Struct rerun::sdk::prelude::Ellipsoids3D
source · pub struct Ellipsoids3D {
pub half_sizes: Option<SerializedComponentBatch>,
pub centers: Option<SerializedComponentBatch>,
pub rotation_axis_angles: Option<SerializedComponentBatch>,
pub quaternions: Option<SerializedComponentBatch>,
pub colors: Option<SerializedComponentBatch>,
pub line_radii: Option<SerializedComponentBatch>,
pub fill_mode: Option<SerializedComponentBatch>,
pub labels: Option<SerializedComponentBatch>,
pub show_labels: Option<SerializedComponentBatch>,
pub class_ids: Option<SerializedComponentBatch>,
}
Expand description
Archetype: 3D ellipsoids or spheres.
This archetype is for ellipsoids or spheres whose size is a key part of the data
(e.g. a bounding sphere).
For points whose radii are for the sake of visualization, use archetypes::Points3D
instead.
Note that orienting and placing the ellipsoids/spheres is handled via [archetypes.InstancePoses3D]
.
Some of its component are repeated here for convenience.
If there’s more instance poses than half sizes, the last half size will be repeated for the remaining poses.
§Example
§Covariance ellipsoid
use rand::distributions::Distribution;
fn main() -> Result<(), Box<dyn std::error::Error>> {
let rec = rerun::RecordingStreamBuilder::new("rerun_example_ellipsoid_simple").spawn()?;
let sigmas: [f32; 3] = [5., 3., 1.];
let mut rng = rand::thread_rng();
let normal = rand_distr::Normal::new(0.0, 1.0)?;
rec.log(
"points",
&rerun::Points3D::new((0..50_000).map(|_| {
(
sigmas[0] * normal.sample(&mut rng),
sigmas[1] * normal.sample(&mut rng),
sigmas[2] * normal.sample(&mut rng),
)
}))
.with_radii([0.02])
.with_colors([rerun::Color::from_rgb(188, 77, 185)]),
)?;
rec.log(
"ellipsoid",
&rerun::Ellipsoids3D::from_centers_and_half_sizes(
[(0.0, 0.0, 0.0), (0.0, 0.0, 0.0)],
[sigmas, [sigmas[0] * 3., sigmas[1] * 3., sigmas[2] * 3.]],
)
.with_colors([
rerun::Color::from_rgb(255, 255, 0),
rerun::Color::from_rgb(64, 64, 0),
]),
)?;
Ok(())
}
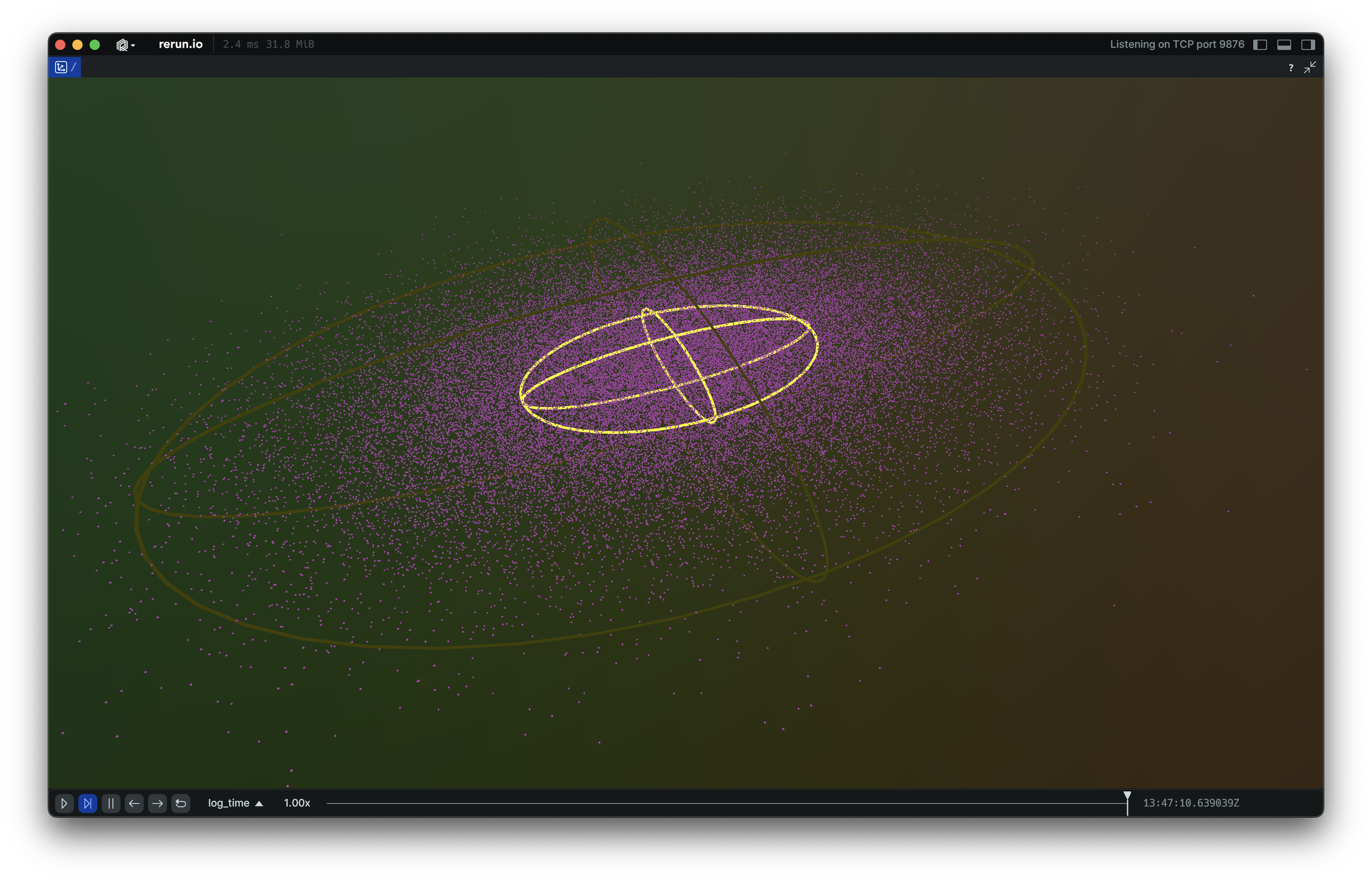
Fields§
§half_sizes: Option<SerializedComponentBatch>
For each ellipsoid, half of its size on its three axes.
If all components are equal, then it is a sphere with that radius.
centers: Option<SerializedComponentBatch>
Optional center positions of the ellipsoids.
If not specified, the centers will be at (0, 0, 0).
Note that this uses a components::PoseTranslation3D
which is also used by archetypes::InstancePoses3D
.
rotation_axis_angles: Option<SerializedComponentBatch>
Rotations via axis + angle.
If no rotation is specified, the axes of the ellipsoid align with the axes of the local coordinate system.
Note that this uses a components::PoseRotationAxisAngle
which is also used by archetypes::InstancePoses3D
.
quaternions: Option<SerializedComponentBatch>
Rotations via quaternion.
If no rotation is specified, the axes of the ellipsoid align with the axes of the local coordinate system.
Note that this uses a components::PoseRotationQuat
which is also used by archetypes::InstancePoses3D
.
colors: Option<SerializedComponentBatch>
Optional colors for the ellipsoids.
line_radii: Option<SerializedComponentBatch>
Optional radii for the lines used when the ellipsoid is rendered as a wireframe.
fill_mode: Option<SerializedComponentBatch>
Optionally choose whether the ellipsoids are drawn with lines or solid.
labels: Option<SerializedComponentBatch>
Optional text labels for the ellipsoids.
show_labels: Option<SerializedComponentBatch>
Optional choice of whether the text labels should be shown by default.
class_ids: Option<SerializedComponentBatch>
Optional class ID for the ellipsoids.
The class ID provides colors and labels if not specified explicitly.
Implementations§
source§impl Ellipsoids3D
impl Ellipsoids3D
sourcepub fn descriptor_half_sizes() -> ComponentDescriptor
pub fn descriptor_half_sizes() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::half_sizes
.
sourcepub fn descriptor_centers() -> ComponentDescriptor
pub fn descriptor_centers() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::centers
.
sourcepub fn descriptor_rotation_axis_angles() -> ComponentDescriptor
pub fn descriptor_rotation_axis_angles() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::rotation_axis_angles
.
sourcepub fn descriptor_quaternions() -> ComponentDescriptor
pub fn descriptor_quaternions() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::quaternions
.
sourcepub fn descriptor_colors() -> ComponentDescriptor
pub fn descriptor_colors() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::colors
.
sourcepub fn descriptor_line_radii() -> ComponentDescriptor
pub fn descriptor_line_radii() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::line_radii
.
sourcepub fn descriptor_fill_mode() -> ComponentDescriptor
pub fn descriptor_fill_mode() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::fill_mode
.
sourcepub fn descriptor_labels() -> ComponentDescriptor
pub fn descriptor_labels() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::labels
.
sourcepub fn descriptor_show_labels() -> ComponentDescriptor
pub fn descriptor_show_labels() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::show_labels
.
sourcepub fn descriptor_class_ids() -> ComponentDescriptor
pub fn descriptor_class_ids() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::class_ids
.
sourcepub fn descriptor_indicator() -> ComponentDescriptor
pub fn descriptor_indicator() -> ComponentDescriptor
Returns the ComponentDescriptor
for the associated indicator component.
source§impl Ellipsoids3D
impl Ellipsoids3D
sourcepub const NUM_COMPONENTS: usize = 11usize
pub const NUM_COMPONENTS: usize = 11usize
The total number of components in the archetype: 1 required, 3 recommended, 7 optional
source§impl Ellipsoids3D
impl Ellipsoids3D
sourcepub fn update_fields() -> Ellipsoids3D
pub fn update_fields() -> Ellipsoids3D
Update only some specific fields of a Ellipsoids3D
.
sourcepub fn clear_fields() -> Ellipsoids3D
pub fn clear_fields() -> Ellipsoids3D
Clear all the fields of a Ellipsoids3D
.
sourcepub fn columns<I>(
self,
_lengths: I,
) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
pub fn columns<I>( self, _lengths: I, ) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
Partitions the component data into multiple sub-batches.
Specifically, this transforms the existing SerializedComponentBatch
es data into SerializedComponentColumn
s
instead, via SerializedComponentBatch::partitioned
.
This makes it possible to use RecordingStream::send_columns
to send columnar data directly into Rerun.
The specified lengths
must sum to the total length of the component batch.
sourcepub fn columns_of_unit_batches(
self,
) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
pub fn columns_of_unit_batches( self, ) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
Helper to partition the component data into unit-length sub-batches.
This is semantically similar to calling Self::columns
with std::iter::take(1).repeat(n)
,
where n
is automatically guessed.
sourcepub fn with_half_sizes(
self,
half_sizes: impl IntoIterator<Item = impl Into<HalfSize3D>>,
) -> Ellipsoids3D
pub fn with_half_sizes( self, half_sizes: impl IntoIterator<Item = impl Into<HalfSize3D>>, ) -> Ellipsoids3D
For each ellipsoid, half of its size on its three axes.
If all components are equal, then it is a sphere with that radius.
sourcepub fn with_centers(
self,
centers: impl IntoIterator<Item = impl Into<PoseTranslation3D>>,
) -> Ellipsoids3D
pub fn with_centers( self, centers: impl IntoIterator<Item = impl Into<PoseTranslation3D>>, ) -> Ellipsoids3D
Optional center positions of the ellipsoids.
If not specified, the centers will be at (0, 0, 0).
Note that this uses a components::PoseTranslation3D
which is also used by archetypes::InstancePoses3D
.
sourcepub fn with_rotation_axis_angles(
self,
rotation_axis_angles: impl IntoIterator<Item = impl Into<PoseRotationAxisAngle>>,
) -> Ellipsoids3D
pub fn with_rotation_axis_angles( self, rotation_axis_angles: impl IntoIterator<Item = impl Into<PoseRotationAxisAngle>>, ) -> Ellipsoids3D
Rotations via axis + angle.
If no rotation is specified, the axes of the ellipsoid align with the axes of the local coordinate system.
Note that this uses a components::PoseRotationAxisAngle
which is also used by archetypes::InstancePoses3D
.
sourcepub fn with_quaternions(
self,
quaternions: impl IntoIterator<Item = impl Into<PoseRotationQuat>>,
) -> Ellipsoids3D
pub fn with_quaternions( self, quaternions: impl IntoIterator<Item = impl Into<PoseRotationQuat>>, ) -> Ellipsoids3D
Rotations via quaternion.
If no rotation is specified, the axes of the ellipsoid align with the axes of the local coordinate system.
Note that this uses a components::PoseRotationQuat
which is also used by archetypes::InstancePoses3D
.
sourcepub fn with_colors(
self,
colors: impl IntoIterator<Item = impl Into<Color>>,
) -> Ellipsoids3D
pub fn with_colors( self, colors: impl IntoIterator<Item = impl Into<Color>>, ) -> Ellipsoids3D
Optional colors for the ellipsoids.
sourcepub fn with_line_radii(
self,
line_radii: impl IntoIterator<Item = impl Into<Radius>>,
) -> Ellipsoids3D
pub fn with_line_radii( self, line_radii: impl IntoIterator<Item = impl Into<Radius>>, ) -> Ellipsoids3D
Optional radii for the lines used when the ellipsoid is rendered as a wireframe.
sourcepub fn with_fill_mode(self, fill_mode: impl Into<FillMode>) -> Ellipsoids3D
pub fn with_fill_mode(self, fill_mode: impl Into<FillMode>) -> Ellipsoids3D
Optionally choose whether the ellipsoids are drawn with lines or solid.
sourcepub fn with_many_fill_mode(
self,
fill_mode: impl IntoIterator<Item = impl Into<FillMode>>,
) -> Ellipsoids3D
pub fn with_many_fill_mode( self, fill_mode: impl IntoIterator<Item = impl Into<FillMode>>, ) -> Ellipsoids3D
This method makes it possible to pack multiple crate::components::FillMode
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_fill_mode
should
be used when logging a single row’s worth of data.
sourcepub fn with_labels(
self,
labels: impl IntoIterator<Item = impl Into<Text>>,
) -> Ellipsoids3D
pub fn with_labels( self, labels: impl IntoIterator<Item = impl Into<Text>>, ) -> Ellipsoids3D
Optional text labels for the ellipsoids.
sourcepub fn with_show_labels(
self,
show_labels: impl Into<ShowLabels>,
) -> Ellipsoids3D
pub fn with_show_labels( self, show_labels: impl Into<ShowLabels>, ) -> Ellipsoids3D
Optional choice of whether the text labels should be shown by default.
sourcepub fn with_many_show_labels(
self,
show_labels: impl IntoIterator<Item = impl Into<ShowLabels>>,
) -> Ellipsoids3D
pub fn with_many_show_labels( self, show_labels: impl IntoIterator<Item = impl Into<ShowLabels>>, ) -> Ellipsoids3D
This method makes it possible to pack multiple crate::components::ShowLabels
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_show_labels
should
be used when logging a single row’s worth of data.
sourcepub fn with_class_ids(
self,
class_ids: impl IntoIterator<Item = impl Into<ClassId>>,
) -> Ellipsoids3D
pub fn with_class_ids( self, class_ids: impl IntoIterator<Item = impl Into<ClassId>>, ) -> Ellipsoids3D
Optional class ID for the ellipsoids.
The class ID provides colors and labels if not specified explicitly.
source§impl Ellipsoids3D
impl Ellipsoids3D
sourcepub fn from_radii(radii: impl IntoIterator<Item = f32>) -> Ellipsoids3D
pub fn from_radii(radii: impl IntoIterator<Item = f32>) -> Ellipsoids3D
Creates a new Ellipsoids3D
for spheres with the given radii.
sourcepub fn from_centers_and_radii(
centers: impl IntoIterator<Item = impl Into<PoseTranslation3D>>,
radii: impl IntoIterator<Item = f32>,
) -> Ellipsoids3D
pub fn from_centers_and_radii( centers: impl IntoIterator<Item = impl Into<PoseTranslation3D>>, radii: impl IntoIterator<Item = f32>, ) -> Ellipsoids3D
Creates a new Ellipsoids3D
for spheres with the given Self::centers
, and
Self::half_sizes
all equal to the given radii.
sourcepub fn from_half_sizes(
half_sizes: impl IntoIterator<Item = impl Into<HalfSize3D>>,
) -> Ellipsoids3D
pub fn from_half_sizes( half_sizes: impl IntoIterator<Item = impl Into<HalfSize3D>>, ) -> Ellipsoids3D
Creates a new Ellipsoids3D
with Self::half_sizes
.
sourcepub fn from_centers_and_half_sizes(
centers: impl IntoIterator<Item = impl Into<PoseTranslation3D>>,
half_sizes: impl IntoIterator<Item = impl Into<HalfSize3D>>,
) -> Ellipsoids3D
pub fn from_centers_and_half_sizes( centers: impl IntoIterator<Item = impl Into<PoseTranslation3D>>, half_sizes: impl IntoIterator<Item = impl Into<HalfSize3D>>, ) -> Ellipsoids3D
Creates a new Ellipsoids3D
with Self::centers
and Self::half_sizes
.
Trait Implementations§
source§impl Archetype for Ellipsoids3D
impl Archetype for Ellipsoids3D
§type Indicator = GenericIndicatorComponent<Ellipsoids3D>
type Indicator = GenericIndicatorComponent<Ellipsoids3D>
source§fn name() -> ArchetypeName
fn name() -> ArchetypeName
rerun.archetypes.Points2D
.source§fn display_name() -> &'static str
fn display_name() -> &'static str
source§fn required_components() -> Cow<'static, [ComponentDescriptor]>
fn required_components() -> Cow<'static, [ComponentDescriptor]>
source§fn recommended_components() -> Cow<'static, [ComponentDescriptor]>
fn recommended_components() -> Cow<'static, [ComponentDescriptor]>
source§fn optional_components() -> Cow<'static, [ComponentDescriptor]>
fn optional_components() -> Cow<'static, [ComponentDescriptor]>
source§fn all_components() -> Cow<'static, [ComponentDescriptor]>
fn all_components() -> Cow<'static, [ComponentDescriptor]>
source§fn from_arrow_components(
arrow_data: impl IntoIterator<Item = (ComponentDescriptor, Arc<dyn Array>)>,
) -> Result<Ellipsoids3D, DeserializationError>
fn from_arrow_components( arrow_data: impl IntoIterator<Item = (ComponentDescriptor, Arc<dyn Array>)>, ) -> Result<Ellipsoids3D, DeserializationError>
ComponentNames
, deserializes them
into this archetype. Read moresource§fn from_arrow(
data: impl IntoIterator<Item = (Field, Arc<dyn Array>)>,
) -> Result<Self, DeserializationError>where
Self: Sized,
fn from_arrow(
data: impl IntoIterator<Item = (Field, Arc<dyn Array>)>,
) -> Result<Self, DeserializationError>where
Self: Sized,
source§impl AsComponents for Ellipsoids3D
impl AsComponents for Ellipsoids3D
source§fn as_serialized_batches(&self) -> Vec<SerializedComponentBatch>
fn as_serialized_batches(&self) -> Vec<SerializedComponentBatch>
SerializedComponentBatch
es. Read moresource§impl Clone for Ellipsoids3D
impl Clone for Ellipsoids3D
source§fn clone(&self) -> Ellipsoids3D
fn clone(&self) -> Ellipsoids3D
1.0.0 · source§fn clone_from(&mut self, source: &Self)
fn clone_from(&mut self, source: &Self)
source
. Read moresource§impl Debug for Ellipsoids3D
impl Debug for Ellipsoids3D
source§impl Default for Ellipsoids3D
impl Default for Ellipsoids3D
source§fn default() -> Ellipsoids3D
fn default() -> Ellipsoids3D
source§impl PartialEq for Ellipsoids3D
impl PartialEq for Ellipsoids3D
source§fn eq(&self, other: &Ellipsoids3D) -> bool
fn eq(&self, other: &Ellipsoids3D) -> bool
self
and other
values to be equal, and is used
by ==
.source§impl SizeBytes for Ellipsoids3D
impl SizeBytes for Ellipsoids3D
source§fn heap_size_bytes(&self) -> u64
fn heap_size_bytes(&self) -> u64
self
uses on the heap. Read moresource§fn total_size_bytes(&self) -> u64
fn total_size_bytes(&self) -> u64
self
in bytes, accounting for both stack and heap space.source§fn stack_size_bytes(&self) -> u64
fn stack_size_bytes(&self) -> u64
self
on the stack, in bytes. Read moreimpl ArchetypeReflectionMarker for Ellipsoids3D
impl StructuralPartialEq for Ellipsoids3D
Auto Trait Implementations§
impl Freeze for Ellipsoids3D
impl !RefUnwindSafe for Ellipsoids3D
impl Send for Ellipsoids3D
impl Sync for Ellipsoids3D
impl Unpin for Ellipsoids3D
impl !UnwindSafe for Ellipsoids3D
Blanket Implementations§
source§impl<T> BorrowMut<T> for Twhere
T: ?Sized,
impl<T> BorrowMut<T> for Twhere
T: ?Sized,
source§fn borrow_mut(&mut self) -> &mut T
fn borrow_mut(&mut self) -> &mut T
source§impl<T> CheckedAs for T
impl<T> CheckedAs for T
source§fn checked_as<Dst>(self) -> Option<Dst>where
T: CheckedCast<Dst>,
fn checked_as<Dst>(self) -> Option<Dst>where
T: CheckedCast<Dst>,
source§impl<Src, Dst> CheckedCastFrom<Src> for Dstwhere
Src: CheckedCast<Dst>,
impl<Src, Dst> CheckedCastFrom<Src> for Dstwhere
Src: CheckedCast<Dst>,
source§fn checked_cast_from(src: Src) -> Option<Dst>
fn checked_cast_from(src: Src) -> Option<Dst>
source§impl<T> CloneToUninit for Twhere
T: Clone,
impl<T> CloneToUninit for Twhere
T: Clone,
source§default unsafe fn clone_to_uninit(&self, dst: *mut T)
default unsafe fn clone_to_uninit(&self, dst: *mut T)
clone_to_uninit
)§impl<T> Conv for T
impl<T> Conv for T
§impl<T> Downcast for Twhere
T: Any,
impl<T> Downcast for Twhere
T: Any,
§fn into_any(self: Box<T>) -> Box<dyn Any>
fn into_any(self: Box<T>) -> Box<dyn Any>
Box<dyn Trait>
(where Trait: Downcast
) to Box<dyn Any>
. Box<dyn Any>
can
then be further downcast
into Box<ConcreteType>
where ConcreteType
implements Trait
.§fn into_any_rc(self: Rc<T>) -> Rc<dyn Any>
fn into_any_rc(self: Rc<T>) -> Rc<dyn Any>
Rc<Trait>
(where Trait: Downcast
) to Rc<Any>
. Rc<Any>
can then be
further downcast
into Rc<ConcreteType>
where ConcreteType
implements Trait
.§fn as_any(&self) -> &(dyn Any + 'static)
fn as_any(&self) -> &(dyn Any + 'static)
&Trait
(where Trait: Downcast
) to &Any
. This is needed since Rust cannot
generate &Any
’s vtable from &Trait
’s.§fn as_any_mut(&mut self) -> &mut (dyn Any + 'static)
fn as_any_mut(&mut self) -> &mut (dyn Any + 'static)
&mut Trait
(where Trait: Downcast
) to &Any
. This is needed since Rust cannot
generate &mut Any
’s vtable from &mut Trait
’s.§impl<T> DowncastSync for T
impl<T> DowncastSync for T
§impl<T> Instrument for T
impl<T> Instrument for T
§fn instrument(self, span: Span) -> Instrumented<Self>
fn instrument(self, span: Span) -> Instrumented<Self>
§fn in_current_span(self) -> Instrumented<Self>
fn in_current_span(self) -> Instrumented<Self>
source§impl<T> IntoEither for T
impl<T> IntoEither for T
source§fn into_either(self, into_left: bool) -> Either<Self, Self>
fn into_either(self, into_left: bool) -> Either<Self, Self>
self
into a Left
variant of Either<Self, Self>
if into_left
is true
.
Converts self
into a Right
variant of Either<Self, Self>
otherwise. Read moresource§fn into_either_with<F>(self, into_left: F) -> Either<Self, Self>
fn into_either_with<F>(self, into_left: F) -> Either<Self, Self>
self
into a Left
variant of Either<Self, Self>
if into_left(&self)
returns true
.
Converts self
into a Right
variant of Either<Self, Self>
otherwise. Read moresource§impl<T> IntoRequest<T> for T
impl<T> IntoRequest<T> for T
source§fn into_request(self) -> Request<T>
fn into_request(self) -> Request<T>
T
in a tonic::Request
source§impl<Src, Dst> LosslessTryInto<Dst> for Srcwhere
Dst: LosslessTryFrom<Src>,
impl<Src, Dst> LosslessTryInto<Dst> for Srcwhere
Dst: LosslessTryFrom<Src>,
source§fn lossless_try_into(self) -> Option<Dst>
fn lossless_try_into(self) -> Option<Dst>
source§impl<Src, Dst> LossyInto<Dst> for Srcwhere
Dst: LossyFrom<Src>,
impl<Src, Dst> LossyInto<Dst> for Srcwhere
Dst: LossyFrom<Src>,
source§fn lossy_into(self) -> Dst
fn lossy_into(self) -> Dst
§impl<T> NoneValue for Twhere
T: Default,
impl<T> NoneValue for Twhere
T: Default,
type NoneType = T
§fn null_value() -> T
fn null_value() -> T
source§impl<T> OverflowingAs for T
impl<T> OverflowingAs for T
source§fn overflowing_as<Dst>(self) -> (Dst, bool)where
T: OverflowingCast<Dst>,
fn overflowing_as<Dst>(self) -> (Dst, bool)where
T: OverflowingCast<Dst>,
source§impl<Src, Dst> OverflowingCastFrom<Src> for Dstwhere
Src: OverflowingCast<Dst>,
impl<Src, Dst> OverflowingCastFrom<Src> for Dstwhere
Src: OverflowingCast<Dst>,
source§fn overflowing_cast_from(src: Src) -> (Dst, bool)
fn overflowing_cast_from(src: Src) -> (Dst, bool)
§impl<T> Pipe for Twhere
T: ?Sized,
impl<T> Pipe for Twhere
T: ?Sized,
§fn pipe<R>(self, func: impl FnOnce(Self) -> R) -> Rwhere
Self: Sized,
fn pipe<R>(self, func: impl FnOnce(Self) -> R) -> Rwhere
Self: Sized,
§fn pipe_ref<'a, R>(&'a self, func: impl FnOnce(&'a Self) -> R) -> Rwhere
R: 'a,
fn pipe_ref<'a, R>(&'a self, func: impl FnOnce(&'a Self) -> R) -> Rwhere
R: 'a,
self
and passes that borrow into the pipe function. Read more§fn pipe_ref_mut<'a, R>(&'a mut self, func: impl FnOnce(&'a mut Self) -> R) -> Rwhere
R: 'a,
fn pipe_ref_mut<'a, R>(&'a mut self, func: impl FnOnce(&'a mut Self) -> R) -> Rwhere
R: 'a,
self
and passes that borrow into the pipe function. Read more§fn pipe_borrow<'a, B, R>(&'a self, func: impl FnOnce(&'a B) -> R) -> R
fn pipe_borrow<'a, B, R>(&'a self, func: impl FnOnce(&'a B) -> R) -> R
§fn pipe_borrow_mut<'a, B, R>(
&'a mut self,
func: impl FnOnce(&'a mut B) -> R,
) -> R
fn pipe_borrow_mut<'a, B, R>( &'a mut self, func: impl FnOnce(&'a mut B) -> R, ) -> R
§fn pipe_as_ref<'a, U, R>(&'a self, func: impl FnOnce(&'a U) -> R) -> R
fn pipe_as_ref<'a, U, R>(&'a self, func: impl FnOnce(&'a U) -> R) -> R
self
, then passes self.as_ref()
into the pipe function.§fn pipe_as_mut<'a, U, R>(&'a mut self, func: impl FnOnce(&'a mut U) -> R) -> R
fn pipe_as_mut<'a, U, R>(&'a mut self, func: impl FnOnce(&'a mut U) -> R) -> R
self
, then passes self.as_mut()
into the pipe
function.§fn pipe_deref<'a, T, R>(&'a self, func: impl FnOnce(&'a T) -> R) -> R
fn pipe_deref<'a, T, R>(&'a self, func: impl FnOnce(&'a T) -> R) -> R
self
, then passes self.deref()
into the pipe function.§impl<T> Pointable for T
impl<T> Pointable for T
source§impl<T> SaturatingAs for T
impl<T> SaturatingAs for T
source§fn saturating_as<Dst>(self) -> Dstwhere
T: SaturatingCast<Dst>,
fn saturating_as<Dst>(self) -> Dstwhere
T: SaturatingCast<Dst>,
source§impl<Src, Dst> SaturatingCastFrom<Src> for Dstwhere
Src: SaturatingCast<Dst>,
impl<Src, Dst> SaturatingCastFrom<Src> for Dstwhere
Src: SaturatingCast<Dst>,
source§fn saturating_cast_from(src: Src) -> Dst
fn saturating_cast_from(src: Src) -> Dst
§impl<T> Tap for T
impl<T> Tap for T
§fn tap_borrow<B>(self, func: impl FnOnce(&B)) -> Self
fn tap_borrow<B>(self, func: impl FnOnce(&B)) -> Self
Borrow<B>
of a value. Read more§fn tap_borrow_mut<B>(self, func: impl FnOnce(&mut B)) -> Self
fn tap_borrow_mut<B>(self, func: impl FnOnce(&mut B)) -> Self
BorrowMut<B>
of a value. Read more§fn tap_ref<R>(self, func: impl FnOnce(&R)) -> Self
fn tap_ref<R>(self, func: impl FnOnce(&R)) -> Self
AsRef<R>
view of a value. Read more§fn tap_ref_mut<R>(self, func: impl FnOnce(&mut R)) -> Self
fn tap_ref_mut<R>(self, func: impl FnOnce(&mut R)) -> Self
AsMut<R>
view of a value. Read more§fn tap_deref<T>(self, func: impl FnOnce(&T)) -> Self
fn tap_deref<T>(self, func: impl FnOnce(&T)) -> Self
Deref::Target
of a value. Read more§fn tap_deref_mut<T>(self, func: impl FnOnce(&mut T)) -> Self
fn tap_deref_mut<T>(self, func: impl FnOnce(&mut T)) -> Self
Deref::Target
of a value. Read more§fn tap_dbg(self, func: impl FnOnce(&Self)) -> Self
fn tap_dbg(self, func: impl FnOnce(&Self)) -> Self
.tap()
only in debug builds, and is erased in release builds.§fn tap_mut_dbg(self, func: impl FnOnce(&mut Self)) -> Self
fn tap_mut_dbg(self, func: impl FnOnce(&mut Self)) -> Self
.tap_mut()
only in debug builds, and is erased in release
builds.§fn tap_borrow_dbg<B>(self, func: impl FnOnce(&B)) -> Self
fn tap_borrow_dbg<B>(self, func: impl FnOnce(&B)) -> Self
.tap_borrow()
only in debug builds, and is erased in release
builds.§fn tap_borrow_mut_dbg<B>(self, func: impl FnOnce(&mut B)) -> Self
fn tap_borrow_mut_dbg<B>(self, func: impl FnOnce(&mut B)) -> Self
.tap_borrow_mut()
only in debug builds, and is erased in release
builds.§fn tap_ref_dbg<R>(self, func: impl FnOnce(&R)) -> Self
fn tap_ref_dbg<R>(self, func: impl FnOnce(&R)) -> Self
.tap_ref()
only in debug builds, and is erased in release
builds.§fn tap_ref_mut_dbg<R>(self, func: impl FnOnce(&mut R)) -> Self
fn tap_ref_mut_dbg<R>(self, func: impl FnOnce(&mut R)) -> Self
.tap_ref_mut()
only in debug builds, and is erased in release
builds.§fn tap_deref_dbg<T>(self, func: impl FnOnce(&T)) -> Self
fn tap_deref_dbg<T>(self, func: impl FnOnce(&T)) -> Self
.tap_deref()
only in debug builds, and is erased in release
builds.