Archetype: 2D arrows with optional colors, radii, labels, etc.
More...
#include <rerun/archetypes/arrows2d.hpp>
Archetype: 2D arrows with optional colors, radii, labels, etc.
Example
Simple batch of 2D arrows
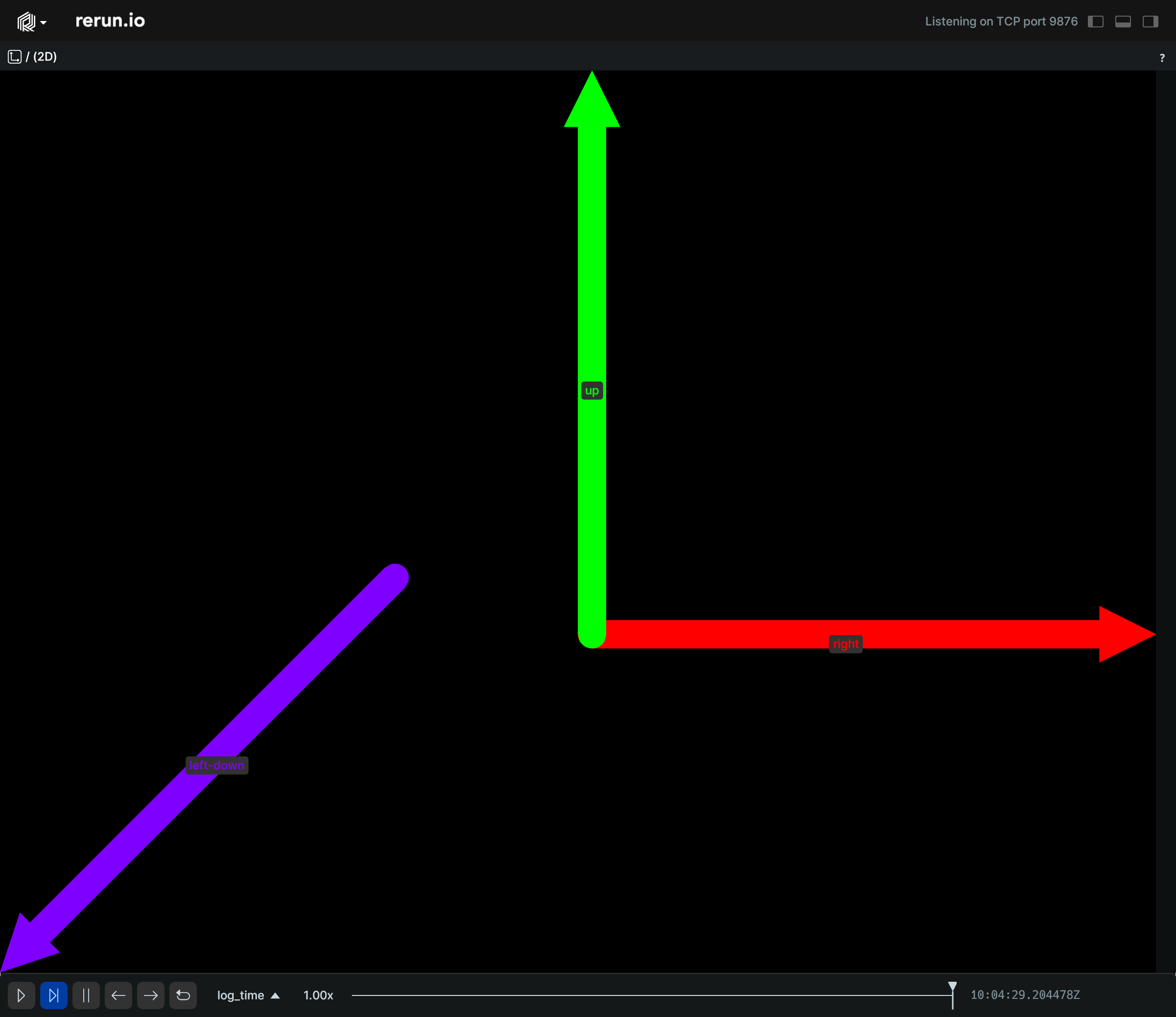
#include <rerun.hpp>
int main() {
rec.spawn().exit_on_failure();
rec.log(
"arrows",
rerun::Arrows2D::from_vectors({{1.0f, 0.0f}, {0.0f, -1.0f}, {-0.7f, 0.7f}})
.
with_origins({{0.25f, 0.0f}, {0.25f, 0.0f}, {-0.1f, -0.1f}})
.
with_colors({{255, 0, 0}, {0, 255, 0}, {127, 0, 255}})
);
}
A RecordingStream handles everything related to logging data into Rerun.
Definition recording_stream.hpp:60
Arrows2D with_colors(const Collection< rerun::components::Color > &_colors) &&
Optional colors for the points.
Definition arrows2d.hpp:180
Arrows2D with_labels(const Collection< rerun::components::Text > &_labels) &&
Optional text labels for the arrows.
Definition arrows2d.hpp:189
Arrows2D with_radii(const Collection< rerun::components::Radius > &_radii) &&
Optional radii for the arrows.
Definition arrows2d.hpp:174
Arrows2D with_origins(const Collection< rerun::components::Position2D > &_origins) &&
All the origin (base) positions for each arrow in the batch.
Definition arrows2d.hpp:165
◆ with_origins()
All the origin (base) positions for each arrow in the batch.
If no origins are set, (0, 0) is used as the origin for each arrow.
◆ with_radii()
Optional radii for the arrows.
The shaft is rendered as a line with radius = 0.5 * radius
. The tip is rendered with height = 2.0 * radius
and radius = 1.0 * radius
.
◆ with_labels()
Optional text labels for the arrows.
If there's a single label present, it will be placed at the center of the entity. Otherwise, each instance will have its own label.
◆ with_many_show_labels()
This method makes it possible to pack multiple show_labels
in a single component batch.
This only makes sense when used in conjunction with columns
. with_show_labels
should be used when logging a single row's worth of data.
◆ with_draw_order()
An optional floating point value that specifies the 2D drawing order.
Objects with higher values are drawn on top of those with lower values.
◆ with_many_draw_order()
This method makes it possible to pack multiple draw_order
in a single component batch.
This only makes sense when used in conjunction with columns
. with_draw_order
should be used when logging a single row's worth of data.
◆ with_class_ids()
Optional class Ids for the points.
The components::ClassId
provides colors and labels if not specified explicitly.
◆ columns() [1/2]
◆ columns() [2/2]
Partitions the component data into unit-length sub-batches.
This is semantically similar to calling columns
with std::vector<uint32_t>(n, 1)
, where n
is automatically guessed.
◆ origins
All the origin (base) positions for each arrow in the batch.
If no origins are set, (0, 0) is used as the origin for each arrow.
◆ radii
Optional radii for the arrows.
The shaft is rendered as a line with radius = 0.5 * radius
. The tip is rendered with height = 2.0 * radius
and radius = 1.0 * radius
.
◆ labels
Optional text labels for the arrows.
If there's a single label present, it will be placed at the center of the entity. Otherwise, each instance will have its own label.
◆ draw_order
An optional floating point value that specifies the 2D drawing order.
Objects with higher values are drawn on top of those with lower values.
◆ class_ids
Optional class Ids for the points.
The components::ClassId
provides colors and labels if not specified explicitly.
◆ Descriptor_vectors
constexpr auto rerun::archetypes::Arrows2D::Descriptor_vectors |
|
staticconstexpr |
Initial value:= ComponentDescriptor(
Loggable<rerun::components::Vector2D>::Descriptor.component_name
)
static constexpr const char ArchetypeName[]
The name of the archetype as used in ComponentDescriptors.
Definition arrows2d.hpp:93
ComponentDescriptor
for the vectors
field.
◆ Descriptor_origins
constexpr auto rerun::archetypes::Arrows2D::Descriptor_origins |
|
staticconstexpr |
Initial value:= ComponentDescriptor(
Loggable<rerun::components::Position2D>::Descriptor.component_name
)
ComponentDescriptor
for the origins
field.
◆ Descriptor_radii
constexpr auto rerun::archetypes::Arrows2D::Descriptor_radii |
|
staticconstexpr |
Initial value:= ComponentDescriptor(
ArchetypeName,
"radii", Loggable<rerun::components::Radius>::Descriptor.component_name
)
ComponentDescriptor
for the radii
field.
◆ Descriptor_colors
constexpr auto rerun::archetypes::Arrows2D::Descriptor_colors |
|
staticconstexpr |
Initial value:= ComponentDescriptor(
ArchetypeName,
"colors", Loggable<rerun::components::Color>::Descriptor.component_name
)
ComponentDescriptor
for the colors
field.
◆ Descriptor_labels
constexpr auto rerun::archetypes::Arrows2D::Descriptor_labels |
|
staticconstexpr |
Initial value:= ComponentDescriptor(
ArchetypeName,
"labels", Loggable<rerun::components::Text>::Descriptor.component_name
)
ComponentDescriptor
for the labels
field.
◆ Descriptor_show_labels
constexpr auto rerun::archetypes::Arrows2D::Descriptor_show_labels |
|
staticconstexpr |
Initial value:= ComponentDescriptor(
Loggable<rerun::components::ShowLabels>::Descriptor.component_name
)
ComponentDescriptor
for the show_labels
field.
◆ Descriptor_draw_order
constexpr auto rerun::archetypes::Arrows2D::Descriptor_draw_order |
|
staticconstexpr |
Initial value:= ComponentDescriptor(
Loggable<rerun::components::DrawOrder>::Descriptor.component_name
)
ComponentDescriptor
for the draw_order
field.
◆ Descriptor_class_ids
constexpr auto rerun::archetypes::Arrows2D::Descriptor_class_ids |
|
staticconstexpr |
Initial value:= ComponentDescriptor(
Loggable<rerun::components::ClassId>::Descriptor.component_name
)
ComponentDescriptor
for the class_ids
field.
The documentation for this struct was generated from the following file: