 1.0, 0.0],
rerun::Angle::from_radians(rad),
)),
)?;
}
// Update only the position of the box.
for t in 0..=50 {
rec.log(
"box",
&rerun::Transform3D::update_fields().with_translation([0.0, 0.0, t as f32 / 10.0]),
)?;
}
// Update only the rotation of the box.
for deg in 0..=45 {
let rad = truncated_radians(((deg + 45) * 4) as f32);
rec.log(
"box",
&rerun::Transform3D::update_fields().with_rotation(rerun::RotationAxisAngle::new(
[0.0, 1.0, 0.0],
rerun::Angle::from_radians(rad),
)),
)?;
}
// Clear all of the box's attributes, and reset its axis length.
rec.log(
"box",
&rerun::Transform3D::clear_fields().with_axis_length(15.0),
)?;
Ok(())
}
fn truncated_radians(deg: f32) -> f32 {
((deg.to_radians() * 1000.0) as i32) as f32 / 1000.0
}
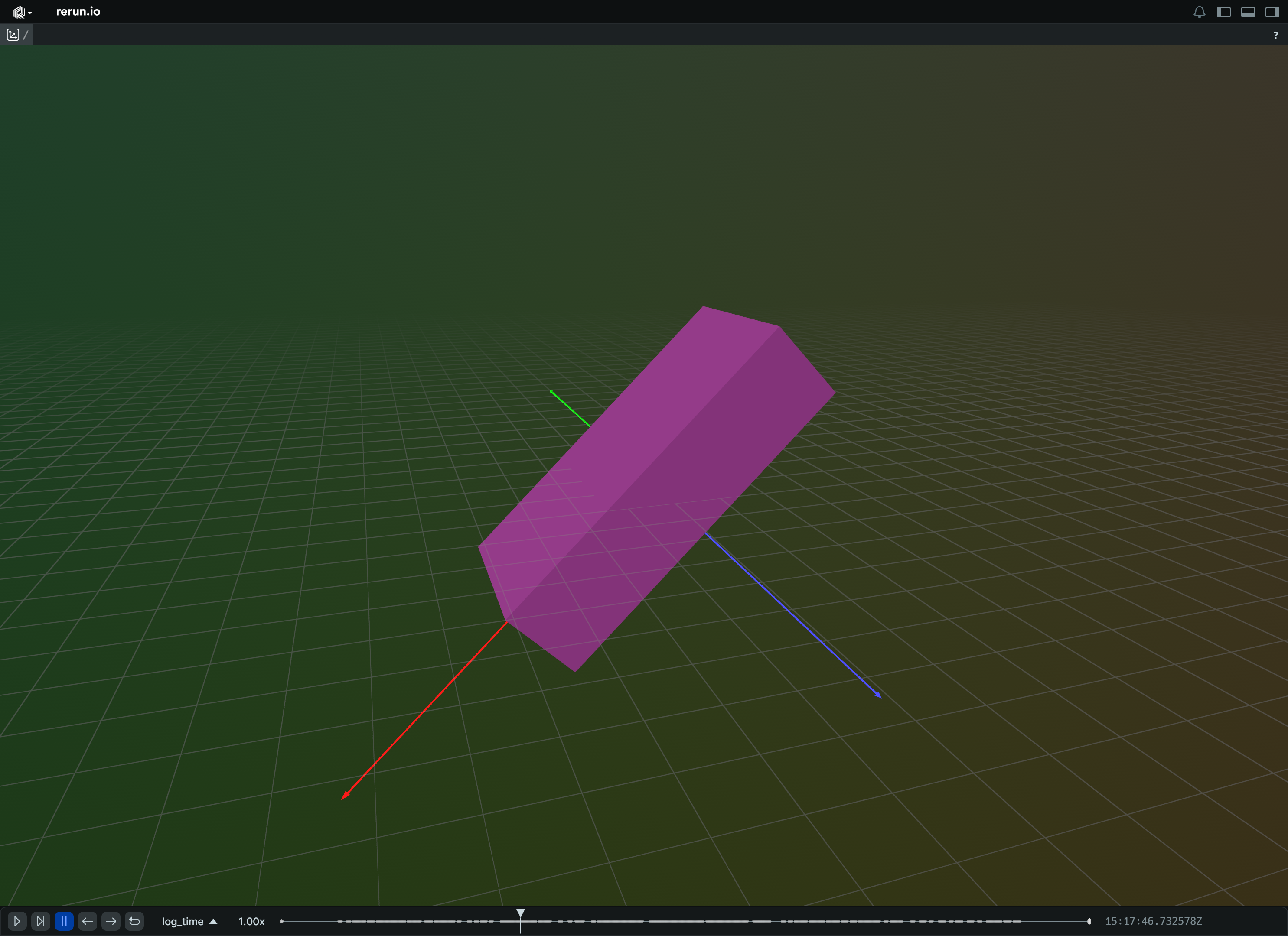
Fields§
§translation: Option<SerializedComponentBatch>
Translation vector.
rotation_axis_angle: Option<SerializedComponentBatch>
Rotation via axis + angle.
quaternion: Option<SerializedComponentBatch>
Rotation via quaternion.
scale: Option<SerializedComponentBatch>
Scaling factor.
mat3x3: Option<SerializedComponentBatch>
3x3 transformation matrix.
relation: Option<SerializedComponentBatch>
Specifies the relation this transform establishes between this entity and its parent.
axis_length: Option<SerializedComponentBatch>
Visual length of the 3 axes.
The length is interpreted in the local coordinate system of the transform. If the transform is scaled, the axes will be scaled accordingly.
Implementations§
source§impl Transform3D
impl Transform3D
sourcepub fn descriptor_translation() -> ComponentDescriptor
pub fn descriptor_translation() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::translation
.
sourcepub fn descriptor_rotation_axis_angle() -> ComponentDescriptor
pub fn descriptor_rotation_axis_angle() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::rotation_axis_angle
.
sourcepub fn descriptor_quaternion() -> ComponentDescriptor
pub fn descriptor_quaternion() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::quaternion
.
sourcepub fn descriptor_scale() -> ComponentDescriptor
pub fn descriptor_scale() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::scale
.
sourcepub fn descriptor_mat3x3() -> ComponentDescriptor
pub fn descriptor_mat3x3() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::mat3x3
.
sourcepub fn descriptor_relation() -> ComponentDescriptor
pub fn descriptor_relation() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::relation
.
sourcepub fn descriptor_axis_length() -> ComponentDescriptor
pub fn descriptor_axis_length() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::axis_length
.
sourcepub fn descriptor_indicator() -> ComponentDescriptor
pub fn descriptor_indicator() -> ComponentDescriptor
Returns the ComponentDescriptor
for the associated indicator component.
source§impl Transform3D
impl Transform3D
sourcepub const NUM_COMPONENTS: usize = 8usize
pub const NUM_COMPONENTS: usize = 8usize
The total number of components in the archetype: 0 required, 1 recommended, 7 optional
source§impl Transform3D
impl Transform3D
sourcepub fn update_fields() -> Transform3D
pub fn update_fields() -> Transform3D
Update only some specific fields of a Transform3D
.
sourcepub fn clear_fields() -> Transform3D
pub fn clear_fields() -> Transform3D
Clear all the fields of a Transform3D
.
sourcepub fn columns<I>(
self,
_lengths: I,
) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
pub fn columns<I>( self, _lengths: I, ) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
Partitions the component data into multiple sub-batches.
Specifically, this transforms the existing SerializedComponentBatch
es data into SerializedComponentColumn
s
instead, via SerializedComponentBatch::partitioned
.
This makes it possible to use RecordingStream::send_columns
to send columnar data directly into Rerun.
The specified lengths
must sum to the total length of the component batch.
sourcepub fn columns_of_unit_batches(
self,
) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
pub fn columns_of_unit_batches( self, ) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
Helper to partition the component data into unit-length sub-batches.
This is semantically similar to calling Self::columns
with std::iter::take(1).repeat(n)
,
where n
is automatically guessed.
sourcepub fn with_translation(
self,
translation: impl Into<Translation3D>,
) -> Transform3D
pub fn with_translation( self, translation: impl Into<Translation3D>, ) -> Transform3D
Translation vector.
sourcepub fn with_many_translation(
self,
translation: impl IntoIterator<Item = impl Into<Translation3D>>,
) -> Transform3D
pub fn with_many_translation( self, translation: impl IntoIterator<Item = impl Into<Translation3D>>, ) -> Transform3D
This method makes it possible to pack multiple crate::components::Translation3D
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_translation
should
be used when logging a single row’s worth of data.
sourcepub fn with_rotation_axis_angle(
self,
rotation_axis_angle: impl Into<RotationAxisAngle>,
) -> Transform3D
pub fn with_rotation_axis_angle( self, rotation_axis_angle: impl Into<RotationAxisAngle>, ) -> Transform3D
Rotation via axis + angle.
sourcepub fn with_many_rotation_axis_angle(
self,
rotation_axis_angle: impl IntoIterator<Item = impl Into<RotationAxisAngle>>,
) -> Transform3D
pub fn with_many_rotation_axis_angle( self, rotation_axis_angle: impl IntoIterator<Item = impl Into<RotationAxisAngle>>, ) -> Transform3D
This method makes it possible to pack multiple crate::components::RotationAxisAngle
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_rotation_axis_angle
should
be used when logging a single row’s worth of data.
sourcepub fn with_quaternion(self, quaternion: impl Into<RotationQuat>) -> Transform3D
pub fn with_quaternion(self, quaternion: impl Into<RotationQuat>) -> Transform3D
Rotation via quaternion.
sourcepub fn with_many_quaternion(
self,
quaternion: impl IntoIterator<Item = impl Into<RotationQuat>>,
) -> Transform3D
pub fn with_many_quaternion( self, quaternion: impl IntoIterator<Item = impl Into<RotationQuat>>, ) -> Transform3D
This method makes it possible to pack multiple crate::components::RotationQuat
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_quaternion
should
be used when logging a single row’s worth of data.
sourcepub fn with_scale(self, scale: impl Into<Scale3D>) -> Transform3D
pub fn with_scale(self, scale: impl Into<Scale3D>) -> Transform3D
Scaling factor.
sourcepub fn with_many_scale(
self,
scale: impl IntoIterator<Item = impl Into<Scale3D>>,
) -> Transform3D
pub fn with_many_scale( self, scale: impl IntoIterator<Item = impl Into<Scale3D>>, ) -> Transform3D
This method makes it possible to pack multiple crate::components::Scale3D
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_scale
should
be used when logging a single row’s worth of data.
sourcepub fn with_mat3x3(self, mat3x3: impl Into<TransformMat3x3>) -> Transform3D
pub fn with_mat3x3(self, mat3x3: impl Into<TransformMat3x3>) -> Transform3D
3x3 transformation matrix.
sourcepub fn with_many_mat3x3(
self,
mat3x3: impl IntoIterator<Item = impl Into<TransformMat3x3>>,
) -> Transform3D
pub fn with_many_mat3x3( self, mat3x3: impl IntoIterator<Item = impl Into<TransformMat3x3>>, ) -> Transform3D
This method makes it possible to pack multiple crate::components::TransformMat3x3
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_mat3x3
should
be used when logging a single row’s worth of data.
sourcepub fn with_relation(
self,
relation: impl Into<TransformRelation>,
) -> Transform3D
pub fn with_relation( self, relation: impl Into<TransformRelation>, ) -> Transform3D
Specifies the relation this transform establishes between this entity and its parent.
sourcepub fn with_many_relation(
self,
relation: impl IntoIterator<Item = impl Into<TransformRelation>>,
) -> Transform3D
pub fn with_many_relation( self, relation: impl IntoIterator<Item = impl Into<TransformRelation>>, ) -> Transform3D
This method makes it possible to pack multiple crate::components::TransformRelation
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_relation
should
be used when logging a single row’s worth of data.
sourcepub fn with_axis_length(self, axis_length: impl Into<AxisLength>) -> Transform3D
pub fn with_axis_length(self, axis_length: impl Into<AxisLength>) -> Transform3D
Visual length of the 3 axes.
The length is interpreted in the local coordinate system of the transform. If the transform is scaled, the axes will be scaled accordingly.
sourcepub fn with_many_axis_length(
self,
axis_length: impl IntoIterator<Item = impl Into<AxisLength>>,
) -> Transform3D
pub fn with_many_axis_length( self, axis_length: impl IntoIterator<Item = impl Into<AxisLength>>, ) -> Transform3D
This method makes it possible to pack multiple crate::components::AxisLength
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_axis_length
should
be used when logging a single row’s worth of data.
source§impl Transform3D
impl Transform3D
sourcepub const IDENTITY: Transform3D = _
pub const IDENTITY: Transform3D = _
The identity transform.
This is the same as Self::clear
, i.e. it logs an empty (default)
value for all components.
sourcepub fn clear() -> Transform3D
👎Deprecated since 0.22.0: Use Self::clear_fields()
instead.
pub fn clear() -> Transform3D
Self::clear_fields()
instead.Clear all the fields of a Transform3D
.
sourcepub fn with_rotation(self, rotation: impl Into<Rotation3D>) -> Transform3D
pub fn with_rotation(self, rotation: impl Into<Rotation3D>) -> Transform3D
Convenience method that takes any kind of (single) rotation representation and sets it on this transform.
sourcepub fn from_translation(translation: impl Into<Translation3D>) -> Transform3D
pub fn from_translation(translation: impl Into<Translation3D>) -> Transform3D
From a translation, clearing all other fields.
sourcepub fn from_mat3x3(mat3x3: impl Into<TransformMat3x3>) -> Transform3D
pub fn from_mat3x3(mat3x3: impl Into<TransformMat3x3>) -> Transform3D
From a 3x3 matrix, clearing all other fields.
sourcepub fn from_rotation(rotation: impl Into<Rotation3D>) -> Transform3D
pub fn from_rotation(rotation: impl Into<Rotation3D>) -> Transform3D
From a rotation, clearing all other fields.
sourcepub fn from_scale(scale: impl Into<Scale3D>) -> Transform3D
pub fn from_scale(scale: impl Into<Scale3D>) -> Transform3D
From a scale, clearing all other fields.
sourcepub fn from_translation_rotation(
translation: impl Into<Translation3D>,
rotation: impl Into<Rotation3D>,
) -> Transform3D
pub fn from_translation_rotation( translation: impl Into<Translation3D>, rotation: impl Into<Rotation3D>, ) -> Transform3D
From a translation applied after a rotation, known as a rigid transformation.
Clears all other fields.
sourcepub fn from_translation_mat3x3(
translation: impl Into<Translation3D>,
mat3x3: impl Into<TransformMat3x3>,
) -> Transform3D
pub fn from_translation_mat3x3( translation: impl Into<Translation3D>, mat3x3: impl Into<TransformMat3x3>, ) -> Transform3D
From a translation applied after a 3x3 matrix, clearing all other fields.
sourcepub fn from_translation_scale(
translation: impl Into<Translation3D>,
scale: impl Into<Scale3D>,
) -> Transform3D
pub fn from_translation_scale( translation: impl Into<Translation3D>, scale: impl Into<Scale3D>, ) -> Transform3D
From a translation applied after a scale, clearing all other fields.
sourcepub fn from_translation_rotation_scale(
translation: impl Into<Translation3D>,
rotation: impl Into<Rotation3D>,
scale: impl Into<Scale3D>,
) -> Transform3D
pub fn from_translation_rotation_scale( translation: impl Into<Translation3D>, rotation: impl Into<Rotation3D>, scale: impl Into<Scale3D>, ) -> Transform3D
From a translation, applied after a rotation & scale, known as an affine transformation, clearing all other fields.
sourcepub fn from_rotation_scale(
rotation: impl Into<Rotation3D>,
scale: impl Into<Scale3D>,
) -> Transform3D
pub fn from_rotation_scale( rotation: impl Into<Rotation3D>, scale: impl Into<Scale3D>, ) -> Transform3D
From a rotation & scale, clearing all other fields.
Trait Implementations§
source§impl Archetype for Transform3D
impl Archetype for Transform3D
§type Indicator = GenericIndicatorComponent<Transform3D>
type Indicator = GenericIndicatorComponent<Transform3D>
source§fn name() -> ArchetypeName
fn name() -> ArchetypeName
rerun.archetypes.Points2D
.source§fn display_name() -> &'static str
fn display_name() -> &'static str
source§fn required_components() -> Cow<'static, [ComponentDescriptor]>
fn required_components() -> Cow<'static, [ComponentDescriptor]>
source§fn recommended_components() -> Cow<'static, [ComponentDescriptor]>
fn recommended_components() -> Cow<'static, [ComponentDescriptor]>
source§fn optional_components() -> Cow<'static, [ComponentDescriptor]>
fn optional_components() -> Cow<'static, [ComponentDescriptor]>
source§fn all_components() -> Cow<'static, [ComponentDescriptor]>
fn all_components() -> Cow<'static, [ComponentDescriptor]>
source§fn from_arrow_components(
arrow_data: impl IntoIterator<Item = (ComponentDescriptor, Arc<dyn Array>)>,
) -> Result<Transform3D, DeserializationError>
fn from_arrow_components( arrow_data: impl IntoIterator<Item = (ComponentDescriptor, Arc<dyn Array>)>, ) -> Result<Transform3D, DeserializationError>
ComponentNames
, deserializes them
into this archetype. Read moresource§fn from_arrow(
data: impl IntoIterator<Item = (Field, Arc<dyn Array>)>,
) -> Result<Self, DeserializationError>where
Self: Sized,
fn from_arrow(
data: impl IntoIterator<Item = (Field, Arc<dyn Array>)>,
) -> Result<Self, DeserializationError>where
Self: Sized,
source§impl AsComponents for Transform3D
impl AsComponents for Transform3D
source§fn as_serialized_batches(&self) -> Vec<SerializedComponentBatch>
fn as_serialized_batches(&self) -> Vec<SerializedComponentBatch>
SerializedComponentBatch
es. Read moresource§impl Clone for Transform3D
impl Clone for Transform3D
source§fn clone(&self) -> Transform3D
fn clone(&self) -> Transform3D
1.0.0 · source§fn clone_from(&mut self, source: &Self)
fn clone_from(&mut self, source: &Self)
source
. Read moresource§impl Debug for Transform3D
impl Debug for Transform3D
source§impl Default for Transform3D
impl Default for Transform3D
source§fn default() -> Transform3D
fn default() -> Transform3D
source§impl PartialEq for Transform3D
impl PartialEq for Transform3D
source§fn eq(&self, other: &Transform3D) -> bool
fn eq(&self, other: &Transform3D) -> bool
self
and other
values to be equal, and is used
by ==
.source§impl SizeBytes for Transform3D
impl SizeBytes for Transform3D
source§fn heap_size_bytes(&self) -> u64
fn heap_size_bytes(&self) -> u64
self
uses on the heap. Read moresource§fn total_size_bytes(&self) -> u64
fn total_size_bytes(&self) -> u64
self
in bytes, accounting for both stack and heap space.source§fn stack_size_bytes(&self) -> u64
fn stack_size_bytes(&self) -> u64
self
on the stack, in bytes. Read moreimpl ArchetypeReflectionMarker for Transform3D
impl StructuralPartialEq for Transform3D
Auto Trait Implementations§
impl Freeze for Transform3D
impl !RefUnwindSafe for Transform3D
impl Send for Transform3D
impl Sync for Transform3D
impl Unpin for Transform3D
impl !UnwindSafe for Transform3D
Blanket Implementations§
source§impl<T> BorrowMut<T> for Twhere
T: ?Sized,
impl<T> BorrowMut<T> for Twhere
T: ?Sized,
source§fn borrow_mut(&mut self) -> &mut T
fn borrow_mut(&mut self) -> &mut T
source§impl<T> CheckedAs for T
impl<T> CheckedAs for T
source§fn checked_as<Dst>(self) -> Option<Dst>where
T: CheckedCast<Dst>,
fn checked_as<Dst>(self) -> Option<Dst>where
T: CheckedCast<Dst>,
source§impl<Src, Dst> CheckedCastFrom<Src> for Dstwhere
Src: CheckedCast<Dst>,
impl<Src, Dst> CheckedCastFrom<Src> for Dstwhere
Src: CheckedCast<Dst>,
source§fn checked_cast_from(src: Src) -> Option<Dst>
fn checked_cast_from(src: Src) -> Option<Dst>
source§impl<T> CloneToUninit for Twhere
T: Clone,
impl<T> CloneToUninit for Twhere
T: Clone,
source§default unsafe fn clone_to_uninit(&self, dst: *mut T)
default unsafe fn clone_to_uninit(&self, dst: *mut T)
clone_to_uninit
)§impl<T> Conv for T
impl<T> Conv for T
§impl<T> Downcast for Twhere
T: Any,
impl<T> Downcast for Twhere
T: Any,
§fn into_any(self: Box<T>) -> Box<dyn Any>
fn into_any(self: Box<T>) -> Box<dyn Any>
Box<dyn Trait>
(where Trait: Downcast
) to Box<dyn Any>
. Box<dyn Any>
can
then be further downcast
into Box<ConcreteType>
where ConcreteType
implements Trait
.§fn into_any_rc(self: Rc<T>) -> Rc<dyn Any>
fn into_any_rc(self: Rc<T>) -> Rc<dyn Any>
Rc<Trait>
(where Trait: Downcast
) to Rc<Any>
. Rc<Any>
can then be
further downcast
into Rc<ConcreteType>
where ConcreteType
implements Trait
.§fn as_any(&self) -> &(dyn Any + 'static)
fn as_any(&self) -> &(dyn Any + 'static)
&Trait
(where Trait: Downcast
) to &Any
. This is needed since Rust cannot
generate &Any
’s vtable from &Trait
’s.§fn as_any_mut(&mut self) -> &mut (dyn Any + 'static)
fn as_any_mut(&mut self) -> &mut (dyn Any + 'static)
&mut Trait
(where Trait: Downcast
) to &Any
. This is needed since Rust cannot
generate &mut Any
’s vtable from &mut Trait
’s.§impl<T> DowncastSync for T
impl<T> DowncastSync for T
§impl<T> Instrument for T
impl<T> Instrument for T
§fn instrument(self, span: Span) -> Instrumented<Self>
fn instrument(self, span: Span) -> Instrumented<Self>
§fn in_current_span(self) -> Instrumented<Self>
fn in_current_span(self) -> Instrumented<Self>
source§impl<T> IntoEither for T
impl<T> IntoEither for T
source§fn into_either(self, into_left: bool) -> Either<Self, Self>
fn into_either(self, into_left: bool) -> Either<Self, Self>
self
into a Left
variant of Either<Self, Self>
if into_left
is true
.
Converts self
into a Right
variant of Either<Self, Self>
otherwise. Read moresource§fn into_either_with<F>(self, into_left: F) -> Either<Self, Self>
fn into_either_with<F>(self, into_left: F) -> Either<Self, Self>
self
into a Left
variant of Either<Self, Self>
if into_left(&self)
returns true
.
Converts self
into a Right
variant of Either<Self, Self>
otherwise. Read moresource§impl<T> IntoRequest<T> for T
impl<T> IntoRequest<T> for T
source§fn into_request(self) -> Request<T>
fn into_request(self) -> Request<T>
T
in a tonic::Request
source§impl<Src, Dst> LosslessTryInto<Dst> for Srcwhere
Dst: LosslessTryFrom<Src>,
impl<Src, Dst> LosslessTryInto<Dst> for Srcwhere
Dst: LosslessTryFrom<Src>,
source§fn lossless_try_into(self) -> Option<Dst>
fn lossless_try_into(self) -> Option<Dst>
source§impl<Src, Dst> LossyInto<Dst> for Srcwhere
Dst: LossyFrom<Src>,
impl<Src, Dst> LossyInto<Dst> for Srcwhere
Dst: LossyFrom<Src>,
source§fn lossy_into(self) -> Dst
fn lossy_into(self) -> Dst
§impl<T> NoneValue for Twhere
T: Default,
impl<T> NoneValue for Twhere
T: Default,
type NoneType = T
§fn null_value() -> T
fn null_value() -> T
source§impl<T> OverflowingAs for T
impl<T> OverflowingAs for T
source§fn overflowing_as<Dst>(self) -> (Dst, bool)where
T: OverflowingCast<Dst>,
fn overflowing_as<Dst>(self) -> (Dst, bool)where
T: OverflowingCast<Dst>,
source§impl<Src, Dst> OverflowingCastFrom<Src> for Dstwhere
Src: OverflowingCast<Dst>,
impl<Src, Dst> OverflowingCastFrom<Src> for Dstwhere
Src: OverflowingCast<Dst>,
source§fn overflowing_cast_from(src: Src) -> (Dst, bool)
fn overflowing_cast_from(src: Src) -> (Dst, bool)
§impl<T> Pipe for Twhere
T: ?Sized,
impl<T> Pipe for Twhere
T: ?Sized,
§fn pipe<R>(self, func: impl FnOnce(Self) -> R) -> Rwhere
Self: Sized,
fn pipe<R>(self, func: impl FnOnce(Self) -> R) -> Rwhere
Self: Sized,
§fn pipe_ref<'a, R>(&'a self, func: impl FnOnce(&'a Self) -> R) -> Rwhere
R: 'a,
fn pipe_ref<'a, R>(&'a self, func: impl FnOnce(&'a Self) -> R) -> Rwhere
R: 'a,
self
and passes that borrow into the pipe function. Read more§fn pipe_ref_mut<'a, R>(&'a mut self, func: impl FnOnce(&'a mut Self) -> R) -> Rwhere
R: 'a,
fn pipe_ref_mut<'a, R>(&'a mut self, func: impl FnOnce(&'a mut Self) -> R) -> Rwhere
R: 'a,
self
and passes that borrow into the pipe function. Read more§fn pipe_borrow<'a, B, R>(&'a self, func: impl FnOnce(&'a B) -> R) -> R
fn pipe_borrow<'a, B, R>(&'a self, func: impl FnOnce(&'a B) -> R) -> R
§fn pipe_borrow_mut<'a, B, R>(
&'a mut self,
func: impl FnOnce(&'a mut B) -> R,
) -> R
fn pipe_borrow_mut<'a, B, R>( &'a mut self, func: impl FnOnce(&'a mut B) -> R, ) -> R
§fn pipe_as_ref<'a, U, R>(&'a self, func: impl FnOnce(&'a U) -> R) -> R
fn pipe_as_ref<'a, U, R>(&'a self, func: impl FnOnce(&'a U) -> R) -> R
self
, then passes self.as_ref()
into the pipe function.§fn pipe_as_mut<'a, U, R>(&'a mut self, func: impl FnOnce(&'a mut U) -> R) -> R
fn pipe_as_mut<'a, U, R>(&'a mut self, func: impl FnOnce(&'a mut U) -> R) -> R
self
, then passes self.as_mut()
into the pipe
function.§fn pipe_deref<'a, T, R>(&'a self, func: impl FnOnce(&'a T) -> R) -> R
fn pipe_deref<'a, T, R>(&'a self, func: impl FnOnce(&'a T) -> R) -> R
self
, then passes self.deref()
into the pipe function.§impl<T> Pointable for T
impl<T> Pointable for T
source§impl<T> SaturatingAs for T
impl<T> SaturatingAs for T
source§fn saturating_as<Dst>(self) -> Dstwhere
T: SaturatingCast<Dst>,
fn saturating_as<Dst>(self) -> Dstwhere
T: SaturatingCast<Dst>,
source§impl<Src, Dst> SaturatingCastFrom<Src> for Dstwhere
Src: SaturatingCast<Dst>,
impl<Src, Dst> SaturatingCastFrom<Src> for Dstwhere
Src: SaturatingCast<Dst>,
source§fn saturating_cast_from(src: Src) -> Dst
fn saturating_cast_from(src: Src) -> Dst
§impl<T> Tap for T
impl<T> Tap for T
§fn tap_borrow<B>(self, func: impl FnOnce(&B)) -> Self
fn tap_borrow<B>(self, func: impl FnOnce(&B)) -> Self
Borrow<B>
of a value. Read more§fn tap_borrow_mut<B>(self, func: impl FnOnce(&mut B)) -> Self
fn tap_borrow_mut<B>(self, func: impl FnOnce(&mut B)) -> Self
BorrowMut<B>
of a value. Read more§fn tap_ref<R>(self, func: impl FnOnce(&R)) -> Self
fn tap_ref<R>(self, func: impl FnOnce(&R)) -> Self
AsRef<R>
view of a value. Read more§fn tap_ref_mut<R>(self, func: impl FnOnce(&mut R)) -> Self
fn tap_ref_mut<R>(self, func: impl FnOnce(&mut R)) -> Self
AsMut<R>
view of a value. Read more§fn tap_deref<T>(self, func: impl FnOnce(&T)) -> Self
fn tap_deref<T>(self, func: impl FnOnce(&T)) -> Self
Deref::Target
of a value. Read more§fn tap_deref_mut<T>(self, func: impl FnOnce(&mut T)) -> Self
fn tap_deref_mut<T>(self, func: impl FnOnce(&mut T)) -> Self
Deref::Target
of a value. Read more§fn tap_dbg(self, func: impl FnOnce(&Self)) -> Self
fn tap_dbg(self, func: impl FnOnce(&Self)) -> Self
.tap()
only in debug builds, and is erased in release builds.§fn tap_mut_dbg(self, func: impl FnOnce(&mut Self)) -> Self
fn tap_mut_dbg(self, func: impl FnOnce(&mut Self)) -> Self
.tap_mut()
only in debug builds, and is erased in release
builds.§fn tap_borrow_dbg<B>(self, func: impl FnOnce(&B)) -> Self
fn tap_borrow_dbg<B>(self, func: impl FnOnce(&B)) -> Self
.tap_borrow()
only in debug builds, and is erased in release
builds.§fn tap_borrow_mut_dbg<B>(self, func: impl FnOnce(&mut B)) -> Self
fn tap_borrow_mut_dbg<B>(self, func: impl FnOnce(&mut B)) -> Self
.tap_borrow_mut()
only in debug builds, and is erased in release
builds.§fn tap_ref_dbg<R>(self, func: impl FnOnce(&R)) -> Self
fn tap_ref_dbg<R>(self, func: impl FnOnce(&R)) -> Self
.tap_ref()
only in debug builds, and is erased in release
builds.§fn tap_ref_mut_dbg<R>(self, func: impl FnOnce(&mut R)) -> Self
fn tap_ref_mut_dbg<R>(self, func: impl FnOnce(&mut R)) -> Self
.tap_ref_mut()
only in debug builds, and is erased in release
builds.§fn tap_deref_dbg<T>(self, func: impl FnOnce(&T)) -> Self
fn tap_deref_dbg<T>(self, func: impl FnOnce(&T)) -> Self
.tap_deref()
only in debug builds, and is erased in release
builds.