Archetype: A 3D point cloud with positions and optional colors, radii, labels, etc.
Examples
Simple 3D points
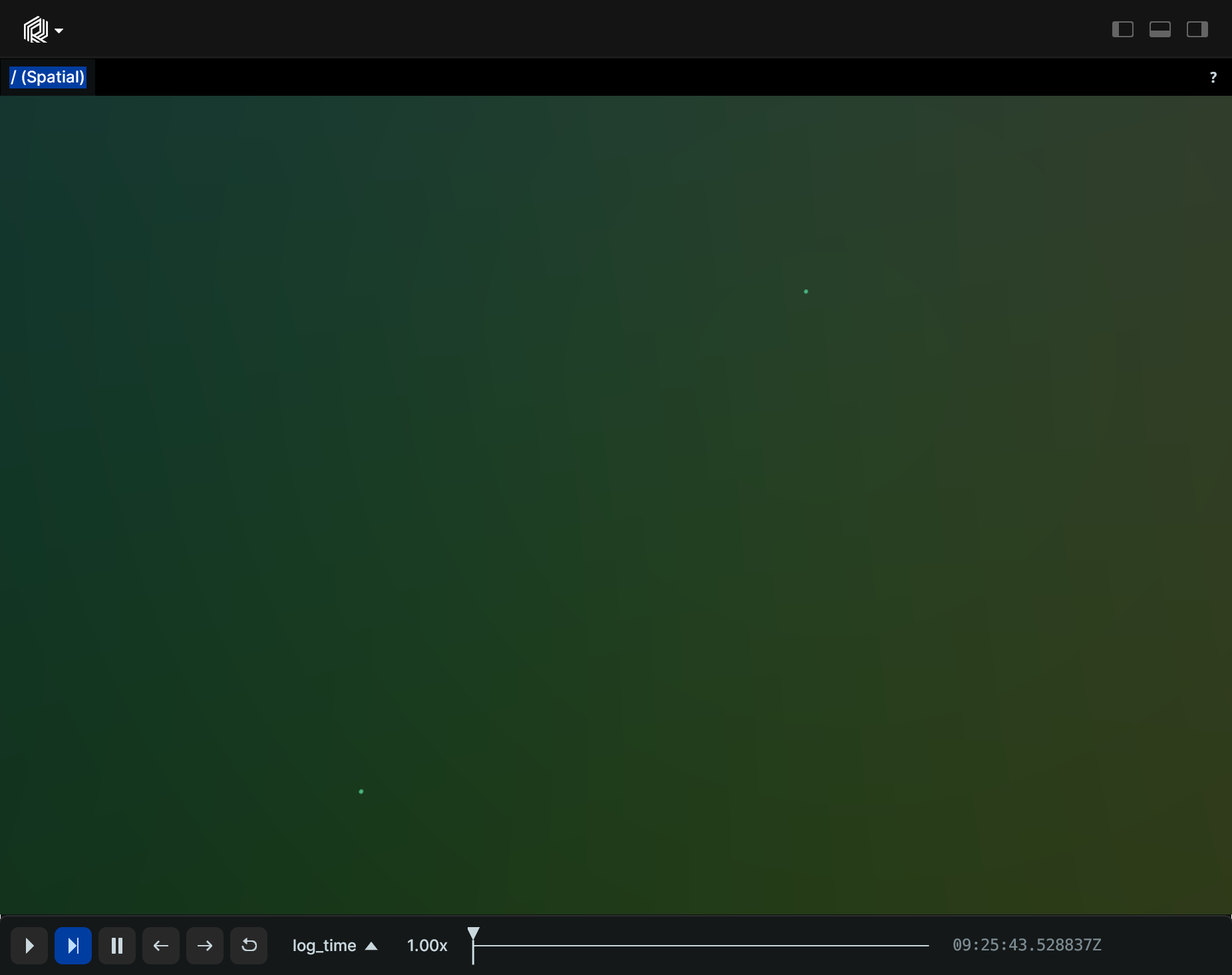
#include <rerun.hpp>
int main() {
rec.spawn().exit_on_failure();
rec.log(
"points",
rerun::Points3D({{0.0f, 0.0f, 0.0f}, {1.0f, 1.0f, 1.0f}}));
}
A RecordingStream handles everything related to logging data into Rerun.
Definition recording_stream.hpp:60
Archetype: A 3D point cloud with positions and optional colors, radii, labels, etc.
Definition points3d.hpp:175
Update a point cloud over time
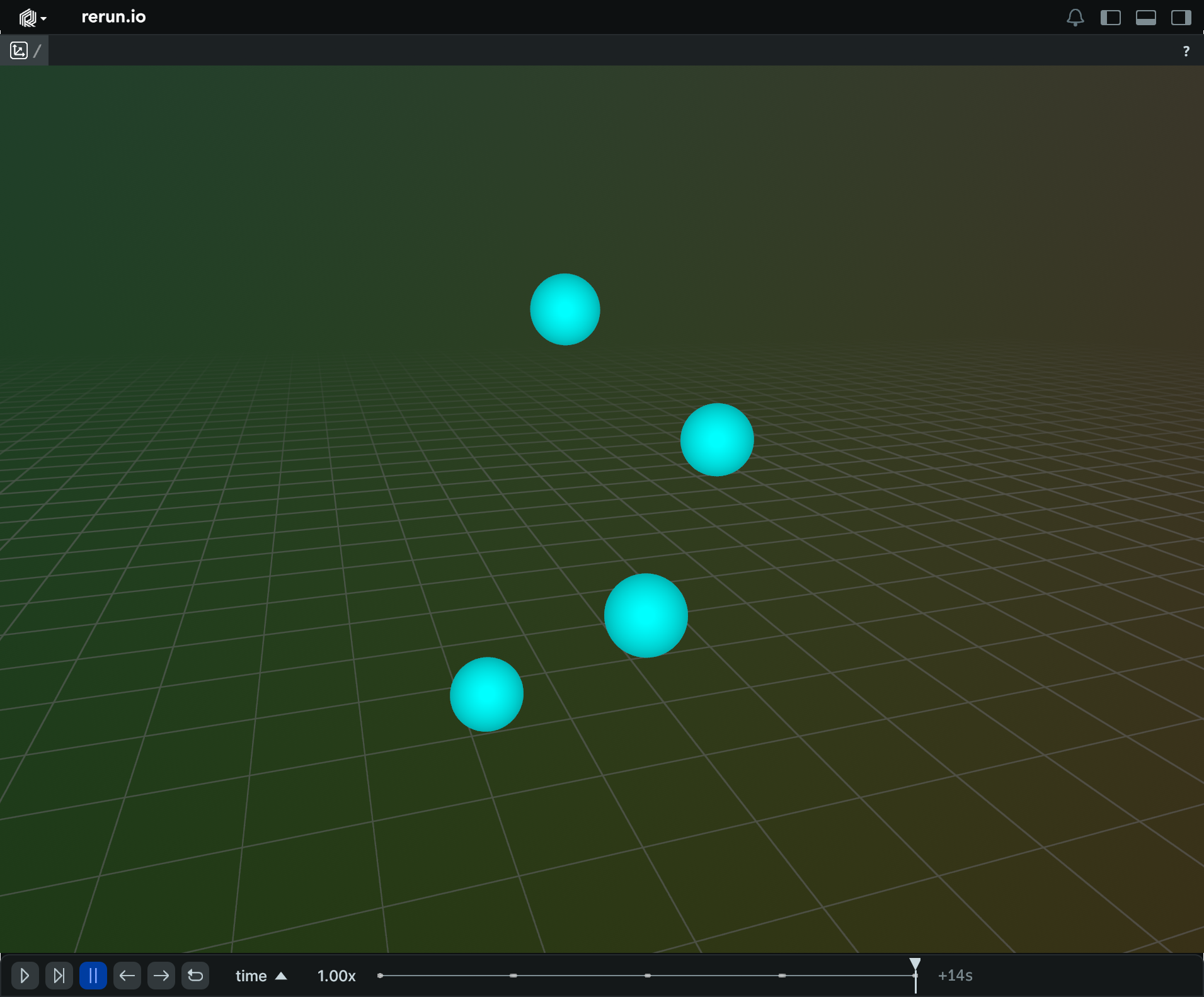
#include <rerun.hpp>
#include <algorithm>
#include <vector>
int main() {
rec.spawn().exit_on_failure();
std::vector<std::array<float, 3>>
positions[] = {
{{1.0, 0.0, 1.0}, {0.5, 0.5, 2.0}},
{{1.5, -0.5, 1.5}, {1.0, 1.0, 2.5}, {-0.5, 1.5, 1.0}, {-1.5, 0.0, 2.0}},
{{2.0, 0.0, 2.0}, {1.5, -1.5, 3.0}, {0.0, -2.0, 2.5}, {1.0, -1.0, 3.5}},
{{-2.0, 0.0, 2.0}, {-1.5, 1.5, 3.0}, {-1.0, 1.0, 3.5}},
{{1.0, -1.0, 1.0}, {2.0, -2.0, 2.0}, {3.0, -1.0, 3.0}, {2.0, 0.0, 4.0}},
};
std::vector<uint32_t>
colors = {0xFF0000FF, 0x00FF00FF, 0x0000FFFF, 0xFFFF00FF, 0x00FFFFFF};
std::vector<float>
radii = {0.05f, 0.01f, 0.2f, 0.1f, 0.3f};
for (size_t i = 0; i <5; i++) {
rec.set_time_duration_secs("time", 10.0 + static_cast<double>(i));
rec.log(
"points",
);
}
}
std::optional< ComponentBatch > positions
All the 3D positions at which the point cloud shows points.
Definition points3d.hpp:177
Points3D with_radii(const Collection< rerun::components::Radius > &_radii) &&
Optional radii for the points, effectively turning them into circles.
Definition points3d.hpp:277
std::optional< ComponentBatch > radii
Optional radii for the points, effectively turning them into circles.
Definition points3d.hpp:180
Points3D with_colors(const Collection< rerun::components::Color > &_colors) &&
Optional colors for the points.
Definition points3d.hpp:283
std::optional< ComponentBatch > colors
Optional colors for the points.
Definition points3d.hpp:183
Update a point cloud over time, in a single operation
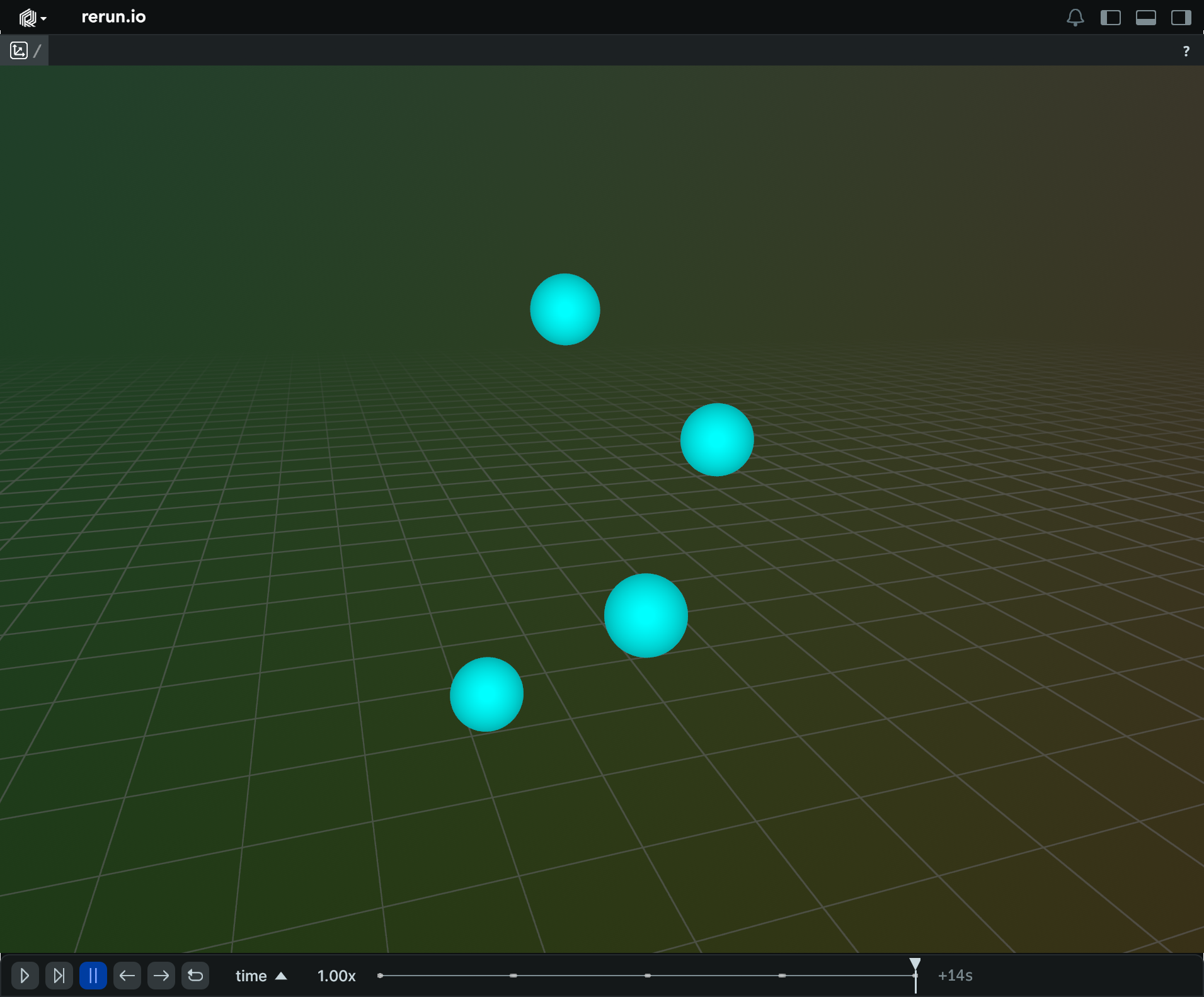
#include <array>
#include <rerun.hpp>
#include <vector>
using namespace std::chrono_literals;
int main() {
rec.spawn().exit_on_failure();
std::vector<std::array<float, 3>>
positions = {
{1.0, 0.0, 1.0}, {0.5, 0.5, 2.0},
{1.5, -0.5, 1.5}, {1.0, 1.0, 2.5}, {-0.5, 1.5, 1.0}, {-1.5, 0.0, 2.0},
{2.0, 0.0, 2.0}, {1.5, -1.5, 3.0}, {0.0, -2.0, 2.5}, {1.0, -1.0, 3.5},
{-2.0, 0.0, 2.0}, {-1.5, 1.5, 3.0}, {-1.0, 1.0, 3.5},
{1.0, -1.0, 1.0}, {2.0, -2.0, 2.0}, {3.0, -1.0, 3.0}, {2.0, 0.0, 4.0},
};
std::vector<uint32_t>
colors = {0xFF0000FF, 0x00FF00FF, 0x0000FFFF, 0xFFFF00FF, 0x00FFFFFF};
std::vector<float>
radii = {0.05f, 0.01f, 0.2f, 0.1f, 0.3f};
rec.send_columns("points", time_column, position, color_and_radius);
}
Generic collection of elements that are roughly contiguous in memory.
Definition collection.hpp:49
static TimeColumn from_durations(std::string timeline_name, const Collection< std::chrono::duration< TRep, TPeriod > > &durations, SortingStatus sorting_status=SortingStatus::Unknown)
Creates a time column from an array of arbitrary std::chrono durations.
Definition time_column.hpp:148
Points3D with_positions(const Collection< rerun::components::Position3D > &_positions) &&
All the 3D positions at which the point cloud shows points.
Definition points3d.hpp:270
Collection< ComponentColumn > columns(const Collection< uint32_t > &lengths_)
Partitions the component data into multiple sub-batches.
Update specific properties of a point cloud over time
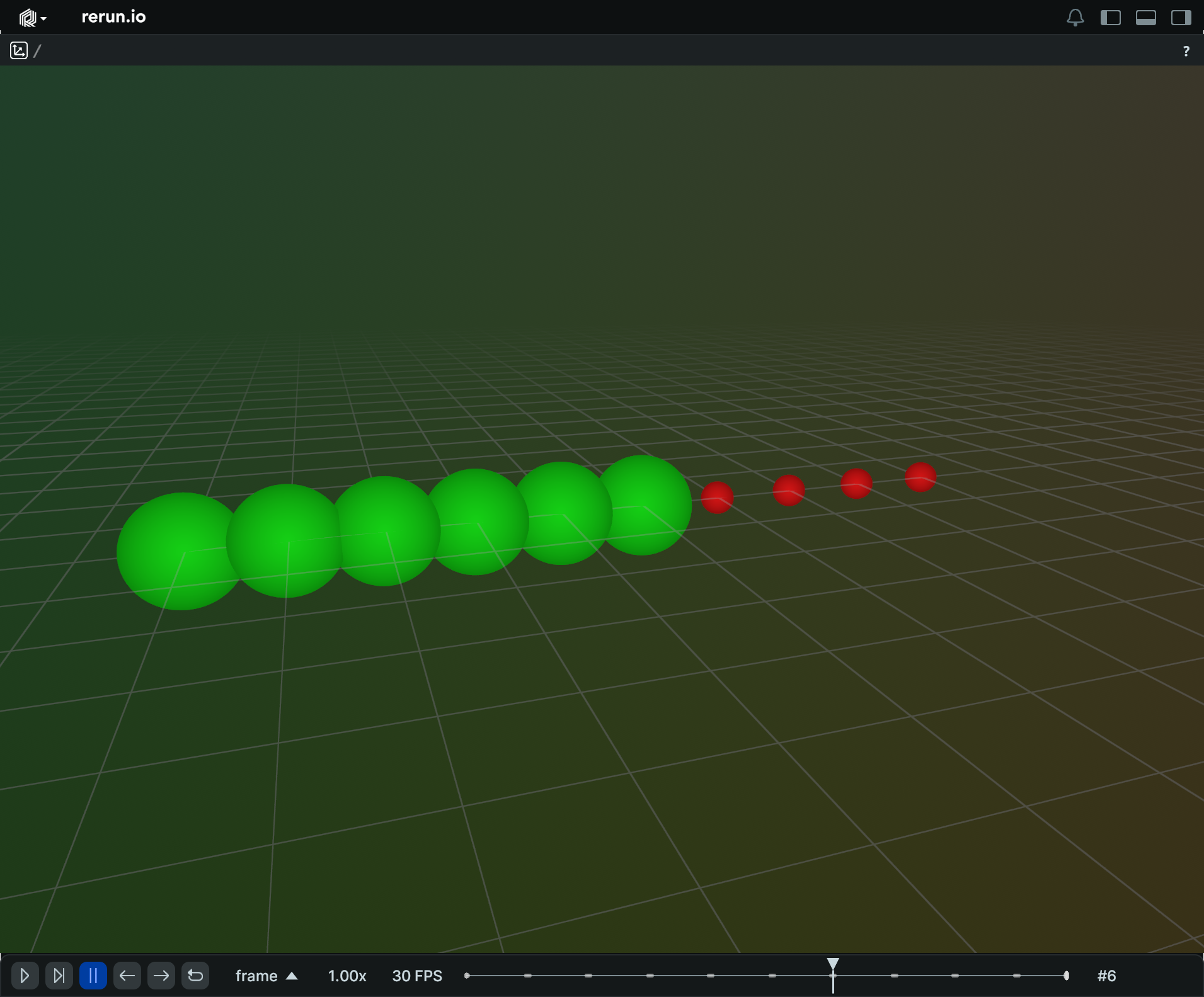
#include <rerun.hpp>
#include <algorithm>
#include <vector>
int main() {
rec.spawn().exit_on_failure();
for (int i = 0; i <10; ++i) {
positions.emplace_back(
static_cast<float>(i), 0.0f, 0.0f);
}
rec.set_time_sequence("frame", 0);
for (int i = 0; i <10; ++i) {
std::vector<rerun::Color>
colors;
for (int n = 0; n <10; ++n) {
if (n <i) {
} else {
}
}
std::vector<rerun::Radius>
radii;
for (int n = 0; n <10; ++n) {
if (n <i) {
} else {
}
}
rec.set_time_sequence("frame", i);
}
std::vector<rerun::Radius>
radii;
radii.emplace_back(0.3f);
rec.set_time_sequence("frame", 20);
}
Component: An RGBA color with unmultiplied/separate alpha, in sRGB gamma space with linear alpha.
Definition color.hpp:18
Component: The radius of something, e.g.
Definition radius.hpp:22