Archetype: A list of nodes in a graph with optional labels, colors, etc.
More...
#include <rerun/archetypes/graph_nodes.hpp>
|
std::optional< ComponentBatch > | node_ids |
| A list of node IDs.
|
|
std::optional< ComponentBatch > | positions |
| Optional center positions of the nodes.
|
|
std::optional< ComponentBatch > | colors |
| Optional colors for the boxes.
|
|
std::optional< ComponentBatch > | labels |
| Optional text labels for the node.
|
|
std::optional< ComponentBatch > | show_labels |
| Optional choice of whether the text labels should be shown by default.
|
|
std::optional< ComponentBatch > | radii |
| Optional radii for nodes.
|
|
Archetype: A list of nodes in a graph with optional labels, colors, etc.
Example
Simple directed graph
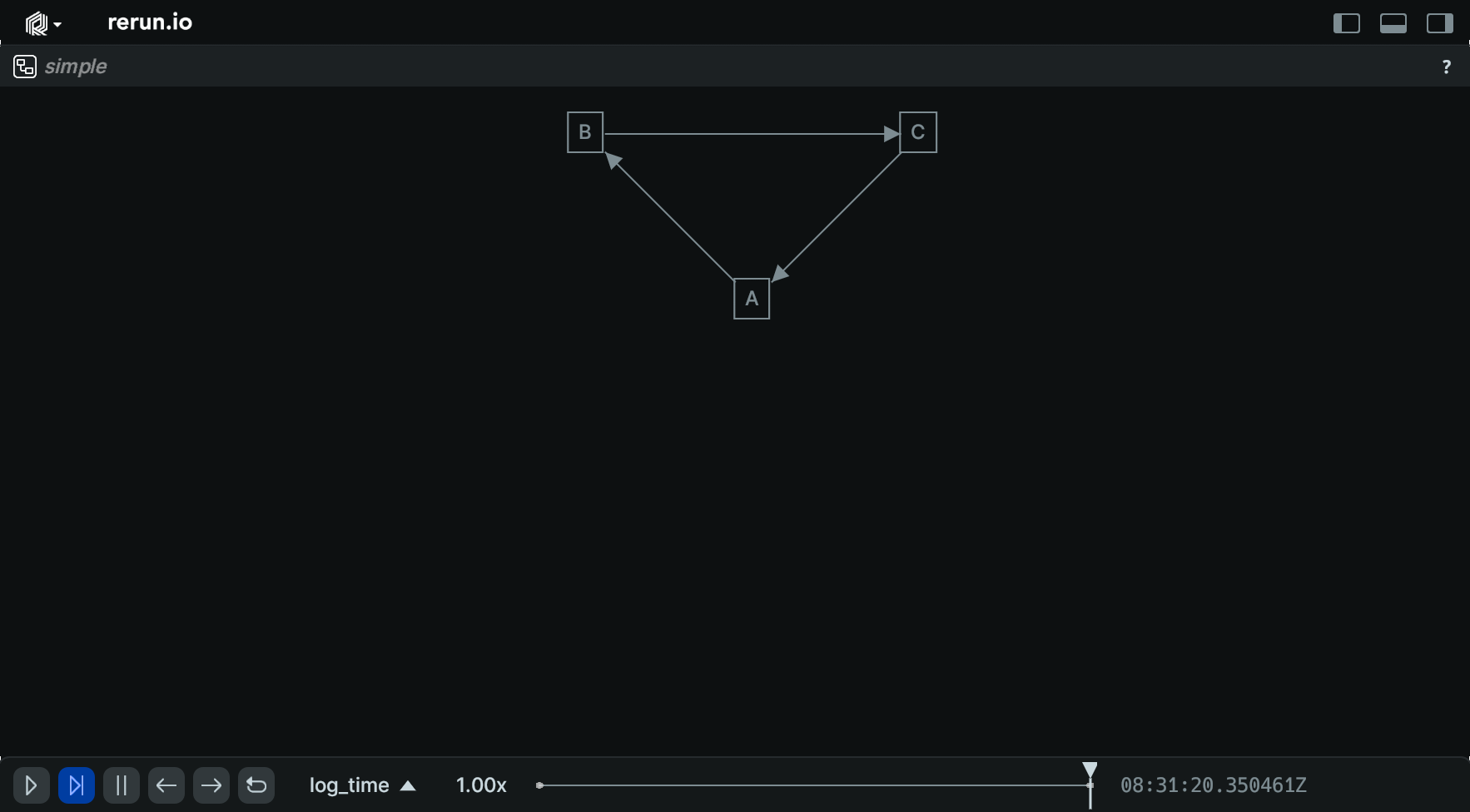
#include <rerun.hpp>
int main() {
rec.spawn().exit_on_failure();
rec.log(
"simple",
);
}
A RecordingStream handles everything related to logging data into Rerun.
Definition recording_stream.hpp:60
@ Directed
The graph has directed edges.
Archetype: A list of edges in a graph.
Definition graph_edges.hpp:47
Archetype: A list of nodes in a graph with optional labels, colors, etc.
Definition graph_nodes.hpp:49
GraphNodes with_labels(const Collection< rerun::components::Text > &_labels) &&
Optional text labels for the node.
Definition graph_nodes.hpp:145
GraphNodes with_positions(const Collection< rerun::components::Position2D > &_positions) &&
Optional center positions of the nodes.
Definition graph_nodes.hpp:132
◆ with_many_show_labels()
This method makes it possible to pack multiple show_labels
in a single component batch.
This only makes sense when used in conjunction with columns
. with_show_labels
should be used when logging a single row's worth of data.
◆ columns() [1/2]
◆ columns() [2/2]
Partitions the component data into unit-length sub-batches.
This is semantically similar to calling columns
with std::vector<uint32_t>(n, 1)
, where n
is automatically guessed.
◆ IndicatorComponentName
constexpr const char rerun::archetypes::GraphNodes::IndicatorComponentName[] |
|
staticconstexpr |
Initial value:=
"rerun.components.GraphNodesIndicator"
◆ Descriptor_node_ids
constexpr auto rerun::archetypes::GraphNodes::Descriptor_node_ids |
|
staticconstexpr |
Initial value:= ComponentDescriptor(
Loggable<rerun::components::GraphNode>::Descriptor.component_name
)
static constexpr const char ArchetypeName[]
The name of the archetype as used in ComponentDescriptors.
Definition graph_nodes.hpp:75
ComponentDescriptor
for the node_ids
field.
◆ Descriptor_positions
constexpr auto rerun::archetypes::GraphNodes::Descriptor_positions |
|
staticconstexpr |
Initial value:= ComponentDescriptor(
Loggable<rerun::components::Position2D>::Descriptor.component_name
)
ComponentDescriptor
for the positions
field.
◆ Descriptor_colors
constexpr auto rerun::archetypes::GraphNodes::Descriptor_colors |
|
staticconstexpr |
Initial value:= ComponentDescriptor(
ArchetypeName,
"colors", Loggable<rerun::components::Color>::Descriptor.component_name
)
ComponentDescriptor
for the colors
field.
◆ Descriptor_labels
constexpr auto rerun::archetypes::GraphNodes::Descriptor_labels |
|
staticconstexpr |
Initial value:= ComponentDescriptor(
ArchetypeName,
"labels", Loggable<rerun::components::Text>::Descriptor.component_name
)
ComponentDescriptor
for the labels
field.
◆ Descriptor_show_labels
constexpr auto rerun::archetypes::GraphNodes::Descriptor_show_labels |
|
staticconstexpr |
Initial value:= ComponentDescriptor(
Loggable<rerun::components::ShowLabels>::Descriptor.component_name
)
ComponentDescriptor
for the show_labels
field.
◆ Descriptor_radii
constexpr auto rerun::archetypes::GraphNodes::Descriptor_radii |
|
staticconstexpr |
Initial value:= ComponentDescriptor(
ArchetypeName,
"radii", Loggable<rerun::components::Radius>::Descriptor.component_name
)
ComponentDescriptor
for the radii
field.
The documentation for this struct was generated from the following file: