Struct rerun::sdk::prelude::DepthImage
source · pub struct DepthImage {
pub buffer: Option<SerializedComponentBatch>,
pub format: Option<SerializedComponentBatch>,
pub meter: Option<SerializedComponentBatch>,
pub colormap: Option<SerializedComponentBatch>,
pub depth_range: Option<SerializedComponentBatch>,
pub point_fill_ratio: Option<SerializedComponentBatch>,
pub draw_order: Option<SerializedComponentBatch>,
}
Expand description
Archetype: A depth image, i.e. as captured by a depth camera.
Each pixel corresponds to a depth value in units specified by components::DepthMeter
.
§Example
§Depth to 3D example
use ndarray::{s, Array, ShapeBuilder};
fn main() -> Result<(), Box<dyn std::error::Error>> {
let rec = rerun::RecordingStreamBuilder::new("rerun_example_depth_image_3d").spawn()?;
let width = 300;
let height = 200;
let mut image = Array::<u16, _>::from_elem((height, width).f(), 65535);
image.slice_mut(s![50..150, 50..150]).fill(20000);
image.slice_mut(s![130..180, 100..280]).fill(45000);
let depth_image = rerun::DepthImage::try_from(image)?
.with_meter(10000.0)
.with_colormap(rerun::components::Colormap::Viridis);
// If we log a pinhole camera model, the depth gets automatically back-projected to 3D
rec.log(
"world/camera",
&rerun::Pinhole::from_focal_length_and_resolution(
[200.0, 200.0],
[width as f32, height as f32],
),
)?;
rec.log("world/camera/depth", &depth_image)?;
Ok(())
}
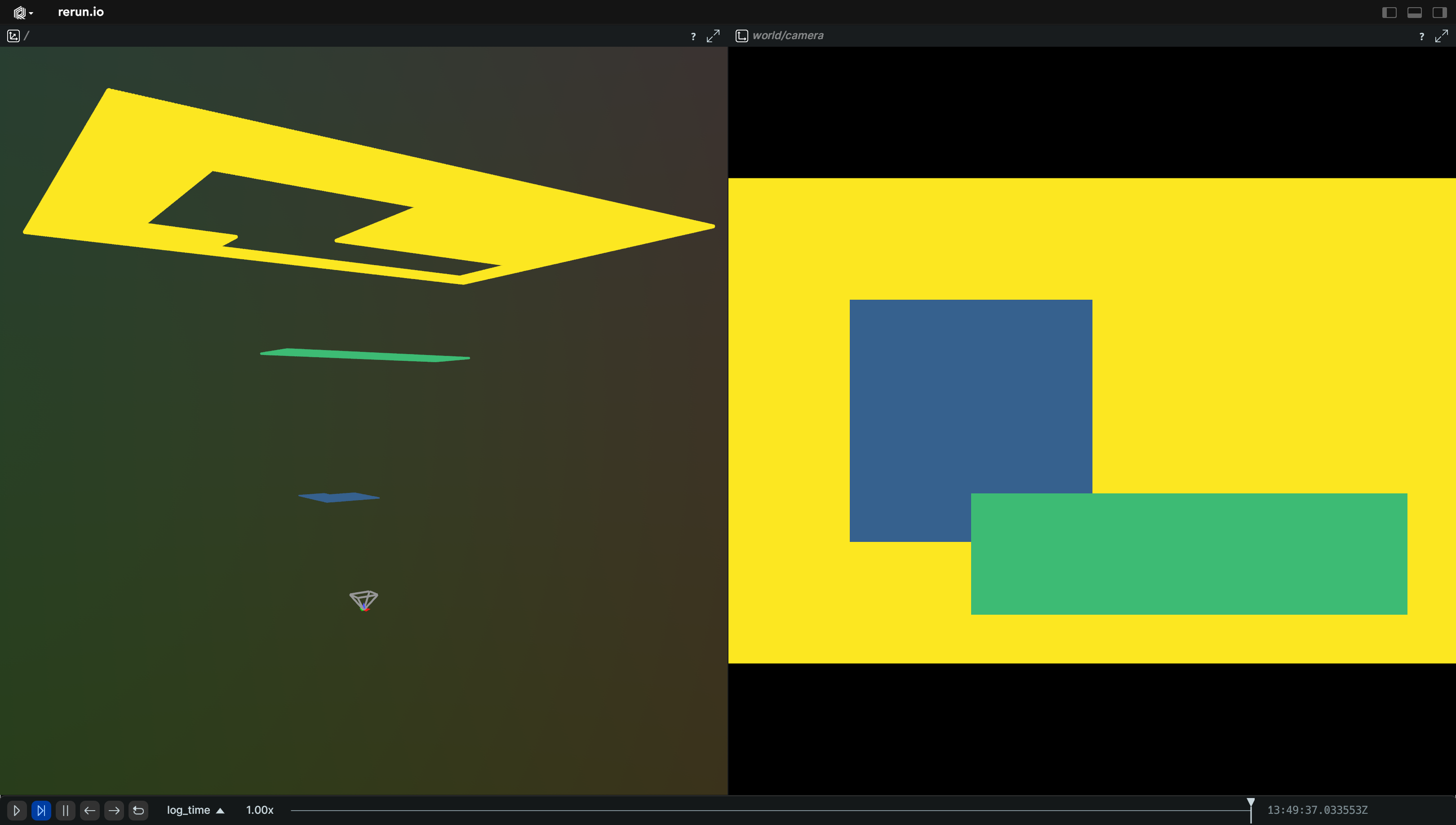
Fields§
§buffer: Option<SerializedComponentBatch>
The raw depth image data.
format: Option<SerializedComponentBatch>
The format of the image.
meter: Option<SerializedComponentBatch>
An optional floating point value that specifies how long a meter is in the native depth units.
For instance: with uint16, perhaps meter=1000 which would mean you have millimeter precision and a range of up to ~65 meters (2^16 / 1000).
Note that the only effect on 2D views is the physical depth values shown when hovering the image. In 3D views on the other hand, this affects where the points of the point cloud are placed.
colormap: Option<SerializedComponentBatch>
Colormap to use for rendering the depth image.
If not set, the depth image will be rendered using the Turbo colormap.
depth_range: Option<SerializedComponentBatch>
The expected range of depth values.
This is typically the expected range of valid values. Everything outside of the range is clamped to the range for the purpose of colormpaping. Note that point clouds generated from this image will still display all points, regardless of this range.
If not specified, the range will be automatically estimated from the data. Note that the Viewer may try to guess a wider range than the minimum/maximum of values in the contents of the depth image. E.g. if all values are positive, some bigger than 1.0 and all smaller than 255.0, the Viewer will guess that the data likely came from an 8bit image, thus assuming a range of 0-255.
point_fill_ratio: Option<SerializedComponentBatch>
Scale the radii of the points in the point cloud generated from this image.
A fill ratio of 1.0 (the default) means that each point is as big as to touch the center of its neighbor if it is at the same depth, leaving no gaps. A fill ratio of 0.5 means that each point touches the edge of its neighbor if it has the same depth.
TODO(#6744): This applies only to 3D views!
draw_order: Option<SerializedComponentBatch>
An optional floating point value that specifies the 2D drawing order, used only if the depth image is shown as a 2D image.
Objects with higher values are drawn on top of those with lower values.
Implementations§
source§impl DepthImage
impl DepthImage
sourcepub fn descriptor_buffer() -> ComponentDescriptor
pub fn descriptor_buffer() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::buffer
.
sourcepub fn descriptor_format() -> ComponentDescriptor
pub fn descriptor_format() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::format
.
sourcepub fn descriptor_meter() -> ComponentDescriptor
pub fn descriptor_meter() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::meter
.
sourcepub fn descriptor_colormap() -> ComponentDescriptor
pub fn descriptor_colormap() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::colormap
.
sourcepub fn descriptor_depth_range() -> ComponentDescriptor
pub fn descriptor_depth_range() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::depth_range
.
sourcepub fn descriptor_point_fill_ratio() -> ComponentDescriptor
pub fn descriptor_point_fill_ratio() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::point_fill_ratio
.
sourcepub fn descriptor_draw_order() -> ComponentDescriptor
pub fn descriptor_draw_order() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::draw_order
.
sourcepub fn descriptor_indicator() -> ComponentDescriptor
pub fn descriptor_indicator() -> ComponentDescriptor
Returns the ComponentDescriptor
for the associated indicator component.
source§impl DepthImage
impl DepthImage
sourcepub const NUM_COMPONENTS: usize = 8usize
pub const NUM_COMPONENTS: usize = 8usize
The total number of components in the archetype: 2 required, 1 recommended, 5 optional
source§impl DepthImage
impl DepthImage
sourcepub fn new(
buffer: impl Into<ImageBuffer>,
format: impl Into<ImageFormat>,
) -> DepthImage
pub fn new( buffer: impl Into<ImageBuffer>, format: impl Into<ImageFormat>, ) -> DepthImage
Create a new DepthImage
.
sourcepub fn update_fields() -> DepthImage
pub fn update_fields() -> DepthImage
Update only some specific fields of a DepthImage
.
sourcepub fn clear_fields() -> DepthImage
pub fn clear_fields() -> DepthImage
Clear all the fields of a DepthImage
.
sourcepub fn columns<I>(
self,
_lengths: I,
) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
pub fn columns<I>( self, _lengths: I, ) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
Partitions the component data into multiple sub-batches.
Specifically, this transforms the existing SerializedComponentBatch
es data into SerializedComponentColumn
s
instead, via SerializedComponentBatch::partitioned
.
This makes it possible to use RecordingStream::send_columns
to send columnar data directly into Rerun.
The specified lengths
must sum to the total length of the component batch.
sourcepub fn columns_of_unit_batches(
self,
) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
pub fn columns_of_unit_batches( self, ) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
Helper to partition the component data into unit-length sub-batches.
This is semantically similar to calling Self::columns
with std::iter::take(1).repeat(n)
,
where n
is automatically guessed.
sourcepub fn with_buffer(self, buffer: impl Into<ImageBuffer>) -> DepthImage
pub fn with_buffer(self, buffer: impl Into<ImageBuffer>) -> DepthImage
The raw depth image data.
sourcepub fn with_many_buffer(
self,
buffer: impl IntoIterator<Item = impl Into<ImageBuffer>>,
) -> DepthImage
pub fn with_many_buffer( self, buffer: impl IntoIterator<Item = impl Into<ImageBuffer>>, ) -> DepthImage
This method makes it possible to pack multiple crate::components::ImageBuffer
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_buffer
should
be used when logging a single row’s worth of data.
sourcepub fn with_format(self, format: impl Into<ImageFormat>) -> DepthImage
pub fn with_format(self, format: impl Into<ImageFormat>) -> DepthImage
The format of the image.
sourcepub fn with_many_format(
self,
format: impl IntoIterator<Item = impl Into<ImageFormat>>,
) -> DepthImage
pub fn with_many_format( self, format: impl IntoIterator<Item = impl Into<ImageFormat>>, ) -> DepthImage
This method makes it possible to pack multiple crate::components::ImageFormat
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_format
should
be used when logging a single row’s worth of data.
sourcepub fn with_meter(self, meter: impl Into<DepthMeter>) -> DepthImage
pub fn with_meter(self, meter: impl Into<DepthMeter>) -> DepthImage
An optional floating point value that specifies how long a meter is in the native depth units.
For instance: with uint16, perhaps meter=1000 which would mean you have millimeter precision and a range of up to ~65 meters (2^16 / 1000).
Note that the only effect on 2D views is the physical depth values shown when hovering the image. In 3D views on the other hand, this affects where the points of the point cloud are placed.
sourcepub fn with_many_meter(
self,
meter: impl IntoIterator<Item = impl Into<DepthMeter>>,
) -> DepthImage
pub fn with_many_meter( self, meter: impl IntoIterator<Item = impl Into<DepthMeter>>, ) -> DepthImage
This method makes it possible to pack multiple crate::components::DepthMeter
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_meter
should
be used when logging a single row’s worth of data.
sourcepub fn with_colormap(self, colormap: impl Into<Colormap>) -> DepthImage
pub fn with_colormap(self, colormap: impl Into<Colormap>) -> DepthImage
Colormap to use for rendering the depth image.
If not set, the depth image will be rendered using the Turbo colormap.
sourcepub fn with_many_colormap(
self,
colormap: impl IntoIterator<Item = impl Into<Colormap>>,
) -> DepthImage
pub fn with_many_colormap( self, colormap: impl IntoIterator<Item = impl Into<Colormap>>, ) -> DepthImage
This method makes it possible to pack multiple crate::components::Colormap
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_colormap
should
be used when logging a single row’s worth of data.
sourcepub fn with_depth_range(self, depth_range: impl Into<ValueRange>) -> DepthImage
pub fn with_depth_range(self, depth_range: impl Into<ValueRange>) -> DepthImage
The expected range of depth values.
This is typically the expected range of valid values. Everything outside of the range is clamped to the range for the purpose of colormpaping. Note that point clouds generated from this image will still display all points, regardless of this range.
If not specified, the range will be automatically estimated from the data. Note that the Viewer may try to guess a wider range than the minimum/maximum of values in the contents of the depth image. E.g. if all values are positive, some bigger than 1.0 and all smaller than 255.0, the Viewer will guess that the data likely came from an 8bit image, thus assuming a range of 0-255.
sourcepub fn with_many_depth_range(
self,
depth_range: impl IntoIterator<Item = impl Into<ValueRange>>,
) -> DepthImage
pub fn with_many_depth_range( self, depth_range: impl IntoIterator<Item = impl Into<ValueRange>>, ) -> DepthImage
This method makes it possible to pack multiple crate::components::ValueRange
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_depth_range
should
be used when logging a single row’s worth of data.
sourcepub fn with_point_fill_ratio(
self,
point_fill_ratio: impl Into<FillRatio>,
) -> DepthImage
pub fn with_point_fill_ratio( self, point_fill_ratio: impl Into<FillRatio>, ) -> DepthImage
Scale the radii of the points in the point cloud generated from this image.
A fill ratio of 1.0 (the default) means that each point is as big as to touch the center of its neighbor if it is at the same depth, leaving no gaps. A fill ratio of 0.5 means that each point touches the edge of its neighbor if it has the same depth.
TODO(#6744): This applies only to 3D views!
sourcepub fn with_many_point_fill_ratio(
self,
point_fill_ratio: impl IntoIterator<Item = impl Into<FillRatio>>,
) -> DepthImage
pub fn with_many_point_fill_ratio( self, point_fill_ratio: impl IntoIterator<Item = impl Into<FillRatio>>, ) -> DepthImage
This method makes it possible to pack multiple crate::components::FillRatio
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_point_fill_ratio
should
be used when logging a single row’s worth of data.
sourcepub fn with_draw_order(self, draw_order: impl Into<DrawOrder>) -> DepthImage
pub fn with_draw_order(self, draw_order: impl Into<DrawOrder>) -> DepthImage
An optional floating point value that specifies the 2D drawing order, used only if the depth image is shown as a 2D image.
Objects with higher values are drawn on top of those with lower values.
sourcepub fn with_many_draw_order(
self,
draw_order: impl IntoIterator<Item = impl Into<DrawOrder>>,
) -> DepthImage
pub fn with_many_draw_order( self, draw_order: impl IntoIterator<Item = impl Into<DrawOrder>>, ) -> DepthImage
This method makes it possible to pack multiple crate::components::DrawOrder
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_draw_order
should
be used when logging a single row’s worth of data.
source§impl DepthImage
impl DepthImage
sourcepub fn try_from<T>(data: T) -> Result<DepthImage, ImageConstructionError<T>>
pub fn try_from<T>(data: T) -> Result<DepthImage, ImageConstructionError<T>>
Try to construct a DepthImage
from anything that can be converted into TensorData
Will return an ImageConstructionError
if the shape of the tensor data is invalid
for treating as an image.
This is useful for constructing a DepthImage
from an ndarray.
sourcepub fn from_data_type_and_bytes(
bytes: impl Into<ImageBuffer>,
_: [u32; 2],
datatype: ChannelDatatype,
) -> DepthImage
pub fn from_data_type_and_bytes( bytes: impl Into<ImageBuffer>, _: [u32; 2], datatype: ChannelDatatype, ) -> DepthImage
Construct a depth image from a byte buffer given its resolution, and data type.
sourcepub fn from_gray16(
bytes: impl Into<ImageBuffer>,
resolution: [u32; 2],
) -> DepthImage
pub fn from_gray16( bytes: impl Into<ImageBuffer>, resolution: [u32; 2], ) -> DepthImage
From an 16-bit grayscale image.
Trait Implementations§
source§impl Archetype for DepthImage
impl Archetype for DepthImage
§type Indicator = GenericIndicatorComponent<DepthImage>
type Indicator = GenericIndicatorComponent<DepthImage>
source§fn name() -> ArchetypeName
fn name() -> ArchetypeName
rerun.archetypes.Points2D
.source§fn display_name() -> &'static str
fn display_name() -> &'static str
source§fn required_components() -> Cow<'static, [ComponentDescriptor]>
fn required_components() -> Cow<'static, [ComponentDescriptor]>
source§fn recommended_components() -> Cow<'static, [ComponentDescriptor]>
fn recommended_components() -> Cow<'static, [ComponentDescriptor]>
source§fn optional_components() -> Cow<'static, [ComponentDescriptor]>
fn optional_components() -> Cow<'static, [ComponentDescriptor]>
source§fn all_components() -> Cow<'static, [ComponentDescriptor]>
fn all_components() -> Cow<'static, [ComponentDescriptor]>
source§fn from_arrow_components(
arrow_data: impl IntoIterator<Item = (ComponentDescriptor, Arc<dyn Array>)>,
) -> Result<DepthImage, DeserializationError>
fn from_arrow_components( arrow_data: impl IntoIterator<Item = (ComponentDescriptor, Arc<dyn Array>)>, ) -> Result<DepthImage, DeserializationError>
ComponentNames
, deserializes them
into this archetype. Read moresource§fn from_arrow(
data: impl IntoIterator<Item = (Field, Arc<dyn Array>)>,
) -> Result<Self, DeserializationError>where
Self: Sized,
fn from_arrow(
data: impl IntoIterator<Item = (Field, Arc<dyn Array>)>,
) -> Result<Self, DeserializationError>where
Self: Sized,
source§impl AsComponents for DepthImage
impl AsComponents for DepthImage
source§fn as_serialized_batches(&self) -> Vec<SerializedComponentBatch>
fn as_serialized_batches(&self) -> Vec<SerializedComponentBatch>
SerializedComponentBatch
es. Read moresource§impl Clone for DepthImage
impl Clone for DepthImage
source§fn clone(&self) -> DepthImage
fn clone(&self) -> DepthImage
1.0.0 · source§fn clone_from(&mut self, source: &Self)
fn clone_from(&mut self, source: &Self)
source
. Read moresource§impl Debug for DepthImage
impl Debug for DepthImage
source§impl Default for DepthImage
impl Default for DepthImage
source§fn default() -> DepthImage
fn default() -> DepthImage
source§impl PartialEq for DepthImage
impl PartialEq for DepthImage
source§fn eq(&self, other: &DepthImage) -> bool
fn eq(&self, other: &DepthImage) -> bool
self
and other
values to be equal, and is used
by ==
.source§impl SizeBytes for DepthImage
impl SizeBytes for DepthImage
source§fn heap_size_bytes(&self) -> u64
fn heap_size_bytes(&self) -> u64
self
uses on the heap. Read moresource§fn total_size_bytes(&self) -> u64
fn total_size_bytes(&self) -> u64
self
in bytes, accounting for both stack and heap space.source§fn stack_size_bytes(&self) -> u64
fn stack_size_bytes(&self) -> u64
self
on the stack, in bytes. Read moreimpl ArchetypeReflectionMarker for DepthImage
impl StructuralPartialEq for DepthImage
Auto Trait Implementations§
impl Freeze for DepthImage
impl !RefUnwindSafe for DepthImage
impl Send for DepthImage
impl Sync for DepthImage
impl Unpin for DepthImage
impl !UnwindSafe for DepthImage
Blanket Implementations§
source§impl<T> BorrowMut<T> for Twhere
T: ?Sized,
impl<T> BorrowMut<T> for Twhere
T: ?Sized,
source§fn borrow_mut(&mut self) -> &mut T
fn borrow_mut(&mut self) -> &mut T
source§impl<T> CheckedAs for T
impl<T> CheckedAs for T
source§fn checked_as<Dst>(self) -> Option<Dst>where
T: CheckedCast<Dst>,
fn checked_as<Dst>(self) -> Option<Dst>where
T: CheckedCast<Dst>,
source§impl<Src, Dst> CheckedCastFrom<Src> for Dstwhere
Src: CheckedCast<Dst>,
impl<Src, Dst> CheckedCastFrom<Src> for Dstwhere
Src: CheckedCast<Dst>,
source§fn checked_cast_from(src: Src) -> Option<Dst>
fn checked_cast_from(src: Src) -> Option<Dst>
source§impl<T> CloneToUninit for Twhere
T: Clone,
impl<T> CloneToUninit for Twhere
T: Clone,
source§default unsafe fn clone_to_uninit(&self, dst: *mut T)
default unsafe fn clone_to_uninit(&self, dst: *mut T)
clone_to_uninit
)§impl<T> Conv for T
impl<T> Conv for T
§impl<T> Downcast for Twhere
T: Any,
impl<T> Downcast for Twhere
T: Any,
§fn into_any(self: Box<T>) -> Box<dyn Any>
fn into_any(self: Box<T>) -> Box<dyn Any>
Box<dyn Trait>
(where Trait: Downcast
) to Box<dyn Any>
. Box<dyn Any>
can
then be further downcast
into Box<ConcreteType>
where ConcreteType
implements Trait
.§fn into_any_rc(self: Rc<T>) -> Rc<dyn Any>
fn into_any_rc(self: Rc<T>) -> Rc<dyn Any>
Rc<Trait>
(where Trait: Downcast
) to Rc<Any>
. Rc<Any>
can then be
further downcast
into Rc<ConcreteType>
where ConcreteType
implements Trait
.§fn as_any(&self) -> &(dyn Any + 'static)
fn as_any(&self) -> &(dyn Any + 'static)
&Trait
(where Trait: Downcast
) to &Any
. This is needed since Rust cannot
generate &Any
’s vtable from &Trait
’s.§fn as_any_mut(&mut self) -> &mut (dyn Any + 'static)
fn as_any_mut(&mut self) -> &mut (dyn Any + 'static)
&mut Trait
(where Trait: Downcast
) to &Any
. This is needed since Rust cannot
generate &mut Any
’s vtable from &mut Trait
’s.§impl<T> DowncastSync for T
impl<T> DowncastSync for T
§impl<T> Instrument for T
impl<T> Instrument for T
§fn instrument(self, span: Span) -> Instrumented<Self>
fn instrument(self, span: Span) -> Instrumented<Self>
§fn in_current_span(self) -> Instrumented<Self>
fn in_current_span(self) -> Instrumented<Self>
source§impl<T> IntoEither for T
impl<T> IntoEither for T
source§fn into_either(self, into_left: bool) -> Either<Self, Self>
fn into_either(self, into_left: bool) -> Either<Self, Self>
self
into a Left
variant of Either<Self, Self>
if into_left
is true
.
Converts self
into a Right
variant of Either<Self, Self>
otherwise. Read moresource§fn into_either_with<F>(self, into_left: F) -> Either<Self, Self>
fn into_either_with<F>(self, into_left: F) -> Either<Self, Self>
self
into a Left
variant of Either<Self, Self>
if into_left(&self)
returns true
.
Converts self
into a Right
variant of Either<Self, Self>
otherwise. Read moresource§impl<T> IntoRequest<T> for T
impl<T> IntoRequest<T> for T
source§fn into_request(self) -> Request<T>
fn into_request(self) -> Request<T>
T
in a tonic::Request
source§impl<Src, Dst> LosslessTryInto<Dst> for Srcwhere
Dst: LosslessTryFrom<Src>,
impl<Src, Dst> LosslessTryInto<Dst> for Srcwhere
Dst: LosslessTryFrom<Src>,
source§fn lossless_try_into(self) -> Option<Dst>
fn lossless_try_into(self) -> Option<Dst>
source§impl<Src, Dst> LossyInto<Dst> for Srcwhere
Dst: LossyFrom<Src>,
impl<Src, Dst> LossyInto<Dst> for Srcwhere
Dst: LossyFrom<Src>,
source§fn lossy_into(self) -> Dst
fn lossy_into(self) -> Dst
§impl<T> NoneValue for Twhere
T: Default,
impl<T> NoneValue for Twhere
T: Default,
type NoneType = T
§fn null_value() -> T
fn null_value() -> T
source§impl<T> OverflowingAs for T
impl<T> OverflowingAs for T
source§fn overflowing_as<Dst>(self) -> (Dst, bool)where
T: OverflowingCast<Dst>,
fn overflowing_as<Dst>(self) -> (Dst, bool)where
T: OverflowingCast<Dst>,
source§impl<Src, Dst> OverflowingCastFrom<Src> for Dstwhere
Src: OverflowingCast<Dst>,
impl<Src, Dst> OverflowingCastFrom<Src> for Dstwhere
Src: OverflowingCast<Dst>,
source§fn overflowing_cast_from(src: Src) -> (Dst, bool)
fn overflowing_cast_from(src: Src) -> (Dst, bool)
§impl<T> Pipe for Twhere
T: ?Sized,
impl<T> Pipe for Twhere
T: ?Sized,
§fn pipe<R>(self, func: impl FnOnce(Self) -> R) -> Rwhere
Self: Sized,
fn pipe<R>(self, func: impl FnOnce(Self) -> R) -> Rwhere
Self: Sized,
§fn pipe_ref<'a, R>(&'a self, func: impl FnOnce(&'a Self) -> R) -> Rwhere
R: 'a,
fn pipe_ref<'a, R>(&'a self, func: impl FnOnce(&'a Self) -> R) -> Rwhere
R: 'a,
self
and passes that borrow into the pipe function. Read more§fn pipe_ref_mut<'a, R>(&'a mut self, func: impl FnOnce(&'a mut Self) -> R) -> Rwhere
R: 'a,
fn pipe_ref_mut<'a, R>(&'a mut self, func: impl FnOnce(&'a mut Self) -> R) -> Rwhere
R: 'a,
self
and passes that borrow into the pipe function. Read more§fn pipe_borrow<'a, B, R>(&'a self, func: impl FnOnce(&'a B) -> R) -> R
fn pipe_borrow<'a, B, R>(&'a self, func: impl FnOnce(&'a B) -> R) -> R
§fn pipe_borrow_mut<'a, B, R>(
&'a mut self,
func: impl FnOnce(&'a mut B) -> R,
) -> R
fn pipe_borrow_mut<'a, B, R>( &'a mut self, func: impl FnOnce(&'a mut B) -> R, ) -> R
§fn pipe_as_ref<'a, U, R>(&'a self, func: impl FnOnce(&'a U) -> R) -> R
fn pipe_as_ref<'a, U, R>(&'a self, func: impl FnOnce(&'a U) -> R) -> R
self
, then passes self.as_ref()
into the pipe function.§fn pipe_as_mut<'a, U, R>(&'a mut self, func: impl FnOnce(&'a mut U) -> R) -> R
fn pipe_as_mut<'a, U, R>(&'a mut self, func: impl FnOnce(&'a mut U) -> R) -> R
self
, then passes self.as_mut()
into the pipe
function.§fn pipe_deref<'a, T, R>(&'a self, func: impl FnOnce(&'a T) -> R) -> R
fn pipe_deref<'a, T, R>(&'a self, func: impl FnOnce(&'a T) -> R) -> R
self
, then passes self.deref()
into the pipe function.§impl<T> Pointable for T
impl<T> Pointable for T
source§impl<T> SaturatingAs for T
impl<T> SaturatingAs for T
source§fn saturating_as<Dst>(self) -> Dstwhere
T: SaturatingCast<Dst>,
fn saturating_as<Dst>(self) -> Dstwhere
T: SaturatingCast<Dst>,
source§impl<Src, Dst> SaturatingCastFrom<Src> for Dstwhere
Src: SaturatingCast<Dst>,
impl<Src, Dst> SaturatingCastFrom<Src> for Dstwhere
Src: SaturatingCast<Dst>,
source§fn saturating_cast_from(src: Src) -> Dst
fn saturating_cast_from(src: Src) -> Dst
§impl<T> Tap for T
impl<T> Tap for T
§fn tap_borrow<B>(self, func: impl FnOnce(&B)) -> Self
fn tap_borrow<B>(self, func: impl FnOnce(&B)) -> Self
Borrow<B>
of a value. Read more§fn tap_borrow_mut<B>(self, func: impl FnOnce(&mut B)) -> Self
fn tap_borrow_mut<B>(self, func: impl FnOnce(&mut B)) -> Self
BorrowMut<B>
of a value. Read more§fn tap_ref<R>(self, func: impl FnOnce(&R)) -> Self
fn tap_ref<R>(self, func: impl FnOnce(&R)) -> Self
AsRef<R>
view of a value. Read more§fn tap_ref_mut<R>(self, func: impl FnOnce(&mut R)) -> Self
fn tap_ref_mut<R>(self, func: impl FnOnce(&mut R)) -> Self
AsMut<R>
view of a value. Read more§fn tap_deref<T>(self, func: impl FnOnce(&T)) -> Self
fn tap_deref<T>(self, func: impl FnOnce(&T)) -> Self
Deref::Target
of a value. Read more§fn tap_deref_mut<T>(self, func: impl FnOnce(&mut T)) -> Self
fn tap_deref_mut<T>(self, func: impl FnOnce(&mut T)) -> Self
Deref::Target
of a value. Read more§fn tap_dbg(self, func: impl FnOnce(&Self)) -> Self
fn tap_dbg(self, func: impl FnOnce(&Self)) -> Self
.tap()
only in debug builds, and is erased in release builds.§fn tap_mut_dbg(self, func: impl FnOnce(&mut Self)) -> Self
fn tap_mut_dbg(self, func: impl FnOnce(&mut Self)) -> Self
.tap_mut()
only in debug builds, and is erased in release
builds.§fn tap_borrow_dbg<B>(self, func: impl FnOnce(&B)) -> Self
fn tap_borrow_dbg<B>(self, func: impl FnOnce(&B)) -> Self
.tap_borrow()
only in debug builds, and is erased in release
builds.§fn tap_borrow_mut_dbg<B>(self, func: impl FnOnce(&mut B)) -> Self
fn tap_borrow_mut_dbg<B>(self, func: impl FnOnce(&mut B)) -> Self
.tap_borrow_mut()
only in debug builds, and is erased in release
builds.§fn tap_ref_dbg<R>(self, func: impl FnOnce(&R)) -> Self
fn tap_ref_dbg<R>(self, func: impl FnOnce(&R)) -> Self
.tap_ref()
only in debug builds, and is erased in release
builds.§fn tap_ref_mut_dbg<R>(self, func: impl FnOnce(&mut R)) -> Self
fn tap_ref_mut_dbg<R>(self, func: impl FnOnce(&mut R)) -> Self
.tap_ref_mut()
only in debug builds, and is erased in release
builds.§fn tap_deref_dbg<T>(self, func: impl FnOnce(&T)) -> Self
fn tap_deref_dbg<T>(self, func: impl FnOnce(&T)) -> Self
.tap_deref()
only in debug builds, and is erased in release
builds.