Struct rerun::sdk::prelude::InstancePoses3D
source · pub struct InstancePoses3D {
pub translations: Option<SerializedComponentBatch>,
pub rotation_axis_angles: Option<SerializedComponentBatch>,
pub quaternions: Option<SerializedComponentBatch>,
pub scales: Option<SerializedComponentBatch>,
pub mat3x3: Option<SerializedComponentBatch>,
}
Expand description
Archetype: One or more transforms between the current entity and its parent. Unlike archetypes::Transform3D
, it is not propagated in the transform hierarchy.
If both archetypes::InstancePoses3D
and archetypes::Transform3D
are present,
first the tree propagating archetypes::Transform3D
is applied, then archetypes::InstancePoses3D
.
From the point of view of the entity’s coordinate system, all components are applied in the inverse order they are listed here. E.g. if both a translation and a max3x3 transform are present, the 3x3 matrix is applied first, followed by the translation.
Currently, many visualizers support only a single instance transform per entity.
Check archetype documentations for details - if not otherwise specified, only the first instance transform is applied.
Some visualizers like the mesh visualizer used for archetypes::Mesh3D
,
will draw an object for every pose, a behavior also known as “instancing”.
§Example
§Regular & instance transforms in tandem
use rerun::{
demo_util::grid,
external::{anyhow, glam},
};
fn main() -> anyhow::Result<()> {
let rec =
rerun::RecordingStreamBuilder::new("rerun_example_instance_pose3d_combined").spawn()?;
rec.set_time_sequence("frame", 0);
// Log a box and points further down in the hierarchy.
rec.log(
"world/box",
&rerun::Boxes3D::from_half_sizes([[1.0, 1.0, 1.0]]),
)?;
rec.log(
"world/box/points",
&rerun::Points3D::new(grid(glam::Vec3::splat(-10.0), glam::Vec3::splat(10.0), 10)),
)?;
for i in 0..180 {
rec.set_time_sequence("frame", i);
// Log a regular transform which affects both the box and the points.
rec.log(
"world/box",
&rerun::Transform3D::from_rotation(rerun::RotationAxisAngle {
axis: [0.0, 0.0, 1.0].into(),
angle: rerun::Angle::from_degrees(i as f32 * 2.0),
}),
)?;
// Log an instance pose which affects only the box.
let translation = [0.0, 0.0, (i as f32 * 0.1 - 5.0).abs() - 5.0];
rec.log(
"world/box",
&rerun::InstancePoses3D::new().with_translations([translation]),
)?;
}
Ok(())
}
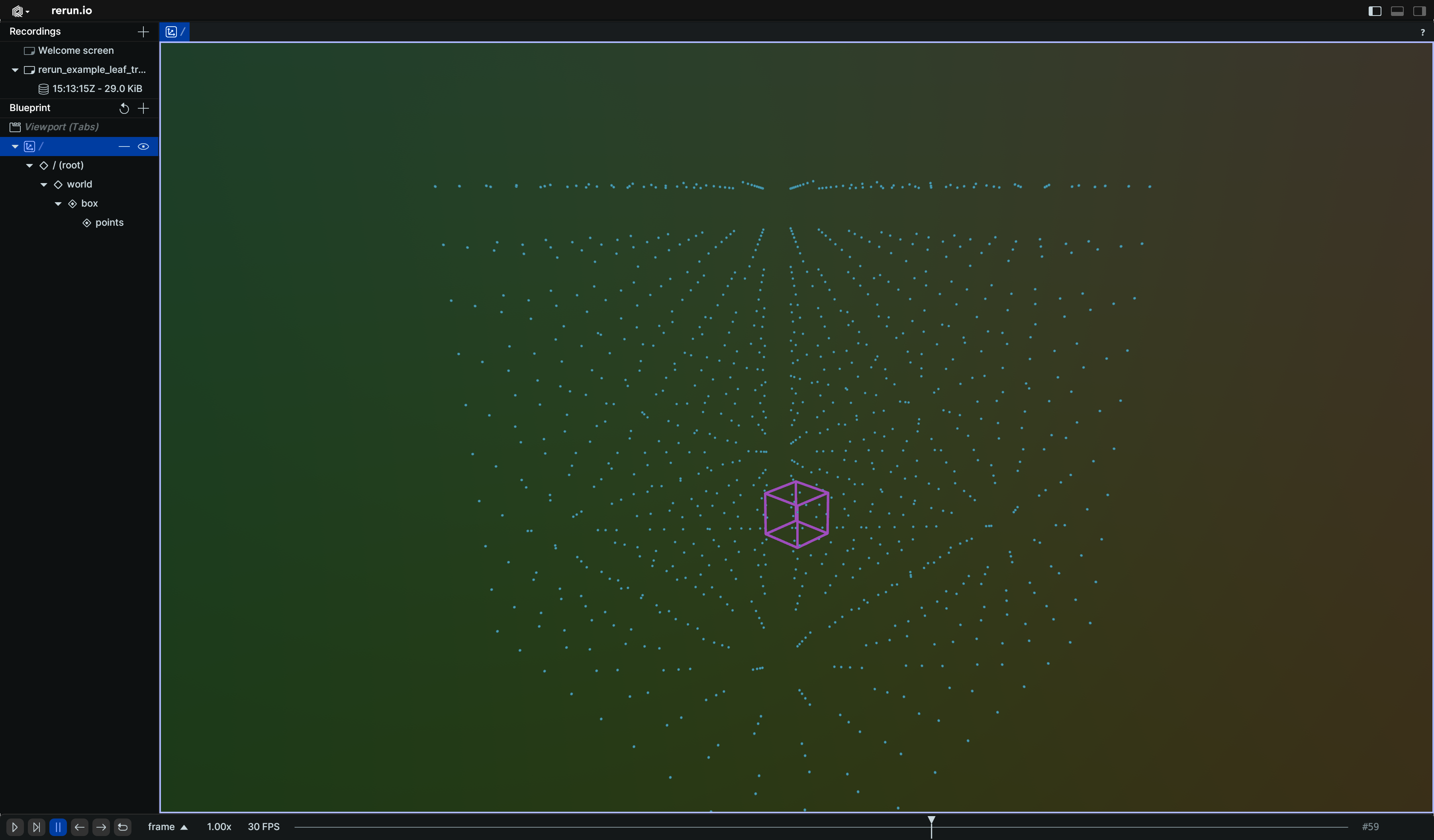
Fields§
§translations: Option<SerializedComponentBatch>
Translation vectors.
rotation_axis_angles: Option<SerializedComponentBatch>
Rotations via axis + angle.
quaternions: Option<SerializedComponentBatch>
Rotations via quaternion.
scales: Option<SerializedComponentBatch>
Scaling factors.
mat3x3: Option<SerializedComponentBatch>
3x3 transformation matrices.
Implementations§
source§impl InstancePoses3D
impl InstancePoses3D
sourcepub fn descriptor_translations() -> ComponentDescriptor
pub fn descriptor_translations() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::translations
.
sourcepub fn descriptor_rotation_axis_angles() -> ComponentDescriptor
pub fn descriptor_rotation_axis_angles() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::rotation_axis_angles
.
sourcepub fn descriptor_quaternions() -> ComponentDescriptor
pub fn descriptor_quaternions() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::quaternions
.
sourcepub fn descriptor_scales() -> ComponentDescriptor
pub fn descriptor_scales() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::scales
.
sourcepub fn descriptor_mat3x3() -> ComponentDescriptor
pub fn descriptor_mat3x3() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::mat3x3
.
sourcepub fn descriptor_indicator() -> ComponentDescriptor
pub fn descriptor_indicator() -> ComponentDescriptor
Returns the ComponentDescriptor
for the associated indicator component.
source§impl InstancePoses3D
impl InstancePoses3D
sourcepub const NUM_COMPONENTS: usize = 6usize
pub const NUM_COMPONENTS: usize = 6usize
The total number of components in the archetype: 0 required, 1 recommended, 5 optional
source§impl InstancePoses3D
impl InstancePoses3D
sourcepub fn new() -> InstancePoses3D
pub fn new() -> InstancePoses3D
Create a new InstancePoses3D
.
sourcepub fn update_fields() -> InstancePoses3D
pub fn update_fields() -> InstancePoses3D
Update only some specific fields of a InstancePoses3D
.
sourcepub fn clear_fields() -> InstancePoses3D
pub fn clear_fields() -> InstancePoses3D
Clear all the fields of a InstancePoses3D
.
sourcepub fn columns<I>(
self,
_lengths: I,
) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
pub fn columns<I>( self, _lengths: I, ) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
Partitions the component data into multiple sub-batches.
Specifically, this transforms the existing SerializedComponentBatch
es data into SerializedComponentColumn
s
instead, via SerializedComponentBatch::partitioned
.
This makes it possible to use RecordingStream::send_columns
to send columnar data directly into Rerun.
The specified lengths
must sum to the total length of the component batch.
sourcepub fn columns_of_unit_batches(
self,
) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
pub fn columns_of_unit_batches( self, ) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
Helper to partition the component data into unit-length sub-batches.
This is semantically similar to calling Self::columns
with std::iter::take(1).repeat(n)
,
where n
is automatically guessed.
sourcepub fn with_translations(
self,
translations: impl IntoIterator<Item = impl Into<PoseTranslation3D>>,
) -> InstancePoses3D
pub fn with_translations( self, translations: impl IntoIterator<Item = impl Into<PoseTranslation3D>>, ) -> InstancePoses3D
Translation vectors.
sourcepub fn with_rotation_axis_angles(
self,
rotation_axis_angles: impl IntoIterator<Item = impl Into<PoseRotationAxisAngle>>,
) -> InstancePoses3D
pub fn with_rotation_axis_angles( self, rotation_axis_angles: impl IntoIterator<Item = impl Into<PoseRotationAxisAngle>>, ) -> InstancePoses3D
Rotations via axis + angle.
sourcepub fn with_quaternions(
self,
quaternions: impl IntoIterator<Item = impl Into<PoseRotationQuat>>,
) -> InstancePoses3D
pub fn with_quaternions( self, quaternions: impl IntoIterator<Item = impl Into<PoseRotationQuat>>, ) -> InstancePoses3D
Rotations via quaternion.
sourcepub fn with_scales(
self,
scales: impl IntoIterator<Item = impl Into<PoseScale3D>>,
) -> InstancePoses3D
pub fn with_scales( self, scales: impl IntoIterator<Item = impl Into<PoseScale3D>>, ) -> InstancePoses3D
Scaling factors.
sourcepub fn with_mat3x3(
self,
mat3x3: impl IntoIterator<Item = impl Into<PoseTransformMat3x3>>,
) -> InstancePoses3D
pub fn with_mat3x3( self, mat3x3: impl IntoIterator<Item = impl Into<PoseTransformMat3x3>>, ) -> InstancePoses3D
3x3 transformation matrices.
Trait Implementations§
source§impl Archetype for InstancePoses3D
impl Archetype for InstancePoses3D
§type Indicator = GenericIndicatorComponent<InstancePoses3D>
type Indicator = GenericIndicatorComponent<InstancePoses3D>
source§fn name() -> ArchetypeName
fn name() -> ArchetypeName
rerun.archetypes.Points2D
.source§fn display_name() -> &'static str
fn display_name() -> &'static str
source§fn required_components() -> Cow<'static, [ComponentDescriptor]>
fn required_components() -> Cow<'static, [ComponentDescriptor]>
source§fn recommended_components() -> Cow<'static, [ComponentDescriptor]>
fn recommended_components() -> Cow<'static, [ComponentDescriptor]>
source§fn optional_components() -> Cow<'static, [ComponentDescriptor]>
fn optional_components() -> Cow<'static, [ComponentDescriptor]>
source§fn all_components() -> Cow<'static, [ComponentDescriptor]>
fn all_components() -> Cow<'static, [ComponentDescriptor]>
source§fn from_arrow_components(
arrow_data: impl IntoIterator<Item = (ComponentDescriptor, Arc<dyn Array>)>,
) -> Result<InstancePoses3D, DeserializationError>
fn from_arrow_components( arrow_data: impl IntoIterator<Item = (ComponentDescriptor, Arc<dyn Array>)>, ) -> Result<InstancePoses3D, DeserializationError>
ComponentNames
, deserializes them
into this archetype. Read moresource§fn from_arrow(
data: impl IntoIterator<Item = (Field, Arc<dyn Array>)>,
) -> Result<Self, DeserializationError>where
Self: Sized,
fn from_arrow(
data: impl IntoIterator<Item = (Field, Arc<dyn Array>)>,
) -> Result<Self, DeserializationError>where
Self: Sized,
source§impl AsComponents for InstancePoses3D
impl AsComponents for InstancePoses3D
source§fn as_serialized_batches(&self) -> Vec<SerializedComponentBatch>
fn as_serialized_batches(&self) -> Vec<SerializedComponentBatch>
SerializedComponentBatch
es. Read moresource§impl Clone for InstancePoses3D
impl Clone for InstancePoses3D
source§fn clone(&self) -> InstancePoses3D
fn clone(&self) -> InstancePoses3D
1.0.0 · source§fn clone_from(&mut self, source: &Self)
fn clone_from(&mut self, source: &Self)
source
. Read moresource§impl Debug for InstancePoses3D
impl Debug for InstancePoses3D
source§impl Default for InstancePoses3D
impl Default for InstancePoses3D
source§fn default() -> InstancePoses3D
fn default() -> InstancePoses3D
source§impl PartialEq for InstancePoses3D
impl PartialEq for InstancePoses3D
source§fn eq(&self, other: &InstancePoses3D) -> bool
fn eq(&self, other: &InstancePoses3D) -> bool
self
and other
values to be equal, and is used
by ==
.source§impl SizeBytes for InstancePoses3D
impl SizeBytes for InstancePoses3D
source§fn heap_size_bytes(&self) -> u64
fn heap_size_bytes(&self) -> u64
self
uses on the heap. Read moresource§fn total_size_bytes(&self) -> u64
fn total_size_bytes(&self) -> u64
self
in bytes, accounting for both stack and heap space.source§fn stack_size_bytes(&self) -> u64
fn stack_size_bytes(&self) -> u64
self
on the stack, in bytes. Read moreimpl ArchetypeReflectionMarker for InstancePoses3D
impl StructuralPartialEq for InstancePoses3D
Auto Trait Implementations§
impl Freeze for InstancePoses3D
impl !RefUnwindSafe for InstancePoses3D
impl Send for InstancePoses3D
impl Sync for InstancePoses3D
impl Unpin for InstancePoses3D
impl !UnwindSafe for InstancePoses3D
Blanket Implementations§
source§impl<T> BorrowMut<T> for Twhere
T: ?Sized,
impl<T> BorrowMut<T> for Twhere
T: ?Sized,
source§fn borrow_mut(&mut self) -> &mut T
fn borrow_mut(&mut self) -> &mut T
source§impl<T> CheckedAs for T
impl<T> CheckedAs for T
source§fn checked_as<Dst>(self) -> Option<Dst>where
T: CheckedCast<Dst>,
fn checked_as<Dst>(self) -> Option<Dst>where
T: CheckedCast<Dst>,
source§impl<Src, Dst> CheckedCastFrom<Src> for Dstwhere
Src: CheckedCast<Dst>,
impl<Src, Dst> CheckedCastFrom<Src> for Dstwhere
Src: CheckedCast<Dst>,
source§fn checked_cast_from(src: Src) -> Option<Dst>
fn checked_cast_from(src: Src) -> Option<Dst>
source§impl<T> CloneToUninit for Twhere
T: Clone,
impl<T> CloneToUninit for Twhere
T: Clone,
source§default unsafe fn clone_to_uninit(&self, dst: *mut T)
default unsafe fn clone_to_uninit(&self, dst: *mut T)
clone_to_uninit
)§impl<T> Conv for T
impl<T> Conv for T
§impl<T> Downcast for Twhere
T: Any,
impl<T> Downcast for Twhere
T: Any,
§fn into_any(self: Box<T>) -> Box<dyn Any>
fn into_any(self: Box<T>) -> Box<dyn Any>
Box<dyn Trait>
(where Trait: Downcast
) to Box<dyn Any>
. Box<dyn Any>
can
then be further downcast
into Box<ConcreteType>
where ConcreteType
implements Trait
.§fn into_any_rc(self: Rc<T>) -> Rc<dyn Any>
fn into_any_rc(self: Rc<T>) -> Rc<dyn Any>
Rc<Trait>
(where Trait: Downcast
) to Rc<Any>
. Rc<Any>
can then be
further downcast
into Rc<ConcreteType>
where ConcreteType
implements Trait
.§fn as_any(&self) -> &(dyn Any + 'static)
fn as_any(&self) -> &(dyn Any + 'static)
&Trait
(where Trait: Downcast
) to &Any
. This is needed since Rust cannot
generate &Any
’s vtable from &Trait
’s.§fn as_any_mut(&mut self) -> &mut (dyn Any + 'static)
fn as_any_mut(&mut self) -> &mut (dyn Any + 'static)
&mut Trait
(where Trait: Downcast
) to &Any
. This is needed since Rust cannot
generate &mut Any
’s vtable from &mut Trait
’s.§impl<T> DowncastSync for T
impl<T> DowncastSync for T
§impl<T> Instrument for T
impl<T> Instrument for T
§fn instrument(self, span: Span) -> Instrumented<Self>
fn instrument(self, span: Span) -> Instrumented<Self>
§fn in_current_span(self) -> Instrumented<Self>
fn in_current_span(self) -> Instrumented<Self>
source§impl<T> IntoEither for T
impl<T> IntoEither for T
source§fn into_either(self, into_left: bool) -> Either<Self, Self>
fn into_either(self, into_left: bool) -> Either<Self, Self>
self
into a Left
variant of Either<Self, Self>
if into_left
is true
.
Converts self
into a Right
variant of Either<Self, Self>
otherwise. Read moresource§fn into_either_with<F>(self, into_left: F) -> Either<Self, Self>
fn into_either_with<F>(self, into_left: F) -> Either<Self, Self>
self
into a Left
variant of Either<Self, Self>
if into_left(&self)
returns true
.
Converts self
into a Right
variant of Either<Self, Self>
otherwise. Read moresource§impl<T> IntoRequest<T> for T
impl<T> IntoRequest<T> for T
source§fn into_request(self) -> Request<T>
fn into_request(self) -> Request<T>
T
in a tonic::Request
source§impl<Src, Dst> LosslessTryInto<Dst> for Srcwhere
Dst: LosslessTryFrom<Src>,
impl<Src, Dst> LosslessTryInto<Dst> for Srcwhere
Dst: LosslessTryFrom<Src>,
source§fn lossless_try_into(self) -> Option<Dst>
fn lossless_try_into(self) -> Option<Dst>
source§impl<Src, Dst> LossyInto<Dst> for Srcwhere
Dst: LossyFrom<Src>,
impl<Src, Dst> LossyInto<Dst> for Srcwhere
Dst: LossyFrom<Src>,
source§fn lossy_into(self) -> Dst
fn lossy_into(self) -> Dst
§impl<T> NoneValue for Twhere
T: Default,
impl<T> NoneValue for Twhere
T: Default,
type NoneType = T
§fn null_value() -> T
fn null_value() -> T
source§impl<T> OverflowingAs for T
impl<T> OverflowingAs for T
source§fn overflowing_as<Dst>(self) -> (Dst, bool)where
T: OverflowingCast<Dst>,
fn overflowing_as<Dst>(self) -> (Dst, bool)where
T: OverflowingCast<Dst>,
source§impl<Src, Dst> OverflowingCastFrom<Src> for Dstwhere
Src: OverflowingCast<Dst>,
impl<Src, Dst> OverflowingCastFrom<Src> for Dstwhere
Src: OverflowingCast<Dst>,
source§fn overflowing_cast_from(src: Src) -> (Dst, bool)
fn overflowing_cast_from(src: Src) -> (Dst, bool)
§impl<T> Pipe for Twhere
T: ?Sized,
impl<T> Pipe for Twhere
T: ?Sized,
§fn pipe<R>(self, func: impl FnOnce(Self) -> R) -> Rwhere
Self: Sized,
fn pipe<R>(self, func: impl FnOnce(Self) -> R) -> Rwhere
Self: Sized,
§fn pipe_ref<'a, R>(&'a self, func: impl FnOnce(&'a Self) -> R) -> Rwhere
R: 'a,
fn pipe_ref<'a, R>(&'a self, func: impl FnOnce(&'a Self) -> R) -> Rwhere
R: 'a,
self
and passes that borrow into the pipe function. Read more§fn pipe_ref_mut<'a, R>(&'a mut self, func: impl FnOnce(&'a mut Self) -> R) -> Rwhere
R: 'a,
fn pipe_ref_mut<'a, R>(&'a mut self, func: impl FnOnce(&'a mut Self) -> R) -> Rwhere
R: 'a,
self
and passes that borrow into the pipe function. Read more§fn pipe_borrow<'a, B, R>(&'a self, func: impl FnOnce(&'a B) -> R) -> R
fn pipe_borrow<'a, B, R>(&'a self, func: impl FnOnce(&'a B) -> R) -> R
§fn pipe_borrow_mut<'a, B, R>(
&'a mut self,
func: impl FnOnce(&'a mut B) -> R,
) -> R
fn pipe_borrow_mut<'a, B, R>( &'a mut self, func: impl FnOnce(&'a mut B) -> R, ) -> R
§fn pipe_as_ref<'a, U, R>(&'a self, func: impl FnOnce(&'a U) -> R) -> R
fn pipe_as_ref<'a, U, R>(&'a self, func: impl FnOnce(&'a U) -> R) -> R
self
, then passes self.as_ref()
into the pipe function.§fn pipe_as_mut<'a, U, R>(&'a mut self, func: impl FnOnce(&'a mut U) -> R) -> R
fn pipe_as_mut<'a, U, R>(&'a mut self, func: impl FnOnce(&'a mut U) -> R) -> R
self
, then passes self.as_mut()
into the pipe
function.§fn pipe_deref<'a, T, R>(&'a self, func: impl FnOnce(&'a T) -> R) -> R
fn pipe_deref<'a, T, R>(&'a self, func: impl FnOnce(&'a T) -> R) -> R
self
, then passes self.deref()
into the pipe function.§impl<T> Pointable for T
impl<T> Pointable for T
source§impl<T> SaturatingAs for T
impl<T> SaturatingAs for T
source§fn saturating_as<Dst>(self) -> Dstwhere
T: SaturatingCast<Dst>,
fn saturating_as<Dst>(self) -> Dstwhere
T: SaturatingCast<Dst>,
source§impl<Src, Dst> SaturatingCastFrom<Src> for Dstwhere
Src: SaturatingCast<Dst>,
impl<Src, Dst> SaturatingCastFrom<Src> for Dstwhere
Src: SaturatingCast<Dst>,
source§fn saturating_cast_from(src: Src) -> Dst
fn saturating_cast_from(src: Src) -> Dst
§impl<T> Tap for T
impl<T> Tap for T
§fn tap_borrow<B>(self, func: impl FnOnce(&B)) -> Self
fn tap_borrow<B>(self, func: impl FnOnce(&B)) -> Self
Borrow<B>
of a value. Read more§fn tap_borrow_mut<B>(self, func: impl FnOnce(&mut B)) -> Self
fn tap_borrow_mut<B>(self, func: impl FnOnce(&mut B)) -> Self
BorrowMut<B>
of a value. Read more§fn tap_ref<R>(self, func: impl FnOnce(&R)) -> Self
fn tap_ref<R>(self, func: impl FnOnce(&R)) -> Self
AsRef<R>
view of a value. Read more§fn tap_ref_mut<R>(self, func: impl FnOnce(&mut R)) -> Self
fn tap_ref_mut<R>(self, func: impl FnOnce(&mut R)) -> Self
AsMut<R>
view of a value. Read more§fn tap_deref<T>(self, func: impl FnOnce(&T)) -> Self
fn tap_deref<T>(self, func: impl FnOnce(&T)) -> Self
Deref::Target
of a value. Read more§fn tap_deref_mut<T>(self, func: impl FnOnce(&mut T)) -> Self
fn tap_deref_mut<T>(self, func: impl FnOnce(&mut T)) -> Self
Deref::Target
of a value. Read more§fn tap_dbg(self, func: impl FnOnce(&Self)) -> Self
fn tap_dbg(self, func: impl FnOnce(&Self)) -> Self
.tap()
only in debug builds, and is erased in release builds.§fn tap_mut_dbg(self, func: impl FnOnce(&mut Self)) -> Self
fn tap_mut_dbg(self, func: impl FnOnce(&mut Self)) -> Self
.tap_mut()
only in debug builds, and is erased in release
builds.§fn tap_borrow_dbg<B>(self, func: impl FnOnce(&B)) -> Self
fn tap_borrow_dbg<B>(self, func: impl FnOnce(&B)) -> Self
.tap_borrow()
only in debug builds, and is erased in release
builds.§fn tap_borrow_mut_dbg<B>(self, func: impl FnOnce(&mut B)) -> Self
fn tap_borrow_mut_dbg<B>(self, func: impl FnOnce(&mut B)) -> Self
.tap_borrow_mut()
only in debug builds, and is erased in release
builds.§fn tap_ref_dbg<R>(self, func: impl FnOnce(&R)) -> Self
fn tap_ref_dbg<R>(self, func: impl FnOnce(&R)) -> Self
.tap_ref()
only in debug builds, and is erased in release
builds.§fn tap_ref_mut_dbg<R>(self, func: impl FnOnce(&mut R)) -> Self
fn tap_ref_mut_dbg<R>(self, func: impl FnOnce(&mut R)) -> Self
.tap_ref_mut()
only in debug builds, and is erased in release
builds.§fn tap_deref_dbg<T>(self, func: impl FnOnce(&T)) -> Self
fn tap_deref_dbg<T>(self, func: impl FnOnce(&T)) -> Self
.tap_deref()
only in debug builds, and is erased in release
builds.