Struct rerun::SeriesLine
source · pub struct SeriesLine {
pub color: Option<SerializedComponentBatch>,
pub width: Option<SerializedComponentBatch>,
pub name: Option<SerializedComponentBatch>,
pub aggregation_policy: Option<SerializedComponentBatch>,
}
Expand description
Archetype: Define the style properties for a line series in a chart.
This archetype only provides styling information and should be logged as static
when possible. The underlying data needs to be logged to the same entity-path using
archetypes::Scalar
.
§Example
§Line series
fn main() -> Result<(), Box<dyn std::error::Error>> {
let rec = rerun::RecordingStreamBuilder::new("rerun_example_series_line_style").spawn()?;
// Set up plot styling:
// They are logged static as they don't change over time and apply to all timelines.
// Log two lines series under a shared root so that they show in the same plot by default.
rec.log_static(
"trig/sin",
&rerun::SeriesLine::new()
.with_color([255, 0, 0])
.with_name("sin(0.01t)")
.with_width(2.0),
)?;
rec.log_static(
"trig/cos",
&rerun::SeriesLine::new()
.with_color([0, 255, 0])
.with_name("cos(0.01t)")
.with_width(4.0),
)?;
for t in 0..((std::f32::consts::TAU * 2.0 * 100.0) as i64) {
rec.set_time_sequence("step", t);
// Log two time series under a shared root so that they show in the same plot by default.
rec.log("trig/sin", &rerun::Scalar::new((t as f64 / 100.0).sin()))?;
rec.log("trig/cos", &rerun::Scalar::new((t as f64 / 100.0).cos()))?;
}
Ok(())
}
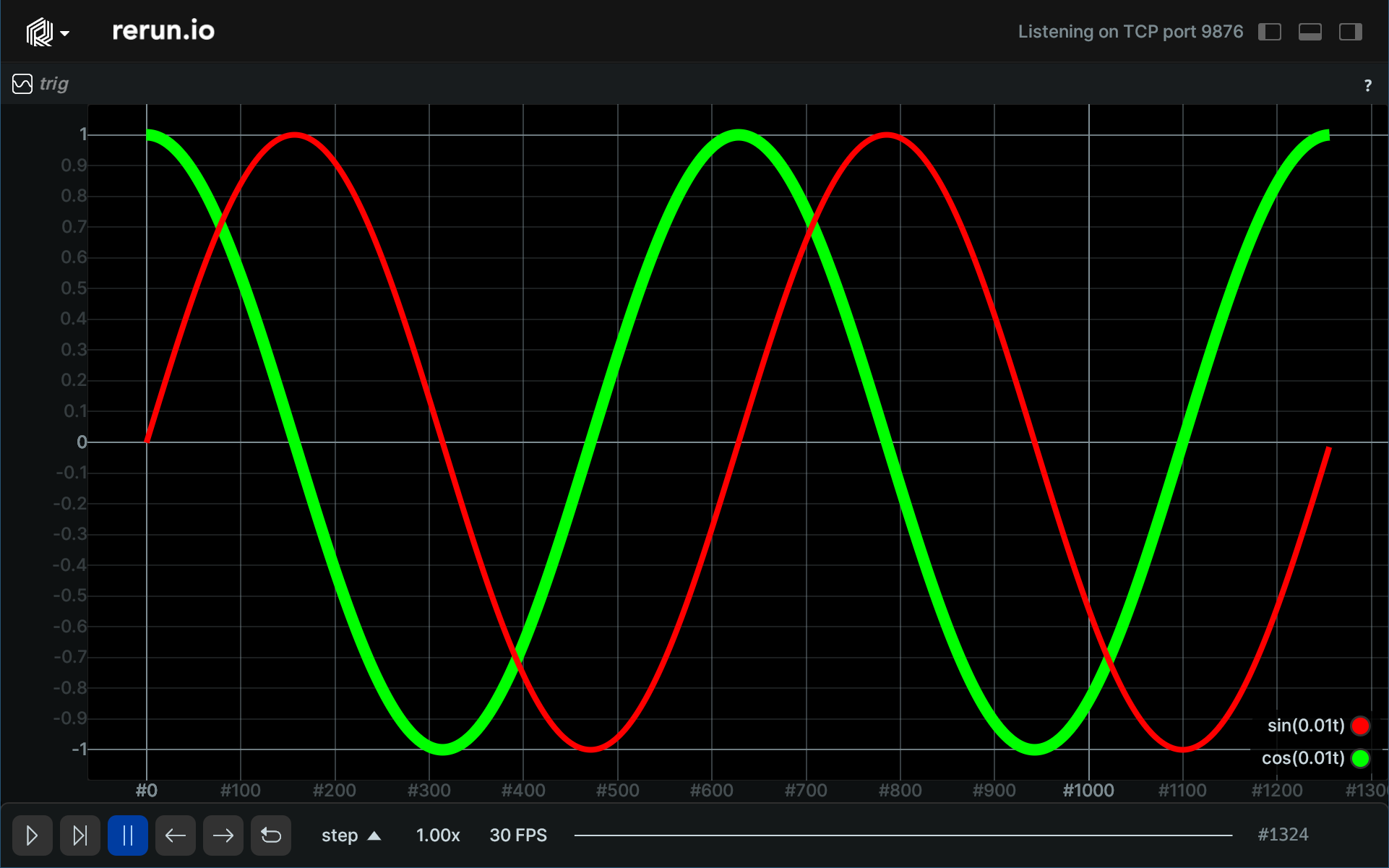
Fields§
§color: Option<SerializedComponentBatch>
Color for the corresponding series.
width: Option<SerializedComponentBatch>
Stroke width for the corresponding series.
name: Option<SerializedComponentBatch>
Display name of the series.
Used in the legend.
aggregation_policy: Option<SerializedComponentBatch>
Configures the zoom-dependent scalar aggregation.
This is done only if steps on the X axis go below a single pixel, i.e. a single pixel covers more than one tick worth of data. It can greatly improve performance (and readability) in such situations as it prevents overdraw.
Implementations§
source§impl SeriesLine
impl SeriesLine
sourcepub fn descriptor_color() -> ComponentDescriptor
pub fn descriptor_color() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::color
.
sourcepub fn descriptor_width() -> ComponentDescriptor
pub fn descriptor_width() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::width
.
sourcepub fn descriptor_name() -> ComponentDescriptor
pub fn descriptor_name() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::name
.
sourcepub fn descriptor_aggregation_policy() -> ComponentDescriptor
pub fn descriptor_aggregation_policy() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::aggregation_policy
.
sourcepub fn descriptor_indicator() -> ComponentDescriptor
pub fn descriptor_indicator() -> ComponentDescriptor
Returns the ComponentDescriptor
for the associated indicator component.
source§impl SeriesLine
impl SeriesLine
sourcepub const NUM_COMPONENTS: usize = 5usize
pub const NUM_COMPONENTS: usize = 5usize
The total number of components in the archetype: 0 required, 1 recommended, 4 optional
source§impl SeriesLine
impl SeriesLine
sourcepub fn new() -> SeriesLine
pub fn new() -> SeriesLine
Create a new SeriesLine
.
sourcepub fn update_fields() -> SeriesLine
pub fn update_fields() -> SeriesLine
Update only some specific fields of a SeriesLine
.
sourcepub fn clear_fields() -> SeriesLine
pub fn clear_fields() -> SeriesLine
Clear all the fields of a SeriesLine
.
sourcepub fn columns<I>(
self,
_lengths: I,
) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
pub fn columns<I>( self, _lengths: I, ) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
Partitions the component data into multiple sub-batches.
Specifically, this transforms the existing SerializedComponentBatch
es data into SerializedComponentColumn
s
instead, via SerializedComponentBatch::partitioned
.
This makes it possible to use RecordingStream::send_columns
to send columnar data directly into Rerun.
The specified lengths
must sum to the total length of the component batch.
sourcepub fn columns_of_unit_batches(
self,
) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
pub fn columns_of_unit_batches( self, ) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
Helper to partition the component data into unit-length sub-batches.
This is semantically similar to calling Self::columns
with std::iter::take(1).repeat(n)
,
where n
is automatically guessed.
sourcepub fn with_color(self, color: impl Into<Color>) -> SeriesLine
pub fn with_color(self, color: impl Into<Color>) -> SeriesLine
Color for the corresponding series.
sourcepub fn with_many_color(
self,
color: impl IntoIterator<Item = impl Into<Color>>,
) -> SeriesLine
pub fn with_many_color( self, color: impl IntoIterator<Item = impl Into<Color>>, ) -> SeriesLine
This method makes it possible to pack multiple crate::components::Color
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_color
should
be used when logging a single row’s worth of data.
sourcepub fn with_width(self, width: impl Into<StrokeWidth>) -> SeriesLine
pub fn with_width(self, width: impl Into<StrokeWidth>) -> SeriesLine
Stroke width for the corresponding series.
sourcepub fn with_many_width(
self,
width: impl IntoIterator<Item = impl Into<StrokeWidth>>,
) -> SeriesLine
pub fn with_many_width( self, width: impl IntoIterator<Item = impl Into<StrokeWidth>>, ) -> SeriesLine
This method makes it possible to pack multiple crate::components::StrokeWidth
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_width
should
be used when logging a single row’s worth of data.
sourcepub fn with_name(self, name: impl Into<Name>) -> SeriesLine
pub fn with_name(self, name: impl Into<Name>) -> SeriesLine
Display name of the series.
Used in the legend.
sourcepub fn with_many_name(
self,
name: impl IntoIterator<Item = impl Into<Name>>,
) -> SeriesLine
pub fn with_many_name( self, name: impl IntoIterator<Item = impl Into<Name>>, ) -> SeriesLine
This method makes it possible to pack multiple crate::components::Name
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_name
should
be used when logging a single row’s worth of data.
sourcepub fn with_aggregation_policy(
self,
aggregation_policy: impl Into<AggregationPolicy>,
) -> SeriesLine
pub fn with_aggregation_policy( self, aggregation_policy: impl Into<AggregationPolicy>, ) -> SeriesLine
Configures the zoom-dependent scalar aggregation.
This is done only if steps on the X axis go below a single pixel, i.e. a single pixel covers more than one tick worth of data. It can greatly improve performance (and readability) in such situations as it prevents overdraw.
sourcepub fn with_many_aggregation_policy(
self,
aggregation_policy: impl IntoIterator<Item = impl Into<AggregationPolicy>>,
) -> SeriesLine
pub fn with_many_aggregation_policy( self, aggregation_policy: impl IntoIterator<Item = impl Into<AggregationPolicy>>, ) -> SeriesLine
This method makes it possible to pack multiple crate::components::AggregationPolicy
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_aggregation_policy
should
be used when logging a single row’s worth of data.
Trait Implementations§
source§impl Archetype for SeriesLine
impl Archetype for SeriesLine
§type Indicator = GenericIndicatorComponent<SeriesLine>
type Indicator = GenericIndicatorComponent<SeriesLine>
source§fn name() -> ArchetypeName
fn name() -> ArchetypeName
rerun.archetypes.Points2D
.source§fn display_name() -> &'static str
fn display_name() -> &'static str
source§fn required_components() -> Cow<'static, [ComponentDescriptor]>
fn required_components() -> Cow<'static, [ComponentDescriptor]>
source§fn recommended_components() -> Cow<'static, [ComponentDescriptor]>
fn recommended_components() -> Cow<'static, [ComponentDescriptor]>
source§fn optional_components() -> Cow<'static, [ComponentDescriptor]>
fn optional_components() -> Cow<'static, [ComponentDescriptor]>
source§fn all_components() -> Cow<'static, [ComponentDescriptor]>
fn all_components() -> Cow<'static, [ComponentDescriptor]>
source§fn from_arrow_components(
arrow_data: impl IntoIterator<Item = (ComponentDescriptor, Arc<dyn Array>)>,
) -> Result<SeriesLine, DeserializationError>
fn from_arrow_components( arrow_data: impl IntoIterator<Item = (ComponentDescriptor, Arc<dyn Array>)>, ) -> Result<SeriesLine, DeserializationError>
ComponentNames
, deserializes them
into this archetype. Read moresource§fn from_arrow(
data: impl IntoIterator<Item = (Field, Arc<dyn Array>)>,
) -> Result<Self, DeserializationError>where
Self: Sized,
fn from_arrow(
data: impl IntoIterator<Item = (Field, Arc<dyn Array>)>,
) -> Result<Self, DeserializationError>where
Self: Sized,
source§impl AsComponents for SeriesLine
impl AsComponents for SeriesLine
source§fn as_serialized_batches(&self) -> Vec<SerializedComponentBatch>
fn as_serialized_batches(&self) -> Vec<SerializedComponentBatch>
SerializedComponentBatch
es. Read moresource§impl Clone for SeriesLine
impl Clone for SeriesLine
source§fn clone(&self) -> SeriesLine
fn clone(&self) -> SeriesLine
1.0.0 · source§fn clone_from(&mut self, source: &Self)
fn clone_from(&mut self, source: &Self)
source
. Read moresource§impl Debug for SeriesLine
impl Debug for SeriesLine
source§impl Default for SeriesLine
impl Default for SeriesLine
source§fn default() -> SeriesLine
fn default() -> SeriesLine
source§impl SizeBytes for SeriesLine
impl SizeBytes for SeriesLine
source§fn heap_size_bytes(&self) -> u64
fn heap_size_bytes(&self) -> u64
self
uses on the heap. Read moresource§fn total_size_bytes(&self) -> u64
fn total_size_bytes(&self) -> u64
self
in bytes, accounting for both stack and heap space.source§fn stack_size_bytes(&self) -> u64
fn stack_size_bytes(&self) -> u64
self
on the stack, in bytes. Read moreimpl ArchetypeReflectionMarker for SeriesLine
Auto Trait Implementations§
impl Freeze for SeriesLine
impl !RefUnwindSafe for SeriesLine
impl Send for SeriesLine
impl Sync for SeriesLine
impl Unpin for SeriesLine
impl !UnwindSafe for SeriesLine
Blanket Implementations§
source§impl<T> BorrowMut<T> for Twhere
T: ?Sized,
impl<T> BorrowMut<T> for Twhere
T: ?Sized,
source§fn borrow_mut(&mut self) -> &mut T
fn borrow_mut(&mut self) -> &mut T
source§impl<T> CheckedAs for T
impl<T> CheckedAs for T
source§fn checked_as<Dst>(self) -> Option<Dst>where
T: CheckedCast<Dst>,
fn checked_as<Dst>(self) -> Option<Dst>where
T: CheckedCast<Dst>,
source§impl<Src, Dst> CheckedCastFrom<Src> for Dstwhere
Src: CheckedCast<Dst>,
impl<Src, Dst> CheckedCastFrom<Src> for Dstwhere
Src: CheckedCast<Dst>,
source§fn checked_cast_from(src: Src) -> Option<Dst>
fn checked_cast_from(src: Src) -> Option<Dst>
source§impl<T> CloneToUninit for Twhere
T: Clone,
impl<T> CloneToUninit for Twhere
T: Clone,
source§default unsafe fn clone_to_uninit(&self, dst: *mut T)
default unsafe fn clone_to_uninit(&self, dst: *mut T)
clone_to_uninit
)§impl<T> Conv for T
impl<T> Conv for T
§impl<T> Downcast for Twhere
T: Any,
impl<T> Downcast for Twhere
T: Any,
§fn into_any(self: Box<T>) -> Box<dyn Any>
fn into_any(self: Box<T>) -> Box<dyn Any>
Box<dyn Trait>
(where Trait: Downcast
) to Box<dyn Any>
. Box<dyn Any>
can
then be further downcast
into Box<ConcreteType>
where ConcreteType
implements Trait
.§fn into_any_rc(self: Rc<T>) -> Rc<dyn Any>
fn into_any_rc(self: Rc<T>) -> Rc<dyn Any>
Rc<Trait>
(where Trait: Downcast
) to Rc<Any>
. Rc<Any>
can then be
further downcast
into Rc<ConcreteType>
where ConcreteType
implements Trait
.§fn as_any(&self) -> &(dyn Any + 'static)
fn as_any(&self) -> &(dyn Any + 'static)
&Trait
(where Trait: Downcast
) to &Any
. This is needed since Rust cannot
generate &Any
’s vtable from &Trait
’s.§fn as_any_mut(&mut self) -> &mut (dyn Any + 'static)
fn as_any_mut(&mut self) -> &mut (dyn Any + 'static)
&mut Trait
(where Trait: Downcast
) to &Any
. This is needed since Rust cannot
generate &mut Any
’s vtable from &mut Trait
’s.§impl<T> DowncastSync for T
impl<T> DowncastSync for T
§impl<T> Instrument for T
impl<T> Instrument for T
§fn instrument(self, span: Span) -> Instrumented<Self>
fn instrument(self, span: Span) -> Instrumented<Self>
§fn in_current_span(self) -> Instrumented<Self>
fn in_current_span(self) -> Instrumented<Self>
source§impl<T> IntoEither for T
impl<T> IntoEither for T
source§fn into_either(self, into_left: bool) -> Either<Self, Self>
fn into_either(self, into_left: bool) -> Either<Self, Self>
self
into a Left
variant of Either<Self, Self>
if into_left
is true
.
Converts self
into a Right
variant of Either<Self, Self>
otherwise. Read moresource§fn into_either_with<F>(self, into_left: F) -> Either<Self, Self>
fn into_either_with<F>(self, into_left: F) -> Either<Self, Self>
self
into a Left
variant of Either<Self, Self>
if into_left(&self)
returns true
.
Converts self
into a Right
variant of Either<Self, Self>
otherwise. Read moresource§impl<T> IntoRequest<T> for T
impl<T> IntoRequest<T> for T
source§fn into_request(self) -> Request<T>
fn into_request(self) -> Request<T>
T
in a tonic::Request
source§impl<Src, Dst> LosslessTryInto<Dst> for Srcwhere
Dst: LosslessTryFrom<Src>,
impl<Src, Dst> LosslessTryInto<Dst> for Srcwhere
Dst: LosslessTryFrom<Src>,
source§fn lossless_try_into(self) -> Option<Dst>
fn lossless_try_into(self) -> Option<Dst>
source§impl<Src, Dst> LossyInto<Dst> for Srcwhere
Dst: LossyFrom<Src>,
impl<Src, Dst> LossyInto<Dst> for Srcwhere
Dst: LossyFrom<Src>,
source§fn lossy_into(self) -> Dst
fn lossy_into(self) -> Dst
§impl<T> NoneValue for Twhere
T: Default,
impl<T> NoneValue for Twhere
T: Default,
type NoneType = T
§fn null_value() -> T
fn null_value() -> T
source§impl<T> OverflowingAs for T
impl<T> OverflowingAs for T
source§fn overflowing_as<Dst>(self) -> (Dst, bool)where
T: OverflowingCast<Dst>,
fn overflowing_as<Dst>(self) -> (Dst, bool)where
T: OverflowingCast<Dst>,
source§impl<Src, Dst> OverflowingCastFrom<Src> for Dstwhere
Src: OverflowingCast<Dst>,
impl<Src, Dst> OverflowingCastFrom<Src> for Dstwhere
Src: OverflowingCast<Dst>,
source§fn overflowing_cast_from(src: Src) -> (Dst, bool)
fn overflowing_cast_from(src: Src) -> (Dst, bool)
§impl<T> Pipe for Twhere
T: ?Sized,
impl<T> Pipe for Twhere
T: ?Sized,
§fn pipe<R>(self, func: impl FnOnce(Self) -> R) -> Rwhere
Self: Sized,
fn pipe<R>(self, func: impl FnOnce(Self) -> R) -> Rwhere
Self: Sized,
§fn pipe_ref<'a, R>(&'a self, func: impl FnOnce(&'a Self) -> R) -> Rwhere
R: 'a,
fn pipe_ref<'a, R>(&'a self, func: impl FnOnce(&'a Self) -> R) -> Rwhere
R: 'a,
self
and passes that borrow into the pipe function. Read more§fn pipe_ref_mut<'a, R>(&'a mut self, func: impl FnOnce(&'a mut Self) -> R) -> Rwhere
R: 'a,
fn pipe_ref_mut<'a, R>(&'a mut self, func: impl FnOnce(&'a mut Self) -> R) -> Rwhere
R: 'a,
self
and passes that borrow into the pipe function. Read more§fn pipe_borrow<'a, B, R>(&'a self, func: impl FnOnce(&'a B) -> R) -> R
fn pipe_borrow<'a, B, R>(&'a self, func: impl FnOnce(&'a B) -> R) -> R
§fn pipe_borrow_mut<'a, B, R>(
&'a mut self,
func: impl FnOnce(&'a mut B) -> R,
) -> R
fn pipe_borrow_mut<'a, B, R>( &'a mut self, func: impl FnOnce(&'a mut B) -> R, ) -> R
§fn pipe_as_ref<'a, U, R>(&'a self, func: impl FnOnce(&'a U) -> R) -> R
fn pipe_as_ref<'a, U, R>(&'a self, func: impl FnOnce(&'a U) -> R) -> R
self
, then passes self.as_ref()
into the pipe function.§fn pipe_as_mut<'a, U, R>(&'a mut self, func: impl FnOnce(&'a mut U) -> R) -> R
fn pipe_as_mut<'a, U, R>(&'a mut self, func: impl FnOnce(&'a mut U) -> R) -> R
self
, then passes self.as_mut()
into the pipe
function.§fn pipe_deref<'a, T, R>(&'a self, func: impl FnOnce(&'a T) -> R) -> R
fn pipe_deref<'a, T, R>(&'a self, func: impl FnOnce(&'a T) -> R) -> R
self
, then passes self.deref()
into the pipe function.§impl<T> Pointable for T
impl<T> Pointable for T
source§impl<T> SaturatingAs for T
impl<T> SaturatingAs for T
source§fn saturating_as<Dst>(self) -> Dstwhere
T: SaturatingCast<Dst>,
fn saturating_as<Dst>(self) -> Dstwhere
T: SaturatingCast<Dst>,
source§impl<Src, Dst> SaturatingCastFrom<Src> for Dstwhere
Src: SaturatingCast<Dst>,
impl<Src, Dst> SaturatingCastFrom<Src> for Dstwhere
Src: SaturatingCast<Dst>,
source§fn saturating_cast_from(src: Src) -> Dst
fn saturating_cast_from(src: Src) -> Dst
§impl<T> Tap for T
impl<T> Tap for T
§fn tap_borrow<B>(self, func: impl FnOnce(&B)) -> Self
fn tap_borrow<B>(self, func: impl FnOnce(&B)) -> Self
Borrow<B>
of a value. Read more§fn tap_borrow_mut<B>(self, func: impl FnOnce(&mut B)) -> Self
fn tap_borrow_mut<B>(self, func: impl FnOnce(&mut B)) -> Self
BorrowMut<B>
of a value. Read more§fn tap_ref<R>(self, func: impl FnOnce(&R)) -> Self
fn tap_ref<R>(self, func: impl FnOnce(&R)) -> Self
AsRef<R>
view of a value. Read more§fn tap_ref_mut<R>(self, func: impl FnOnce(&mut R)) -> Self
fn tap_ref_mut<R>(self, func: impl FnOnce(&mut R)) -> Self
AsMut<R>
view of a value. Read more§fn tap_deref<T>(self, func: impl FnOnce(&T)) -> Self
fn tap_deref<T>(self, func: impl FnOnce(&T)) -> Self
Deref::Target
of a value. Read more§fn tap_deref_mut<T>(self, func: impl FnOnce(&mut T)) -> Self
fn tap_deref_mut<T>(self, func: impl FnOnce(&mut T)) -> Self
Deref::Target
of a value. Read more§fn tap_dbg(self, func: impl FnOnce(&Self)) -> Self
fn tap_dbg(self, func: impl FnOnce(&Self)) -> Self
.tap()
only in debug builds, and is erased in release builds.§fn tap_mut_dbg(self, func: impl FnOnce(&mut Self)) -> Self
fn tap_mut_dbg(self, func: impl FnOnce(&mut Self)) -> Self
.tap_mut()
only in debug builds, and is erased in release
builds.§fn tap_borrow_dbg<B>(self, func: impl FnOnce(&B)) -> Self
fn tap_borrow_dbg<B>(self, func: impl FnOnce(&B)) -> Self
.tap_borrow()
only in debug builds, and is erased in release
builds.§fn tap_borrow_mut_dbg<B>(self, func: impl FnOnce(&mut B)) -> Self
fn tap_borrow_mut_dbg<B>(self, func: impl FnOnce(&mut B)) -> Self
.tap_borrow_mut()
only in debug builds, and is erased in release
builds.§fn tap_ref_dbg<R>(self, func: impl FnOnce(&R)) -> Self
fn tap_ref_dbg<R>(self, func: impl FnOnce(&R)) -> Self
.tap_ref()
only in debug builds, and is erased in release
builds.§fn tap_ref_mut_dbg<R>(self, func: impl FnOnce(&mut R)) -> Self
fn tap_ref_mut_dbg<R>(self, func: impl FnOnce(&mut R)) -> Self
.tap_ref_mut()
only in debug builds, and is erased in release
builds.§fn tap_deref_dbg<T>(self, func: impl FnOnce(&T)) -> Self
fn tap_deref_dbg<T>(self, func: impl FnOnce(&T)) -> Self
.tap_deref()
only in debug builds, and is erased in release
builds.