pub struct Points2D {
pub positions: Option<SerializedComponentBatch>,
pub radii: Option<SerializedComponentBatch>,
pub colors: Option<SerializedComponentBatch>,
pub labels: Option<SerializedComponentBatch>,
pub show_labels: Option<SerializedComponentBatch>,
pub draw_order: Option<SerializedComponentBatch>,
pub class_ids: Option<SerializedComponentBatch>,
pub keypoint_ids: Option<SerializedComponentBatch>,
}
Expand description
Archetype: A 2D point cloud with positions and optional colors, radii, labels, etc.
§Examples
§Randomly distributed 2D points with varying color and radius
use rand::{distributions::Uniform, Rng as _};
fn main() -> Result<(), Box<dyn std::error::Error>> {
let rec = rerun::RecordingStreamBuilder::new("rerun_example_points2d_random").spawn()?;
let mut rng = rand::thread_rng();
let dist = Uniform::new(-3., 3.);
rec.log(
"random",
&rerun::Points2D::new((0..10).map(|_| (rng.sample(dist), rng.sample(dist))))
.with_colors((0..10).map(|_| rerun::Color::from_rgb(rng.gen(), rng.gen(), rng.gen())))
.with_radii((0..10).map(|_| rng.gen::<f32>())),
)?;
// TODO(#5521): log VisualBounds2D
Ok(())
}
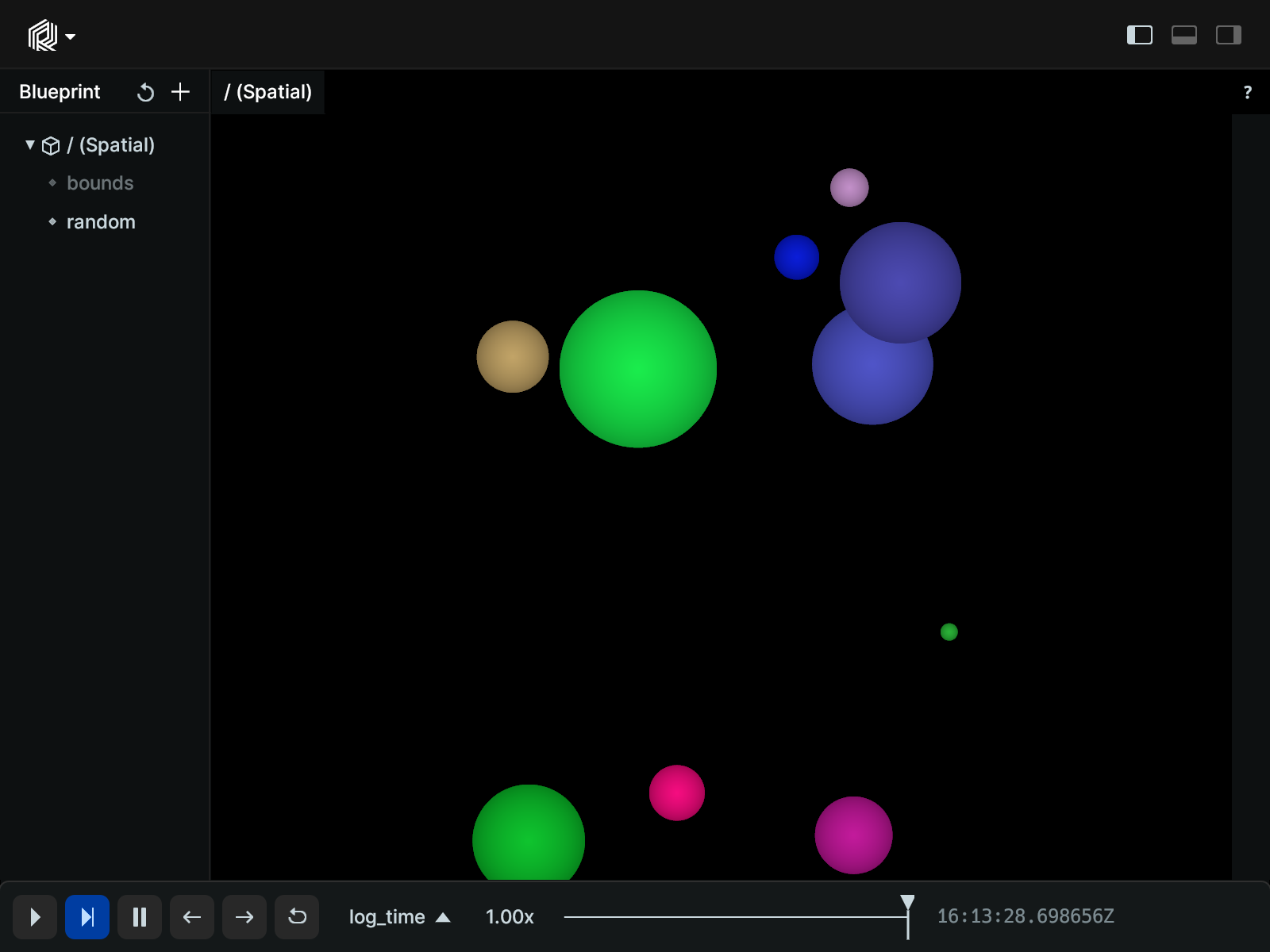
§Log points with radii given in UI points
fn main() -> Result<(), Box<dyn std::error::Error>> {
let rec = rerun::RecordingStreamBuilder::new("rerun_example_points2d_ui_radius").spawn()?;
// Two blue points with scene unit radii of 0.1 and 0.3.
rec.log(
"scene_units",
&rerun::Points2D::new([(0.0, 0.0), (0.0, 1.0)])
// By default, radii are interpreted as world-space units.
.with_radii([0.1, 0.3])
.with_colors([rerun::Color::from_rgb(0, 0, 255)]),
)?;
// Two red points with ui point radii of 40 and 60.
// UI points are independent of zooming in Views, but are sensitive to the application UI scaling.
// For 100% ui scaling, UI points are equal to pixels.
rec.log(
"ui_points",
&rerun::Points2D::new([(1.0, 0.0), (1.0, 1.0)])
// rerun::Radius::new_ui_points produces a radius that the viewer interprets as given in ui points.
.with_radii([
rerun::Radius::new_ui_points(40.0),
rerun::Radius::new_ui_points(60.0),
])
.with_colors([rerun::Color::from_rgb(255, 0, 0)]),
)?;
// TODO(#5521): log VisualBounds2D
Ok(())
}
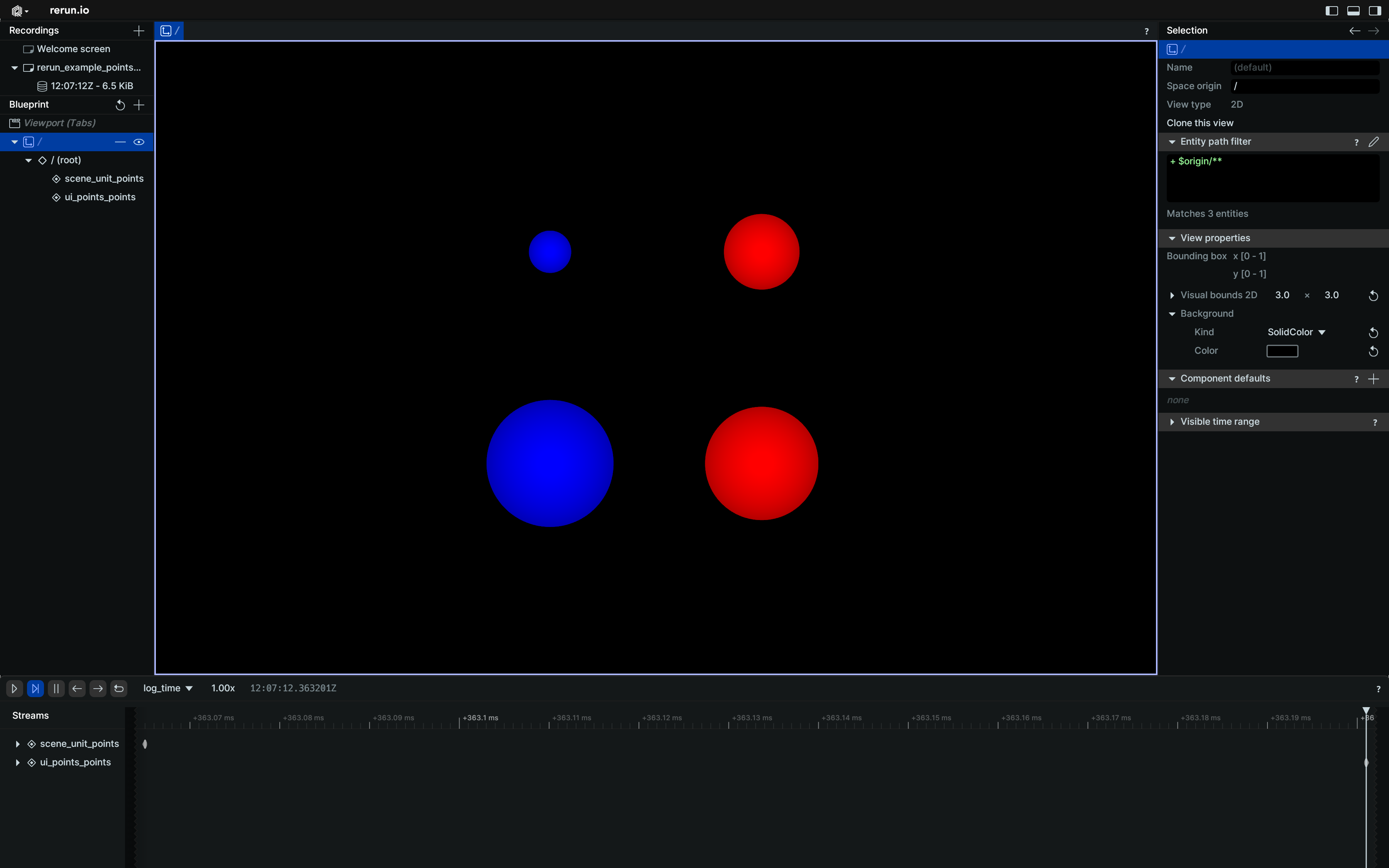
Fields§
§positions: Option<SerializedComponentBatch>
All the 2D positions at which the point cloud shows points.
radii: Option<SerializedComponentBatch>
Optional radii for the points, effectively turning them into circles.
colors: Option<SerializedComponentBatch>
Optional colors for the points.
labels: Option<SerializedComponentBatch>
Optional text labels for the points.
If there’s a single label present, it will be placed at the center of the entity. Otherwise, each instance will have its own label.
show_labels: Option<SerializedComponentBatch>
Optional choice of whether the text labels should be shown by default.
draw_order: Option<SerializedComponentBatch>
An optional floating point value that specifies the 2D drawing order.
Objects with higher values are drawn on top of those with lower values.
class_ids: Option<SerializedComponentBatch>
Optional class Ids for the points.
The components::ClassId
provides colors and labels if not specified explicitly.
keypoint_ids: Option<SerializedComponentBatch>
Optional keypoint IDs for the points, identifying them within a class.
If keypoint IDs are passed in but no components::ClassId
s were specified, the components::ClassId
will
default to 0.
This is useful to identify points within a single classification (which is identified
with class_id
).
E.g. the classification might be ‘Person’ and the keypoints refer to joints on a
detected skeleton.
Implementations§
source§impl Points2D
impl Points2D
sourcepub fn descriptor_positions() -> ComponentDescriptor
pub fn descriptor_positions() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::positions
.
sourcepub fn descriptor_radii() -> ComponentDescriptor
pub fn descriptor_radii() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::radii
.
sourcepub fn descriptor_colors() -> ComponentDescriptor
pub fn descriptor_colors() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::colors
.
sourcepub fn descriptor_labels() -> ComponentDescriptor
pub fn descriptor_labels() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::labels
.
sourcepub fn descriptor_show_labels() -> ComponentDescriptor
pub fn descriptor_show_labels() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::show_labels
.
sourcepub fn descriptor_draw_order() -> ComponentDescriptor
pub fn descriptor_draw_order() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::draw_order
.
sourcepub fn descriptor_class_ids() -> ComponentDescriptor
pub fn descriptor_class_ids() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::class_ids
.
sourcepub fn descriptor_keypoint_ids() -> ComponentDescriptor
pub fn descriptor_keypoint_ids() -> ComponentDescriptor
Returns the ComponentDescriptor
for Self::keypoint_ids
.
sourcepub fn descriptor_indicator() -> ComponentDescriptor
pub fn descriptor_indicator() -> ComponentDescriptor
Returns the ComponentDescriptor
for the associated indicator component.
source§impl Points2D
impl Points2D
sourcepub const NUM_COMPONENTS: usize = 9usize
pub const NUM_COMPONENTS: usize = 9usize
The total number of components in the archetype: 1 required, 3 recommended, 5 optional
source§impl Points2D
impl Points2D
sourcepub fn new(
positions: impl IntoIterator<Item = impl Into<Position2D>>,
) -> Points2D
pub fn new( positions: impl IntoIterator<Item = impl Into<Position2D>>, ) -> Points2D
Create a new Points2D
.
sourcepub fn update_fields() -> Points2D
pub fn update_fields() -> Points2D
Update only some specific fields of a Points2D
.
sourcepub fn clear_fields() -> Points2D
pub fn clear_fields() -> Points2D
Clear all the fields of a Points2D
.
sourcepub fn columns<I>(
self,
_lengths: I,
) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
pub fn columns<I>( self, _lengths: I, ) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
Partitions the component data into multiple sub-batches.
Specifically, this transforms the existing SerializedComponentBatch
es data into SerializedComponentColumn
s
instead, via SerializedComponentBatch::partitioned
.
This makes it possible to use RecordingStream::send_columns
to send columnar data directly into Rerun.
The specified lengths
must sum to the total length of the component batch.
sourcepub fn columns_of_unit_batches(
self,
) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
pub fn columns_of_unit_batches( self, ) -> Result<impl Iterator<Item = SerializedComponentColumn>, SerializationError>
Helper to partition the component data into unit-length sub-batches.
This is semantically similar to calling Self::columns
with std::iter::take(1).repeat(n)
,
where n
is automatically guessed.
sourcepub fn with_positions(
self,
positions: impl IntoIterator<Item = impl Into<Position2D>>,
) -> Points2D
pub fn with_positions( self, positions: impl IntoIterator<Item = impl Into<Position2D>>, ) -> Points2D
All the 2D positions at which the point cloud shows points.
sourcepub fn with_radii(
self,
radii: impl IntoIterator<Item = impl Into<Radius>>,
) -> Points2D
pub fn with_radii( self, radii: impl IntoIterator<Item = impl Into<Radius>>, ) -> Points2D
Optional radii for the points, effectively turning them into circles.
sourcepub fn with_colors(
self,
colors: impl IntoIterator<Item = impl Into<Color>>,
) -> Points2D
pub fn with_colors( self, colors: impl IntoIterator<Item = impl Into<Color>>, ) -> Points2D
Optional colors for the points.
sourcepub fn with_labels(
self,
labels: impl IntoIterator<Item = impl Into<Text>>,
) -> Points2D
pub fn with_labels( self, labels: impl IntoIterator<Item = impl Into<Text>>, ) -> Points2D
Optional text labels for the points.
If there’s a single label present, it will be placed at the center of the entity. Otherwise, each instance will have its own label.
sourcepub fn with_show_labels(self, show_labels: impl Into<ShowLabels>) -> Points2D
pub fn with_show_labels(self, show_labels: impl Into<ShowLabels>) -> Points2D
Optional choice of whether the text labels should be shown by default.
sourcepub fn with_many_show_labels(
self,
show_labels: impl IntoIterator<Item = impl Into<ShowLabels>>,
) -> Points2D
pub fn with_many_show_labels( self, show_labels: impl IntoIterator<Item = impl Into<ShowLabels>>, ) -> Points2D
This method makes it possible to pack multiple crate::components::ShowLabels
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_show_labels
should
be used when logging a single row’s worth of data.
sourcepub fn with_draw_order(self, draw_order: impl Into<DrawOrder>) -> Points2D
pub fn with_draw_order(self, draw_order: impl Into<DrawOrder>) -> Points2D
An optional floating point value that specifies the 2D drawing order.
Objects with higher values are drawn on top of those with lower values.
sourcepub fn with_many_draw_order(
self,
draw_order: impl IntoIterator<Item = impl Into<DrawOrder>>,
) -> Points2D
pub fn with_many_draw_order( self, draw_order: impl IntoIterator<Item = impl Into<DrawOrder>>, ) -> Points2D
This method makes it possible to pack multiple crate::components::DrawOrder
in a single component batch.
This only makes sense when used in conjunction with Self::columns
. Self::with_draw_order
should
be used when logging a single row’s worth of data.
sourcepub fn with_class_ids(
self,
class_ids: impl IntoIterator<Item = impl Into<ClassId>>,
) -> Points2D
pub fn with_class_ids( self, class_ids: impl IntoIterator<Item = impl Into<ClassId>>, ) -> Points2D
Optional class Ids for the points.
The components::ClassId
provides colors and labels if not specified explicitly.
sourcepub fn with_keypoint_ids(
self,
keypoint_ids: impl IntoIterator<Item = impl Into<KeypointId>>,
) -> Points2D
pub fn with_keypoint_ids( self, keypoint_ids: impl IntoIterator<Item = impl Into<KeypointId>>, ) -> Points2D
Optional keypoint IDs for the points, identifying them within a class.
If keypoint IDs are passed in but no components::ClassId
s were specified, the components::ClassId
will
default to 0.
This is useful to identify points within a single classification (which is identified
with class_id
).
E.g. the classification might be ‘Person’ and the keypoints refer to joints on a
detected skeleton.
Trait Implementations§
source§impl Archetype for Points2D
impl Archetype for Points2D
§type Indicator = GenericIndicatorComponent<Points2D>
type Indicator = GenericIndicatorComponent<Points2D>
source§fn name() -> ArchetypeName
fn name() -> ArchetypeName
rerun.archetypes.Points2D
.source§fn display_name() -> &'static str
fn display_name() -> &'static str
source§fn required_components() -> Cow<'static, [ComponentDescriptor]>
fn required_components() -> Cow<'static, [ComponentDescriptor]>
source§fn recommended_components() -> Cow<'static, [ComponentDescriptor]>
fn recommended_components() -> Cow<'static, [ComponentDescriptor]>
source§fn optional_components() -> Cow<'static, [ComponentDescriptor]>
fn optional_components() -> Cow<'static, [ComponentDescriptor]>
source§fn all_components() -> Cow<'static, [ComponentDescriptor]>
fn all_components() -> Cow<'static, [ComponentDescriptor]>
source§fn from_arrow_components(
arrow_data: impl IntoIterator<Item = (ComponentDescriptor, Arc<dyn Array>)>,
) -> Result<Points2D, DeserializationError>
fn from_arrow_components( arrow_data: impl IntoIterator<Item = (ComponentDescriptor, Arc<dyn Array>)>, ) -> Result<Points2D, DeserializationError>
ComponentNames
, deserializes them
into this archetype. Read moresource§fn from_arrow(
data: impl IntoIterator<Item = (Field, Arc<dyn Array>)>,
) -> Result<Self, DeserializationError>where
Self: Sized,
fn from_arrow(
data: impl IntoIterator<Item = (Field, Arc<dyn Array>)>,
) -> Result<Self, DeserializationError>where
Self: Sized,
source§impl AsComponents for Points2D
impl AsComponents for Points2D
source§fn as_serialized_batches(&self) -> Vec<SerializedComponentBatch>
fn as_serialized_batches(&self) -> Vec<SerializedComponentBatch>
SerializedComponentBatch
es. Read moresource§impl PartialEq for Points2D
impl PartialEq for Points2D
source§impl SizeBytes for Points2D
impl SizeBytes for Points2D
source§fn heap_size_bytes(&self) -> u64
fn heap_size_bytes(&self) -> u64
self
uses on the heap. Read moresource§fn total_size_bytes(&self) -> u64
fn total_size_bytes(&self) -> u64
self
in bytes, accounting for both stack and heap space.source§fn stack_size_bytes(&self) -> u64
fn stack_size_bytes(&self) -> u64
self
on the stack, in bytes. Read moreimpl ArchetypeReflectionMarker for Points2D
impl StructuralPartialEq for Points2D
Auto Trait Implementations§
impl Freeze for Points2D
impl !RefUnwindSafe for Points2D
impl Send for Points2D
impl Sync for Points2D
impl Unpin for Points2D
impl !UnwindSafe for Points2D
Blanket Implementations§
source§impl<T> BorrowMut<T> for Twhere
T: ?Sized,
impl<T> BorrowMut<T> for Twhere
T: ?Sized,
source§fn borrow_mut(&mut self) -> &mut T
fn borrow_mut(&mut self) -> &mut T
source§impl<T> CheckedAs for T
impl<T> CheckedAs for T
source§fn checked_as<Dst>(self) -> Option<Dst>where
T: CheckedCast<Dst>,
fn checked_as<Dst>(self) -> Option<Dst>where
T: CheckedCast<Dst>,
source§impl<Src, Dst> CheckedCastFrom<Src> for Dstwhere
Src: CheckedCast<Dst>,
impl<Src, Dst> CheckedCastFrom<Src> for Dstwhere
Src: CheckedCast<Dst>,
source§fn checked_cast_from(src: Src) -> Option<Dst>
fn checked_cast_from(src: Src) -> Option<Dst>
source§impl<T> CloneToUninit for Twhere
T: Clone,
impl<T> CloneToUninit for Twhere
T: Clone,
source§default unsafe fn clone_to_uninit(&self, dst: *mut T)
default unsafe fn clone_to_uninit(&self, dst: *mut T)
clone_to_uninit
)§impl<T> Conv for T
impl<T> Conv for T
§impl<T> Downcast for Twhere
T: Any,
impl<T> Downcast for Twhere
T: Any,
§fn into_any(self: Box<T>) -> Box<dyn Any>
fn into_any(self: Box<T>) -> Box<dyn Any>
Box<dyn Trait>
(where Trait: Downcast
) to Box<dyn Any>
. Box<dyn Any>
can
then be further downcast
into Box<ConcreteType>
where ConcreteType
implements Trait
.§fn into_any_rc(self: Rc<T>) -> Rc<dyn Any>
fn into_any_rc(self: Rc<T>) -> Rc<dyn Any>
Rc<Trait>
(where Trait: Downcast
) to Rc<Any>
. Rc<Any>
can then be
further downcast
into Rc<ConcreteType>
where ConcreteType
implements Trait
.§fn as_any(&self) -> &(dyn Any + 'static)
fn as_any(&self) -> &(dyn Any + 'static)
&Trait
(where Trait: Downcast
) to &Any
. This is needed since Rust cannot
generate &Any
’s vtable from &Trait
’s.§fn as_any_mut(&mut self) -> &mut (dyn Any + 'static)
fn as_any_mut(&mut self) -> &mut (dyn Any + 'static)
&mut Trait
(where Trait: Downcast
) to &Any
. This is needed since Rust cannot
generate &mut Any
’s vtable from &mut Trait
’s.§impl<T> DowncastSync for T
impl<T> DowncastSync for T
§impl<T> Instrument for T
impl<T> Instrument for T
§fn instrument(self, span: Span) -> Instrumented<Self>
fn instrument(self, span: Span) -> Instrumented<Self>
§fn in_current_span(self) -> Instrumented<Self>
fn in_current_span(self) -> Instrumented<Self>
source§impl<T> IntoEither for T
impl<T> IntoEither for T
source§fn into_either(self, into_left: bool) -> Either<Self, Self>
fn into_either(self, into_left: bool) -> Either<Self, Self>
self
into a Left
variant of Either<Self, Self>
if into_left
is true
.
Converts self
into a Right
variant of Either<Self, Self>
otherwise. Read moresource§fn into_either_with<F>(self, into_left: F) -> Either<Self, Self>
fn into_either_with<F>(self, into_left: F) -> Either<Self, Self>
self
into a Left
variant of Either<Self, Self>
if into_left(&self)
returns true
.
Converts self
into a Right
variant of Either<Self, Self>
otherwise. Read moresource§impl<T> IntoRequest<T> for T
impl<T> IntoRequest<T> for T
source§fn into_request(self) -> Request<T>
fn into_request(self) -> Request<T>
T
in a tonic::Request
source§impl<Src, Dst> LosslessTryInto<Dst> for Srcwhere
Dst: LosslessTryFrom<Src>,
impl<Src, Dst> LosslessTryInto<Dst> for Srcwhere
Dst: LosslessTryFrom<Src>,
source§fn lossless_try_into(self) -> Option<Dst>
fn lossless_try_into(self) -> Option<Dst>
source§impl<Src, Dst> LossyInto<Dst> for Srcwhere
Dst: LossyFrom<Src>,
impl<Src, Dst> LossyInto<Dst> for Srcwhere
Dst: LossyFrom<Src>,
source§fn lossy_into(self) -> Dst
fn lossy_into(self) -> Dst
§impl<T> NoneValue for Twhere
T: Default,
impl<T> NoneValue for Twhere
T: Default,
type NoneType = T
§fn null_value() -> T
fn null_value() -> T
source§impl<T> OverflowingAs for T
impl<T> OverflowingAs for T
source§fn overflowing_as<Dst>(self) -> (Dst, bool)where
T: OverflowingCast<Dst>,
fn overflowing_as<Dst>(self) -> (Dst, bool)where
T: OverflowingCast<Dst>,
source§impl<Src, Dst> OverflowingCastFrom<Src> for Dstwhere
Src: OverflowingCast<Dst>,
impl<Src, Dst> OverflowingCastFrom<Src> for Dstwhere
Src: OverflowingCast<Dst>,
source§fn overflowing_cast_from(src: Src) -> (Dst, bool)
fn overflowing_cast_from(src: Src) -> (Dst, bool)
§impl<T> Pipe for Twhere
T: ?Sized,
impl<T> Pipe for Twhere
T: ?Sized,
§fn pipe<R>(self, func: impl FnOnce(Self) -> R) -> Rwhere
Self: Sized,
fn pipe<R>(self, func: impl FnOnce(Self) -> R) -> Rwhere
Self: Sized,
§fn pipe_ref<'a, R>(&'a self, func: impl FnOnce(&'a Self) -> R) -> Rwhere
R: 'a,
fn pipe_ref<'a, R>(&'a self, func: impl FnOnce(&'a Self) -> R) -> Rwhere
R: 'a,
self
and passes that borrow into the pipe function. Read more§fn pipe_ref_mut<'a, R>(&'a mut self, func: impl FnOnce(&'a mut Self) -> R) -> Rwhere
R: 'a,
fn pipe_ref_mut<'a, R>(&'a mut self, func: impl FnOnce(&'a mut Self) -> R) -> Rwhere
R: 'a,
self
and passes that borrow into the pipe function. Read more§fn pipe_borrow<'a, B, R>(&'a self, func: impl FnOnce(&'a B) -> R) -> R
fn pipe_borrow<'a, B, R>(&'a self, func: impl FnOnce(&'a B) -> R) -> R
§fn pipe_borrow_mut<'a, B, R>(
&'a mut self,
func: impl FnOnce(&'a mut B) -> R,
) -> R
fn pipe_borrow_mut<'a, B, R>( &'a mut self, func: impl FnOnce(&'a mut B) -> R, ) -> R
§fn pipe_as_ref<'a, U, R>(&'a self, func: impl FnOnce(&'a U) -> R) -> R
fn pipe_as_ref<'a, U, R>(&'a self, func: impl FnOnce(&'a U) -> R) -> R
self
, then passes self.as_ref()
into the pipe function.§fn pipe_as_mut<'a, U, R>(&'a mut self, func: impl FnOnce(&'a mut U) -> R) -> R
fn pipe_as_mut<'a, U, R>(&'a mut self, func: impl FnOnce(&'a mut U) -> R) -> R
self
, then passes self.as_mut()
into the pipe
function.§fn pipe_deref<'a, T, R>(&'a self, func: impl FnOnce(&'a T) -> R) -> R
fn pipe_deref<'a, T, R>(&'a self, func: impl FnOnce(&'a T) -> R) -> R
self
, then passes self.deref()
into the pipe function.§impl<T> Pointable for T
impl<T> Pointable for T
source§impl<T> SaturatingAs for T
impl<T> SaturatingAs for T
source§fn saturating_as<Dst>(self) -> Dstwhere
T: SaturatingCast<Dst>,
fn saturating_as<Dst>(self) -> Dstwhere
T: SaturatingCast<Dst>,
source§impl<Src, Dst> SaturatingCastFrom<Src> for Dstwhere
Src: SaturatingCast<Dst>,
impl<Src, Dst> SaturatingCastFrom<Src> for Dstwhere
Src: SaturatingCast<Dst>,
source§fn saturating_cast_from(src: Src) -> Dst
fn saturating_cast_from(src: Src) -> Dst
§impl<T> Tap for T
impl<T> Tap for T
§fn tap_borrow<B>(self, func: impl FnOnce(&B)) -> Self
fn tap_borrow<B>(self, func: impl FnOnce(&B)) -> Self
Borrow<B>
of a value. Read more§fn tap_borrow_mut<B>(self, func: impl FnOnce(&mut B)) -> Self
fn tap_borrow_mut<B>(self, func: impl FnOnce(&mut B)) -> Self
BorrowMut<B>
of a value. Read more§fn tap_ref<R>(self, func: impl FnOnce(&R)) -> Self
fn tap_ref<R>(self, func: impl FnOnce(&R)) -> Self
AsRef<R>
view of a value. Read more§fn tap_ref_mut<R>(self, func: impl FnOnce(&mut R)) -> Self
fn tap_ref_mut<R>(self, func: impl FnOnce(&mut R)) -> Self
AsMut<R>
view of a value. Read more§fn tap_deref<T>(self, func: impl FnOnce(&T)) -> Self
fn tap_deref<T>(self, func: impl FnOnce(&T)) -> Self
Deref::Target
of a value. Read more§fn tap_deref_mut<T>(self, func: impl FnOnce(&mut T)) -> Self
fn tap_deref_mut<T>(self, func: impl FnOnce(&mut T)) -> Self
Deref::Target
of a value. Read more§fn tap_dbg(self, func: impl FnOnce(&Self)) -> Self
fn tap_dbg(self, func: impl FnOnce(&Self)) -> Self
.tap()
only in debug builds, and is erased in release builds.§fn tap_mut_dbg(self, func: impl FnOnce(&mut Self)) -> Self
fn tap_mut_dbg(self, func: impl FnOnce(&mut Self)) -> Self
.tap_mut()
only in debug builds, and is erased in release
builds.§fn tap_borrow_dbg<B>(self, func: impl FnOnce(&B)) -> Self
fn tap_borrow_dbg<B>(self, func: impl FnOnce(&B)) -> Self
.tap_borrow()
only in debug builds, and is erased in release
builds.§fn tap_borrow_mut_dbg<B>(self, func: impl FnOnce(&mut B)) -> Self
fn tap_borrow_mut_dbg<B>(self, func: impl FnOnce(&mut B)) -> Self
.tap_borrow_mut()
only in debug builds, and is erased in release
builds.§fn tap_ref_dbg<R>(self, func: impl FnOnce(&R)) -> Self
fn tap_ref_dbg<R>(self, func: impl FnOnce(&R)) -> Self
.tap_ref()
only in debug builds, and is erased in release
builds.§fn tap_ref_mut_dbg<R>(self, func: impl FnOnce(&mut R)) -> Self
fn tap_ref_mut_dbg<R>(self, func: impl FnOnce(&mut R)) -> Self
.tap_ref_mut()
only in debug builds, and is erased in release
builds.§fn tap_deref_dbg<T>(self, func: impl FnOnce(&T)) -> Self
fn tap_deref_dbg<T>(self, func: impl FnOnce(&T)) -> Self
.tap_deref()
only in debug builds, and is erased in release
builds.